One line if without else in Python
by Nathan Sebhastian
Posted on Feb 22, 2023
Reading time: 4 minutes
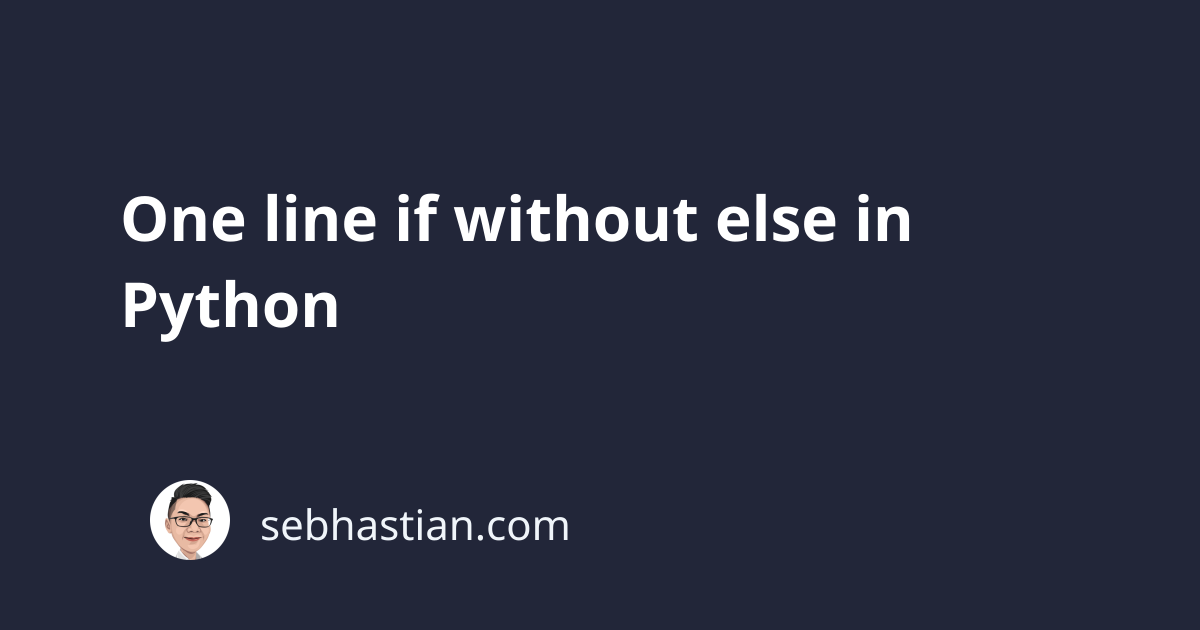
If you don’t have an else statement, then you can write a one line if statement in Python by removing the line break as follows:
There are 3 different ways you can create a one line if conditional without an else statement:
- The regular if statement in one line
- Using the ternary operator syntax
- Shorthand syntax with the and operator
This tutorial shows you examples of writing intuitive one line if statements in practice.

1. One line if statement
In the following example, the if statement prints something if x value is greater than 10 :
Now here’s how you convert the code into one line if statement:
Yup! Just remove the line break and you’re good to go.
If you have more than one line in the if body, you can use a semicolon to mark the end of the statements. See the example below:
But keep in mind that this code violates Python coding conventions by defining multiple statements in one line.
It’s also harder to read when you have complex statements inside the if body. Still, the code works well when you have simple conditionals.
2. Ternary operator syntax without else
The Python ternary operator is used to create a one line if-else statement.
It comes in handy when you need to write a short and simple if-else statement as follows:
You can read this article to learn more about how ternary operators work in Python.
You can use the ternary operator to write a one line if statement without an else as follows:
By returning None , Python will do nothing when the else statement is executed.
Although this one line if statement works, it’s not recommended when you have an assignment in your if body as follows:
Using the ternary operator, you still need to assign the original value of y in the else statement as follows:
The ternary operator doesn’t allow you to discard the else statement, so this code redundantly assigns the value of y to y in the else statement.
It’s better to use a regular if statement as follows:
Using a regular if statement has less repetition and easier to read.
3. Shorthand syntax with the and operator
The and operator can be used to shorthand an if statement because this operator executes the second operand only when the first operand returns True .
Suppose you have an if statement as follows:
You can use the and operator to rewrite the code above as follows:
The print() function above is only executed when the expression on the left side of the and operator returns True .
But this method also falls short when you need to do an assignment as it will raise an error:
If your conditional involves an assignment, then you need to use the regular if statement.
This tutorial has shown you examples of writing a one line if without else statement in Python.
In practice, writing a one line if statement is discouraged as it means you’re writing at least two statements in one line: the condition and the code to run when that condition is True .
Just because you can, doesn’t mean you should. Writing a regular if statement with line breaks and indents provides you with a clean and consistent way to define conditionals in your code.
I hope this tutorial helps. Happy coding! 👍
Take your skills to the next level ⚡️
I'm sending out an occasional email with the latest tutorials on programming, web development, and statistics. Drop your email in the box below and I'll send new stuff straight into your inbox!
Hello! This website is dedicated to help you learn tech and data science skills with its step-by-step, beginner-friendly tutorials. Learn statistics, JavaScript and other programming languages using clear examples written for people.
Learn more about this website
Connect with me on Twitter
Or LinkedIn
Type the keyword below and hit enter
Click to see all tutorials tagged with:
If-Then-Else in One Line Python
Quick answer : How to put a simple if-then-else statement in one line of Python code?
To put an if-then-else statement in one line, use Python’s ternary operator x if c else y . This returns the result of expression x if the Boolean condition c evaluates to True . Otherwise, the ternary operator returns the alternative expression y .
While you read through the article to boost your one-liner power, you can listen to my detailed video explanation:

