How to Overload Assignment Operator in C#
- How to Overload Assignment Operator in …

Operator Overloading in C#
Use implicit conversion operators to implement assignment operator overloading in c#, c# overload assignment operator using a copy constructor, use the op_assignment method to overload the assignment operator in c#, define a property to overload assignment operator in c#.
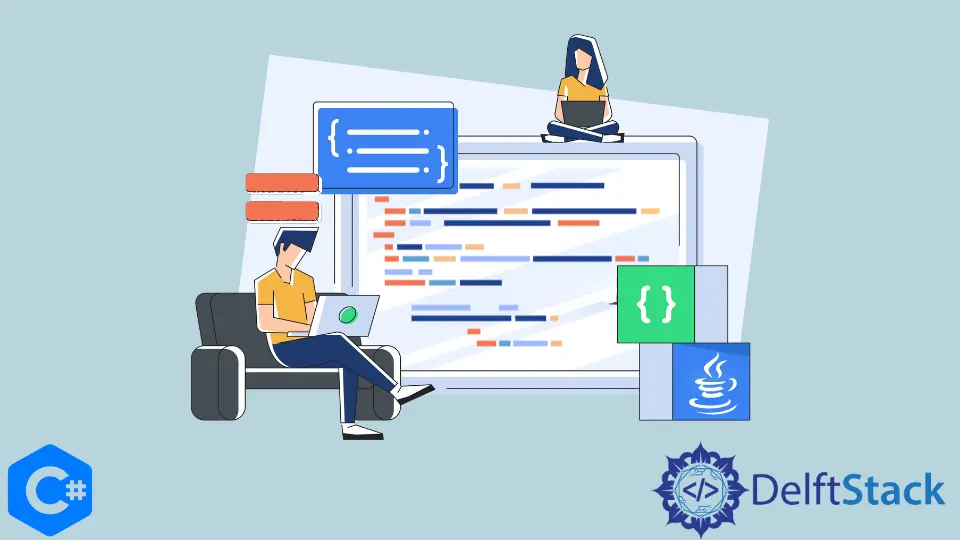
In C#, the assignment operator ( = ) is fundamental for assigning values to variables.
However, when working with custom classes, you might want to define your behavior for the assignment operation. This process is known as overloading the assignment operator.
In this article, we will explore different methods on how to overload assignment operators using C#, providing detailed examples for each approach. Let’s first have a look at operator overloading in C#.
A method for the redefinition of a built-in operator is called operator overloading. When one or both operands are of the user-defined type, we can create user-defined implementations of many different operations.
Operator overloading in C# allows you to define custom behavior for operators when working with objects of your classes.
It provides a way to extend the functionality of operators beyond their default behavior for built-in types. This can lead to more intuitive and expressive code when dealing with custom data types.
Operators in C# are symbols that perform operations on variables and values. The basic syntax for operator overloading involves defining a special method for the operator within your class.
The method must be marked with the operator keyword followed by the operator you want to overload. Here is an example of overloading the + operator for a custom class:
In this example, the + operator is overloaded to add two instances of CustomClass together. The operator + method takes two parameters of the same type and returns a new instance with the sum of their values.
You can achieve assignment overloading by using implicit conversion operators. This method allows you to define how instances of your class can be implicitly converted to each other.
Implicit conversion operations can be developed. Making them immutable structs is another smart move.
The primitives are just that, and that is what makes it impossible to inherit from them. We’ll develop an implicit conversion operator in the example below, along with addition and other operators.
To begin, import the following libraries:
We’ll create a struct named Velocity and create a double variable called value .
Instruct Velocity to create a public Velocity receiving a variable as a parameter.
Now, we’ll create an implicit operator, Velocity .
Then, we’ll create the Addition and Subtraction operators to overload.
Lastly, in the Main() method, we’ll call the object of Velocity to overload.
Complete Source Code:
This code defines a Velocity struct with custom operators for addition and subtraction, allowing instances of the struct to be created from double values and supporting arithmetic operations. The Example class showcases the usage of this struct with implicit conversion and overloaded operators.
Another approach is to overload the assignment operator by using a copy constructor. This method involves creating a new instance of the class and copying the values from the provided object.
A copy constructor is a special type of constructor that creates a new object by copying the values of another object of the same type. It enables the creation of a deep copy, ensuring that the new object is an independent instance with the same values as the original object.
In the context of overloading the assignment operator, a copy constructor serves as an effective alternative.
In this example, the CustomClass has a private value field, a constructor to initialize it, and a copy constructor that allows us to create a new object by copying the values from an existing object.
In this scenario, obj2 is created by invoking the copy constructor with new CustomClass(obj1) . As a result, obj2 becomes a distinct object with the same values as obj1 .
This approach effectively emulates the behavior of an overloaded assignment operator.
The most common approach to overload the assignment operator is by defining a method named op_Assignment within your class. This method takes two parameters - the object being assigned to ( this ) and the value being assigned.
The op_Assignment method is a user-defined method that allows developers to customize the behavior of the assignment operator for their own types. By defining this method within a class, you can control how instances of that class are assigned values.
Consider the following CustomClass with an op_Assignment method:
In this code, the CustomClass has a private value field, a constructor to initialize it, and an op_Assignment method that allows us to customize the behavior of the assignment operator.
The obj1.op_Assignment(obj2) triggers the op_Assignment method, customizing the behavior of the assignment operator and copying the values from obj2 to obj1 .
Overloading the assignment operator using a property involves defining a property with both a getter and a setter. The setter, in this case, will be responsible for customizing the assignment behavior.
This approach provides a clean and concise syntax for assignments, making the code more readable.
Consider the following CustomClass with a property named AssignValue :
In this code, the CustomClass has a private value field, a constructor to initialize it, and a property named AssignValue that will be used to overload the assignment operator.
The obj1.AssignValue = obj2.AssignValue; triggers the setter of the AssignValue property, customizing the behavior of the assignment and copying the values from obj2 to obj1 .
In conclusion, while direct overloading of the assignment operator is not directly supported in C#, these alternative methods provide powerful and flexible ways to achieve similar functionality. Choosing the appropriate method depends on the specific requirements and design considerations of your project, and each approach offers its advantages in terms of readability, control, and encapsulation.
By mastering these techniques, developers can enhance the expressiveness and maintainability of their code when dealing with user-defined types in C#.
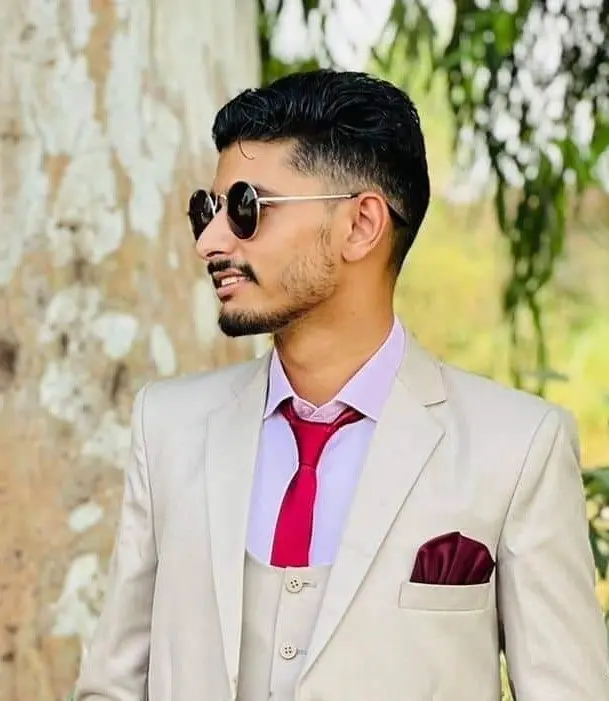
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
Related Article - Csharp Operator
- Modulo Operator in C#
- The Use of += in C#
- C# Equals() vs ==
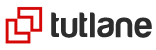
C# Assignment Operators with Examples
In c#, Assignment Operators are useful to assign a new value to the operand, and these operators will work with only one operand.
For example, we can declare and assign a value to the variable using the assignment operator ( = ) like as shown below.
If you observe the above sample, we defined a variable called “ a ” and assigned a new value using an assignment operator ( = ) based on our requirements.
The following table lists the different types of operators available in c# assignment operators.
