Python Assignment Operators: Explained With Examples
Table of Contents

Assignment Operators in Python
1. basic assignment (=), 2. addition assignment (+=), 3. subtraction assignment (-=), 4. multiplication assignment (*=), 5. division assignment (/=), 6. modulus assignment (%=), 7. floor division assignment (//=), 8. exponent assignment (**=), 9. bitwise and assignment (&=), 10. bitwise or assignment (|=), 11. bitwise xor assignment (^=), 12. bitwise right shift assignment (>>=), 13. bitwise left shift assignment (<<=), simple savings calculator.
Python assignment operators play a crucial role by allocating a specific value to a variable. This operator is symbolized by the equals sign (=), marking its significance as one of the most frequently used operators in Python programming.
Python assignment operators facilitate the storage of a value in a variable by assigning the value on the right side of the operator to the variable on the left. This process is vital in programming because it enables developers to save data in variables for later use within their programs.
Examples of Python Assignment Operators
The basic assignment operator assigns the value on the right to the variable on the left. It’s a fundamental operation in Python.
Run the code using our Python Online Compiler .
This operator adds the right operand to the left operand and assigns the result back to the left operand.
Subtracts the right operand from the left and assigns the result to the left operand.
Multiplies the left operand with the right and assigns the result back to the left operand.
Divides the left operand by the right and assigns the quotient to the left operand.
0.5714285714285714
Applies modulus operation and assigns the remainder to the left operand.
Performs floor division and assigns the result to the left operand.
Raises the left operand to the power of the right operand and assigns the result back to the left.
Performs a bitwise AND operation and assigns the result to the left operand.
Applies a bitwise OR operation and assigns the result to the left operand.
Performs a bitwise XOR operation and updates the left operand with the result.
Shifts the left operand right by the number of bits specified by the right operand and assigns the result to the left.
Shifts the left operand left by the number of bits specified by the right operand and assigns the result back to the left.
Real-World Application Example of Python Assignment Operators
Imagine you want to calculate how much money you will have in your savings account after adding monthly deposits and applying annual interest. This simple calculator will:
- Start with an initial savings balance.
- Add a fixed monthly deposit amount.
- Apply annual interest at the end of the year.
Implementation:
Explanation:
- Initial and Monthly Savings: The program starts with an initial savings balance and simulates adding a fixed monthly deposit using the += operator, which adds the right operand to the left operand and assigns the result to the left operand.
- Applying Interest: At the end of each year, the program applies annual interest to the savings balance. This is done using the *= operator, which multiplies the left operand by the right operand and assigns the result to the left operand.
- Looping Through Years and Months: The program uses nested loops to iterate through each year and each month, updating the savings balance accordingly.
Related Posts:
- Python Variables: Explained with Examples
- Python Functions: Explained With Examples
- Python String format() Method: Explained With Examples
- Python Syntax: Explained with Examples
- Python Literals: Explained With Examples
- Python Regular Expression: Explained With Examples
- Python Numbers: Explained with Examples
- Python Operators: Explained With Examples
- Python Collections Module: Explained With Examples
- Python Built-in Modules: Explained With Examples
Gaurav Karwayun is the founder and executive editor of FullStackDive . He has done Computer Science Engineering and has over 12+ Years of Experience in the software industry. He has experience working in both service and product-based companies. He has vast experience in all popular programming languages, Web technologies, DevOps, etc. Follow him on LinkedIn and Twitter .
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python assignment operators.
Assignment operators are used to assign values to variables:
Related Pages

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
- Read Tutorial
- Watch Guide Video
If that is about as clear as mud don't worry we're going to walk through a number of examples. And one very nice thing about the syntax for assignment operators is that it is nearly identical to a standard type of operator. So if you memorize the list of all the python operators then you're going to be able to use each one of these assignment operators quite easily.
The very first thing I'm going to do is let's first make sure that we can print out the total. So right here we have a total and it's an integer that equals 100. Now if we wanted to add say 10 to 100 how would we go about doing that? We could reassign the value total and we could say total and then just add 10. So let's see if this works right here. I'm going to run it and you can see we have a hundred and ten. So that works.

However, whenever you find yourself performing this type of calculation what you can do is use an assignment operator. And so the syntax for that is going to get rid of everything here in the middle and say plus equals and then whatever value. In this case I want to add onto it.
So you can see we have our operator and then right afterward you have an equal sign. And this is going to do is exactly like what we had before. So if I run this again you can see total is a hundred and ten

I'm going to just so you have a reference in the show notes I'm going to say that total equals total plus 10. This is exactly the same as what we're doing right here we're simply using assignment in order to do it.
I'm going to quickly go through each one of the other elements that you can use assignment for. And if you go back and you reference the show notes or your own notes for whenever you kept track of all of the different operators you're going to notice a trend. And that is because they're all exactly the same. So here if I want to subtract 10 from the total I can simply use the subtraction operator here run it again. And now you can see we have 90. Now don't be confused because we only temporarily change the value to 1 10. So when I commented this out and I ran it from scratch it took the total and it subtracted 10 from that total and that's what got printed out.