Here’s another minimal example where you return 21 if the condition 3>2 evaluates to True , otherwise, you return 42 :
The output 42 is stored in the variable var .
Introduction and Overview
Python is so powerful, that you can even compress whole algorithms in a single line of code .
(I’m so fascinated about this that I even wrote a whole book with NoStarch on Python One-Liners:)
Check out my new Python book Python One-Liners (Amazon Link).
If you like one-liners, you’ll LOVE the book. It’ll teach you everything there is to know about a single line of Python code. But it’s also an introduction to computer science , data science, machine learning, and algorithms. The universe in a single line of Python!
The book was released in 2020 with the world-class programming book publisher NoStarch Press (San Francisco).
Publisher Link : https://nostarch.com/pythononeliners
So the natural question arises: can you write conditional if-then-else statements in a single line of code?
This article explores this mission-critical question in all detail.
Can you write the if-then-else statement in a single line of code?
Yes, you can write most if statements in a single line of Python using any of the following methods:
- Write the if statement without else branch as a Python one-liner: if 42 in range(100): print("42") .
- If you want to set a variable, use the ternary operator : x = "Alice" if "Jon" in "My name is Jonas" else "Bob" .
- If you want to conditionally execute a function, still use the ternary operator : print("42") if 42 in range(100) else print("21") .
In the previous paragraph, you’ve unwillingly learned about the ternary operator in Python.
The ternary operator is something you’ll see in most advanced code bases so make sure to understand it thoroughly by reading the following section of this article.
I drew this picture to show visually how the ternary operator works:
Let’s dive into the three different ways to write the if-then-else statement as a Python one-liner .
Related articles : Python One Line Ternary
How to Write the If-Then-Else Statement as a Python One-Liner?
Let’s have a look at all the ways how you can write the if-then-else statement in one line.
The trivial answer is to just write it in one line—but only if you don’t have an else branch:
Case 1: You Don’t Have an Else Branch
Consider the following code snippet where you check for the number 42 whether it falls in a range of numbers:
This code snippet will indeed print the output because the integer 42 falls into the range of numbers from 0 to 99.
But how can we write this if statement in a single line of code?
Just use a single line like this:
The two statements are identical so this is the way to do it—if you can write the conditional body in a single line of code.
However, if you try to become too fancy and you use a nested if body, it won’t work:
Python cannot handle this anymore: the interpreter throws an “invalid syntax” error because the statement became ambiguous.
But don’t worry, there’s a workaround: the ternary operator .