Operator | Name | Description | Example |
---|---|---|---|
= | Equal to | It is used to assign the values to variables. | int a; a = 10 |
+= | Addition Assignment | It performs the addition of left and right operands and assigns a result to the left operand. | a += 10 is equals to a = a + 10 |
-= | Subtraction Assignment | It performs the subtraction of left and right operands and assigns a result to the left operand. | a -= 10 is equals to a = a - 10 |
*= | Multiplication Assignment | It performs the multiplication of left and right operands and assigns a result to the left operand. | a *= 10 is equals to a = a * 10 |
/= | Division Assignment | It performs the division of left and right operands and assigns a result to the left operand. | a /= 10 is equals to a = a / 10 |
%= | Modulo Assignment | It performs the modulo operation on two operands and assigns a result to the left operand. | a %= 10 is equals to a = a % 10 |
&= | Bitwise AND Assignment | It performs the Bitwise AND operation on two operands and assigns a result to the left operand. | a &= 10 is equals to a = a & 10 |
|= | Bitwise OR Assignment | It performs the Bitwise OR operation on two operands and assigns a result to the left operand. | a |= 10 is equals to a = a | 10 |
^= | Bitwise Exclusive OR Assignment | It performs the Bitwise XOR operation on two operands and assigns a result to the left operand. | a ^= 10 is equals to a = a ^ 10 |
>>= | Right Shift Assignment | It moves the left operand bit values to the right based on the number of positions specified by the second operand. | a >>= 2 is equals to a = a >> 2 |
<<= | Left Shift Assignment | It moves the left operand bit values to the left based on the number of positions specified by the second operand. | a <<= 2 is equals to a = a << 2 |
C# Assignment Operators Example
Following is the example of using assignment Operators in the c# programming language.
If you observe the above example, we defined a variable or operand “ x ” and assigning new values to that variable by using assignment operators in the c# programming language.
Output of C# Assignment Operators Example
When we execute the above c# program, we will get the result as shown below.
This is how we can use assignment operators in c# to assign new values to the variable based on our requirements.
Table of Contents
- Assignment Operators in C# with Examples
- C# Assignment Operator Example
- Output of C# Assignment Operator Example
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
operator-overloading.md
Latest commit, file metadata and controls.
title | description | ms.date | f1_keywords | helpviewer_keywords |
---|---|---|---|---|
Operator overloading - predefined unary, arithmetic, equality and comparison operators
A user-defined type can overload a predefined C# operator. That is, a type can provide the custom implementation of an operation in case one or both of the operands are of that type. The Overloadable operators section shows which C# operators can be overloaded.
Use the operator keyword to declare an operator. An operator declaration must satisfy the following rules:
- It includes both a public and a static modifier.
- A unary operator has one input parameter. A binary operator has two input parameters. In each case, at least one parameter must have type T or T? where T is the type that contains the operator declaration.
The following example defines a simplified structure to represent a rational number. The structure overloads some of the arithmetic operators :
[!code-csharp fraction example ]
You could extend the preceding example by defining an implicit conversion from int to Fraction . Then, overloaded operators would support arguments of those two types. That is, it would become possible to add an integer to a fraction and obtain a fraction as a result.
You also use the operator keyword to define a custom type conversion. For more information, see User-defined conversion operators .
Overloadable operators
The following table shows the operators that can be overloaded:
Operators | Notes |
---|---|
, , , , , , , | The and operators must be overloaded together. |
, , , , , , , , , , | |
, , , , , | Must be overloaded in pairs as follows: and , and , and . |
Non overloadable operators
The following table shows the operators that can't be overloaded:
Operators | Alternatives |
---|---|
, | Overload both the and operators and the or operators. For more information, see . |
, | Define an . |
Define custom type conversions that can be performed by a cast expression. For more information, see . | |
, , , , , , , , , , | Overload the corresponding binary operator. For example, when you overload the binary operator, is implicitly overloaded. |
, , , , , , , , , , , , , , , , , , , , , , , , | None. |
C# language specification
For more information, see the following sections of the C# language specification :
- Operator overloading
- C# operators and expressions
- User-defined conversion operators
- Design guidelines - Operator overloads
- Design guidelines - Equality operators
- Why are overloaded operators always static in C#?
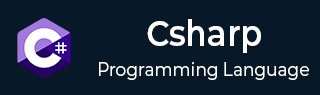
- C# Basic Tutorial
- C# - Overview
- C# - Environment
- C# - Program Structure
- C# - Basic Syntax
- C# - Data Types
- C# - Type Conversion
- C# - Variables
- C# - Constants
- C# - Operators
- C# - Decision Making
- C# - Encapsulation
- C# - Methods
- C# - Nullables
- C# - Arrays
- C# - Strings
- C# - Structure
- C# - Classes
- C# - Inheritance
- C# - Polymorphism
- C# - Operator Overloading
- C# - Interfaces
- C# - Namespaces
- C# - Preprocessor Directives
- C# - Regular Expressions
- C# - Exception Handling
- C# - File I/O
- C# Advanced Tutorial
- C# - Attributes
- C# - Reflection
- C# - Properties
- C# - Indexers
- C# - Delegates
- C# - Events
- C# - Collections
- C# - Generics
- C# - Anonymous Methods
- C# - Unsafe Codes
- C# - Multithreading
- C# Useful Resources
- C# - Questions and Answers
- C# - Quick Guide
- C# - Useful Resources
- C# - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
C# - Assignment Operators
There are following assignment operators supported by C# −
Operator | Description | Example |
---|---|---|
= | Simple assignment operator, Assigns values from right side operands to left side operand | C = A + B assigns value of A + B into C |
+= | Add AND assignment operator, It adds right operand to the left operand and assign the result to left operand | C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator, It subtracts right operand from the left operand and assign the result to left operand | C -= A is equivalent to C = C - A |
*= | Multiply AND assignment operator, It multiplies right operand with the left operand and assign the result to left operand | C *= A is equivalent to C = C * A |
/= | Divide AND assignment operator, It divides left operand with the right operand and assign the result to left operand | C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator, It takes modulus using two operands and assign the result to left operand | C %= A is equivalent to C = C % A |
<<= | Left shift AND assignment operator | C <<= 2 is same as C = C << 2 |
>>= | Right shift AND assignment operator | C >>= 2 is same as C = C >> 2 |
&= | Bitwise AND assignment operator | C &= 2 is same as C = C & 2 |
^= | bitwise exclusive OR and assignment operator | C ^= 2 is same as C = C ^ 2 |
|= | bitwise inclusive OR and assignment operator | C |= 2 is same as C = C | 2 |
The following example demonstrates all the assignment operators available in C# −
When the above code is compiled and executed, it produces the following result −
C# operator
last modified July 5, 2023
Expressions are constructed from operands and operators. The operators of an expression indicate which operations to apply to the operands. The order of evaluation of operators in an expression is determined by the precedence and associativity of the operators.
An operator is a special symbol which indicates a certain process is carried out. Operators in programming languages are taken from mathematics. Programmers work with data. The operators are used to process data. An operand is one of the inputs (arguments) of an operator.
C# operator list
The following table shows a set of operators used in the C# language.
Category | Symbol |
---|---|
Sign operators | |
Arithmetic | |
Logical (boolean and bitwise) | |
String concatenation | |
Increment, decrement | |
Shift | |
Relational | |
Assignment | |
Member access | |
Indexing | |
Cast | |
Ternary | |
Delegate concatenation and removal | |
Object creation | |
Type information | |
Overflow exception control | |
Indirection and address | |
Lambda |
An operator usually has one or two operands. Those operators that work with only one operand are called unary operators . Those who work with two operands are called binary operators . There is also one ternary operator ?: , which works with three operands.
Certain operators may be used in different contexts. For example the + operator. From the above table we can see that it is used in different cases. It adds numbers, concatenates strings or delegates; indicates the sign of a number. We say that the operator is overloaded .
C# unary operators
C# unary operators include: +, -, ++, --, cast operator (), and negation !.
C# sign operators
There are two sign operators: + and - . They are used to indicate or change the sign of a value.
The + and - signs indicate the sign of a value. The plus sign can be used to indicate that we have a positive number. It can be omitted and it is mostly done so.
The minus sign changes the sign of a value.
C# increment and decrement operators
The above two pairs of expressions do the same.
In the above example, we demonstrate the usage of both operators.
C# explicit cast operator
The explicit cast operator () can be used to cast a type to another type. Note that this operator works only on certain types.
In the example, we explicitly cast a float type to int .
Negation operator
In the example, we build a negative condition: it is executed if the inverse of the expression is valid.
C# assignment operator
The assignment operator = assigns a value to a variable. A variable is a placeholder for a value. In mathematics, the = operator has a different meaning. In an equation, the = operator is an equality operator. The left side of the equation is equal to the right one.