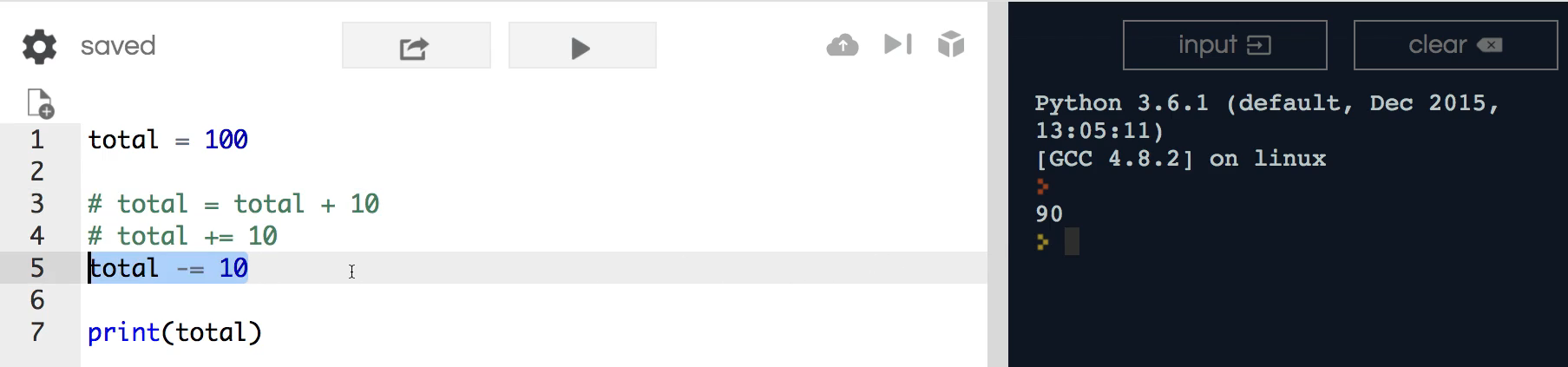
I'm going to copy this and the next one down the line is going to be multiplication. So in this case I'm going to say multiply with the asterisk the total and I'm just going to say times two just so we can see exactly what the value is going to be. And now we can see that's 200 which makes sense.
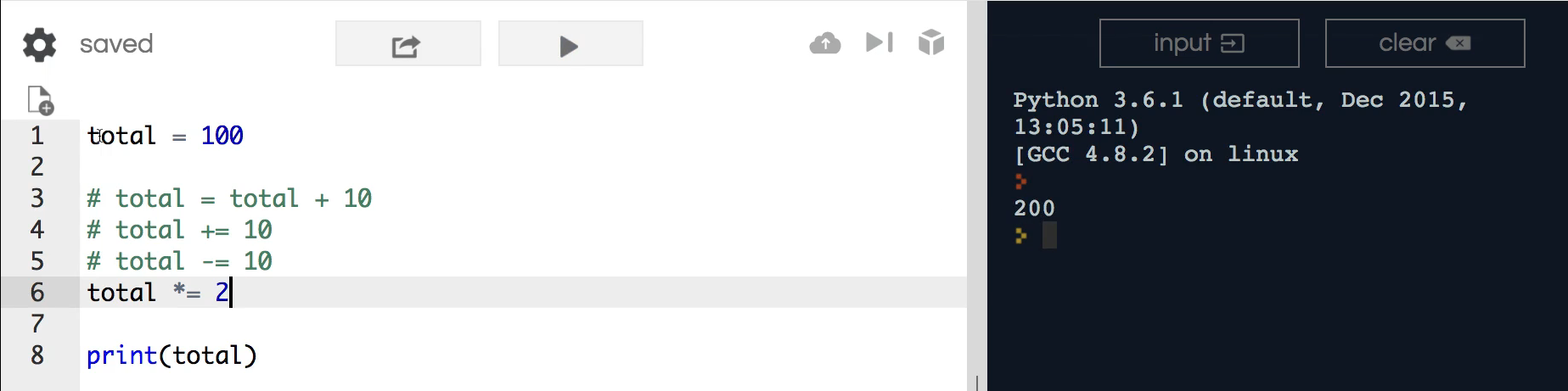
So we've taken total we have multiplied it by two and we have piped the entire thing into the total variable. So far so good. As you may have guessed next when we're going to do is division. So now I'm going to say total and then we're going to perform this division assignment and we're going to say divide this by 10 run it and you can see it gives us the value and it converts it to a float of ten point zero.
