

Python Conditional Assignment
When you want to assign a value to a variable based on some condition, like if the condition is true then assign a value to the variable, else assign some other value to the variable, then you can use the conditional assignment operator.
In this tutorial, we will look at different ways to assign values to a variable based on some condition.
1. Using Ternary Operator
The ternary operator is very special operator in Python, it is used to assign a value to a variable based on some condition.
It goes like this:
Here, the value of variable will be value_if_true if the condition is true, else it will be value_if_false .
Let's see a code snippet to understand it better.
You can see we have conditionally assigned a value to variable c based on the condition a > b .
2. Using if-else statement
if-else statements are the core part of any programming language, they are used to execute a block of code based on some condition.
Using an if-else statement, we can assign a value to a variable based on the condition we provide.
Here is an example of replacing the above code snippet with the if-else statement.
3. Using Logical Short Circuit Evaluation
Logical short circuit evaluation is another way using which you can assign a value to a variable conditionally.
The format of logical short circuit evaluation is:
It looks similar to ternary operator, but it is not. Here the condition and value_if_true performs logical AND operation, if both are true then the value of variable will be value_if_true , or else it will be value_if_false .
Let's see an example:
But if we make condition True but value_if_true False (or 0 or None), then the value of variable will be value_if_false .
So, you can see that the value of c is 20 even though the condition a < b is True .
So, you should be careful while using logical short circuit evaluation.
While working with lists , we often need to check if a list is empty or not, and if it is empty then we need to assign some default value to it.
Let's see how we can do it using conditional assignment.
Here, we have assigned a default value to my_list if it is empty.
Assign a value to a variable conditionally based on the presence of an element in a list.
Now you know 3 different ways to assign a value to a variable conditionally. Any of these methods can be used to assign a value when there is a condition.
The cleanest and fastest way to conditional value assignment is the ternary operator .
if-else statement is recommended to use when you have to execute a block of code based on some condition.
Happy coding! 😊
- Module 2: The Essentials of Python »
- Conditional Statements
- View page source
Conditional Statements
There are reading-comprehension exercises included throughout the text. These are meant to help you put your reading to practice. Solutions for the exercises are included at the bottom of this page.
In this section, we will be introduced to the if , else , and elif statements. These allow you to specify that blocks of code are to be executed only if specified conditions are found to be true, or perhaps alternative code if the condition is found to be false. For example, the following code will square x if it is a negative number, and will cube x if it is a positive number:
Please refer to the “Basic Python Object Types” subsection to recall the basics of the “boolean” type, which represents True and False values. We will extend that discussion by introducing comparison operations and membership-checking, and then expanding on the utility of the built-in bool type.
Comparison Operations
Comparison statements will evaluate explicitly to either of the boolean-objects: True or False . There are eight comparison operations in Python:
The first six of these operators are familiar from mathematics:
Note that = and == have very different meanings. The former is the assignment operator, and the latter is the equality operator:
Python allows you to chain comparison operators to create “compound” comparisons:
Whereas == checks to see if two objects have the same value, the is operator checks to see if two objects are actually the same object. For example, creating two lists with the same contents produces two distinct lists, that have the same “value”:
Thus the is operator is most commonly used to check if a variable references the None object, or either of the boolean objects:
Use is not to check if two objects are distinct:
bool and Truth Values of Non-Boolean Objects
Recall that the two boolean objects True and False formally belong to the int type in addition to bool , and are associated with the values 1 and 0 , respectively:
Likewise Python ascribes boolean values to non-boolean objects. For example,the number 0 is associated with False and non-zero numbers are associated with True . The boolean values of built-in objects can be evaluated with the built-in Python command bool :
and non-zero Python integers are associated with True :
The following built-in Python objects evaluate to False via bool :
Zero of any numeric type: 0 , 0.0 , 0j
Any empty sequence, such as an empty string or list: '' , tuple() , [] , numpy.array([])
Empty dictionaries and sets
Thus non-zero numbers and non-empty sequences/collections evaluate to True via bool .
The bool function allows you to evaluate the boolean values ascribed to various non-boolean objects. For instance, bool([]) returns False wherease bool([1, 2]) returns True .
if , else , and elif
We now introduce the simple, but powerful if , else , and elif conditional statements. This will allow us to create simple branches in our code. For instance, suppose you are writing code for a video game, and you want to update a character’s status based on her/his number of health-points (an integer). The following code is representative of this:
Each if , elif , and else statement must end in a colon character, and the body of each of these statements is delimited by whitespace .
The following pseudo-code demonstrates the general template for conditional statements:
In practice this can look like:
In its simplest form, a conditional statement requires only an if clause. else and elif clauses can only follow an if clause.
Similarly, conditional statements can have an if and an else without an elif :
Conditional statements can also have an if and an elif without an else :
Note that only one code block within a single if-elif-else statement can be executed: either the “if-block” is executed, or an “elif-block” is executed, or the “else-block” is executed. Consecutive if-statements, however, are completely independent of one another, and thus their code blocks can be executed in sequence, if their respective conditional statements resolve to True .
Reading Comprehension: Conditional statements
Assume my_list is a list. Given the following code:
What will happen if my_list is [] ? Will IndexError be raised? What will first_item be?
Assume variable my_file is a string storing a filename, where a period denotes the end of the filename and the beginning of the file-type. Write code that extracts only the filename.
my_file will have at most one period in it. Accommodate cases where my_file does not include a file-type.
"code.py" \(\rightarrow\) "code"
"doc2.pdf" \(\rightarrow\) "doc2"
"hello_world" \(\rightarrow\) "hello_world"
Inline if-else statements
Python supports a syntax for writing a restricted version of if-else statements in a single line. The following code:
can be written in a single line as:
This is suggestive of the general underlying syntax for inline if-else statements:
The inline if-else statement :
The expression A if <condition> else B returns A if bool(<condition>) evaluates to True , otherwise this expression will return B .
This syntax is highly restricted compared to the full “if-elif-else” expressions - no “elif” statement is permitted by this inline syntax, nor are multi-line code blocks within the if/else clauses.
Inline if-else statements can be used anywhere, not just on the right side of an assignment statement, and can be quite convenient:
We will see this syntax shine when we learn about comprehension statements. That being said, this syntax should be used judiciously. For example, inline if-else statements ought not be used in arithmetic expressions, for therein lies madness:
Short-Circuiting Logical Expressions
Armed with our newfound understanding of conditional statements, we briefly return to our discussion of Python’s logic expressions to discuss “short-circuiting”. In Python, a logical expression is evaluated from left to right and will return its boolean value as soon as it is unambiguously determined, leaving any remaining portions of the expression unevaluated . That is, the expression may be short-circuited .
For example, consider the fact that an and operation will only return True if both of its arguments evaluate to True . Thus the expression False and <anything> is guaranteed to return False ; furthermore, when executed, this expression will return False without having evaluated bool(<anything>) .
To demonstrate this behavior, consider the following example:
According to our discussion, the pattern False and short-circuits this expression without it ever evaluating bool(1/0) . Reversing the ordering of the arguments makes this clear.
In practice, short-circuiting can be leveraged in order to condense one’s code. Suppose a section of our code is processing a variable x , which may be either a number or a string . Suppose further that we want to process x in a special way if it is an all-uppercased string. The code
is problematic because isupper can only be called once we are sure that x is a string; this code will raise an error if x is a number. We could instead write
but the more elegant and concise way of handling the nestled checking is to leverage our ability to short-circuit logic expressions.
See, that if x is not a string, that isinstance(x, str) will return False ; thus isinstance(x, str) and x.isupper() will short-circuit and return False without ever evaluating bool(x.isupper()) . This is the preferable way to handle this sort of checking. This code is more concise and readable than the equivalent nested if-statements.
Reading Comprehension: short-circuited expressions
Consider the preceding example of short-circuiting, where we want to catch the case where x is an uppercased string. What is the “bug” in the following code? Why does this fail to utilize short-circuiting correctly?
Links to Official Documentation
Truth testing
Boolean operations
Comparisons
‘if’ statements
Reading Comprehension Exercise Solutions:
Conditional statements
If my_list is [] , then bool(my_list) will return False , and the code block will be skipped. Thus first_item will be None .
First, check to see if . is even contained in my_file . If it is, find its index-position, and slice the string up to that index. Otherwise, my_file is already the file name.
Short-circuited expressions
fails to account for the fact that expressions are always evaluated from left to right. That is, bool(x.isupper()) will always be evaluated first in this instance and will raise an error if x is not a string. Thus the following isinstance(x, str) statement is useless.
Conditional Assignment Operator in Python
- Python How-To's
- Conditional Assignment Operator in …
Meaning of ||= Operator in Ruby
Implement ruby’s ||= conditional assignment operator in python using the try...except statement, implement ruby’s ||= conditional assignment operator in python using local and global variables.
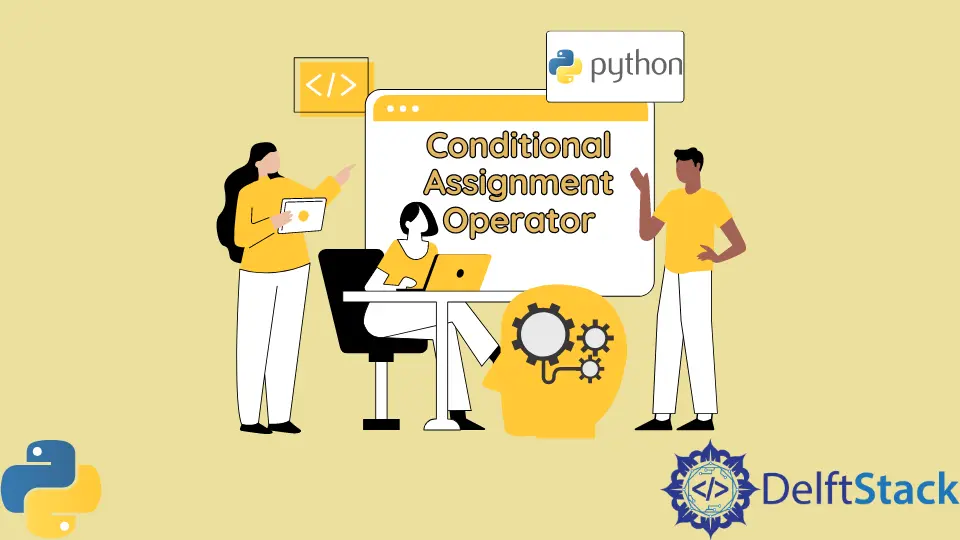
There isn’t any exact equivalent of Ruby’s ||= operator in Python. However, we can use the try...except method and concepts of local and global variables to emulate Ruby’s conditional assignment operator ||= in Python.