The previous expression does not make sense in mathematics. But it is legal in programming. The expression adds 1 to the x variable. The right side is equal to 2 and 2 is assigned to x .
C# concatenating strings
The + operator is also used to concatenate strings.
C# arithmetic operators
Symbol | Name |
---|---|
Addition | |
Subtraction | |
Multiplication | |
/ | Division |
Remainder |
In the preceding example, we use addition, subtraction, multiplication, division, and remainder operations. This is all familiar from the mathematics.
In this code, we have done integer division. The returned value of the division operation is an integer. When we divide two integers the result is an integer.
C# Boolean operators
Symbol | Name |
---|---|
logical and | |
logical or | |
negation |
Boolean operators are also called logical.
Many expressions result in a boolean value. Boolean values are used in conditional statements.
Relational operators always result in a boolean value. These two lines print false and true.
The body of the if statement is executed only if the condition inside the parentheses is met. The y > x returns true, so the message "y is greater than x" is printed to the terminal.
Example shows the logical and operator. It evaluates to true only if both operands are true.
Three of four expressions result in true.
The || , and && operators are short circuit evaluated. Short circuit evaluation means that the second argument is only evaluated if the first argument does not suffice to determine the value of the expression: when the first argument of the logical and evaluates to false, the overall value must be false; and when the first argument of logical or evaluates to true, the overall value must be true. Short circuit evaluation is used mainly to improve performance.
An example may clarify this a bit more.
In the second case, we use the || operator and use the Two method as the first operand. In this case, "Inside two" and "Pass" strings are printed to the terminal. It is again not necessary to evaluate the second operand, since once the first operand evaluates to true , the logical or is always true .
C# relational operators
Relational operators are used to compare values. These operators always result in boolean value.
Symbol | Meaning |
---|---|
less than | |
less than or equal to | |
greater than | |
greater than or equal to | |
equal to | |
not equal to |
In the code example, we have four expressions. These expressions compare integer values. The result of each of the expressions is either true or false. In C# we use == to compare numbers. Some languages like Ada, Visual Basic, or Pascal use = for comparing numbers.
C# bitwise operators
Decimal numbers are natural to humans. Binary numbers are native to computers. Binary, octal, decimal, or hexadecimal symbols are only notations of the same number. Bitwise operators work with bits of a binary number. Bitwise operators are seldom used in higher level languages like C#.
Symbol | Meaning |
---|---|
bitwise negation | |
bitwise exclusive or | |
bitwise and | |
bitwise or |
The bitwise negation operator changes each 1 to 0 and 0 to 1.
The bitwise and operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 only if both corresponding bits in the operands are 1.
The bitwise exclusive or operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 if one or the other (but not both) of the corresponding bits in the operands is 1.
C# compound assignment operators
The compound assignment operators consist of two operators. They are shorthand operators.
The a variable is initiated to one. 1 is added to the variable using the non-shorthand notation.
Using a += compound operator, we add 5 to the a variable. The statement is equal to a = a + 5; .
C# new operator
The new operator is used to create objects and invoke constructors.
C# access operator
The access operator [] is used with arrays, indexers, and attributes.
A dictionary is created. With domains["de"] , we get the value of the pair that has the "de" key.
C# index from end operator ^
The index from end operator ^ indicates the element position from the end of a sequence. For instance, ^1 points to the last element of a sequence and ^n points to the element with offset length - n .
In the example, we apply the operator on an array and a string.
We print the last letter of the word.
C# range operator ..
The .. operator specifies the start and end of a range of indices as its operands. The left-hand operand is an inclusive start of a range. The right-hand operand is an exclusive end of a range.
We create an array slice from index 1 till index 4; the last index 4 is not included.
C# type information
We use the sizeof and typeof operators.
The is operator checks if an object is compatible with a given type.
We create two objects from user defined types.
We have a Base and a Derived class. The Derived class inherits from the Base class.
The derived object is compatible with the Base class because it explicitly inherits from the Base class. On the other hand, the _base object has nothing to do with the Derived class.
The as operator is used to perform conversions between compatible reference types. When the conversion is not possible, the operator returns null. Unlike the cast operation which raises an exception.
We try to cast various types to the string type. But only once the casting is valid.
C# operator precedence
Like in mathematics, the multiplication operator has a higher precedence than addition operator. So the outcome is 28.
The following table shows common C# operators ordered by precedence (highest precedence first):
Operator(s) | Category | Associativity |
---|---|---|
Primary | Left | |
Unary | Left | |
Multiplicative | Left | |
Additive | Left | |
Shift | Left | |
Equality | Right | |
Logical AND | Left | |
Logical XOR | Left | |
Logical OR | Left | |
Conditional AND | Left | |
Conditional OR | Left | |
Null Coalescing | Left | |
Ternary | Right | |
Assignment | Right |
Operators on the same row of the table have the same precedence.
In this case, the negation operator has a higher precedence. First, the first true value is negated to false, then the | operator combines false and true, which gives true in the end.
C# associativity rule
Arithmetic, boolean, relational, and bitwise operators are all left to right associated.
The compound assignment operators are right to left associated. We might expect the result to be 1. But the actual result is 0. Because of the associativity. The expression on the right is evaluated first and than the compound assignment operator is applied.
C# null-conditional operator
A null-conditional operator applies a member access, ?. , or element access, ?[] , operation to its operand only if that operand evaluates to non-null. If the operand evaluates to null , the result of applying the operator is null.
We have a list of users. One of them is initialized with null values.
We use the ?. to access the Name member and call the ToUpper method. The ?. prevents the System.NullReferenceException by not calling the ToUpper on the null value.
In this example, we have a null value in an array. We prevent the System.NullReferenceException by applying the ?. operator on the array elements.
C# null-coalescing operator
The null-coalescing operator ?? is used to define a default value for a nullable type. It returns the left-hand operand if it is not null; otherwise it returns the right operand. When we work with databases, we often deal with absent values. These values come as nulls to the program. This operator is a convenient way to deal with such situations.
We want to assign a value to z variable. But it must not be null . This is our requirement. We can easily use the null-coalescing operator for that. In case both x and y variables are null, we assign -1 to z .
C# null-coalescing assignment operator
First, the list is assigned to null .
We use the ??= to assign a new list object to the variable. Since it is null , the list is assigned.
C# ternary operator
The ternary operator ?: is a conditional operator. It is a convenient operator for cases where we want to pick up one of two values, depending on the conditional expression.
In most countries the adulthood is based on your age. You are adult if you are older than a certain age. This is a situation for a ternary operator.
First the expression on the right side of the assignment operator is evaluated. The first phase of the ternary operator is the condition expression evaluation. So if the age is greater or equal to 18, the value following the ? character is returned. If not, the value following the : character is returned. The returned value is then assigned to the adult variable.
C# Lambda operator
Wherever we can use a delegate, we also can use a lambda expression. A definition for a lambda expression is: a lambda expression is an anonymous function that can contain expressions and statements. On the left side we have a group of data and on the right side an expression or a block of statements. These statements are applied on each item of the data.
In lambda expressions we do not have a return keyword. The last statement is automatically returned. And we do not need to specify types for our parameters. The compiler will guess the correct parameter type. This is called type inference.
The compiler will expect an int type. The val is a current input value from the list. It is compared if it is greater than 3 and a boolean true or false is returned. Finally, the FindAll will return all values that met the condition. They are assigned to the sublist collection.
The items of the sublist collection are printed to the terminal.
This is the same example. We use a anonymous delegate instead of a lambda expression.
C# calculating prime numbers
In the above example, we deal with many various operators. A prime number (or a prime) is a natural number that has exactly two distinct natural number divisors: 1 and itself. We pick up a number and divide it by numbers, from 1 up to the picked up number. Actually, we do not have to try all smaller numbers; we can divide by numbers up to the square root of the chosen number. The formula will work. We use the remainder division operator.
By definition, 1 is not a prime
We skip the calculations for 2 and 3: they are primes. Note the usage of the equality and conditional or operators. The == has a higher precedence than the || operator. So we do not need to use parentheses.
We are OK if we only try numbers smaller than the square root of a number in question. It was mathematically proven that it is sufficient to take into account values up to the square root of the number in question.
This is a while loop. The i is the calculated square root of the number. We use the decrement operator to decrease the i by one each loop cycle. When the i is smaller than 1, we terminate the loop. For example, we have number 9. The square root of 9 is 3. We divide the 9 number by 3 and 2.
C# operators and expressions
In this article we covered C# operators.