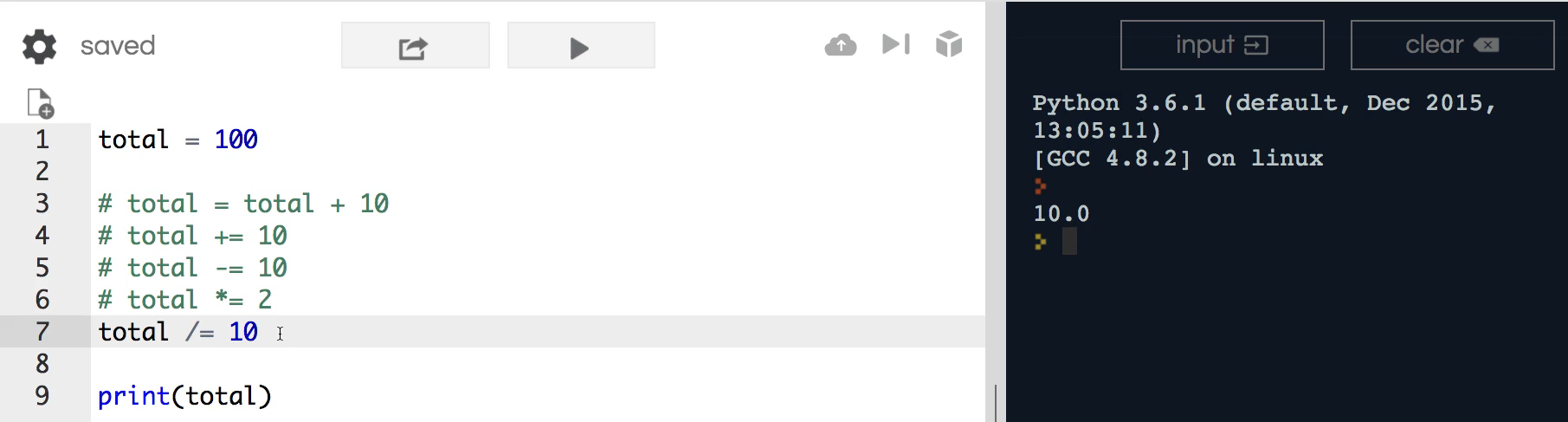
Now if this is starting to get a little bit much. Let's take a quick pause and see exactly what this is doing. Remember that all we're doing here is it's a shortcut. You could still perform it the same way we have in number 3 I could say total is equal to the total divided by 10. And if I run this you'll see we get ten point zero. And let's see what this warning is it says redefinition of total type from int to float. So we don't have to worry about this and this for if you're building Python programs you're very rarely ever going to see the syntax and it's because we have this assignment operator right here. So that is for division. And we also have the ability to use floor division as well. So if I run this you're going to see it's 10. But one thing you may notice is it's 10 it's not ten point zero. So remember that our floor division returns an integer it doesn't return a floating-point number. So if that is what you want then you can perform that task just like we did there.
Next one on the list is our exponents. I'm going to say the total and we're going to say we're going to assign that to the total squared. So going to run this and we get ten thousand. Just like you'd expect. And we have one more which is the modulus operator. So here remember it is the percent equals 2. And this is going to return zero because 100 is even if we changed 100 to be 101. This is going to return one because remember the typical purpose of the modulus operator is to let you know if you're working with an event or an odd value.
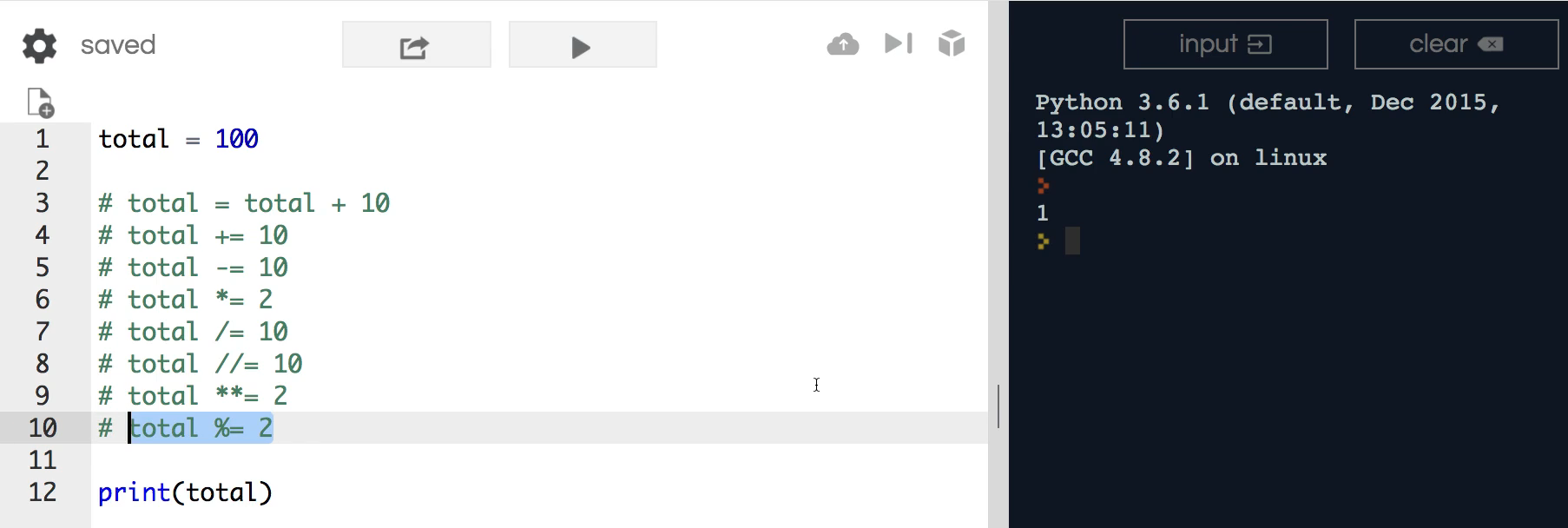
Now with all this being said, I wanted to show you every different option that you could use the assignment operator on. But I want to say that the most common way that you're going to use this or the most common one is going to be this one right here where we're adding or subtracting. So those are going to be the two most common. And what usually you're going to use that for is when you're incrementing or decrementing values so a very common way to do this would actually be like we have our total right here. So we have a total of 100 and you could imagine it being a shopping cart and it's 100 dollars and you could say product 2 and set this equal to 120. And then if I say product 3 and set this equal to 10. And so what I could do here is I could say total plus equals product to and then we could take the value and say product 3 and now if I run this you can see the value is 230.