The basic meaning of this operator is to assign the value of the variable y to variable x if variable x is undefined or is falsy value, otherwise no assignment operation is performed.
But this operator is much more complex and confusing than other simpler conditional operators like += , -= because whenever any variable is encountered as undefined, the console throws out NameError .
a+=b evaluates to a=a+b .
a||=b looks as a=a||b but actually behaves as a||a=b .
We use try...except to catch and handle errors. Whenever the try except block runs, at first, the code lying within the try block executes. If the block of code within the try block successfully executes, then the except block is ignored; otherwise, the except block code will be executed, and the error is handled. Ruby’s ||= operator can roughly be translated in Python’s try-catch method as :
Here, if the variable x is defined, the try block will execute smoothly with no NameError exception. Hence, no assignment operation is performed. If x is not defined, the try block will generate NameError , then the except block gets executed, and variable x is assigned to 10 .
The scope of local variables is confined within a specific code scope, whereas global variables have their scope defined in the entire code space.
All the local variables in a particular scope are available as keys of the locals dictionary in that particular scope. All the global variables are stored as keys of the globals dictionary. We can access those variables whenever necessary using the locals and the globals dictionary.
We can check if a variable exists in any of the dictionaries and set its value only if it does not exist to translate Ruby’s ||= conditional assignment operator in Python.
Here, if the variable x is present in either global or local scope, we don’t perform any assignment operation; otherwise, we assign the value of x to 10 . It is similar to x||=10 in Ruby.
Related Article - Python Operator
- Python Bitwise NOT
- How to Unpack Operator ** in Python
- How to Overload Operator in Python
- Python Annotation ->
- The Walrus Operator := in Python
Conditional expression (ternary operator) in Python
Python has a conditional expression (sometimes called a "ternary operator"). You can write operations like if statements in one line with conditional expressions.
- 6. Expressions - Conditional expressions — Python 3.11.3 documentation
Basics of the conditional expression (ternary operator)
If ... elif ... else ... by conditional expressions, list comprehensions and conditional expressions, lambda expressions and conditional expressions.
See the following article for if statements in Python.
- Python if statements (if, elif, else)
In Python, the conditional expression is written as follows.
The condition is evaluated first. If condition is True , X is evaluated and its value is returned, and if condition is False , Y is evaluated and its value is returned.
If you want to switch the value based on a condition, simply use the desired values in the conditional expression.
If you want to switch between operations based on a condition, simply describe each corresponding expression in the conditional expression.
An expression that does not return a value (i.e., an expression that returns None ) is also acceptable in a conditional expression. Depending on the condition, either expression will be evaluated and executed.
The above example is equivalent to the following code written with an if statement.
You can also combine multiple conditions using logical operators such as and or or .
- Boolean operators in Python (and, or, not)
By combining conditional expressions, you can write an operation like if ... elif ... else ... in one line.
However, it is difficult to understand, so it may be better not to use it often.
The following two interpretations are possible, but the expression is processed as the first one.
In the sample code below, which includes three expressions, the first expression is interpreted like the second, rather than the third:
By using conditional expressions in list comprehensions, you can apply operations to the elements of the list based on the condition.
See the following article for details on list comprehensions.
- List comprehensions in Python
Conditional expressions are also useful when you want to apply an operation similar to an if statement within lambda expressions.
In the example above, the lambda expression is assigned to a variable for convenience, but this is not recommended by PEP8.
Refer to the following article for more details on lambda expressions.
- Lambda expressions in Python
Related Categories
Related articles.
- Shallow and deep copy in Python: copy(), deepcopy()
- Composite two images according to a mask image with Python, Pillow
- OpenCV, NumPy: Rotate and flip image
- pandas: Check if DataFrame/Series is empty
- Check pandas version: pd.show_versions
- Python if statement (if, elif, else)
- pandas: Find the quantile with quantile()
- Handle date and time with the datetime module in Python
- Get image size (width, height) with Python, OpenCV, Pillow (PIL)
- Convert between Unix time (Epoch time) and datetime in Python
- Convert BGR and RGB with Python, OpenCV (cvtColor)
- Matrix operations with NumPy in Python
- pandas: Replace values in DataFrame and Series with replace()
- Uppercase and lowercase strings in Python (conversion and checking)
- Calculate mean, median, mode, variance, standard deviation in Python
A Comprehensive Guide to Using Conditionals in Python with Real-World Examples
Conditionals are a fundamental concept in programming that allow code to execute differently based on certain conditions. In Python, conditionals take the form of if , elif , and else statements. Mastering conditionals is key to writing dynamic, flexible programs that can handle different scenarios and make decisions.
This comprehensive guide will provide a deep dive into using conditionals in Python for real-world applications. We will cover the following topics:
Table of Contents
Basic syntax and structure of conditionals, comparison operators, logic operators, if statements, if-else statements, if-elif-else statements, nested conditionals, ternary operator, common errors and mistakes, user input validation, handling different user types, recommendation systems, data analysis and visualization, game design and gameplay logic.
The basic syntax for an if statement in Python is:
The condition can be any expression that evaluates to True or False. The code block indented under the if statement runs only when the condition is True.
Some key points:
- The condition follows the if keyword and ends with a colon (:)
- The code block after the condition is indented (usually 4 spaces)
- if , elif , and else are lowercase
- Code blocks end when the indentation returns to the left margin
Let’s look at a simple example:
Here we check if the value of age is greater than or equal to 18. If so, we print a message saying the person can vote. The print statement is indented under the if to indicate it runs conditionally.
Comparison operators allow us to compare two values and evaluate to True or False. They are essential for writing conditional expressions.
Here are some examples of using comparison operators in conditional statements:
We can also chain multiple comparisons using logic operators like and and or .
Logic operators allow us to combine multiple conditional expressions and evaluate the overall logic.
The two main logic operators are:
- and - Both conditions must be True for overall expression to be True
- or - Either one condition must be True for overall expression to be True
Both age >= 18 and citizen must be True for the print statement to execute.
Other logical operators include:
- not - Negates or flips the Boolean value
- in - Checks if a value is present in a sequence
- not in - Checks if a value is not present
Logic operators allow us to handle complex conditional logic in a concise way.
The if statement is used when we want to execute code only when some condition is fulfilled. For example:
Here we only want to print “Great job!” when the score is 80 or higher. The if statement allows us to specify this condition.
Some things to note about if statements:
- They execute the code block only when condition evaluates to True
- The condition can use any comparison or logical operators
- We can use complex logic by chaining multiple conditions with and , or , not
- The code block must be indented under the if statement
Let’s look at some more examples:
The if statement allows us to execute code conditioned on any criteria we specify in the conditional expression.
The if-else statement extends the simple if by allowing us to specify code that executes when the condition evaluates to False.
The syntax is:
Let’s look at an example:
Here if age is less than 18, we print a different message using the else block.
Key points on if-else :
- The else can only be used after an if statement
- The else block runs when the if condition is False
- We can chain multiple elif blocks for more conditions (see next section)
- Only one code block will execute - either if or else
More examples:
The if-else statement allows us to conditionally run different code blocks based on the evaluation of the condition expression.
The elif statement is used to chain multiple conditional checks. Using elif we can have multiple conditions evaluated in order.
This allows us to check many conditions and selectively run code for each case. For example:
Here we check the score against multiple grade thresholds. First if to check for A, then elif to check for B, etc. The final else acts as a default case if none match.
Some key points on if-elif-else :
- Only one block will execute
- Each condition is checked in order
- elif lets us chain multiple conditions
- The else block is optional
The elif conditionals allow us to concisely handle multiple scenarios without writing nested if statements.
Nested conditionals refer to if statements within if statements. We can nest conditionals indefinitely to handle complex logic.
For example:
The outer if checks age, and the inner if-else selectively prints messages for students vs non-students.
Nested conditionals are useful when:
- We want to check secondary conditions after initial condition passes
- Breaking down complex conditional logic into simple steps
- Handling specific cases before handling general cases
However, deeply nested conditionals can make code hard to read. In those cases, functions may be better for readability.
The ternary operator provides a compact syntax for basic conditional logic:
This condenses a basic if-else check into one line.
Some points on ternary operator usage:
- Best for simple one line conditionals
- Hard to read for complex logic
- Can be nested but not recommended
- Has form value_if_true if condition else value_if_false
The ternary operator is ideal for quick conditional assignments or returning values conditionally from functions.
Some common errors when using conditionals include:
- Forgetting colons : after conditionals
- Indentation errors with code blocks
- Using assignment = instead of comparisons ==
- Misspellings in conditionals like adn , ro , etc.
- Missing parentheses around conditions
- Checking equality on two different types
These often cause syntax errors or unexpected logic errors. Always double check the condition expressions and indentations when debugging conditional issues.
Proper code commenting and leaving notes during coding can help identify issues with complex conditional statements. Start small and test conditionals thoroughly when chaining many elif clauses.
Real-World Examples and Exercises
Next we’ll explore some real-world examples to illustrate how conditionals are used in Python programming for tasks like user input validation, handling different user types, recommendation engines, data analysis, game design, and more.
Validating user input is crucial for many programs. For example:
We first check if the input is a digit, then convert to an integer. Next we check if age meets the 18+ requirement for access. The else handles any non-digit input.
Here are some other user input validation examples:
Careful input validation prevents bugs and errors down the line.
We can use conditionals to handle different features or pricing for various user types:
Different access levels can also be handled:
Conditionals allow flexible user handling in large applications.
Many recommendation systems use conditional logic to provide personalized suggestions based on certain factors. For example:
Products can be intelligently recommended using if-elif conditional chains.
When analyzing and visualizing data in Python, we can use conditionals to handle missing data or special cases:
Conditionals help account for incomplete data and customize data visualization.
Games make heavy use of conditionals to implement gameplay mechanics, physics, ballistics, animations, etc.
This implements a basic combat loop with damage dealt conditionally based on hit chance rolls. The end condition checks remaining health to determine winner.
Many other gameplay elements can be implemented using conditionals - physics, animations, resource management, abilities, etc.