My name is Jan Bodnar and I am a passionate programmer with many years of programming experience. I have been writing programming articles since 2007. So far, I have written over 1400 articles and 8 e-books. I have over eight years of experience in teaching programming.
List all C# tutorials .
Luis Llamas
Engineering, computing, design
Assignment Operators in C#
Assignment operators allow us to assign values to variables.
The most common assignment operator is the = operator. This operator is used to assign the value on the right to the variable on the left.
In addition to the basic assignment operator (=), there are compound assignment operators that combine an arithmetic operation with the assignment, simplifying and optimizing the code.
Assignment operators in C# include:
- = (Assignment): Assigns a value to a variable.
- += (Add and Assign): Adds the value to the variable and assigns the result to the variable.
- -= (Subtract and Assign): Subtracts the value from the variable and assigns the result to the variable.
- *= (Multiply and Assign): Multiplies the value of the variable and assigns the result to the variable.
- /= (Divide and Assign): Divides the value of the variable and assigns the result to the variable.
- %= (Modulo and Assign): Calculates the modulo of the value of the variable and assigns the result to the variable.
Do not confuse the assignment operator = with the equality operator == :::
List of assignment operators
Assignment (=).
The basic assignment operator ( = ) is used to assign a value to a variable. For example:
The assignment operator is also used to update the value of a variable. For example:
Subtract and Assign (-=)
The -= operator subtracts a value from a variable and assigns the result to that variable. For example:
Multiply and Assign (*=)
The *= operator multiplies a variable by a value and assigns the result to that variable. For example:
Divide and Assign (/=)
The /= operator divides a variable by a value and assigns the result to that variable. For example:
Modulo and Assign (%=)
The %= operator calculates the modulo of a variable by a value and assigns the result to that variable. For example:
Operator precedence
It is important to remember that assignment operators have a lower precedence than most other operators in C#. Therefore, expressions on the right side of assignment operators are evaluated first.
For example:
On this page
C# Tutorial
C# examples, c# assignment operators, assignment operators.
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
Try it Yourself »
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:
Operator | Example | Same As | Try it |
---|---|---|---|
= | x = 5 | x = 5 | |
+= | x += 3 | x = x + 3 | |
-= | x -= 3 | x = x - 3 | |
*= | x *= 3 | x = x * 3 | |
/= | x /= 3 | x = x / 3 | |
%= | x %= 3 | x = x % 3 | |
&= | x &= 3 | x = x & 3 | |
|= | x |= 3 | x = x | 3 | |
^= | x ^= 3 | x = x ^ 3 | |
>>= | x >>= 3 | x = x >> 3 | |
<<= | x <<= 3 | x = x << 3 |

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]

Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
C# Assignment Operators
Symbol | Operation | Example |
---|---|---|
+= | Plus Equal To | x+=15 is x=x+15 |
-= | Minus Equal To | x- =15 is x=x-15 |
*= | Multiply Equal To | x*=16 is x=x+16 |
%= | Modulus Equal To | x%=15 is x=x%15 |
/= | Divide Equal To | x/=16 is x=x/16 |
C# Assignment Operators Example
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Why it is not possible to overload compound assignment operator in C#?
The title is be misleading, so please read entire question :-) .
By "compound assignment operator" I have in mind a construct like this op= , for example += . Pure assignment operator ( = ) does not belong to my question.
By "why" I don't mean an opinion, but resource (book, article, etc) when some of the designers, or their coworkers, etc. express their reasoning (i.e. the source of the design choice).
I am puzzled by asymmetry found in C++ and C# ( yes, I know C# is not C++ 2.0 ) -- in C++ you would overload operator += and then almost automatically write appropriate + operator relying on previously defined operator. In C# it is in reverse -- you overload + and += is synthesised for you.
If I am not mistaken the later approach kills a chance for optimization in case of actual += , because new object has to be created. So there has to be some big advantage of such approach, but MSDN is too shy to tell about it.
And I wonder what that advantage is -- so if you spotted explanation in some C# book, tech-talk video, blog entry, I will be grateful for the reference.
The closest thing I found is a comment on Eric Lippert blog Why are overloaded operators always static in C#? by Tom Brown. If the static overloading was decided first it simply dictates which operators can be overloaded for structs. This further dictates what can be overloaded for classes.
- language-design

- 3 Do you have a link for the new C++ style? Naively, overloading += first seems absurd; why would you overload a combined operation rather than the parts of it? – Telastyn Commented May 30, 2015 at 16:18
- 1 I believe the term for these is "compound assignment operators". – Ixrec Commented May 30, 2015 at 16:30
- 2 @greenoldman Telastyn was probably implying that implementing += in terms of + seems more natural than the other way around, since semantically += is a composition of + and =. To the best of my knowledge , having + call += is largely an optimization trick. – Ixrec Commented May 30, 2015 at 16:33
- 1 @Ixrec: Sometimes, a+=b makes sense, while a=a+b doesn't: Consider that identity might be important, an A cannot be duplicated, whatever. Sometimes, a=a+b makes sense, while a+=b doesn't: Consider immutable strings. So, one actually needs the ability to decide which one to overload separately. Of course, auto-generating the missing one, if all neccessary building-blocks exist and it's not explicitly disables, is a good idea. Not that C# allows that atm, afaik. – Deduplicator Commented May 30, 2015 at 16:40
- 4 @greenoldman - I understand that motivation, but personally, I would find *= mutating a reference type to be semantically incorrect. – Telastyn Commented May 30, 2015 at 17:52
I can't find the reference for this, but my understanding was that the C# team wanted to provide a sane subset of operator overloading. At the time, operator overloading had a bad rap. People asserted that it obfuscated code, and could only be used for evil. By the time C# was being designed, Java had shown us that no operator overloading was kind of annoying.
So C# wanted to balance operator overloading so that it was more difficult to do evil, but you could make nice things too. Changing the semantics of assignment was one of those things that was deemed always evil. By allowing += and its kin to be overloaded, it would allow that sort of thing. For example, if += mutated the reference rather than making a new one, it wouldn't follow the expected semantics, leading to bugs.

- Thank you, if you don't mind I will keep this question open bit longer, and if nobody beat you with the reasoning of actual design, I will close it with accepting yours. Once again -- thank you. – greenoldman Commented May 30, 2015 at 18:29
- @greenoldman - as well you should. I too hope someone come with a answer with more concrete references. – Telastyn Commented May 30, 2015 at 20:04
- So, this is a place where the impossibility of talking both about the pointer and the pointee comfortably and unambiguously rears it's head? Damn shame. – Deduplicator Commented May 30, 2015 at 21:15
- "People asserted that it obfuscated code" - it's still true though, have you seen some of the F# libraries? Operator noise. E.g. quanttec.com/fparsec – Den Commented Jun 3, 2015 at 9:39
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Software Engineering Stack Exchange. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c# language-design operators or ask your own question .
- Featured on Meta
- We spent a sprint addressing your requests — here’s how it went
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
Hot Network Questions
- Infinity is not a number
- Translation of the two lines of Latin in Shakespear's Venus and Adonis
- Why the number of bits or bytes is different for a folder that has been copied between two external drives?
- Adjusting the indentation and the horizontal spacing in tables in latex
- Coincidence between coefficients of tanh(tan(x/2)) and Chow ring computations?
- Does the damage from Thunderwave occur before or after the target is moved
- Where can I find a complete archive of audio recordings of Hitler speeches?
- Equivalence of first/second choice with naive probability - I don't buy it
- 8x8 grid with no unmarked L-pentomino
- Real-life problems involving solving triangles
- What is the computational scaling of DFT energy vs gradient vs Hessian?
- How can I search File Explorer for files only (i.e. exclude folders) in Windows 10?
- Rolling median of all K-length ranges
- How can I export my Location History now that this data is only stored locally on the phone?
- Can I put multiple journeys and dates on one train ticket in UK?
- Finding the Zeckendorf Representation of a Positive Integer (BIO 2023 Q1)
- Reproducing Ruth Vollmer's acrylic Steiner surface
- What was Korach's argument?
- What real-world cultures inspired the various human name examples?
- How can I watch a timelapse movie on the Nikon D7100?
- What enforcement exists for medical informed consent?
- When, if ever, is bribery legal?
- What caused the builder to change plans midstream on this 1905 library in New England?
- Fill the grid subject to product, sum and knight move constraints
C# Assignment Operators
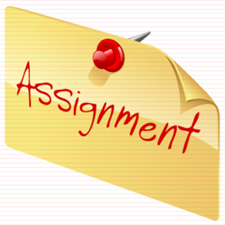
- What is C# Assignment Operators?