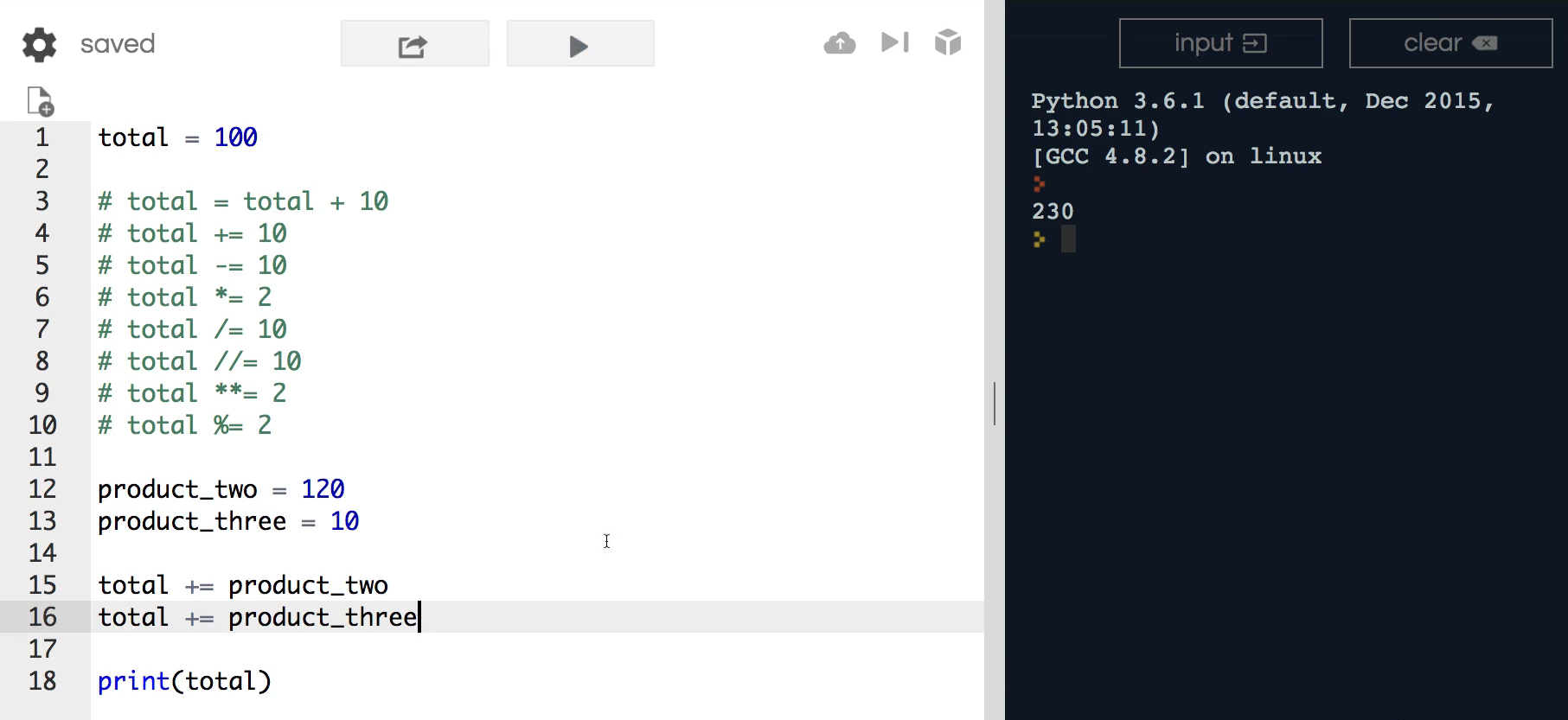
So that's a very common way whenever you want to generate a sum you can use this type of syntax which is much faster and it's also going to be a more pythonic way it's going to be the way you're going to see in standard Python programs whenever you're wanting to generate a sum and then reset and reassign the value.
So in review, that is how you can use assignment operators in Python.
devCamp does not support ancient browsers. Install a modern version for best experience.
Python Assignment Operators
Lesson Contents
Python assignment operators are one of the operator types and assign values to variables . We use arithmetic operators here in combination with a variable.
Let’s take a look at some examples.
Operator Assignment (=)
This is the most basic assignment operator and we used it before in the lessons about lists , tuples , and dictionaries . For example, we can assign a value (integer) to a variable:
Operator Addition (+=)
We can add a number to our variable like this:
Using the above operator is the same as doing this:
The += operator is shorter to write but the end result is the same.