Conditionals allow us to execute code selectively based on Boolean logic and are a core programming concept in any language. Python provides an intuitive syntax using if , else , elif for implementing conditional code execution.
In this guide, we covered the basics of conditionals in Python including operators, complex conditional chains, nesting conditionals, ternary expressions, and common errors. We examined real-world examples of using conditional logic for input validation, handling user types, recommendation systems, data analysis, and game mechanics.
Conditionals enable you to write dynamic, flexible programs that can make intelligent decisions and handle varying scenarios. Mastering their usage takes practice, but being comfortable with conditional logic will enable you to take on more advanced programming tasks.
- python
- control-flow
Python One Line Conditional Assignment
Problem : How to perform one-line if conditional assignments in Python?
Example : Say, you start with the following code.
You want to set the value of x to 42 if boo is True , and do nothing otherwise.
Let’s dive into the different ways to accomplish this in Python. We start with an overview:
Exercise : Run the code. Are all outputs the same?
Next, you’ll dive into each of those methods and boost your one-liner superpower !
Method 1: Ternary Operator
The most basic ternary operator x if c else y returns expression x if the Boolean expression c evaluates to True . Otherwise, if the expression c evaluates to False , the ternary operator returns the alternative expression y .
Let’s go back to our example problem! You want to set the value of x to 42 if boo is True , and do nothing otherwise. Here’s how to do this in a single line:
While using the ternary operator works, you may wonder whether it’s possible to avoid the ...else x part for clarity of the code? In the next method, you’ll learn how!
If you need to improve your understanding of the ternary operator, watch the following video:

You can also read the related article:
- Python One Line Ternary
Method 2: Single-Line If Statement
Like in the previous method, you want to set the value of x to 42 if boo is True , and do nothing otherwise. But you don’t want to have a redundant else branch. How to do this in Python?
The solution to skip the else part of the ternary operator is surprisingly simple— use a standard if statement without else branch and write it into a single line of code :
To learn more about what you can pack into a single line, watch my tutorial video “If-Then-Else in One Line Python” :

Method 3: Ternary Tuple Syntax Hack
A shorthand form of the ternary operator is the following tuple syntax .
Syntax : You can use the tuple syntax (x, y)[c] consisting of a tuple (x, y) and a condition c enclosed in a square bracket. Here’s a more intuitive way to represent this tuple syntax.
In fact, the order of the <OnFalse> and <OnTrue> operands is just flipped when compared to the basic ternary operator. First, you have the branch that’s returned if the condition does NOT hold. Second, you run the branch that’s returned if the condition holds.
Clever! The condition boo holds so the return value passed into the x variable is the <OnTrue> branch 42 .
Don’t worry if this confuses you—you’re not alone. You can clarify the tuple syntax once and for all by studying my detailed blog article.
Related Article : Python Ternary — Tuple Syntax Hack
Python One-Liners Book: Master the Single Line First!
Python programmers will improve their computer science skills with these useful one-liners.
Python One-Liners will teach you how to read and write “one-liners”: concise statements of useful functionality packed into a single line of code. You’ll learn how to systematically unpack and understand any line of Python code, and write eloquent, powerfully compressed Python like an expert.
The book’s five chapters cover (1) tips and tricks, (2) regular expressions, (3) machine learning, (4) core data science topics, and (5) useful algorithms.
Detailed explanations of one-liners introduce key computer science concepts and boost your coding and analytical skills . You’ll learn about advanced Python features such as list comprehension , slicing , lambda functions , regular expressions , map and reduce functions, and slice assignments .
You’ll also learn how to:
- Leverage data structures to solve real-world problems , like using Boolean indexing to find cities with above-average pollution
- Use NumPy basics such as array , shape , axis , type , broadcasting , advanced indexing , slicing , sorting , searching , aggregating , and statistics
- Calculate basic statistics of multidimensional data arrays and the K-Means algorithms for unsupervised learning
- Create more advanced regular expressions using grouping and named groups , negative lookaheads , escaped characters , whitespaces, character sets (and negative characters sets ), and greedy/nongreedy operators
- Understand a wide range of computer science topics , including anagrams , palindromes , supersets , permutations , factorials , prime numbers , Fibonacci numbers, obfuscation , searching , and algorithmic sorting
By the end of the book, you’ll know how to write Python at its most refined , and create concise, beautiful pieces of “Python art” in merely a single line.
Get your Python One-Liners on Amazon!!
While working as a researcher in distributed systems, Dr. Christian Mayer found his love for teaching computer science students.
To help students reach higher levels of Python success, he founded the programming education website Finxter.com that has taught exponential skills to millions of coders worldwide. He’s the author of the best-selling programming books Python One-Liners (NoStarch 2020), The Art of Clean Code (NoStarch 2022), and The Book of Dash (NoStarch 2022). Chris also coauthored the Coffee Break Python series of self-published books. He’s a computer science enthusiast, freelancer , and owner of one of the top 10 largest Python blogs worldwide.
His passions are writing, reading, and coding. But his greatest passion is to serve aspiring coders through Finxter and help them to boost their skills. You can join his free email academy here.
Python Enhancement Proposals
- Python »
- PEP Index »
PEP 572 – Assignment Expressions
The importance of real code, exceptional cases, scope of the target, relative precedence of :=, change to evaluation order, differences between assignment expressions and assignment statements, specification changes during implementation, _pydecimal.py, datetime.py, sysconfig.py, simplifying list comprehensions, capturing condition values, changing the scope rules for comprehensions, alternative spellings, special-casing conditional statements, special-casing comprehensions, lowering operator precedence, allowing commas to the right, always requiring parentheses, why not just turn existing assignment into an expression, with assignment expressions, why bother with assignment statements, why not use a sublocal scope and prevent namespace pollution, style guide recommendations, acknowledgements, a numeric example, appendix b: rough code translations for comprehensions, appendix c: no changes to scope semantics.
This is a proposal for creating a way to assign to variables within an expression using the notation NAME := expr .
As part of this change, there is also an update to dictionary comprehension evaluation order to ensure key expressions are executed before value expressions (allowing the key to be bound to a name and then re-used as part of calculating the corresponding value).
During discussion of this PEP, the operator became informally known as “the walrus operator”. The construct’s formal name is “Assignment Expressions” (as per the PEP title), but they may also be referred to as “Named Expressions” (e.g. the CPython reference implementation uses that name internally).
Naming the result of an expression is an important part of programming, allowing a descriptive name to be used in place of a longer expression, and permitting reuse. Currently, this feature is available only in statement form, making it unavailable in list comprehensions and other expression contexts.
Additionally, naming sub-parts of a large expression can assist an interactive debugger, providing useful display hooks and partial results. Without a way to capture sub-expressions inline, this would require refactoring of the original code; with assignment expressions, this merely requires the insertion of a few name := markers. Removing the need to refactor reduces the likelihood that the code be inadvertently changed as part of debugging (a common cause of Heisenbugs), and is easier to dictate to another programmer.
During the development of this PEP many people (supporters and critics both) have had a tendency to focus on toy examples on the one hand, and on overly complex examples on the other.
The danger of toy examples is twofold: they are often too abstract to make anyone go “ooh, that’s compelling”, and they are easily refuted with “I would never write it that way anyway”.
The danger of overly complex examples is that they provide a convenient strawman for critics of the proposal to shoot down (“that’s obfuscated”).
Yet there is some use for both extremely simple and extremely complex examples: they are helpful to clarify the intended semantics. Therefore, there will be some of each below.
However, in order to be compelling , examples should be rooted in real code, i.e. code that was written without any thought of this PEP, as part of a useful application, however large or small. Tim Peters has been extremely helpful by going over his own personal code repository and picking examples of code he had written that (in his view) would have been clearer if rewritten with (sparing) use of assignment expressions. His conclusion: the current proposal would have allowed a modest but clear improvement in quite a few bits of code.
Another use of real code is to observe indirectly how much value programmers place on compactness. Guido van Rossum searched through a Dropbox code base and discovered some evidence that programmers value writing fewer lines over shorter lines.
Case in point: Guido found several examples where a programmer repeated a subexpression, slowing down the program, in order to save one line of code, e.g. instead of writing:
they would write:
Another example illustrates that programmers sometimes do more work to save an extra level of indentation:
This code tries to match pattern2 even if pattern1 has a match (in which case the match on pattern2 is never used). The more efficient rewrite would have been:
Syntax and semantics
In most contexts where arbitrary Python expressions can be used, a named expression can appear. This is of the form NAME := expr where expr is any valid Python expression other than an unparenthesized tuple, and NAME is an identifier.
The value of such a named expression is the same as the incorporated expression, with the additional side-effect that the target is assigned that value:
There are a few places where assignment expressions are not allowed, in order to avoid ambiguities or user confusion:
This rule is included to simplify the choice for the user between an assignment statement and an assignment expression – there is no syntactic position where both are valid.
Again, this rule is included to avoid two visually similar ways of saying the same thing.
This rule is included to disallow excessively confusing code, and because parsing keyword arguments is complex enough already.
This rule is included to discourage side effects in a position whose exact semantics are already confusing to many users (cf. the common style recommendation against mutable default values), and also to echo the similar prohibition in calls (the previous bullet).
The reasoning here is similar to the two previous cases; this ungrouped assortment of symbols and operators composed of : and = is hard to read correctly.
This allows lambda to always bind less tightly than := ; having a name binding at the top level inside a lambda function is unlikely to be of value, as there is no way to make use of it. In cases where the name will be used more than once, the expression is likely to need parenthesizing anyway, so this prohibition will rarely affect code.
This shows that what looks like an assignment operator in an f-string is not always an assignment operator. The f-string parser uses : to indicate formatting options. To preserve backwards compatibility, assignment operator usage inside of f-strings must be parenthesized. As noted above, this usage of the assignment operator is not recommended.
An assignment expression does not introduce a new scope. In most cases the scope in which the target will be bound is self-explanatory: it is the current scope. If this scope contains a nonlocal or global declaration for the target, the assignment expression honors that. A lambda (being an explicit, if anonymous, function definition) counts as a scope for this purpose.
There is one special case: an assignment expression occurring in a list, set or dict comprehension or in a generator expression (below collectively referred to as “comprehensions”) binds the target in the containing scope, honoring a nonlocal or global declaration for the target in that scope, if one exists. For the purpose of this rule the containing scope of a nested comprehension is the scope that contains the outermost comprehension. A lambda counts as a containing scope.