- How many types of assignment operators in C sharp?
- How to use assignment operator in program?
The C# assignment operator is generally suffix with arithmetic operators. The symbol of c sharp assignment operator is "=" without quotes. The assignment operator widely used with C# programming. Consider a simple example:
result=num1+num2;
In this example, the equal to (=) assignment operator assigns the value of num1 + num2 into result variable.
Various types of C# assignment operators are mentioned below:
Assignment Operators:
Assignment Operators | Usage | Examples |
---|---|---|
(Equal to) | result=5 | Assign the value 5 for result |
(Plus Equal to) | result+=5 | Same as result=result+5 |
(Minus Equal to) | result-=5 | Same as result=result-5 |
(Multiply Equal to) | result*=5 | Same as result=result*5 |
(Divide Equal to) | result/=5 | Same as result=result/5 |
(Modulus Equal to) | result%=5 | Same as result=result%5 |
In this chapter, you learned about different types of assignment operators in C# . You also learned how to use these assignment operators in a program. In next chapter, you will learn about Unary Operator in C# .
Share your thought
Complete Csharp Tutorial
Please support us by enabling ads on this page. Refresh YOU DON'T LIKE ADS, WE ALSO DON'T LIKE ADS! But we have to show ads on our site to keep it free and updated . We have to pay huge server costs, domain costs, CDN Costs, Developer Costs, Electricity and Internet Bill . Your little contribution will encourage us to regularly update this site.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
C# operators and expressions
- 13 contributors
C# provides a number of operators. Many of them are supported by the built-in types and allow you to perform basic operations with values of those types. Those operators include the following groups:
- Arithmetic operators that perform arithmetic operations with numeric operands
- Comparison operators that compare numeric operands
- Boolean logical operators that perform logical operations with bool operands
- Bitwise and shift operators that perform bitwise or shift operations with operands of the integral types
- Equality operators that check if their operands are equal or not
Typically, you can overload those operators, that is, specify the operator behavior for the operands of a user-defined type.
The simplest C# expressions are literals (for example, integer and real numbers) and names of variables. You can combine them into complex expressions by using operators. Operator precedence and associativity determine the order in which the operations in an expression are performed. You can use parentheses to change the order of evaluation imposed by operator precedence and associativity.
In the following code, examples of expressions are at the right-hand side of assignments:
Typically, an expression produces a result and can be included in another expression. A void method call is an example of an expression that doesn't produce a result. It can be used only as a statement , as the following example shows:
Here are some other kinds of expressions that C# provides:
Interpolated string expressions that provide convenient syntax to create formatted strings:
Lambda expressions that allow you to create anonymous functions:
Query expressions that allow you to use query capabilities directly in C#:
You can use an expression body definition to provide a concise definition for a method, constructor, property, indexer, or finalizer.
Operator precedence
In an expression with multiple operators, the operators with higher precedence are evaluated before the operators with lower precedence. In the following example, the multiplication is performed first because it has higher precedence than addition:
Use parentheses to change the order of evaluation imposed by operator precedence:
The following table lists the C# operators starting with the highest precedence to the lowest. The operators within each row have the same precedence.
Operators | Category or name |
---|---|
, , , , , , , , , , , , , , , , , | Primary |
, , , , , , , , , , , | Unary |
Range | |
, | and expressions |
, , | Multiplicative |
, | Additive |
, , | Shift |
, , , , , | Relational and type-testing |
, | Equality |
or | |
or | |
or | |
Conditional AND | |
Conditional OR | |
Null-coalescing operator | |
Conditional operator | |
, , , , , , , , , , , , , | Assignment and lambda declaration |
For information about the precedence of logical pattern combinators , see the Precedence and order of checking of logical patterns section of the Patterns article.
Operator associativity
When operators have the same precedence, associativity of the operators determines the order in which the operations are performed:
- Left-associative operators are evaluated in order from left to right. Except for the assignment operators and the null-coalescing operators , all binary operators are left-associative. For example, a + b - c is evaluated as (a + b) - c .
- Right-associative operators are evaluated in order from right to left. The assignment operators, the null-coalescing operators, lambdas, and the conditional operator ?: are right-associative. For example, x = y = z is evaluated as x = (y = z) .
In an expression of the form P?.A0?.A1 , if P is null , neither A0 nor A1 are evaluated. Similarly, in an expression of the form P?.A0.A1 , because A0 isn't evaluated when P is null, neither is A0.A1 . See the C# language specification for more details.
Use parentheses to change the order of evaluation imposed by operator associativity:
Operand evaluation
Unrelated to operator precedence and associativity, operands in an expression are evaluated from left to right. The following examples demonstrate the order in which operators and operands are evaluated:
Expression | Order of evaluation |
---|---|
a, b, + | |
a, b, c, *, + | |
a, b, /, c, d, *, + | |
a, b, c, +, /, d, * |
Typically, all operator operands are evaluated. However, some operators evaluate operands conditionally. That is, the value of the leftmost operand of such an operator defines if (or which) other operands should be evaluated. These operators are the conditional logical AND ( && ) and OR ( || ) operators, the null-coalescing operators ?? and ??= , the null-conditional operators ?. and ?[] , and the conditional operator ?: . For more information, see the description of each operator.
C# language specification
For more information, see the following sections of the C# language specification :
- Expressions
- Operator overloading
- Expression trees
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
C# in a Nutshell by Ben Albahari, Ted Neward, Peter Drayton
Get full access to C# in a Nutshell and 60K+ other titles, with a free 10-day trial of O'Reilly.
There are also live events, courses curated by job role, and more.
Operator Overloading
C# lets you overload operators to work with operands that are custom classes or structs using operators. An operator is a static method with the keyword operator preceding the operator to overload (instead of a method name), parameters representing the operands, and return types representing the result of an expression. Table 4-1 lists the available overloadable operators.
Table 4-1. Overloadable operators
Literals that also act as overloadable operators are true and false .
Implementing Value Equality
A pair of references exhibit referential equality when both references point to the same object. By default, the == and != operators will compare two reference-type variables by reference. However, it is occasionally more natural for the == and != operators to exhibit value equality, whereby the comparison is based on the value of the objects that the references point to.
Whenever overloading the == and != operators, you should always override the virtual Equals method to route its functionality to the == operator. This allows a class to be used polymorphically (which is essential if you want to take advantage of functionality such as the collection classes). It also provides compatibility with other .NET languages that don’t overload operators.
A good guideline for knowing whether to implement the == and != operators is if it is natural for the class to overload other operators too, such as < , > , + , or - ; otherwise, don’t bother — just implement the Equals method. For structs, overloading the == and != operators provides a more efficient implementation than the default one.
Logically Paired Operators
The C# compiler enforces operators that are logical pairs to both be defined. These operators are == != , < > , and <= >= .
Custom Implicit and Explicit Conversions
As explained in the discussion on types, the rationale behind implicit conversions is they are guaranteed to succeed and do not lose information during the conversion. Conversely, an explicit conversion is required either when runtime circumstances will determine whether the conversion will succeed or if information may be lost during the conversion. In this example, we define conversions between our musical Note type and a double (which represents the frequency in hertz of that note):
Three-State Logic Operators
The true and false keywords are used as operators when defining types with three-state logic to enable these types to work seamlessly with constructs that take boolean expressions — namely, the if , do , while , for , and conditional (?:) statements. The System.Data.SQLTypes.SQLBoolean struct provides this functionality:
Indirectly Overloadable Operators
The && and || operators are automatically evaluated from & and | , so they do not need to be overloaded. The [] operators can be customized with indexers (see Section 3.1.5 in Chapter 3 ). The assignment operator = cannot be overloaded, but all other assignment operators are automatically evaluated from their corresponding binary operators (e.g., += is evaluated from + ).
Get C# in a Nutshell now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.
Don’t leave empty-handed
Get Mark Richards’s Software Architecture Patterns ebook to better understand how to design components—and how they should interact.
It’s yours, free.
