

C# - Ternary Operator ?:
C# includes a decision-making operator ?: which is called the conditional operator or ternary operator. It is the short form of the if else conditions.
The ternary operator starts with a boolean condition. If this condition evaluates to true then it will execute the first statement after ? , otherwise the second statement after : will be executed.
The following example demonstrates the ternary operator.
Above, a conditional expression x > y returns true, so the first statement after ? will be execute.
The following executes the second statement.
Thus, a ternary operator is short form of if else statement. The above example can be re-write using if else condition, as shown below.
Nested Ternary Operator
Nested ternary operators are possible by including a conditional expression as a second statement.
The ternary operator is right-associative. The expression a ? b : c ? d : e is evaluated as a ? b : (c ? d : e) , not as (a ? b : c) ? d : e .
- Difference between Array and ArrayList
- Difference between Hashtable and Dictionary
- How to write file using StreamWriter in C#?
- How to sort the generic SortedList in the descending order?
- Difference between delegates and events in C#
- How to read file using StreamReader in C#?
- How to calculate the code execution time in C#?
- Design Principle vs Design Pattern
- How to convert string to int in C#?
- Boxing and Unboxing in C#
- More C# articles
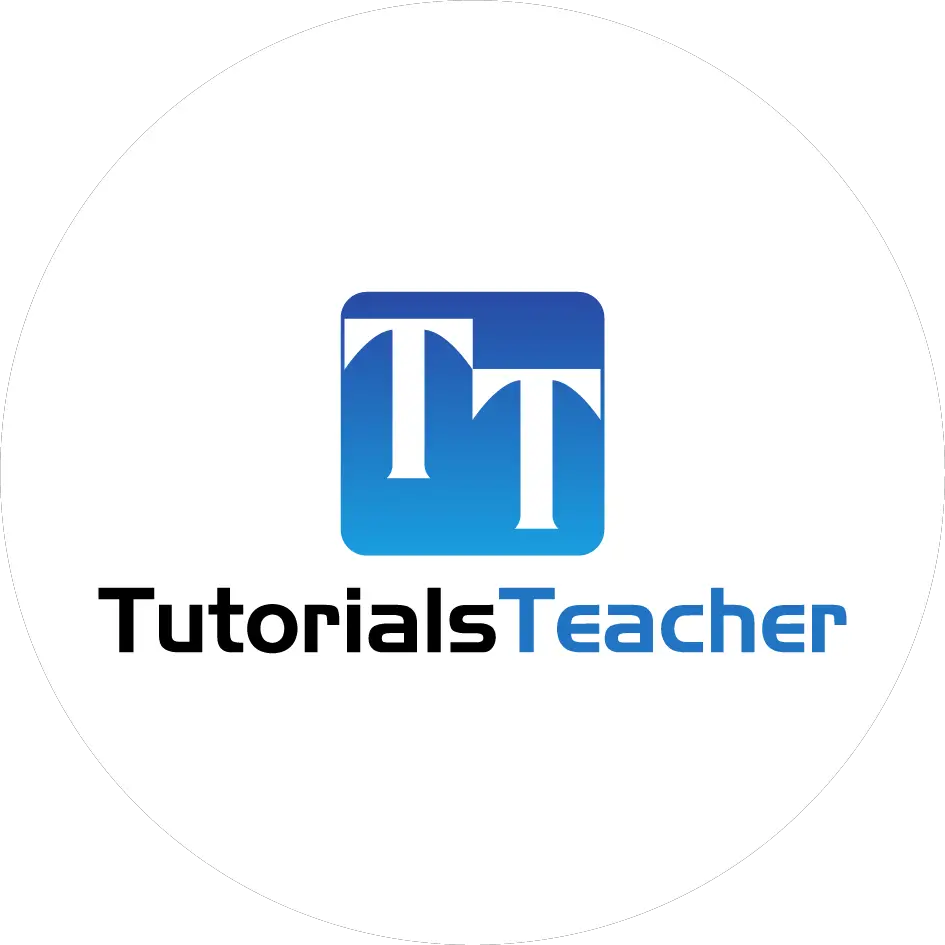
We are a team of passionate developers, educators, and technology enthusiasts who, with their combined expertise and experience, create in -depth, comprehensive, and easy to understand tutorials.We focus on a blend of theoretical explanations and practical examples to encourages hands - on learning. Visit About Us page for more information.