The motivation for this special case is twofold. First, it allows us to conveniently capture a “witness” for an any() expression, or a counterexample for all() , for example:
Second, it allows a compact way of updating mutable state from a comprehension, for example:
However, an assignment expression target name cannot be the same as a for -target name appearing in any comprehension containing the assignment expression. The latter names are local to the comprehension in which they appear, so it would be contradictory for a contained use of the same name to refer to the scope containing the outermost comprehension instead.
For example, [i := i+1 for i in range(5)] is invalid: the for i part establishes that i is local to the comprehension, but the i := part insists that i is not local to the comprehension. The same reason makes these examples invalid too:
While it’s technically possible to assign consistent semantics to these cases, it’s difficult to determine whether those semantics actually make sense in the absence of real use cases. Accordingly, the reference implementation [1] will ensure that such cases raise SyntaxError , rather than executing with implementation defined behaviour.
This restriction applies even if the assignment expression is never executed:
For the comprehension body (the part before the first “for” keyword) and the filter expression (the part after “if” and before any nested “for”), this restriction applies solely to target names that are also used as iteration variables in the comprehension. Lambda expressions appearing in these positions introduce a new explicit function scope, and hence may use assignment expressions with no additional restrictions.
Due to design constraints in the reference implementation (the symbol table analyser cannot easily detect when names are re-used between the leftmost comprehension iterable expression and the rest of the comprehension), named expressions are disallowed entirely as part of comprehension iterable expressions (the part after each “in”, and before any subsequent “if” or “for” keyword):
A further exception applies when an assignment expression occurs in a comprehension whose containing scope is a class scope. If the rules above were to result in the target being assigned in that class’s scope, the assignment expression is expressly invalid. This case also raises SyntaxError :
(The reason for the latter exception is the implicit function scope created for comprehensions – there is currently no runtime mechanism for a function to refer to a variable in the containing class scope, and we do not want to add such a mechanism. If this issue ever gets resolved this special case may be removed from the specification of assignment expressions. Note that the problem already exists for using a variable defined in the class scope from a comprehension.)
See Appendix B for some examples of how the rules for targets in comprehensions translate to equivalent code.
The := operator groups more tightly than a comma in all syntactic positions where it is legal, but less tightly than all other operators, including or , and , not , and conditional expressions ( A if C else B ). As follows from section “Exceptional cases” above, it is never allowed at the same level as = . In case a different grouping is desired, parentheses should be used.
The := operator may be used directly in a positional function call argument; however it is invalid directly in a keyword argument.
Some examples to clarify what’s technically valid or invalid:
Most of the “valid” examples above are not recommended, since human readers of Python source code who are quickly glancing at some code may miss the distinction. But simple cases are not objectionable:
This PEP recommends always putting spaces around := , similar to PEP 8 ’s recommendation for = when used for assignment, whereas the latter disallows spaces around = used for keyword arguments.)
In order to have precisely defined semantics, the proposal requires evaluation order to be well-defined. This is technically not a new requirement, as function calls may already have side effects. Python already has a rule that subexpressions are generally evaluated from left to right. However, assignment expressions make these side effects more visible, and we propose a single change to the current evaluation order:
- In a dict comprehension {X: Y for ...} , Y is currently evaluated before X . We propose to change this so that X is evaluated before Y . (In a dict display like {X: Y} this is already the case, and also in dict((X, Y) for ...) which should clearly be equivalent to the dict comprehension.)
Most importantly, since := is an expression, it can be used in contexts where statements are illegal, including lambda functions and comprehensions.
Conversely, assignment expressions don’t support the advanced features found in assignment statements:
- Multiple targets are not directly supported: x = y = z = 0 # Equivalent: (z := (y := (x := 0)))
- Single assignment targets other than a single NAME are not supported: # No equivalent a [ i ] = x self . rest = []
- Priority around commas is different: x = 1 , 2 # Sets x to (1, 2) ( x := 1 , 2 ) # Sets x to 1
- Iterable packing and unpacking (both regular or extended forms) are not supported: # Equivalent needs extra parentheses loc = x , y # Use (loc := (x, y)) info = name , phone , * rest # Use (info := (name, phone, *rest)) # No equivalent px , py , pz = position name , phone , email , * other_info = contact
- Inline type annotations are not supported: # Closest equivalent is "p: Optional[int]" as a separate declaration p : Optional [ int ] = None
- Augmented assignment is not supported: total += tax # Equivalent: (total := total + tax)
The following changes have been made based on implementation experience and additional review after the PEP was first accepted and before Python 3.8 was released:
- for consistency with other similar exceptions, and to avoid locking in an exception name that is not necessarily going to improve clarity for end users, the originally proposed TargetScopeError subclass of SyntaxError was dropped in favour of just raising SyntaxError directly. [3]
- due to a limitation in CPython’s symbol table analysis process, the reference implementation raises SyntaxError for all uses of named expressions inside comprehension iterable expressions, rather than only raising them when the named expression target conflicts with one of the iteration variables in the comprehension. This could be revisited given sufficiently compelling examples, but the extra complexity needed to implement the more selective restriction doesn’t seem worthwhile for purely hypothetical use cases.
Examples from the Python standard library
env_base is only used on these lines, putting its assignment on the if moves it as the “header” of the block.
- Current: env_base = os . environ . get ( "PYTHONUSERBASE" , None ) if env_base : return env_base
- Improved: if env_base := os . environ . get ( "PYTHONUSERBASE" , None ): return env_base
Avoid nested if and remove one indentation level.
- Current: if self . _is_special : ans = self . _check_nans ( context = context ) if ans : return ans
- Improved: if self . _is_special and ( ans := self . _check_nans ( context = context )): return ans
Code looks more regular and avoid multiple nested if. (See Appendix A for the origin of this example.)
- Current: reductor = dispatch_table . get ( cls ) if reductor : rv = reductor ( x ) else : reductor = getattr ( x , "__reduce_ex__" , None ) if reductor : rv = reductor ( 4 ) else : reductor = getattr ( x , "__reduce__" , None ) if reductor : rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
- Improved: if reductor := dispatch_table . get ( cls ): rv = reductor ( x ) elif reductor := getattr ( x , "__reduce_ex__" , None ): rv = reductor ( 4 ) elif reductor := getattr ( x , "__reduce__" , None ): rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
tz is only used for s += tz , moving its assignment inside the if helps to show its scope.
- Current: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) tz = self . _tzstr () if tz : s += tz return s
- Improved: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) if tz := self . _tzstr (): s += tz return s
Calling fp.readline() in the while condition and calling .match() on the if lines make the code more compact without making it harder to understand.
- Current: while True : line = fp . readline () if not line : break m = define_rx . match ( line ) if m : n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v else : m = undef_rx . match ( line ) if m : vars [ m . group ( 1 )] = 0
- Improved: while line := fp . readline (): if m := define_rx . match ( line ): n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v elif m := undef_rx . match ( line ): vars [ m . group ( 1 )] = 0
A list comprehension can map and filter efficiently by capturing the condition:
Similarly, a subexpression can be reused within the main expression, by giving it a name on first use:
Note that in both cases the variable y is bound in the containing scope (i.e. at the same level as results or stuff ).
Assignment expressions can be used to good effect in the header of an if or while statement:
Particularly with the while loop, this can remove the need to have an infinite loop, an assignment, and a condition. It also creates a smooth parallel between a loop which simply uses a function call as its condition, and one which uses that as its condition but also uses the actual value.
An example from the low-level UNIX world:
Rejected alternative proposals
Proposals broadly similar to this one have come up frequently on python-ideas. Below are a number of alternative syntaxes, some of them specific to comprehensions, which have been rejected in favour of the one given above.
A previous version of this PEP proposed subtle changes to the scope rules for comprehensions, to make them more usable in class scope and to unify the scope of the “outermost iterable” and the rest of the comprehension. However, this part of the proposal would have caused backwards incompatibilities, and has been withdrawn so the PEP can focus on assignment expressions.
Broadly the same semantics as the current proposal, but spelled differently.
Since EXPR as NAME already has meaning in import , except and with statements (with different semantics), this would create unnecessary confusion or require special-casing (e.g. to forbid assignment within the headers of these statements).
(Note that with EXPR as VAR does not simply assign the value of EXPR to VAR – it calls EXPR.__enter__() and assigns the result of that to VAR .)
Additional reasons to prefer := over this spelling include:
- In if f(x) as y the assignment target doesn’t jump out at you – it just reads like if f x blah blah and it is too similar visually to if f(x) and y .
- import foo as bar
- except Exc as var
- with ctxmgr() as var
To the contrary, the assignment expression does not belong to the if or while that starts the line, and we intentionally allow assignment expressions in other contexts as well.
- NAME = EXPR
- if NAME := EXPR
reinforces the visual recognition of assignment expressions.
This syntax is inspired by languages such as R and Haskell, and some programmable calculators. (Note that a left-facing arrow y <- f(x) is not possible in Python, as it would be interpreted as less-than and unary minus.) This syntax has a slight advantage over ‘as’ in that it does not conflict with with , except and import , but otherwise is equivalent. But it is entirely unrelated to Python’s other use of -> (function return type annotations), and compared to := (which dates back to Algol-58) it has a much weaker tradition.
This has the advantage that leaked usage can be readily detected, removing some forms of syntactic ambiguity. However, this would be the only place in Python where a variable’s scope is encoded into its name, making refactoring harder.
Execution order is inverted (the indented body is performed first, followed by the “header”). This requires a new keyword, unless an existing keyword is repurposed (most likely with: ). See PEP 3150 for prior discussion on this subject (with the proposed keyword being given: ).
This syntax has fewer conflicts than as does (conflicting only with the raise Exc from Exc notation), but is otherwise comparable to it. Instead of paralleling with expr as target: (which can be useful but can also be confusing), this has no parallels, but is evocative.
One of the most popular use-cases is if and while statements. Instead of a more general solution, this proposal enhances the syntax of these two statements to add a means of capturing the compared value:
This works beautifully if and ONLY if the desired condition is based on the truthiness of the captured value. It is thus effective for specific use-cases (regex matches, socket reads that return '' when done), and completely useless in more complicated cases (e.g. where the condition is f(x) < 0 and you want to capture the value of f(x) ). It also has no benefit to list comprehensions.
Advantages: No syntactic ambiguities. Disadvantages: Answers only a fraction of possible use-cases, even in if / while statements.