Operator Subtraction (-=)
We can also subtract a value. For example:
Using this operator is the same as doing this:
Operator Multiplication (*=)
We can also use multiplication. We’ll multiply our variable by 4:
Which is similar to:
Operator Division (/=)
Let’s try the divide operator:
This is the same as:
Operator Modulus (%=)
We can also calculate the modulus. How about this:
This is the same as doing it like this:
Operator Exponentiation (**=)
How about exponentiation? Let’s give it a try:
Which is the same as doing it like this:
Operator Floor Division (//=)
The last one, floor division:
You have now learned how to use the Python assignment operators to assign values to variables and how you can use them with arithmetic operators . I hope you enjoyed this lesson. If you have any questions, please leave a comment.
Ask a question or start a discussion by visiting our Community Forum

Python Assignment Operators
In Python, an assignment operator is used to assign a value to a variable. The assignment operator is a single equals sign (=). Here is an example of using the assignment operator to assign a value to a variable:
In this example, the variable x is assigned the value 5.
There are also several compound assignment operators in Python, which are used to perform an operation and assign the result to a variable in a single step. These operators include:
- +=: adds the right operand to the left operand and assigns the result to the left operand
- -=: subtracts the right operand from the left operand and assigns the result to the left operand
- *=: multiplies the left operand by the right operand and assigns the result to the left operand
- /=: divides the left operand by the right operand and assigns the result to the left operand
- %=: calculates the remainder of the left operand divided by the right operand and assigns the result to the left operand
- //=: divides the left operand by the right operand and assigns the result as an integer to the left operand
- **=: raises the left operand to the power of the right operand and assigns the result to the left operand
Here are some examples of using compound assignment operators:
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Getting Started
- Keywords and Identifier
- Python Comments
- Python Variables
- Python Data Types
- Python Type Conversion
- Python I/O and Import
Python Operators
- Python Namespace
Python Flow Control
- Python if...else
- Python for Loop
- Python while Loop
- Python break and continue
- Python Pass
Python Functions
- Python Function
- Function Argument
- Python Recursion
- Anonymous Function
- Global, Local and Nonlocal
- Python Global Keyword
- Python Modules
- Python Package
Python Datatypes
- Python Numbers
- Python List
- Python Tuple
- Python String
- Python Dictionary
Python Files
- Python File Operation
- Python Directory
- Python Exception
- Exception Handling
- User-defined Exception
Python Object & Class
- Classes & Objects
- Python Inheritance
- Multiple Inheritance
- Operator Overloading
Python Advanced Topics
- Python Iterator
- Python Generator
- Python Closure
- Python Decorators
- Python Property
- Python RegEx
Python Date and time
- Python datetime Module
- Python datetime.strftime()
- Python datetime.strptime()
- Current date & time
- Get current time
- Timestamp to datetime
- Python time Module
- Python time.sleep()
Python Tutorials
Precedence and Associativity of Operators in Python
Python Operator Overloading
Python if...else Statement
Python 3 Tutorial
- Python Strings
- Python any()
Operators are special symbols that perform operations on variables and values. For example,
Here, + is an operator that adds two numbers: 5 and 6 .
- Types of Python Operators
Here's a list of different types of Python operators that we will learn in this tutorial.
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Special Operators
1. Python Arithmetic Operators
Arithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication, etc. For example,
Here, - is an arithmetic operator that subtracts two values or variables.
Example 1: Arithmetic Operators in Python
In the above example, we have used multiple arithmetic operators,
- + to add a and b
- - to subtract b from a
- * to multiply a and b
- / to divide a by b
- // to floor divide a by b
- % to get the remainder
- ** to get a to the power b
2. Python Assignment Operators
Assignment operators are used to assign values to variables. For example,
Here, = is an assignment operator that assigns 5 to x .
Here's a list of different assignment operators available in Python.
Example 2: Assignment Operators
Here, we have used the += operator to assign the sum of a and b to a .
Similarly, we can use any other assignment operators as per our needs.
3. Python Comparison Operators
Comparison operators compare two values/variables and return a boolean result: True or False . For example,
Here, the > comparison operator is used to compare whether a is greater than b or not.
Example 3: Comparison Operators
Note: Comparison operators are used in decision-making and loops . We'll discuss more of the comparison operator and decision-making in later tutorials.

4. Python Logical Operators
Logical operators are used to check whether an expression is True or False . They are used in decision-making. For example,
Here, and is the logical operator AND . Since both a > 2 and b >= 6 are True , the result is True .
Example 4: Logical Operators
Note : Here is the truth table for these logical operators.
5. Python Bitwise operators
Bitwise operators act on operands as if they were strings of binary digits. They operate bit by bit, hence the name.
For example, 2 is 10 in binary, and 7 is 111 .
In the table below: Let x = 10 ( 0000 1010 in binary) and y = 4 ( 0000 0100 in binary)
6. Python Special operators
Python language offers some special types of operators like the identity operator and the membership operator. They are described below with examples.
- Identity operators
In Python, is and is not are used to check if two values are located at the same memory location.
It's important to note that having two variables with equal values doesn't necessarily mean they are identical.
Example 4: Identity operators in Python
Here, we see that x1 and y1 are integers of the same values, so they are equal as well as identical. The same is the case with x2 and y2 (strings).
But x3 and y3 are lists. They are equal but not identical. It is because the interpreter locates them separately in memory, although they are equal.
- Membership operators
In Python, in and not in are the membership operators. They are used to test whether a value or variable is found in a sequence ( string , list , tuple , set and dictionary ).