Case 2: You Have an Else Branch And You Want to Conditionally Assign a Value
In case you have an else branch and you want to conditionally assign a value to a variable, the ternary operator is your friend.
Say, you want to write the following if-then-else statement in a single line of code:
As the string "Jon" appears in the string "My name is Jonas" , the variable x will take value "Alice" .
Can we write it in a single line? Sure—by using the ternary operator .
The ternary operator is very intuitive. Just read it from left to right and you’ll understand its meaning.
We assign the value "Alice" to the variable x in case the following condition holds: "Jon" in "My name is Jonas" . Otherwise, we assign the string "Bob" to the variable x .
Ternary Operator Syntax : The three operands are written as x if c else y which reads as “return x if c else return y “. Let’s write this more intuitively as:
Case 3: What If You Don’t Want to Assign Any Value But You Have an Else Branch?
Well, there’s a quick and dirty hack: just ignore the return value of the ternary operator.
Say, we want to compress the following if-then-else statement in a single line of code:
The problem is that we don’t have a return value. So can we still use the ternary operator?
As it turns out, we can. Let’s write this if-then-else statement in a single line:
We use the ternary operator. The return value of the print() function is simply None . But we don’t really care about the return value, so we don’t store it in any variable.
We only care about executing the print function in case the if condition is met.
How to Write an If-Elif-Else Statement in a Single Line of Python?
In the previous paragraphs, you’ve learned that we can write the if-else statement in a single line of code.
But can you one-linerize an elif expression with multiple conditions?
Of course, you can!
(Heuristic: If you’re in doubt about whether you can do XYZ in a single line of Python, just assume that you can. See here .)
Say, you want to write the following if-then-else condition in a single line of code:
The elif branch wins! We print the output "yes" to the shell.
But how to do it in a single line of code? Just use the ternary operator with an elif statement won’t work (it’ll throw a syntax error).
The answer is simple: nest two ternary operators like so:
If the value x is larger than 42 , we print "no" to the shell.
Otherwise, we execute the remainder of the code (which is a ternary operator by itself). If the value x is equal to 42 , we print "yes" , otherwise "maybe" .
So by nesting multiple ternary operators, we can greatly increase our Python one-liner power!
Before you and I move on, let me present our brand-new Python book Python One-Liners .
More With Less: Buy The Python One-Liner Book
The book is released in 2020 with the world-class programming book publisher NoStarch Press (San Francisco).
Related Questions
Let’s quickly handle a bunch of related questions:
How to Write If Without Else in One Line?
We’ve already seen an example above: we simply write the if statement in one line without using the ternary operator: if 42 in range(100): print("42") .
Python is perfectly able to understand a simple if statement without an else branch in a single line of code.
How to Write Elif in One Line?
We cannot directly write the elif branch in one line of Python code. But we can nest two ternary operators instead:
Python If-Else One-Liner: What Does It Return?
The ternary operator always returns the result of the conditional evaluation. Again, the code snippet 100 if x>42 else 42 returns the integer value 42.
If you only execute functions within the ternary operator, it’ll return the None value.
Related Video Tutorial

Master the power of the single line of code—get your Python One-Liners book now! (Amazon Link)
Where to Go From Here
Knowing small Python one-liner tricks such as the ternary operator is vital for your success in the Python language. Every expert coder knows them by heart—after all, this is what makes them very productive.
If you want to learn the language Python by heart, join my free Python email course . It’s 100% based on free Python cheat sheets and Python lessons. It’s fun, easy, and you can leave anytime. Try it!
Python One-Liners Book: Master the Single Line First!
Python programmers will improve their computer science skills with these useful one-liners.
Python One-Liners will teach you how to read and write “one-liners”: concise statements of useful functionality packed into a single line of code. You’ll learn how to systematically unpack and understand any line of Python code, and write eloquent, powerfully compressed Python like an expert.
The book’s five chapters cover (1) tips and tricks, (2) regular expressions, (3) machine learning, (4) core data science topics, and (5) useful algorithms.
Detailed explanations of one-liners introduce key computer science concepts and boost your coding and analytical skills . You’ll learn about advanced Python features such as list comprehension , slicing , lambda functions , regular expressions , map and reduce functions, and slice assignments .
You’ll also learn how to:
- Leverage data structures to solve real-world problems , like using Boolean indexing to find cities with above-average pollution
- Use NumPy basics such as array , shape , axis , type , broadcasting , advanced indexing , slicing , sorting , searching , aggregating , and statistics
- Calculate basic statistics of multidimensional data arrays and the K-Means algorithms for unsupervised learning
- Create more advanced regular expressions using grouping and named groups , negative lookaheads , escaped characters , whitespaces, character sets (and negative characters sets ), and greedy/nongreedy operators
- Understand a wide range of computer science topics , including anagrams , palindromes , supersets , permutations , factorials , prime numbers , Fibonacci numbers, obfuscation , searching , and algorithmic sorting
By the end of the book, you’ll know how to write Python at its most refined , and create concise, beautiful pieces of “Python art” in merely a single line.
Get your Python One-Liners on Amazon!!
While working as a researcher in distributed systems, Dr. Christian Mayer found his love for teaching computer science students.
To help students reach higher levels of Python success, he founded the programming education website Finxter.com that has taught exponential skills to millions of coders worldwide. He’s the author of the best-selling programming books Python One-Liners (NoStarch 2020), The Art of Clean Code (NoStarch 2022), and The Book of Dash (NoStarch 2022). Chris also coauthored the Coffee Break Python series of self-published books. He’s a computer science enthusiast, freelancer , and owner of one of the top 10 largest Python blogs worldwide.
His passions are writing, reading, and coding. But his greatest passion is to serve aspiring coders through Finxter and help them to boost their skills. You can join his free email academy here.

IMAGES
VIDEO