Another common use-case is comprehensions (list/set/dict, and genexps). As above, proposals have been made for comprehension-specific solutions.
This brings the subexpression to a location in between the ‘for’ loop and the expression. It introduces an additional language keyword, which creates conflicts. Of the three, where reads the most cleanly, but also has the greatest potential for conflict (e.g. SQLAlchemy and numpy have where methods, as does tkinter.dnd.Icon in the standard library).
As above, but reusing the with keyword. Doesn’t read too badly, and needs no additional language keyword. Is restricted to comprehensions, though, and cannot as easily be transformed into “longhand” for-loop syntax. Has the C problem that an equals sign in an expression can now create a name binding, rather than performing a comparison. Would raise the question of why “with NAME = EXPR:” cannot be used as a statement on its own.
As per option 2, but using as rather than an equals sign. Aligns syntactically with other uses of as for name binding, but a simple transformation to for-loop longhand would create drastically different semantics; the meaning of with inside a comprehension would be completely different from the meaning as a stand-alone statement, while retaining identical syntax.
Regardless of the spelling chosen, this introduces a stark difference between comprehensions and the equivalent unrolled long-hand form of the loop. It is no longer possible to unwrap the loop into statement form without reworking any name bindings. The only keyword that can be repurposed to this task is with , thus giving it sneakily different semantics in a comprehension than in a statement; alternatively, a new keyword is needed, with all the costs therein.
There are two logical precedences for the := operator. Either it should bind as loosely as possible, as does statement-assignment; or it should bind more tightly than comparison operators. Placing its precedence between the comparison and arithmetic operators (to be precise: just lower than bitwise OR) allows most uses inside while and if conditions to be spelled without parentheses, as it is most likely that you wish to capture the value of something, then perform a comparison on it:
Once find() returns -1, the loop terminates. If := binds as loosely as = does, this would capture the result of the comparison (generally either True or False ), which is less useful.
While this behaviour would be convenient in many situations, it is also harder to explain than “the := operator behaves just like the assignment statement”, and as such, the precedence for := has been made as close as possible to that of = (with the exception that it binds tighter than comma).
Some critics have claimed that the assignment expressions should allow unparenthesized tuples on the right, so that these two would be equivalent:
(With the current version of the proposal, the latter would be equivalent to ((point := x), y) .)
However, adopting this stance would logically lead to the conclusion that when used in a function call, assignment expressions also bind less tight than comma, so we’d have the following confusing equivalence:
The less confusing option is to make := bind more tightly than comma.
It’s been proposed to just always require parentheses around an assignment expression. This would resolve many ambiguities, and indeed parentheses will frequently be needed to extract the desired subexpression. But in the following cases the extra parentheses feel redundant:
Frequently Raised Objections
C and its derivatives define the = operator as an expression, rather than a statement as is Python’s way. This allows assignments in more contexts, including contexts where comparisons are more common. The syntactic similarity between if (x == y) and if (x = y) belies their drastically different semantics. Thus this proposal uses := to clarify the distinction.
The two forms have different flexibilities. The := operator can be used inside a larger expression; the = statement can be augmented to += and its friends, can be chained, and can assign to attributes and subscripts.
Previous revisions of this proposal involved sublocal scope (restricted to a single statement), preventing name leakage and namespace pollution. While a definite advantage in a number of situations, this increases complexity in many others, and the costs are not justified by the benefits. In the interests of language simplicity, the name bindings created here are exactly equivalent to any other name bindings, including that usage at class or module scope will create externally-visible names. This is no different from for loops or other constructs, and can be solved the same way: del the name once it is no longer needed, or prefix it with an underscore.
(The author wishes to thank Guido van Rossum and Christoph Groth for their suggestions to move the proposal in this direction. [2] )
As expression assignments can sometimes be used equivalently to statement assignments, the question of which should be preferred will arise. For the benefit of style guides such as PEP 8 , two recommendations are suggested.
- If either assignment statements or assignment expressions can be used, prefer statements; they are a clear declaration of intent.
- If using assignment expressions would lead to ambiguity about execution order, restructure it to use statements instead.
The authors wish to thank Alyssa Coghlan and Steven D’Aprano for their considerable contributions to this proposal, and members of the core-mentorship mailing list for assistance with implementation.
Appendix A: Tim Peters’s findings
Here’s a brief essay Tim Peters wrote on the topic.
I dislike “busy” lines of code, and also dislike putting conceptually unrelated logic on a single line. So, for example, instead of:
instead. So I suspected I’d find few places I’d want to use assignment expressions. I didn’t even consider them for lines already stretching halfway across the screen. In other cases, “unrelated” ruled:
is a vast improvement over the briefer:
The original two statements are doing entirely different conceptual things, and slamming them together is conceptually insane.
In other cases, combining related logic made it harder to understand, such as rewriting:
as the briefer:
The while test there is too subtle, crucially relying on strict left-to-right evaluation in a non-short-circuiting or method-chaining context. My brain isn’t wired that way.
But cases like that were rare. Name binding is very frequent, and “sparse is better than dense” does not mean “almost empty is better than sparse”. For example, I have many functions that return None or 0 to communicate “I have nothing useful to return in this case, but since that’s expected often I’m not going to annoy you with an exception”. This is essentially the same as regular expression search functions returning None when there is no match. So there was lots of code of the form:
I find that clearer, and certainly a bit less typing and pattern-matching reading, as:
It’s also nice to trade away a small amount of horizontal whitespace to get another _line_ of surrounding code on screen. I didn’t give much weight to this at first, but it was so very frequent it added up, and I soon enough became annoyed that I couldn’t actually run the briefer code. That surprised me!
There are other cases where assignment expressions really shine. Rather than pick another from my code, Kirill Balunov gave a lovely example from the standard library’s copy() function in copy.py :
The ever-increasing indentation is semantically misleading: the logic is conceptually flat, “the first test that succeeds wins”:
Using easy assignment expressions allows the visual structure of the code to emphasize the conceptual flatness of the logic; ever-increasing indentation obscured it.
A smaller example from my code delighted me, both allowing to put inherently related logic in a single line, and allowing to remove an annoying “artificial” indentation level:
That if is about as long as I want my lines to get, but remains easy to follow.
So, in all, in most lines binding a name, I wouldn’t use assignment expressions, but because that construct is so very frequent, that leaves many places I would. In most of the latter, I found a small win that adds up due to how often it occurs, and in the rest I found a moderate to major win. I’d certainly use it more often than ternary if , but significantly less often than augmented assignment.
I have another example that quite impressed me at the time.
Where all variables are positive integers, and a is at least as large as the n’th root of x, this algorithm returns the floor of the n’th root of x (and roughly doubling the number of accurate bits per iteration):
It’s not obvious why that works, but is no more obvious in the “loop and a half” form. It’s hard to prove correctness without building on the right insight (the “arithmetic mean - geometric mean inequality”), and knowing some non-trivial things about how nested floor functions behave. That is, the challenges are in the math, not really in the coding.
If you do know all that, then the assignment-expression form is easily read as “while the current guess is too large, get a smaller guess”, where the “too large?” test and the new guess share an expensive sub-expression.
To my eyes, the original form is harder to understand:
This appendix attempts to clarify (though not specify) the rules when a target occurs in a comprehension or in a generator expression. For a number of illustrative examples we show the original code, containing a comprehension, and the translation, where the comprehension has been replaced by an equivalent generator function plus some scaffolding.
Since [x for ...] is equivalent to list(x for ...) these examples all use list comprehensions without loss of generality. And since these examples are meant to clarify edge cases of the rules, they aren’t trying to look like real code.
Note: comprehensions are already implemented via synthesizing nested generator functions like those in this appendix. The new part is adding appropriate declarations to establish the intended scope of assignment expression targets (the same scope they resolve to as if the assignment were performed in the block containing the outermost comprehension). For type inference purposes, these illustrative expansions do not imply that assignment expression targets are always Optional (but they do indicate the target binding scope).
Let’s start with a reminder of what code is generated for a generator expression without assignment expression.
- Original code (EXPR usually references VAR): def f (): a = [ EXPR for VAR in ITERABLE ]
- Translation (let’s not worry about name conflicts): def f (): def genexpr ( iterator ): for VAR in iterator : yield EXPR a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a simple assignment expression.
- Original code: def f (): a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): if False : TARGET = None # Dead code to ensure TARGET is a local variable def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a global TARGET declaration in f() .
- Original code: def f (): global TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): global TARGET def genexpr ( iterator ): global TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Or instead let’s add a nonlocal TARGET declaration in f() .
- Original code: def g (): TARGET = ... def f (): nonlocal TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def g (): TARGET = ... def f (): nonlocal TARGET def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Finally, let’s nest two comprehensions.
- Original code: def f (): a = [[ TARGET := i for i in range ( 3 )] for j in range ( 2 )] # I.e., a = [[0, 1, 2], [0, 1, 2]] print ( TARGET ) # prints 2
- Translation: def f (): if False : TARGET = None def outer_genexpr ( outer_iterator ): nonlocal TARGET def inner_generator ( inner_iterator ): nonlocal TARGET for i in inner_iterator : TARGET = i yield i for j in outer_iterator : yield list ( inner_generator ( range ( 3 ))) a = list ( outer_genexpr ( range ( 2 ))) print ( TARGET )
Because it has been a point of confusion, note that nothing about Python’s scoping semantics is changed. Function-local scopes continue to be resolved at compile time, and to have indefinite temporal extent at run time (“full closures”). Example:
This document has been placed in the public domain.
Source: https://github.com/python/peps/blob/main/peps/pep-0572.rst
Last modified: 2023-10-11 12:05:51 GMT
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- How to Get Started With Python?