- C# Questions & Answers
- C# Skill Test
- C# Latest Articles

Conditional operator(?:) in C#
Conditional operator (?:) in C# is used as a single line if-else assignment statement, it is also known as Ternary Operator in C#. It evaluates a Boolean expression and on the basis of the evaluated True and False value, it executes corresponding statement.
Precisely, conditional operator (?:) can be explained as follows.
It has three operands: condition , consequence and alternative . The conditional expression returns a Boolean value as true or false . If the value is true , then it evaluates the consequence expression. If false , then it evaluates the alternative expression.
Syntax of c onditional operator (?:) in C#
It uses a question mark ( ? ) operator and a colon ( : ) operator , the general format is as follows.
Condition ( Boolean expression ) ? consequence ( if true ) : alternative ( if false )
Lets look at below example, here we are doing a conditional check and based on the true/false, assigning the variable value to taxPercentage .
isSeniorCitizen = true; taxPercentage = (isSeniorCitizen) ? 20 : 30; |
In above case, If isSiniorCitizen == true then taxPercentage will be 20 else it will be 30 , in the above case it sets to 20 since we have already set the boolean value isSiniorCitizen = true .
Example of (?:) operator in C#
Lets look at an example.
System; ConditionalOperatorExample class Program { static void Main(string[] args) { string FirstName = "Ramesh"; string LastName = ""; //True statement string FullName = (String.IsNullOrEmpty(LastName)) ? FirstName : (FirstName + " " + LastName); Console.WriteLine(FullName); } } |
In above example code snippet, the boolean expression String . IsNullOrEmpty ( LastName ) returns True value, so the consequence statement executes, which gives output as “Ramesh” .

Lets take another example, where conditional expression returns False value.
System; ConditionalOperatorExample class Program { static void Main(string[] args) { string FirstName = "Ramesh"; string LastName = "Kumar"; //False statement string FullName = (String.IsNullOrEmpty(LastName)) ? FirstName : (FirstName + " " + LastName); Console.WriteLine(FullName); } } |
In above example, the boolean expression String . IsNullOrEmpty ( LastName returns False value, so the alternative statement ( FirstName + " " + LastName ) executes, which gives output as “Ramesh Kumar” .

Conditional operator(?:) vs Null Coalescing (??) Operator ?
In the case of Null coalescing operator (??) , It checks for the null-ability of first operand and based on that assigns the value. If the first operand value is null then assigns second operand else assigns the first.
Lets have a look at below expression.
= y ?? z ; // Null Coalescing operator |
In the above example, If y is null then z is assigned to x else y . For more details on Null coalescing operator (??) , please check here .
We can rewrite above expression using conditional operator (?:) as follows.
= (y == null)? z : y ; |
Here, if y is null (that means if the expression ( Y == null ) return true) then assign z else assign y to x .
In single line if else statement:
Lets take below if-else statement.
i = 5; x; ( i >= 5) x = 10 * 5; x = 10 * 4; |
In above if-else statement, boolean expression determines variable assignment. This is the right scenario where we can use Conditional operator (?:) as a replacement of if-else statement.
i = 5; x = (i >= 5) ? 10 * 5 : 10 * 4; |
In above example, here in this case i >= 5 is a True condition, hence this statement 10 * 5 executes and 50 is assigned to x . Lets take below example.
i = 3; x = (i >= 5) ? 10 * 5 : 10 * 4; |
In above example, here condition i >= 5 is False , hence 10 * 4 executes and 40 is assigned to x .
Nested conditional operator (?:) in C#
In some scenarios, where there are cascading if-else conditions of variable assignment. We can use chaining conditional operators to replace cascading if-else conditions to a single line, by including a conditional expression as a second statement.