In a dictionary, we can only test for the presence of a key, not the value.
Example 5: Membership operators in Python
Here, 'H' is in message , but 'hello' is not present in message (remember, Python is case-sensitive).
Similarly, 1 is key, and 'a' is the value in dictionary dict1 . Hence, 'a' in y returns False .
- Precedence and Associativity of operators in Python
Table of Contents
- Introduction
- Python Arithmetic Operators
- Python Assignment Operators
- Python Comparison Operators
- Python Logical Operators
- Python Bitwise operators
- Python Special operators
Video: Operators in Python
Sorry about that.
Related Tutorials
Python Tutorial
The += Operator In Python – A Complete Guide
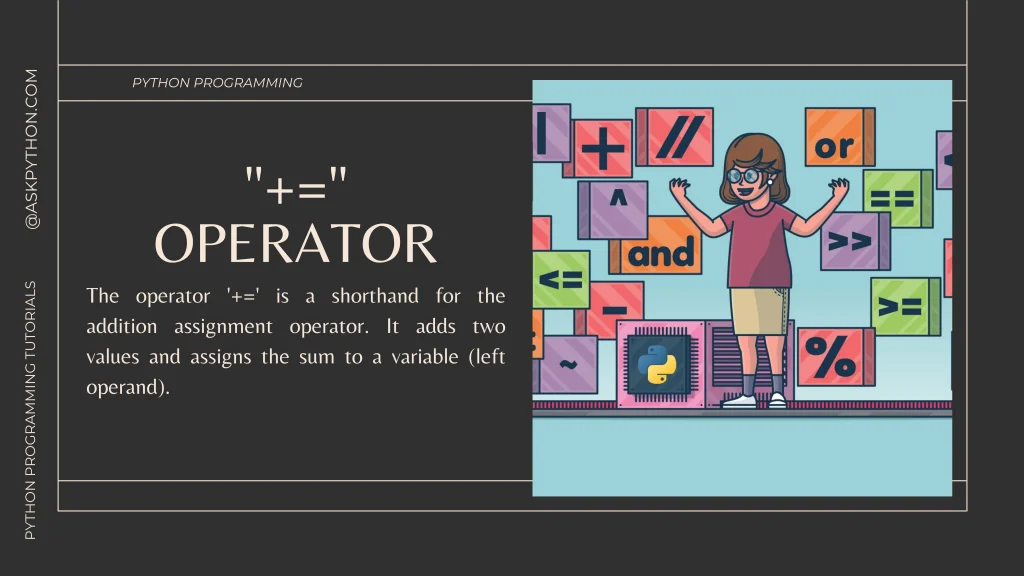
In this lesson, we will look at the += operator in Python and see how it works with several simple examples.
The operator ‘+=’ is a shorthand for the addition assignment operator . It adds two values and assigns the sum to a variable (left operand).
Let’s look at three instances to have a better idea of how this operator works.
1. Adding Two Numeric Values With += Operator
In the code mentioned below, we have initialized a variable X with an initial value of 5 and then add value 15 to it and store the resultant value in the same variable X.
The output of the Code is as follows:
2. Adding Two Strings
In the code mentioned above, we initialized two variables S1 and S2 with initial values as “Welcome to ” and ”AskPython” respectively.
We then add the two strings using the ‘+=’ operator which will concatenate the values of the string.
The output of the code is as follows:
3. Understanding Associativity of “+=” operator in Python
The associativity property of the ‘+=’ operator is from right to left. Let’s look at the example code mentioned below.
We initialized two variables X and Y with initial values as 5 and 10 respectively. In the code, we right shift the value of Y by 1 bit and then add the result to variable X and store the final result to X.
The output comes out to be X = 10 and Y = 10.
Congratulations! You just learned about the ‘+=’ operator in python and also learned about its various implementations.
Liked the tutorial? In any case, I would recommend you to have a look at the tutorials mentioned below:
- The “in” and “not in” operators in Python
- Python // operator – Floor Based Division
- Python Not Equal operator
- Operator Overloading in Python
Thank you for taking your time out! Hope you learned something new!! 😄
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- NCERT Solutions for Class 10 History Chapter 4 : The Age of Industrialisation
- Check if given path between two nodes of a graph represents a shortest paths
- 5 Tips On Learning How to Code - General Advice For Programmers
- Introduction to React-Redux
- Set value of unsigned char array in C during runtime
- How to perform a real time search and filter on a HTML table?
- CSS will-change property
- HTTP headers | Range
- Sirion Labs Interview Experience (Off-Campus FTE)
- Amazon Interview Experience for SDE-2
- Standard Chartered Interview Experience | On-Campus Internship
- BlackRock Interview Experience | On-Campus Internship 2019
- DE-Shaw Interview Experience (On-Campus)
- Amazon Interview Experience for SDE 1 (1.5 years Experienced)
- Cvent Interview Experience(Pool Campus for fulltime)
- Check if any K ranges overlap at any point
- How Software Is Made?
- IIT Madras M.S Admission Experience
- Public vs Protected in C++ with Examples
Difference between Operator Precedence and Operator Associativity
In programming, operators are used to perform various operations on data. Understanding how operators interact with each other is crucial for writing correct and efficient code. In this article, we will explore the two most important concepts which are Operator Precedence and Operator Associativity .