- Python Comments
- Python Variables, Constants and Literals
- Python Type Conversion
- Python Basic Input and Output
- Python Operators
- Precedence and Associativity of Operators in Python (programiz.com)
Python Flow Control
- Python if...else Statement
- Python for Loop
Python while Loop
Python break and continue
Python pass Statement
Python Data types
- Python Numbers, Type Conversion and Mathematics
- Python List
- Python Tuple
- Python Sets
- Python Dictionary
- Python String
- Python Functions
- Python Function Arguments
- Python Variable Scope
- Python Global Keyword
- Python Recursion
- Python Modules
- Python Package
- Python Main function (programiz.com)

Python Files
- Python Directory and Files Management
- Python CSV: Read and Write CSV files (programiz.com)
- Reading CSV files in Python (programiz.com)
- Writing CSV files in Python (programiz.com)
- Python Exception Handling
- Python Exceptions
- Python Custom Exceptions
Python Object & Class
- Python Objects and Classes
- Python Inheritance
- Python Multiple Inheritance
- Polymorphism in Python(with Examples) (programiz.com)
- Python Operator Overloading
Python Advanced Topics
- List comprehension
- Python Lambda/Anonymous Function
- Python Iterators
- Python Generators
- Python Namespace and Scope
- Python Closures
- Python Decorators
- Python @property decorator
- Python RegEx
Python Date and Time
- Python datetime
- Python strftime()
- Python strptime()
- How to get current date and time in Python?
- Python Get Current Time
- Python timestamp to datetime and vice-versa
- Python time Module
- Python sleep()
Additional Topic
- Python Keywords and Identifiers
- Python Asserts
- Python Json
- Python *args and **kwargs (With Examples) (programiz.com)
Python Tutorials
Python Assert Statement
- List of Keywords in Python
In computer programming, the if statement is a conditional statement. It is used to execute a block of code only when a specific condition is met. For example,
Suppose we need to assign different grades to students based on their scores.
- If a student scores above 90 , assign grade A
- If a student scores above 75 , assign grade B
- If a student scores above 65 , assign grade C
These conditional tasks can be achieved using the if statement.
- Python if Statement
An if statement executes a block of code only if the specified condition is met.
Here, if the condition of the if statement is:
- True - the body of the if statement executes.
- False - the body of the if statement is skipped from execution.
Let's look at an example.
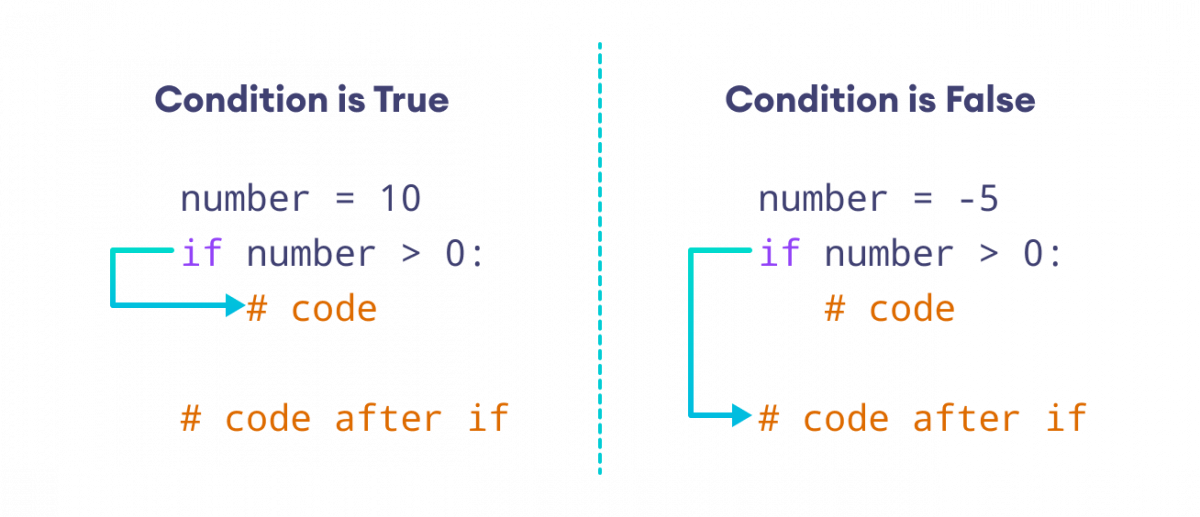
Note: Be mindful of the indentation while writing the if statements. Indentation is the whitespace at the beginning of the code.
Here, the spaces before the print() statement denote that it's the body of the if statement.
- Example: Python if Statement
Sample Output 1
In the above example, we have created a variable named number . Notice the test condition ,
As the number is greater than 0 , the condition evaluates True . Hence, the body of the if statement executes.
Sample Output 2
Now, let's change the value of the number to a negative integer, say -5 .
Now, when we run the program, the output will be:
This is because the value of the number is less than 0 . Hence, the condition evaluates to False . And, the body of the if statement is skipped.
An if statement can have an optional else clause. The else statement executes if the condition in the if statement evaluates to False .
Here, if the condition inside the if statement evaluates to
- True - the body of if executes, and the body of else is skipped.
- False - the body of else executes, and the body of if is skipped
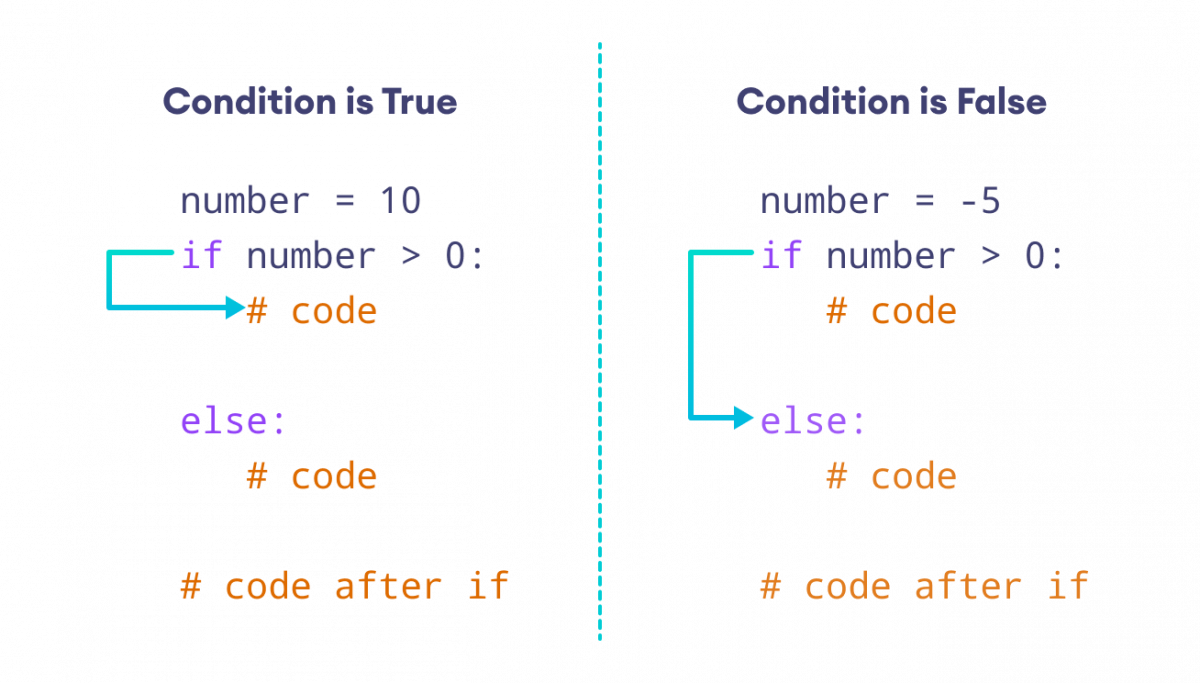
- Example: Python if…else Statement
In the above example, we have created a variable named number .
Since the value of the number is 10 , the condition evaluates to True . Hence, code inside the body of if is executed.
If we change the value of the variable to a negative integer, let's say -5 , our output will be:
Here, the test condition evaluates to False . Hence code inside the body of else is executed.
- Python if…elif…else Statement
The if...else statement is used to execute a block of code among two alternatives.
However, if we need to make a choice between more than two alternatives, we use the if...elif...else statement.
- if condition1 - This checks if condition1 is True . If it is, the program executes code block 1 .
- elif condition2 - If condition1 is not True , the program checks condition2 . If condition2 is True , it executes code block 2 .
- else - If neither condition1 nor condition2 is True , the program defaults to executing code block 3 .
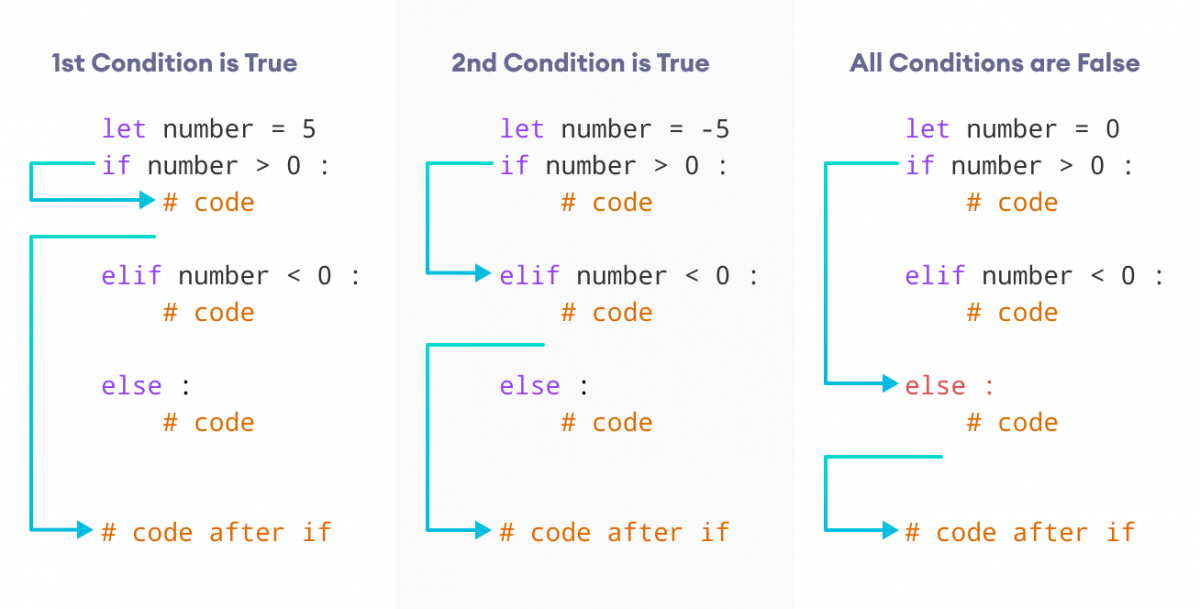
- Example: Python if…elif…else Statement
Since the value of the number is 0 , both the test conditions evaluate to False .
Hence, the statement inside the body of else is executed.