Let’s take below example of cascading/nested if-else statement.
static decimal CalculateBonusAmount(int SalesLimit) decimal BonusAmount = 0; if (SalesLimit >= 1000) { BonusAmount = 20000; } else if (SalesLimit >= 500) { BonusAmount = 10000; } else { BonusAmount = 5000; } return BonusAmount; |
Above cascading if-else condition can be re-written as follows.
static decimal CalculateBonusAmount(int SalesLimit) decimal BonusAmount = 0; //Usage of conditional operator BonusAmount = (SalesLimit >= 1000) ? 2000 : (SalesLimit >= 500) ? 10000 : 5000; return BonusAmount; |
Share this:
One thought on “ conditional operator(:) in c# ”.
Add Comment
VB will use If(Condition, TruePart, FalsePart) if not same types then IIf can be used.
Leave a Reply Cancel reply
This site uses Akismet to reduce spam. Learn how your comment data is processed .
Discover more from
Subscribe now to keep reading and get access to the full archive.
Type your email…
Continue reading
- Essential C#
- Announcements
The parenthesized condition of the if statement is a Boolean expression . In Listing 4.29 , the condition is highlighted.
Boolean expressions appear within many control flow statements. Their key characteristic is that they always evaluate to true or false . For input < 9 to be allowed as a Boolean expression, it must result in a bool . The compiler disallows x = 42 , for example, because this expression assigns x and results in the value that was assigned instead of checking whether the value of the variable is 42 .
C# eliminates a coding error commonly found in C and C++. In C++, Listing 4.30 is allowed.
Although at first glance this code appears to check whether input equals 9 , Chapter 1 showed that = represents the assignment operator, not a check for equality. The return from the assignment operator is the value assigned to the variable—in this case, 9 . However, 9 is an int , so it does not qualify as a Boolean expression and is not allowed by the C# compiler. The C and C++ languages treat integers that are nonzero as true and integers that are zero as false . C#, by contrast, requires that the condition actually be of a Boolean type; integers are not allowed.
Relational and equality operators determine whether a value is greater than, less than, or equal to another value. Table 4.2 lists all the relational and equality operators. All are binary operators.
Operator | Description | Example |
< | Less than | input < 9; |
> | Greater than | input > 9; |
<= | Less than or equal to | input <= 9; |
>= | Greater than or equal to | input >= 9; |
== | Equality operator | input == 9; |
!= | Inequality operator | input != 9; |
The C# syntax for equality uses == , just as many other programming languages do. For example, to determine whether input equals 9 , you use input == 9 . The equality operator uses two equal signs to distinguish it from the assignment operator, = . The exclamation point signifies NOT in C#, so to test for inequality you use the inequality operator, != .
Relational and equality operators always produce a bool value, as shown in Listing 4.31 .
In the full tic-tac-toe program listing, you use the equality operator to determine whether a user has quit. The Boolean expression in Listing 4.32 includes an OR ( || ) logical operator, which the next section discusses in detail.
The logical operators have Boolean operands and produce a Boolean result. Logical operators allow you to combine multiple Boolean expressions to form more complex Boolean expressions. The logical operators are | , || , & , && , and ^ , corresponding to OR, AND, and exclusive OR. The | and & versions of OR and AND are rarely used for Boolean logic, for reasons which we discuss in this section.
In Listing 4.32 , if the user enters quit or presses the Enter key without typing in a value, it is assumed that they want to exit the program. To enable two ways for the user to resign, you can use the logical OR operator, || . The || operator evaluates Boolean expressions and results in a true value if either operand is true (see Listing 4.33 ).
It is not necessary to evaluate both sides of an OR expression, because if one operand is true , the result is known to be true regardless of the value of the other operand. Like all operators in C#, the left operand is evaluated before the right one, so if the left portion of the expression evaluates to true , the right portion is ignored. In the example in Listing 4.33 , if hourOfTheDay has the value 33 , then (hourOfTheDay > 23) evaluates to true and the OR operator ignores the second half of the expression, short-circuiting it. Short-circuiting an expression also occurs with the Boolean AND operator. (Note that the parentheses are not necessary here; the logical operators are of lower precedence than the relational operators. However, it is clearer to the novice reader when the subexpressions are parenthesized for clarity.)