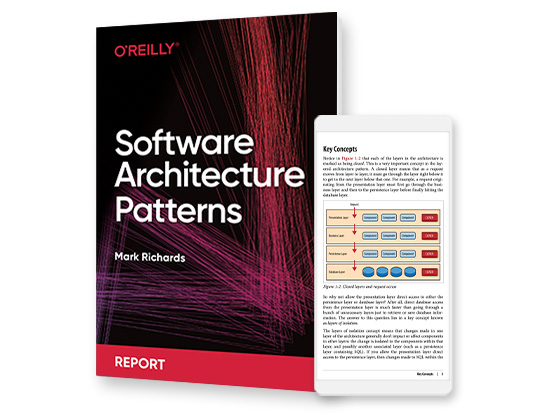
Check it out now on O’Reilly
Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
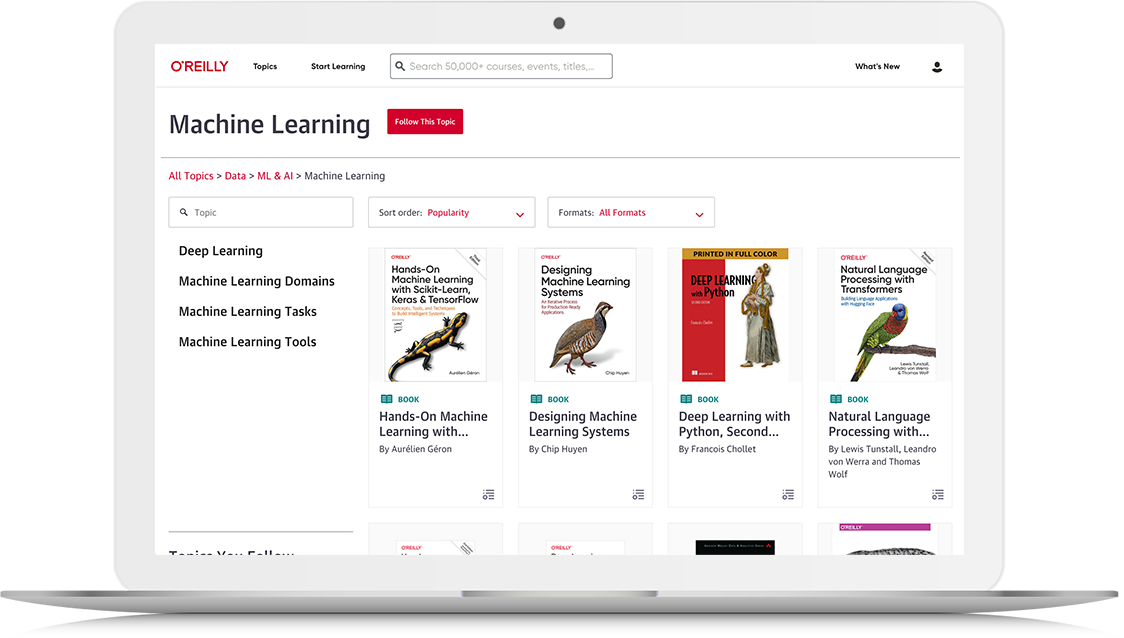
Get notified in your email when a new post is published to this blog
Refactor your code with C# collection expressions

May 8th, 2024 14 14
This post is the second in a series of posts covering various refactoring scenarios that explore C# 12 features. In this post, we’ll look at how you can refactor your code using collection expressions, we’ll learn about collection initializers, various expression usages, supported collection target types, and the spread syntax. Here’s how the series is shaping up:
- Refactor your C# code with primary constructors
- Refactor your C# code with collection expressions (this post)
- Refactor your C# code by aliasing any type
- Refactor your C# code to use default lambda parameters
These features continue our journey to make our code more readable and maintainable, and these are considered “Everyday C#” features that developers should know.
Collection Expressions 🎨
C# 12 introduced collection expressions that offer a simple and consistent syntax across many different collection types. When initializing a collection with a collection expression, the compiler generates code that is functionally equivalent to using a collection initializer. The feature emphasizes consistency , while allowing for the compiler to optimize the lowered C#. Of course, every team decides what new features to adopt, and you can experiment and introduce this new syntax if you like it, since all of the previous ways to initialize collections will continue to work.
With collections expressions elements appear inlined sequences of elements between an opening [ and closing ] bracket. Read on to hear more about how collection expressions work.
Initialization 🌱
C# provides many syntaxes for initializing different collections. Collection expressions replace all of these, so let’s start with a look at different ways you can initialize an array of integers like this:
All four versions are functionally equivalent, and the compiler generates identical code for each version. The last example is similar to the new collection expressions syntax. If you squint your eyes a bit, you could imagine the curly braces as { and } as square brackets [ and ] , then you’d be reading the new collection expression syntax. Collection expressions don’t use curly braces. This is to avoid ambiguity with existing syntax, especially { } to indicate any not-null in patterns.
The last example is the only to declare the type explicitly, instead of relying on var . The following example creates a List<char> :
Again, collection expressions cannot be used with the var keyword. You must declare the type because a collection expression doesn’t currently have a natural type and can be converted to a wide variety of collection types . Supporting assignment to var is still under consideration, but the team has not settled on the what the natural type should be. In other words, the C# compiler errors out with CS9176: There is no target type for the collection expression, when writing the following code:
You might be asking yourself, “with all these different approaches to initializing collections, why would I use the new collection expression syntax?” The answer is that with collection expressions, you can use the same syntax to express collections in a consistent way. This can help to make your code more readable and maintainable. We’ll explore more advantages in the coming sections.
Collection expression variations 🎭
You can express that a collection is empty , using the following syntax:
The empty collection expression initialization is a great replacement for code that was otherwise using the new keyword, as it’s optimized by the compiler to avoid allocating memory for some collection types. For example, when the collection type is an array T[] , the compiler generates an Array.Empty<T>() , which is more efficient than new int[] { } . Another shortcut is to use the number of elements in the collection expression to set the collection size, such as new List<int>(2) for List<T> x = [1, 2]; .
Collection expressions also allow you to assign to interfaces without stating an explicit type. The compiler determines the type to use for types, such as IEnumerable<T> , IReadOnlyList<T> , and IReadOnlyCollection<T> . If the actual type used is important, you’ll want to state it because this may change if more efficient types become available. Likewise, in situations where the compiler cannot generate more efficient code, for example when the collection type is a List<T> , the compiler generates a new List<int>() , which is then equivalent.
The advantages of using the empty collection expression are threefold:
- It provides a consistent means of initializing all collections, regardless of their target type.
- It allows the compiler to generate efficient code.
- It’s less code to write. For example, instead of writing Array.Empty<T>() or Enumerable.Empty<T>() , you can simply write [] .
A few more details about the efficient generated code: using the [] syntax generates known IL. This allows the runtime to optimize by reusing the storage for Array.Empty<T> (for each T ), or even more aggressively inline the code.
Empty collections serve their purpose, but you may need a collection that has some initial values. You can initialize a collection with a single element, using the following syntax:
Initializing a single element collection is similar to initializing a collection with more than a single element. You can initialize a collection with multiple elements by adding other literal values, using the following syntax:
A bit of history
Let’s look at another code sample, but this uses spread element , to include the elements of another collection, using the following syntax:
The spread element is a powerful feature that allows you to include the elements of another collection in the current collection. The spread element is a great way to combine collections in a concise way. The expression in a spread element must be enumerable ( foreach -able). For more information, see the Spread ✨ section.
Supported collection types 🎯
There are many target types that collection expressions can be used with. The feature recognizes the “shape” of a type that represents a collection. Therefore, most collections you’re familiar with are supported out-of-the-box. For types that don’t match that “shape” (mostly readonly collections), there are attributes you can apply to describe the builder pattern. The collection types in the BCL that needed the attributes/builder pattern approaches, have already been updated.
It’s unlikely that you’ll ever need to think about how target types are selected, but if you are curious about the rules see the C# Language Reference: Collection expressions—conversions .
Collection expressions don’t yet support dictionaries. You can find a proposal to extend the feature C# Feature Proposal: Dictionary expressions .
Refactoring scenarios 🛠️
Collection expressions can be useful in many scenarios, such as:
- properties.
- local variables.
- method parameters.
- return values.
- a coalescing expression as the final fallthrough to safely avoid exceptions.
- Passing arguments to methods that expect collection type parameters.
Let’s use this section to explore some sample usage scenarios, and consider potential refactoring opportunities. When you define a class or struct that contains fields and/or properties with non-nullable collection types, you can initialize them with collection expressions. For example, consider the following example ResultRegistry object:
In the preceding code, the result registry class contains a private _results field that is initialized with a new HashSet<Result>() constructor expression. In your IDE of choice (that supports these refactoring features), right-click on the new keyword, select Quick Actions and Refactorings... (or press Ctrl + . ), and choose Collection initialization can be simplified , as shown in the following video:
The code is updated to use the collection expression syntax, as shown in the following code:
The previous code, instantiated the HashSet<Result> with the new HashSet<Result>() constructor expression. However, in this case [] is identical.