- Python Nested if Statements
It is possible to include an if statement inside another if statement. For example,
Here's how this program works.
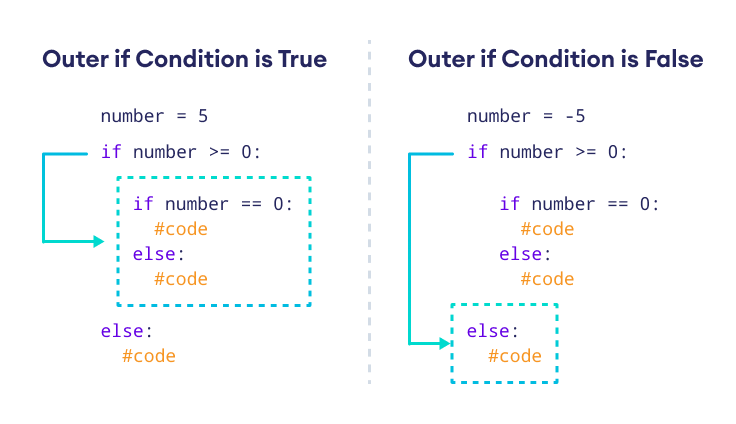
More on Python if…else Statement
In certain situations, the if statement can be simplified into a single line. For example,
This code can be compactly written as
This one-liner approach retains the same functionality but in a more concise format.
Python doesn't have a ternary operator. However, we can use if...else to work like a ternary operator in other languages. For example,
can be written as
We can use logical operators such as and and or within an if statement.
Here, we used the logical operator and to add two conditions in the if statement.
We also used >= (comparison operator) to compare two values.
Logical and comparison operators are often used with if...else statements. Visit Python Operators to learn more.
Table of Contents
- Introduction
Video: Python if...else Statement
Sorry about that.
Related Tutorials
Python Tutorial
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Logical Operators in Python with Examples
- How To Do Math in Python 3 with Operators?
- Python 3 - Logical Operators
- Understanding Boolean Logic in Python 3
- Concatenate two strings using Operator Overloading in Python
- Relational Operators in Python
- Difference between "__eq__" VS "is" VS "==" in Python
- Modulo operator (%) in Python
- Python Bitwise Operators
- Python - Star or Asterisk operator ( * )
- New '=' Operator in Python3.8 f-string
- Format a Number Width in Python
- Difference between != and is not operator in Python
- Operator Overloading in Python
- Python Object Comparison : "is" vs "=="
- Python | a += b is not always a = a + b
- Python Arithmetic Operators
- Python Operators
- Python | Operator.countOf
Assignment Operators in Python
Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, bitwise computations. The value the operator operates on is known as Operand .
Here, we will cover Assignment Operators in Python. So, Assignment Operators are used to assigning values to variables.
Now Let’s see each Assignment Operator one by one.
1) Assign: This operator is used to assign the value of the right side of the expression to the left side operand.
2) Add and Assign: This operator is used to add the right side operand with the left side operand and then assigning the result to the left operand.
Syntax:
3) Subtract and Assign: This operator is used to subtract the right operand from the left operand and then assigning the result to the left operand.
Example –
4) Multiply and Assign: This operator is used to multiply the right operand with the left operand and then assigning the result to the left operand.
5) Divide and Assign: This operator is used to divide the left operand with the right operand and then assigning the result to the left operand.
6) Modulus and Assign: This operator is used to take the modulus using the left and the right operands and then assigning the result to the left operand.
7) Divide (floor) and Assign: This operator is used to divide the left operand with the right operand and then assigning the result(floor) to the left operand.
8) Exponent and Assign: This operator is used to calculate the exponent(raise power) value using operands and then assigning the result to the left operand.
9) Bitwise AND and Assign: This operator is used to perform Bitwise AND on both operands and then assigning the result to the left operand.
10) Bitwise OR and Assign: This operator is used to perform Bitwise OR on the operands and then assigning result to the left operand.
11) Bitwise XOR and Assign: This operator is used to perform Bitwise XOR on the operands and then assigning result to the left operand.
12) Bitwise Right Shift and Assign: This operator is used to perform Bitwise right shift on the operands and then assigning result to the left operand.
13) Bitwise Left Shift and Assign: This operator is used to perform Bitwise left shift on the operands and then assigning result to the left operand.
Please Login to comment...
Similar reads.
- Python-Operators
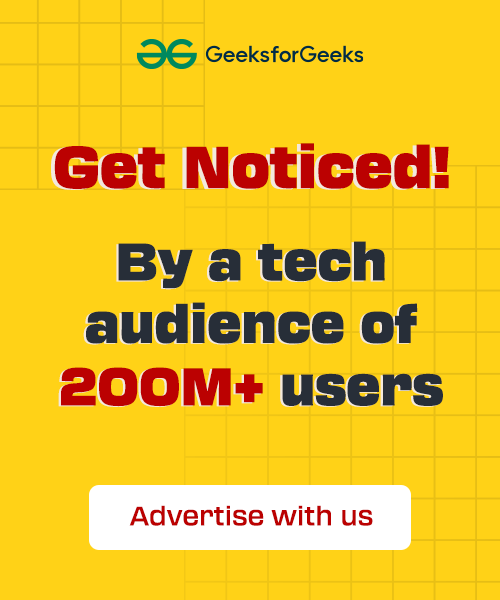
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Assignment operator in python.
Last Updated on June 8, 2023 by Prepbytes
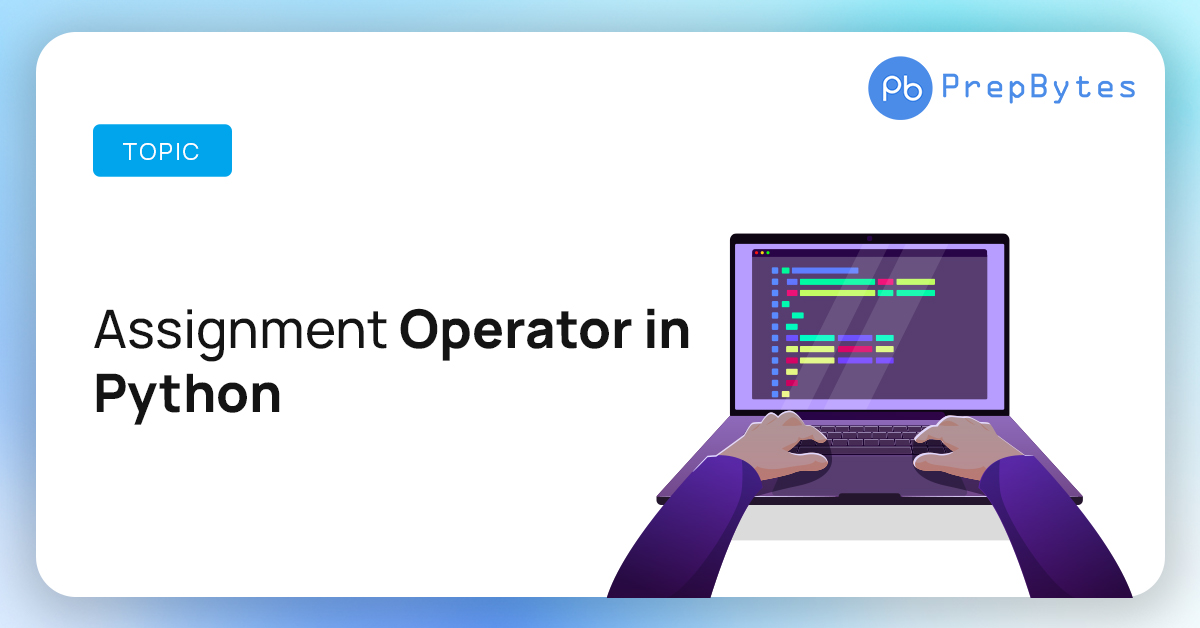
To fully comprehend the assignment operators in Python, it is important to have a basic understanding of what operators are. Operators are utilized to carry out a variety of operations, including mathematical, bitwise, and logical operations, among others, by connecting operands. Operands are the values that are acted upon by operators. In Python, the assignment operator is used to assign a value to a variable. The assignment operator is represented by the equals sign (=), and it is the most commonly used operator in Python. In this article, we will explore the assignment operator in Python, how it works, and its different types.
What is an Assignment Operator in Python?
The assignment operator in Python is used to assign a value to a variable. The assignment operator is represented by the equals sign (=), and it is used to assign a value to a variable. When an assignment operator is used, the value on the right-hand side is assigned to the variable on the left-hand side. This is a fundamental operation in programming, as it allows developers to store data in variables that can be used throughout their code.
For example, consider the following line of code:
Explanation: In this case, the value 10 is assigned to the variable a using the assignment operator. The variable a now holds the value 10, and this value can be used in other parts of the code. This simple example illustrates the basic usage and importance of assignment operators in Python programming.
Types of Assignment Operator in Python
There are several types of assignment operator in Python that are used to perform different operations. Let’s explore each type of assignment operator in Python in detail with the help of some code examples.
1. Simple Assignment Operator (=)
The simple assignment operator is the most commonly used operator in Python. It is used to assign a value to a variable. The syntax for the simple assignment operator is:
Here, the value on the right-hand side of the equals sign is assigned to the variable on the left-hand side. For example
Explanation: In this case, the value 25 is assigned to the variable a using the simple assignment operator. The variable a now holds the value 25.
2. Addition Assignment Operator (+=)
The addition assignment operator is used to add a value to a variable and store the result in the same variable. The syntax for the addition assignment operator is:
Here, the value on the right-hand side is added to the variable on the left-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is incremented by 5 using the addition assignment operator. The result, 15, is then printed to the console.
3. Subtraction Assignment Operator (-=)
The subtraction assignment operator is used to subtract a value from a variable and store the result in the same variable. The syntax for the subtraction assignment operator is
Here, the value on the right-hand side is subtracted from the variable on the left-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is decremented by 5 using the subtraction assignment operator. The result, 5, is then printed to the console.
4. Multiplication Assignment Operator (*=)
The multiplication assignment operator is used to multiply a variable by a value and store the result in the same variable. The syntax for the multiplication assignment operator is:
Here, the value on the right-hand side is multiplied by the variable on the left-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is multiplied by 5 using the multiplication assignment operator. The result, 50, is then printed to the console.