The Boolean AND operator, && , evaluates to true only if both operands evaluate to true . If either operand is false , the result will be false . Listing 4.34 writes a message if the given variable is both greater than 10 and less than 24. 3 Similarly to the OR operator, the AND operator will not always evaluate the right side of the expression. If the left operand is determined to be false , the overall result will be false regardless of the value of the right operand, so the runtime skips evaluating the right operand.
The caret symbol, ^ , is the exclusive OR (XOR) operator. When applied to two Boolean operands, the XOR operator returns true only if exactly one of the operands is true, as shown in Table 4.3 .
Left Operand | Right Operand | Result |
True | True | False |
True | False | True |
False | True | True |
False | False | False |
Unlike the Boolean AND and Boolean OR operators, the Boolean XOR operator does not short-circuit: It always checks both operands, because the result cannot be determined unless the values of both operands are known. Note that the XOR operator is exactly the same as the Boolean inequality operator.
The logical negation operator , or NOT operator , ! , inverts a bool value. This operator is a unary operator, meaning it requires only one operand. Listing 4.35 demonstrates how it works, and Output 4.16 shows the result.
At the beginning of Listing 4.35 , valid is set to false . You then use the negation operator on valid and assign the value to result .
As an alternative to using an if - else statement to select one of two values, you can use the conditional operator . The conditional operator uses both a question mark and a colon; the general format is as follows:
condition ? consequent : alternative
The conditional operator is a ternary operator because it has three operands: condition , consequent , and alternative . (As it is the only ternary operator in C#, it is often called the ternary operator , but it is clearer to refer to it by its name than by the number of operands it takes.) Like the logical operators, the conditional operator uses a form of short-circuiting. If the condition operator evaluates to true , the conditional operator evaluates only consequent . If the conditional evaluates to false , it evaluates only alternative . The result of the operator is the evaluated expression.
Listing 4.36 illustrates the use of the conditional operator. The full listing of this program appears in of the source code.
The program swaps the current player. To do so, it checks whether the current value is 2 . This is the conditional portion of the conditional expression. If the result of the condition is true , the conditional operator results in the consequent value, 1 . Otherwise, it results in the alternative value, 2 . Unlike in an if statement, the result of the conditional operator must be assigned (or passed as a parameter); it cannot appear as an entire statement on its own.
Prior to C# 9.0, the language required that the output (the consequent and alternative) expressions in a conditional operator be consistently typed and that the consistent type be determined without examination of the surrounding context of the expression, including the resulting type assigned. However, C# 9.0 added support for target-typed conditional expressions so that even if the consequent and alternative expressions are of different types (such as string and int ) and neither is convertible to the other, the statement is still allowed if both types will implicitly cast to the targeted type. For example, even if you have object result = condition ? "abc" : 123; , the C# compiler will allow it because both the potential conditional output types will implicitly cast to object .
________________________________________
- p.key == item.key) && !currentPage.some(p => p.level > item.level), }" > p.key == item.key) && !currentPage.some(p => p.level > item.level), }" :href="item.href"> Introduction
- p.key == item.key) && !currentPage.some(p => p.level > item.level), }" > p.key == item.key), }" :href="item.href"> {{item.title}}
Conditional Statements in C#
Master the art of writing flexible code with C#’s powerful conditional statements. Learn how to use if-else, switch, and ternary operators to control the flow of your program and create robust, maintainable software.
Conditional statements are a fundamental part of any programming language, and C# is no exception. These statements allow you to execute different blocks of code based on certain conditions. In this article, we’ll explore the different types of conditional statements available in C#, as well as some best practices for using them effectively.
If Statements
The most basic type of conditional statement in C# is the if statement. Here’s an example:
In this example, condition is a boolean expression that evaluates to either true or false . If condition is true , the code inside the if statement will be executed. If condition is false , the code inside the if statement will be skipped.
Here are some examples of if statements in C#:
Else Statements
In some cases, you may want to execute different code depending on whether a condition is true or false. This is where else statements come in. Here’s an example:
In this example, the code inside the if statement will be executed if condition is true . The code inside the else statement will be executed if condition is false .