Many popular programming languages such as Python and JavaScript/TypeScript, among others provide their variation of the spread syntax, which serves as a succinct way to work with collections. In C#, the spread element is the syntax used to express the concatenation of various collections into a single collection.
Proper terminology
So what exactly is spread element ? It takes the individual values from the collection being “spread” and places them in the destination collection at that position. The spread element functionality also comes with a refactoring opportunity. If you have code that calls .ToList or .ToArray , or you want to use eager evaluation, your IDE might be suggesting to use the spread element syntax instead. For example, consider the following code:
The preceding code could be refactored to use the spread element syntax, consider the following code that removes the .ToList method call, and uses an expression-bodied method as a bonus refactored version:
Span<T> and ReadOnlySpan<T> support 📏
Collection expressions support Span<T> and ReadOnlySpan<T> types that are used to represent a contiguous region of arbitrary memory. You benefit from the performance improvements they offer, even if you don’t use them directly in your code. Collection expressions allow the runtime to offer optimizations, especially where overloads using span can be selected when collection expressions are used as arguments.
You can also assign directly to span, if your application uses spans:
If you’re using the stackalloc keyword, there’s even a provided refactoring to use collection expressions. For example, consider the following code:
If you right-click on the stackalloc keyword, select Quick Actions and Refactorings... (or press Ctrl + . ), and choose Collection initialization can be simplified , as shown in the following video:
https://devblogs.microsoft.com/dotnet/wp-content/uploads/sites/10/2024/05/refactor-collection-ex.mp4
For more information, see Memory<T> and Span<T> usage guidelines .
Semantic considerations ⚙️
When initializing a collection with a collection expression, the compiler generates code that is functionally equivalent to using a collection initializer. Sometimes the generated code is much more efficient than using a collection initializer. Consider the following example:
The rules for a collection initializer require that the compiler call the Add method for each element in the initializer. However, if you’re to use the collection expression syntax:
The compiler generates code that instead uses AddRange , that might be faster or better optimized. The compiler is able to make these optimizations because it knows the target type of the collection expression.
Next steps 🚀
Be sure to try this out in your own code! Check back soon for the next post in this series, where we’ll explore how to refactor your C# code by aliasing any type. In the meantime, you can learn more about collection expressions in the following resources:
- C# Feature Proposal: Collection expressions
- C# Language Reference: Collection expressions
- C# Docs: Collections

David Pine Senior Content Developer, .NET
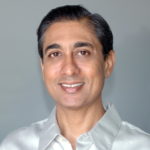
14 comments
Leave a comment cancel reply.
Log in to join the discussion or edit/delete existing comments.
This is all extremely nice!
This is a neat implementation of the Collection expression.
IMHO this is the annoying part of the feature Again, collection expressions cannot be used with the var keyword.
hope this will be addressed sooner then later, and if that’s all the reason behind it but the team has not settled on the what the natural type should be.
roll a dice if you can’t make up your mind. Or put up a poll and let majority decide.
I disagree. var support is not needed.
If anyone is wondering, the question is which is the correct choice when var is used: a) Default to the fastest and most efficient, such as Span or ReadOnlySpan b) Default to the one with the best developer experience, such as List
You can argue both cases, so it is just hard for everyone to reach agreement here.
I can’t think of a worse idea for software development than having the masses “vote” on how a feature should work. Especially a feature as bad as the var keyword. Support in this case would just result in lazy programmers declaring collection types whose implementation they aren’t even aware of.
Love or hate the collection expressions, but the use of emojis here is world class.
Regarding the LINQ example with the spread operator: does use of the collection expression result in the same call to .ToList() or is there some additional optimization going on? The original code could have been written as expression-bodied member as well, without declaring a local variable, so the new syntax isn’t much shorter and arguably the intent isn’t really clearer either. I wouldn’t use collection expressions in that case, unless perhaps it’s more efficient – hence the question.
(that aside, a very useful feature! the spread operator will replace a bunch of help methods in our code that were used to combine individual items and lists into a single list in an efficient manner, glad to let the compiler do that now)
I don’t know who decided to have the analyzers suggest the use of the morse code syntax instead of the much more clear .ToList(). I’m about to turn off the style rule to suggest collection initializers because of a couple of rules like that. At least split them in separate settings
This feature should come with a big warning: HERE BE DRAGONS
You don’t know if the compiler team has chosen the optimal code generation strategy or if the generated code is a fallback to just work. Best example is that on release this did generate the worst possible code for efcore DbSet .ToList(). I don’t know if this is already fixed.
I have a question on Spread .
I agree .. is not really an operator, just noticed Collection expressions (official documentation) is referring .. as spread operator.
It’s very important that we maintain correct terminology across.

Hi Jaliya, yes, you’re correct that we need to update the docs. Good catch. Thank you
This is beautiful, expressive, and performant. Thank you all.
You should really split the style options for this. I think your query example is terrible, having ToList() is much more clear. One more thing that drives me mad is that the analyzers insist on replacing ICollection customers = new HashSet(); with [] which results in completely different behavior. The use of HashSet to assign to an ICollection is a common pattern in EF and the analyzer screams at it demanding to change the behavior and introduce breaking changes

Insert/edit link
Enter the destination URL
Or link to existing content
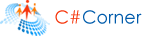
- TECHNOLOGIES
- An Interview Question

Mastering the Null-Coalescing Operator in C#

- Jitendra Mesavaniya
- Jun 30, 2024
- Other Artcile
Learn how to efficiently handle null values in C# using the null-coalescing operator. This guide covers the syntax, practical applications, and advanced techniques to enhance your programming skills.
Handling null values efficiently is a common requirement in software development. C# offers powerful tools to manage nulls, including the null-coalescing operator (??). This article explores the null-coalescing operator, its benefits, and how it can simplify and enhance your code.
Table of Contents
- Introduction to Null-Coalescing Operator
Basic Usage of Null-Coalescing Operator
Combining with null-conditional operator, chaining null-coalescing operators, the null-coalescing assignment operator, practical examples.
- Traditional Null Handling vs. Null-Coalescing Operator
Introduction
The null-coalescing operator (??) in C# allows you to provide a default value for an expression that might be null. This operator simplifies the handling of null values, making your code more readable and less error-prone.
The syntax of the null-coalescing operator is straightforward.
In this example, displayName is assigned the value "Guest" because the name is null.
The null-conditional operator (?.) can be used with the null-coalescing operator to safely navigate through potential null references.
In this example, a person.Address?.The city evaluates to null, so "Unknown" is returned.
You can chain multiple null-coalescing operators to provide multiple fallback values.
In this example, firstNonNullName is assigned the first non-null value in the chain, which is "John".
Introduced in C# 8.0, the null-coalescing assignment operator (??=) assigns a value to a variable if it is currently null.
Here, the name is assigned "Default Name" because it was initially null.
Example 1. Default Configuration.
Example 2. Safe Navigation with Fallback
Traditional Null Handling vs. Null-Coalescing Operator
Traditional approach.
Before these operators were available, handling null values required explicit null checks, which could be verbose and cumbersome.
Modern Approach
With the null-coalescing operator and null-conditional operator, the same logic becomes much simpler and more readable.
The null-coalescing operator (??) and its companion, the null-coalescing assignment operator (??=), provide powerful and concise ways to handle null values in C#. They significantly reduce the boilerplate code needed for null checks and make your code more readable and maintainable. By mastering these operators, you can write cleaner, more robust C# code that efficiently handles null values.
- .NET Programming
- C# Operators
- C# Programming
- Null-Coalescing
- Null-Coalescing Operator
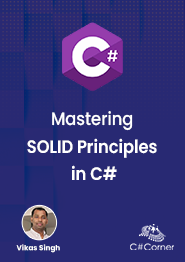
Mastering SOLID Principles in CSharp
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Is it possible to make custom operators in C#? [duplicate]
Possible Duplicate: Is it possible to create a new operator in c#?
I was wondering if it is at all possible to create custom operators such as if (a atleast 5) in C#.
The above statement would read, if a is at least 5 then do this.
I am looking for more of a keyword type operator like typeof or is .
- operator-keyword
- Why would you want to do this? I am assuming your example is not the real reason... >= 5.. – Fosco Commented May 24, 2011 at 16:12
- 1 It's more of a readability type thing, really trying to make the language my own. – Kyle Uithoven Commented May 24, 2011 at 16:14
No, C# does not allow custom operators. You can overload certain pre-existing ones, but you cannot create your own like in Haskell or F#.