Operator Precedence:
Operator precedence determines which operation is performed first in an expression with more than one operator with different precedence. Operators with higher precedence are evaluated before those with lower precedence.
Example of Operator Precedence:
Let’s try to evaluate the following expression,
The expression contains two operators, + (plus) , and * (multiply). The multiplication operator * has higher precedence than + so, the first evaluation will be
After evaluating the higher precedence operator, the expression is
Now, the + operator will be evaluated.
Operator Associativity:
Operator associativity defines the direction in which operators of the same precedence are evaluated when they appear in an expression. It can be either left-to-right or right-to-left.
Example of Operator Associativity:
Let’s evaluate the following expression,
Both / (division) and % (Modulus) operators have the same precedence, so the order of evaluation will be decided by associativity.
The associativity of the multiplicative operators is from Left to Right. So,
After evaluation, the expression will be
Now, the % operator will be evaluated.
Operator Precedence and Associativity Table:
The following tables list the operator precedence from highest to lowest and the associativity for each of the operators:
Difference between Operator Precedence and Operator Associativity:
Please login to comment....
- Programming
- 10 Best Tools to Convert DOC to DOCX
- How To Summarize PDF Documents Using Google Bard for Free
- Best free Android apps for Meditation and Mindfulness
- TikTok Is Paying Creators To Up Its Search Game
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

Hiring? Flexiple helps you build your dream team of developers and designers .
How to add spaces in Python

Harsh Pandey
Last updated on 26 Mar 2024
Introduction
Adding spaces for formatting the text is easier in Python as compared to other languages as it offers various methods to achieve this task. To put spaces between words, we can use the "join" method. For adding spaces between characters, we can use a for-loop to go through the string and add spaces. To add spaces at the end and beginning of a string, we can use the "ljust" and “rjust” methods respectively.
Through this blog - “How to add spaces in Python”, let's explore these methods in detail using easy-to-follow examples!
How to add spaces in Python between words in a string
Method 1: using join() method.
The join() method joins a sequence of strings with a specified delimiter. To add spaces between words, we can split the string into words using split() method, and then rejoin them with spaces using ' '.join(words).
In the above code example, we split the sentence string into words using split() with "Is" as the delimiter. Then, we use ' '.join(words) to join the words back together with a space between them, creating the modified_sentence with spaces.
Method 2: Using ‘+’ Operator
We can also add spaces between words by using the ‘+’ operator to concatenate them with a space in between.
When using the ‘+’ operator, ensure that both operands on the left and right-hand sides are strings.
Method 3: Using f-string syntax
In Python, f-strings (formatted strings) are a convenient way to create strings with dynamic content. We can use f-strings to insert expressions, variables, or even expressions involving multiple variables directly into a string. To add spaces between words in a string using f-string, we first split the original string into words, and then we construct a new string using f-string syntax with spaces between the words.
In the above example code, we split the sentence into a list of words using the split() method, with "Is" as the delimiter. As a result, the words list now contains two elements: "PythonProgramming" and "Fun." To create the modified_sentence, we use f-string syntax within the curly braces {}. Inside these braces:
- words[0] represents the first word, which is "PythonProgramming."
- " Is " is a string containing spaces on both sides, effectively adding spaces between the words.
- words[1] represents the second word, which is "Fun."
Upon evaluation, the f-string substitutes the expressions inside the curly braces with their respective values. This yields a new string, modified_sentence, containing spaces between the words: "PythonProgramming Is Fun."
How to add spaces in Python between characters in a string
We can use the join() method to join a sequence of characters with a specified delimiter. Here, we'll use it to add spaces between each character of a string.
Method 2: Using for loop
We can achieve the same result of adding spaces between each character of a string using a for loop to iterate through each character and add spaces between them.
Note: rstrip() method is used to remove extra whitespaces at the end of the string.
Method 3: Using replace() method
Although it's not the most efficient way, we can use replace() method to add spaces between characters of a string.
Note: strip() method is used to remove extra whitespaces at both the ends of the string.
How to add spaces in Python to the end of a string
Method 1: using ljust() method.
The ljust() method is used for left-justifying a string within a specified width, padding spaces on the right if necessary.
How to add spaces in Python to the beginning of a string
Method 1: using rjust() method.
The rjust() method returns a right-justified version of the original string, padding the left side with spaces to achieve the desired width.
Using Multiplication Operator
In Python, the multiplication operator * can be used with strings to repeat the string a specified number of times, useful for adding spaces at the beginning or end of a string.
Through this blog - “How to add spaces in Python”, we have explored various methods to add spaces between words and characters, as well as padding at the beginning and end of strings. Methods like `join()`, `for` loop, and `replace()` are effective for adding spaces between characters, while `ljust()`, `rjust()`, and the multiplication operator provide options for padding. These capabilities make Python an excellent choice for text formatting and achieving visually appealing displays.
Work with top startups & companies. Get paid on time.
Try a top quality developer for 7 days. pay only if satisfied..
// Find jobs by category
You've got the vision, we help you create the best squad. Pick from our highly skilled lineup of the best independent engineers in the world.
- Ruby on Rails
- Elasticsearch
- Google Cloud
- React Native
- Contact Details
- 2093, Philadelphia Pike, DE 19703, Claymont
- [email protected]
- Explore jobs
- We are Hiring!