Here’s an example of an else statement in C#:
Switch Statements
Switch statements are another type of conditional statement in C#. Here’s an example:
In this example, expression is a variable or expression that evaluates to one of the case values. The code inside each case block will be executed if expression evaluates to that value. The default block will be executed if expression does not match any of the case values.
Here’s an example of a switch statement in C#:
Ternary Operator
The ternary operator is a shorthand way of writing an if statement with a simple condition. Here’s an example:
In this example, condition is a boolean expression that evaluates to either true or false . If condition is true , trueStatement will be executed. If condition is false , falseStatement will be executed.
Here’s an example of the ternary operator in C#:
Best Practices
When using conditional statements in your C# code, it’s important to follow some best practices:
- Use clear and descriptive variable names for your conditions and statements. This will make your code easier to understand and maintain.
- Keep your if statements and else statements as simple as possible. Complex conditions can make your code difficult to read and understand.
- Use the ternary operator sparingly. While it can be a useful shorthand, it can also make your code more difficult to read if used too frequently.
- Use switch statements instead of multiple if statements when you need to match against multiple values. This will make your code more concise and easier to read.
In conclusion, conditional statements are an essential part of any programming language, and C# is no exception. By understanding the different types of conditional statements available in C#, as well as some best practices for using them effectively, you’ll be well on your way to writing clean, readable, and maintainable C# code.
C# Tutorial
C# examples, c# short hand if...else, short hand if...else (ternary operator).
There is also a short-hand if else, which is known as the ternary operator because it consists of three operands. It can be used to replace multiple lines of code with a single line. It is often used to replace simple if else statements:
Instead of writing:
Try it Yourself »
You can simply write:
C# Exercises
Test yourself with exercises.
Print "Hello World" if x is greater than y .
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Conditional Coalescing and Assignment Operators #7897
{{editor}}'s edit
Ai-n3rd feb 1, 2024.
I would like to propose the addition of conditional assignment operators >?= and <?= to improve the expressiveness and conciseness of conditional assignments in C#. Currently, conditional assignments often involve an extra line of code or ternary operators, making the code less readable and more verbose. The proposed operators aim to enhance code readability and reduce verbosity in certain scenarios. Syntax Proposal x = 10; int y = 5; x >?= y; // Expands to x = x > y ? x : y x <?= y; // Expands to x = x > y ? x : y Console.WriteLine(x >? y); // Expands to Console.WriteLine(x > y ? x : y); Console.WriteLine(x <? y); // Expands to Console.WriteLine(x < y ? x : y); Edits: Fixed formatting mistakes |
Beta Was this translation helpful? Give feedback.
Making C# cryptic has never been a goal of the LDM folks. Including a ? might be necessary to avoid collision with other operators, but there's nothing here about null tolerance, which is what ? is often used for - so these would be very confusing symbols.
Reducing character count isn't of itself a sufficient goal either.
Check out -100 points .
Replies: 2 comments · 4 replies
Halofour feb 1, 2024.
static System.Math; x = Max(x, y); x = Min(x, y); |
jnm2 Feb 1, 2024 Collaborator
Or extension methods: = x.Max(y); x = x.Min(y); |
ufcpp Feb 2, 2024
System.Numerics; var x = 5; var y = 3; x.MaxBy(y); Console.WriteLine(x); static class Ex { public static void MaxBy<T>(ref this T x, T y) where T: struct, INumber<T> { x = T.Max(x, y); } } |
Ai-N3rd Feb 5, 2024 Author
These do not account for operator overloading nor anything besides numbers. |
colejohnson66 Feb 5, 2024
Are you saying 's code won't work for non-number types? Why is that needed? What non-number type needs a "min" and "max" comparison? How would your proposal work for things that aren't numbers? |
theunrepentantgeek Feb 5, 2024
Making C# cryptic has never been a goal of the LDM folks. Including a might be necessary to avoid collision with other operators, but there's nothing here about null tolerance, which is what is often used for - so these would be very confusing symbols. Reducing character count isn't a sufficient goal either. Check out . |
- Numbered list
- Unordered list
- Attach files
Select a reply
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Boolean logical operators - AND, OR, NOT, XOR
- 5 contributors
The logical Boolean operators perform logical operations with bool operands. The operators include the unary logical negation ( ! ), binary logical AND ( & ), OR ( | ), and exclusive OR ( ^ ), and the binary conditional logical AND ( && ) and OR ( || ).