- If using Visual Studio is there a way to do this with macros? or any type of environment add-ons? – Kyle Uithoven Commented May 24, 2011 at 16:12
- 1 @Kyle Uithoven: no. How would that possibly work? If it were a VS macro, it would have to put in regular C# code with function calls. You can't get around the fact that C# does not let you define custom operators (and thank $DEITY it doesn't). – siride Commented May 24, 2011 at 16:14
- @Kyle c# doesn't support macros either (by design, and for good reasons). Of course, add enough upstream custom tooling and you can probably do anything you want: I just wouldn't. – Marc Gravell Commented May 24, 2011 at 16:15
- 5 @Kyle: No, there is no way to modify C# like that. You might consider looking at extension methods, though, to allow you to type if (a.atleast(5)) – Gabe Commented May 24, 2011 at 16:16
- 1 programmers are supposed to be open minded :P come on what's the harm?! I actually totally disagree with peeps who don't want a custom operator here. If an operator being customized allows a dev team to customize their app domain's programming techniques then do it! I think as nerds we get too caught up in trying to look smart with our code (definitely guilty myself), when instead we need to look as newb as possible at least on the surface level. This allows newer people to not be intimidated and more intra-dev trust! trust = good business!! :D – Michael Socha Commented Mar 5, 2015 at 21:39
Not the answer you're looking for? Browse other questions tagged c# operators keyword operator-keyword or ask your own question .
- Featured on Meta
- We spent a sprint addressing your requests — here’s how it went
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- The [lib] tag is being burninated
- What makes a homepage useful for logged-in users
Hot Network Questions
- MOSFET Datasheet Confusion
- Equivalence of first/second choice with naive probability - I don't buy it
- Which "other travel websites" does Booking.com fetch reviews from?
- Is ElGamal homomorphic encryption using additive groups works only for Discrete Log ElGamal? What about EC ElGamal?
- Mathematical Realism and 0=1
- What Does Feynman Mean When He Says Amplitude and Probabilities?
- Why do jet aircraft need chocks when they have parking brakes?
- Finding the Zeckendorf Representation of a Positive Integer (BIO 2023 Q1)
- Fill the grid subject to product, sum and knight move constraints
- How do I know that my saddle fits me
- How to POSIX-ly ignore "warning: command substitution: ignored null byte in input"?
- Was I wrongfully denied boarding for a flight where the airliner lands to a gate that doesn't directly connect to the international part the airport?
- Why bother with planetary battlefields?
- We have differing types of infinity; do we also have differing types of randomness?
- xfrac reverses numerator and denominator in align environment with babel
- Why does King Aegon speak to his dragon in the Common Tongue (English)?
- These two Qatar flights with slightly different times and different flight number must actually be the same flight, right?
- Should I apologise to a professor after a gift authorship attempt, which they refused?
- What is the computational scaling of DFT energy vs gradient vs Hessian?
- Adjusting the indentation and the horizontal spacing in tables in latex
- Does there exist a Dehn filling of an irreducible 3-manifold with toroidal boundaries which is still irreducible?
- 为什么「五二〇运动」没有别称「五廿运动」?
- Is it possible to understand in simple terms what a Symplectic Structure is?
- How can one count how many pixels a GIF image has via command line?

IMAGES
VIDEO
COMMENTS
There is already a special instance of overloading = in place that the designers deemed ok: property setters. Let X be a property of foo. In foo.X = 3, the = symbol is replaced by the compiler by a call to foo.set_X(3). You can already define a public static T op_Assign(ref T assigned, T assignee) method. All that is left is for the compiler to ...
In this article. The assignment operator = assigns the value of its right-hand operand to a variable, a property, or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or ...
A user-defined type can overload a predefined C# operator. That is, a type can provide the custom implementation of an operation in case one or both of the operands are of that type. The Overloadable operators section shows which C# operators can be overloaded. Use the operator keyword to declare an operator. An operator declaration must ...
For more information, see the User-defined checked operators section of the Arithmetic operators article. You also use the operator keyword to overload a predefined C# operator. For more information, see Operator overloading. C# language specification. For more information, see the following sections of the C# language specification: Conversion ...
The obj1.op_Assignment(obj2) triggers the op_Assignment method, customizing the behavior of the assignment operator and copying the values from obj2 to obj1. Define a Property to Overload Assignment Operator in C#. Overloading the assignment operator using a property involves defining a property with both a getter and a setter.
Assignment operators (C# reference) The assignment operator = assigns the value of its right-hand operand to a variable, a property, or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the ...
In c#, Assignment Operators are useful to assign a new value to the operand, and these operators will work with only one operand. For example, we can declare and assign a value to the variable using the assignment operator ( =) like as shown below. int a; a = 10; If you observe the above sample, we defined a variable called " a " and ...
A user-defined type can overload a predefined C# operator. That is, a type can provide the custom implementation of an operation in case one or both of the operands are of that type. The Overloadable operators section shows which C# operators can be overloaded. Use the operator keyword to declare an operator.
Simple assignment operator, Assigns values from right side operands to left side operand. C = A + B assigns value of A + B into C. +=. Add AND assignment operator, It adds right operand to the left operand and assign the result to left operand. C += A is equivalent to C = C + A.
C# compound assignment operators. The compound assignment operators consist of two operators. They are shorthand operators. a = a + 3; a += 3; The += compound operator is one of these shorthand operators. The above two expressions are equal. Value 3 is added to the a variable. Other compound operators are:
This operator is used to assign the value on the right to the variable on the left. In addition to the basic assignment operator (=), there are compound assignment operators that combine an arithmetic operation with the assignment, simplifying and optimizing the code. Assignment operators in C# include: = (Assignment): Assigns a value to a ...
C# Assignment Operators Previous Next Assignment Operators. Assignment operators are used to assign values to variables. In the example below, we use the assignment operator (=) to assign the value 10 to a variable called x: Example int x = 10;
A. Overload the = operator so it will automatically check for a property which is a boolean and has "Specified" concatenated to the current property's name. If such a property exists, it will be assigned true when the value is assigned; if not, then assignment will operate as normal.
x %= 4; Console.WriteLine("x %= 4 results x = " + x); x is an integer on which we have applied all the available in the above C# code. The C# Assignment operators are associated with arithmetic as a prefix. The C# assignment operators are +=, -=, *=, /=, and %=.
The title is be misleading, so please read entire question :-). By "compound assignment operator" I have in mind a construct like this op=, for example +=.Pure assignment operator (=) does not belong to my question.By "why" I don't mean an opinion, but resource (book, article, etc) when some of the designers, or their coworkers, etc. express their reasoning (i.e. the source of the design choice).
public Field<T> WithValue(T value) {. return new Field<T>(this.Ordinal, this.Number, value); } And then call it like this: dateOfBirth = dateOfBirth.WithValue(DateTime.Today); Also note, I'd strongly recommend making the operator T explicit, because it discards the Ordinal and Numeric values. See this note from MSDN:
The C# assignment operator is generally suffix with arithmetic operators. The symbol of c sharp assignment operator is "=" without quotes. The assignment operator widely used with C# programming. Consider a simple example: result=num1+num2; In this example, the equal to (=) assignment operator assigns the value of num1 + num2 into result variable.
In this article. C# provides a number of operators. Many of them are supported by the built-in types and allow you to perform basic operations with values of those types. Those operators include the following groups: Arithmetic operators that perform arithmetic operations with numeric operands; Comparison operators that compare numeric operands; Boolean logical operators that perform logical ...
C# lets you overload operators to work with operands that are custom classes or structs using operators. An operator is a static method with the keyword operator preceding the operator to overload (instead of a method name), parameters representing the operands, and return types representing the result of an expression.Table 4-1 lists the available overloadable operators.
The spread element is often confused with the term "spread operator". In C#, there's no such thing as a "spread operator". The .. expression isn't an operator, it's an expression that's part of the spread element syntax. By definition, this syntax doesn't align with that of an operator, as it doesn't perform an operation on its operands.
3. No, this is not possible. The assignment operator is not overridable and for good reason. Developers are, even within the confines of C# and modern object-oriented programming languages, often "abusing" the system to make it do things it's not supposed to do.
The null-coalescing operator (??) in C# allows you to provide a default value for an expression that might be null. This operator simplifies the handling of null values, making your code more readable and less error-prone. Basic Usage of Null-Coalescing Operator. The syntax of the null-coalescing operator is straightforward.
5. No, C# does not allow custom operators. You can overload certain pre-existing ones, but you cannot create your own like in Haskell or F#. answered May 24, 2011 at 16:10. Gabe.