5. Division Assignment Operator (/=)
The division assignment operator is used to divide a variable by a value and store the result in the same variable. The syntax for the division assignment operator is:
Here, the variable on the left-hand side is divided by the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is divided by 5 using the division assignment operator. The result, 2.0, is then printed to the console.
6. Modulus Assignment Operator (%=)
The modulus assignment operator is used to find the remainder of the division of a variable by a value and store the result in the same variable. The syntax for the modulus assignment operator is
Here, the variable on the left-hand side is divided by the value on the right-hand side, and the remainder is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is divided by 3 using the modulus assignment operator. The remainder, 1, is then printed to the console.
7. Floor Division Assignment Operator (//=)
The floor division assignment operator is used to divide a variable by a value and round the result down to the nearest integer, and store the result in the same variable. The syntax for the floor division assignment operator is:
Here, the variable on the left-hand side is divided by the value on the right-hand side, and the result is rounded down to the nearest integer. The rounded result is then stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is divided by 3 using the floor division assignment operator. The result, 3, is then printed to the console.
8. Exponentiation Assignment Operator (**=)
The exponentiation assignment operator is used to raise a variable to the power of a value and store the result in the same variable. The syntax for the exponentiation assignment operator is:
Here, the variable on the left-hand side is raised to the power of the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is raised to the power of 3 using the exponentiation assignment operator. The result, 8, is then printed to the console.
9. Bitwise AND Assignment Operator (&=)
The bitwise AND assignment operator is used to perform a bitwise AND operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise AND assignment operator is:
Here, the variable on the left-hand side is ANDed with the value on the right-hand side using the bitwise AND operator, and the result is stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is ANDed with 3 using the bitwise AND assignment operator. The result, 2, is then printed to the console.
10. Bitwise OR Assignment Operator (|=)
The bitwise OR assignment operator is used to perform a bitwise OR operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise OR assignment operator is:
Here, the variable on the left-hand side is ORed with the value on the right-hand side using the bitwise OR operator, and the result is stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is ORed with 3 using the bitwise OR assignment operator. The result, 7, is then printed to the console.
11. Bitwise XOR Assignment Operator (^=)
The bitwise XOR assignment operator is used to perform a bitwise XOR operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise XOR assignment operator is:
Here, the variable on the left-hand side is XORed with the value on the right-hand side using the bitwise XOR operator, and the result are stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is XORed with 3 using the bitwise XOR assignment operator. The result, 5, is then printed to the console.
12. Bitwise Right Shift Assignment Operator (>>=)
The bitwise right shift assignment operator is used to shift the bits of a variable to the right by a specified number of positions, and store the result in the same variable. The syntax for the bitwise right shift assignment operator is:
Here, the variable on the left-hand side has its bits shifted to the right by the number of positions specified by the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is shifted 2 positions to the right using the bitwise right shift assignment operator. The result, 2, is then printed to the console.
13. Bitwise Left Shift Assignment Operator (<<=)
The bitwise left shift assignment operator is used to shift the bits of a variable to the left by a specified number of positions, and store the result in the same variable. The syntax for the bitwise left shift assignment operator is:
Here, the variable on the left-hand side has its bits shifted to the left by the number of positions specified by the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example,
Conclusion Assignment operator in Python is used to assign values to variables, and it comes in different types. The simple assignment operator (=) assigns a value to a variable. The augmented assignment operators (+=, -=, *=, /=, %=, &=, |=, ^=, >>=, <<=) perform a specified operation and assign the result to the same variable in one step. The modulus assignment operator (%) calculates the remainder of a division operation and assigns the result to the same variable. The bitwise assignment operators (&=, |=, ^=, >>=, <<=) perform bitwise operations and assign the result to the same variable. The bitwise right shift assignment operator (>>=) shifts the bits of a variable to the right by a specified number of positions and stores the result in the same variable. The bitwise left shift assignment operator (<<=) shifts the bits of a variable to the left by a specified number of positions and stores the result in the same variable. These operators are useful in simplifying and shortening code that involves assigning and manipulating values in a single step.
Here are some Frequently Asked Questions on Assignment Operator in Python:
Q1 – Can I use the assignment operator to assign multiple values to multiple variables at once? Ans – Yes, you can use the assignment operator to assign multiple values to multiple variables at once, separated by commas. For example, "x, y, z = 1, 2, 3" would assign the value 1 to x, 2 to y, and 3 to z.
Q2 – Is it possible to chain assignment operators in Python? Ans – Yes, you can chain assignment operators in Python to perform multiple operations in one line of code. For example, "x = y = z = 1" would assign the value 1 to all three variables.
Q3 – How do I perform a conditional assignment in Python? Ans – To perform a conditional assignment in Python, you can use the ternary operator. For example, "x = a (if a > b) else b" would assign the value of a to x if a is greater than b, otherwise it would assign the value of b to x.
Q4 – What happens if I use an undefined variable in an assignment operation in Python? Ans – If you use an undefined variable in an assignment operation in Python, you will get a NameError. Make sure you have defined the variable before trying to assign a value to it.
Q5 – Can I use assignment operators with non-numeric data types in Python? Ans – Yes, you can use assignment operators with non-numeric data types in Python, such as strings or lists. For example, "my_list += [4, 5, 6]" would append the values 4, 5, and 6 to the end of the list named my_list.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Python list functions & python list methods, python interview questions, namespaces and scope in python, what is the difference between append and extend in python, python program to check for the perfect square, python program to find the sum of first n natural numbers.
brandon-pearson
Brandon Pearson
Assignment-7-Using If-Condition and Loops in Python
Leave a reply cancel reply.
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.

IMAGES
VIDEO
COMMENTS
The assignment operator - also known informally as the the walrus operator - was created at 28-Feb-2018 in PEP572. ... Why are complex arithmetic operation not allowed in the while condition in python. 0. Start Python 'while' loop before condition is defined-1.
For the future time traveler from Google, here is a new way (available from Python 3.8 onward): b = 1 if a := b: # this section is only reached if b is not 0 or false. # Also, a is set to b print(a, b) This is known as "the walrus operator". More info at the What's New In Python 3.8 page.
Let's see a code snippet to understand it better. a = 10. b = 20 # assigning value to variable c based on condition. c = a if a > b else b. print(c) # output: 20. You can see we have conditionally assigned a value to variable c based on the condition a > b. 2. Using if-else statement.
Python supports one additional decision-making entity called a conditional expression. (It is also referred to as a conditional operator or ternary operator in various places in the Python documentation.) Conditional expressions were proposed for addition to the language in PEP 308 and green-lighted by Guido in 2005.
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
In its simplest form, a conditional statement requires only an if clause. else and elif clauses can only follow an if clause. # A conditional statement consisting of # an "if"-clause, only. x = -1 if x < 0: x = x ** 2 # x is now 1. Similarly, conditional statements can have an if and an else without an elif:
In this lesson, you'll learn about the biggest change in Python 3.8: the introduction of assignment expressions.Assignment expression are written with a new notation (:=).This operator is often called the walrus operator as it resembles the eyes and tusks of a walrus on its side.. Assignment expressions allow you to assign and return a value in the same expression.
Meaning of ||= Operator in Ruby. x ||= y. The basic meaning of this operator is to assign the value of the variable y to variable x if variable x is undefined or is falsy value, otherwise no assignment operation is performed. But this operator is much more complex and confusing than other simpler conditional operators like +=, -= because ...
Basics of the conditional expression (ternary operator) In Python, the conditional expression is written as follows. The condition is evaluated first. If condition is True, X is evaluated and its value is returned, and if condition is False, Y is evaluated and its value is returned. If you want to switch the value based on a condition, simply ...
The author selected the COVID-19 Relief Fund to receive a donation as part of the Write for DOnations program.. Introduction. Python 3.8, released in October 2019, adds assignment expressions to Python via the := syntax. The assignment expression syntax is also sometimes called "the walrus operator" because := vaguely resembles a walrus with tusks. ...
In this guide, we covered the basics of conditionals in Python including operators, complex conditional chains, nesting conditionals, ternary expressions, and common errors. We examined real-world examples of using conditional logic for input validation, handling user types, recommendation systems, data analysis, and game mechanics.
Tuple Syntax of the Ternary Operator. In fact, the order of the <OnFalse> and <OnTrue> operands is just flipped when compared to the basic ternary operator. First, you have the branch that's returned if the condition does NOT hold. Second, you run the branch that's returned if the condition holds. x = (x, 42)[boo] Clever!
The Python ternary Expression determines if a condition is true or false and then returns the appropriate value in accordance with the result. The ternary Expression is useful in cases where we need to assign a value to a variable based on a simple condition, and we want to keep our code more concise — all in just one line of code.
Each new version of Python adds new features to the language. For Python 3.8, the biggest change is the addition of assignment expressions.Specifically, the := operator gives you a new syntax for assigning variables in the middle of expressions. This operator is colloquially known as the walrus operator.. This tutorial is an in-depth introduction to the walrus operator.
Python Enhancement Proposals. Python » PEP Index » PEP 572; ... This shows that what looks like an assignment operator in an f-string is not always an assignment operator. ... this can remove the need to have an infinite loop, an assignment, and a condition. It also creates a smooth parallel between a loop which simply uses a function call as ...
Python if Statement. An if statement executes a block of code only if the specified condition is met.. Syntax. if condition: # body of if statement. Here, if the condition of the if statement is: . True - the body of the if statement executes.; False - the body of the if statement is skipped from execution.; Let's look at an example. Working of if Statement
Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, bitwise computations. The value the operator operates on is known as Operand. Here, we will cover Assignment Operators in Python. So, Assignment Operators are used to assigning values to variables.
The simple assignment operator is the most commonly used operator in Python. It is used to assign a value to a variable. The syntax for the simple assignment operator is: variable = value. Here, the value on the right-hand side of the equals sign is assigned to the variable on the left-hand side. For example.
Assignment-7-Using If-Condition and Loops in Python. Posted by bpear003 on April 20, 2024. assignment-week-10-Cybersecurity-Programming-1 Download. annotated-python20proj207 Download. Previous PostAssignment-5-Data Type and List. Next PostAssignment-8-While Loop in Python.
(In 3.8 and above, the := "walrus" operator allows simple assignment of values as an expression, which is then compatible with this syntax. But please don't write code like that; it will quickly become very difficult to understand.) ... Yes, Python has a ternary conditional operator, also known as the conditional expression or the ternary ...