- Write for us
- 2093, Philadelphia Pike DE 19703, Claymont
Copyright @ 2024 Flexiple Inc

IMAGES
VIDEO
COMMENTS
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
So, Assignment Operators are used to assigning values to variables. Now Let's see each Assignment Operator one by one. 1) Assign: This operator is used to assign the value of the right side of the expression to the left side operand. Syntax: Example: Output: 2) Add and Assign: This operator is used to add the right side operand with the left ...
Each new version of Python adds new features to the language. For Python 3.8, the biggest change is the addition of assignment expressions.Specifically, the := operator gives you a new syntax for assigning variables in the middle of expressions. This operator is colloquially known as the walrus operator.. This tutorial is an in-depth introduction to the walrus operator.
Python assignment operators facilitate the storage of a value in a variable by assigning the value on the right side of the operator to the variable on the left. This process is vital in programming because it enables developers to save data in variables for later use within their programs. Operator Operation
Create your own server using Python, PHP, React.js, Node.js, Java, C#, etc. How To's. Large collection of code snippets for HTML, CSS and JavaScript. ... Python Assignment Operators. Assignment operators are used to assign values to variables: Operator Example Same As Try it = x = 5: x = 5:
Python 3.8, released in October 2019, adds assignment expressions to Python via the := syntax. The assignment expression syntax is also sometimes called "the walrus operator" because := vaguely resembles a walrus with tusks. Assignment expressions allow variable assignments to occur inside of larger expressions.
How to use the assignment operators in Python, including multiple assignment, and the short-form operators which combine an assignment and arithmetic operati...
So we don't have to worry about this and this for if you're building Python programs you're very rarely ever going to see the syntax and it's because we have this assignment operator right here. So that is for division. And we also have the ability to use floor division as well. So if I run this you're going to see it's 10.
Operator Multiplication (*=) Operator Division (/=) Operator Modulus (%=) Operator Exponentiation (**=) Operator Floor Division (//=) Conclusion. Python assignment operators are one of the operator types and assign values to variables. We use arithmetic operators here in combination with a variable. Let's take a look at some examples.
In Python, an assignment operator is used to assign a value to a variable. The assignment operator is a single equals sign (=). Here is an example of using the assignment operator to assign a value to a variable: x = 5. In this example, the variable x is assigned the value 5. There are also several compound assignment operators in Python, which ...
Since Python 3.8, code can use the so-called "walrus" operator (:=), documented in PEP 572, for assignment expressions. This seems like a really substantial new feature, since it allows this form of assignment within comprehensions and lambdas. What exactly are the syntax, semantics, and grammar specifications of assignment expressions?
🎓Welcome back to Digital Academy, the Complete Python Development Tutorial for Beginners, which will help you Learn Python from A to Z!🖥️ Types of Operator...
Here's a list of different assignment operators available in Python. Operator Name Example = Assignment Operator: a = 7 += Addition Assignment: a += 1 # a = a + 1-= Subtraction Assignment: ... Similarly, we can use any other assignment operators as per our needs. 3. Python Comparison Operators.
The Simple assignment operator in Python is denoted by = and is used to assign values from the right side of the operator to the value on the left side. Input: a = b + c. Add and equal operator. This operator adds the value on the right side to the value on the left side and stores the result in the operand on the left side. Input: a = 5. a += 10.
Python Programming: Assignment Operators in PythonTopics discussed:1. Introduction to Assignment Operators.2. Assignment Operators in Python.Python Programmi...
It basically combines the functioning of the arithmetic or bitwise operator with the assignment operator. So assume if we need to add 7 to a variable "a" and assign the result back to "a", then instead of writing normally as "a = a + 7", we can use the augmented assignment operator and write the expression as "a += 7". Here ...
In this lesson, we will look at the += operator in Python and see how it works with several simple examples. The operator '+=' is a shorthand for the addition assignment operator. It adds two values and assigns the sum to a variable (left operand). Let's look at three instances to have a better idea of how this operator works.
This behavior shouldn't be a problem unless you try to use the is for comparing equality of two objects. So always use the == operator to check if any two Python objects have the same value. 4. Tuple Assignment and Mutable Objects . If you're familiar with built-in data structures in Python, you know that tuples are immutable.
32. Python does not have a "comma operator" as in C. Instead, the comma indicates that a tuple should be constructed. The right-hand side of. a, b = a + b, a. is a tuple with th two items a + b and a. On the left-hand side of an assignment, the comma indicates that sequence unpacking should be performed according to the rules you quoted: a will ...
In programming, operators are used to perform various operations on data. Understanding how operators interact with each other is crucial for writing correct and efficient code. In this article, we will explore the two most important concepts which are Operator Precedence and Operator Associativity. Operator Precedence:
Then, we use ' '.join(words) to join the words back together with a space between them, creating the modified_sentence with spaces. Method 2: Using '+' Operator. We can also add spaces between words by using the '+' operator to concatenate them with a space in between.
Python for Everybody assignment 11.1 The actual goal is to read a file, look for integers using the re.findall() looking for a regular expression of [0-9]+, then converting the extracted strings to integers and finally summing up the integers. Python 3.12 is returning. traceback: 'hand' not defined.