- Unary ! (logical negation) operator.
- Binary & (logical AND) , | (logical OR) , and ^ (logical exclusive OR) operators. Those operators always evaluate both operands.
- Binary && (conditional logical AND) and || (conditional logical OR) operators. Those operators evaluate the right-hand operand only if it's necessary.
For operands of the integral numeric types , the & , | , and ^ operators perform bitwise logical operations. For more information, see Bitwise and shift operators .
- Logical negation operator !
The unary prefix ! operator computes logical negation of its operand. That is, it produces true , if the operand evaluates to false , and false , if the operand evaluates to true :
The unary postfix ! operator is the null-forgiving operator .
- Logical AND operator &
The & operator computes the logical AND of its operands. The result of x & y is true if both x and y evaluate to true . Otherwise, the result is false .
The & operator always evaluates both operands. When the left-hand operand evaluates to false , the operation result is false regardless of the value of the right-hand operand. However, even then, the right-hand operand is evaluated.
In the following example, the right-hand operand of the & operator is a method call, which is performed regardless of the value of the left-hand operand:
The conditional logical AND operator && also computes the logical AND of its operands, but doesn't evaluate the right-hand operand if the left-hand operand evaluates to false .
For operands of the integral numeric types , the & operator computes the bitwise logical AND of its operands. The unary & operator is the address-of operator .
- Logical exclusive OR operator ^
The ^ operator computes the logical exclusive OR, also known as the logical XOR, of its operands. The result of x ^ y is true if x evaluates to true and y evaluates to false , or x evaluates to false and y evaluates to true . Otherwise, the result is false . That is, for the bool operands, the ^ operator computes the same result as the inequality operator != .
For operands of the integral numeric types , the ^ operator computes the bitwise logical exclusive OR of its operands.
- Logical OR operator |
The | operator computes the logical OR of its operands. The result of x | y is true if either x or y evaluates to true . Otherwise, the result is false .
The | operator always evaluates both operands. When the left-hand operand evaluates to true , the operation result is true regardless of the value of the right-hand operand. However, even then, the right-hand operand is evaluated.
In the following example, the right-hand operand of the | operator is a method call, which is performed regardless of the value of the left-hand operand:
The conditional logical OR operator || also computes the logical OR of its operands, but doesn't evaluate the right-hand operand if the left-hand operand evaluates to true .
For operands of the integral numeric types , the | operator computes the bitwise logical OR of its operands.
- Conditional logical AND operator &&
The conditional logical AND operator && , also known as the "short-circuiting" logical AND operator, computes the logical AND of its operands. The result of x && y is true if both x and y evaluate to true . Otherwise, the result is false . If x evaluates to false , y isn't evaluated.
In the following example, the right-hand operand of the && operator is a method call, which isn't performed if the left-hand operand evaluates to false :
The logical AND operator & also computes the logical AND of its operands, but always evaluates both operands.
- Conditional logical OR operator ||
The conditional logical OR operator || , also known as the "short-circuiting" logical OR operator, computes the logical OR of its operands. The result of x || y is true if either x or y evaluates to true . Otherwise, the result is false . If x evaluates to true , y isn't evaluated.
In the following example, the right-hand operand of the || operator is a method call, which isn't performed if the left-hand operand evaluates to true :
The logical OR operator | also computes the logical OR of its operands, but always evaluates both operands.
Nullable Boolean logical operators
For bool? operands, the & (logical AND) and | (logical OR) operators support the three-valued logic as follows:
The & operator produces true only if both its operands evaluate to true . If either x or y evaluates to false , x & y produces false (even if another operand evaluates to null ). Otherwise, the result of x & y is null .
The | operator produces false only if both its operands evaluate to false . If either x or y evaluates to true , x | y produces true (even if another operand evaluates to null ). Otherwise, the result of x | y is null .
The following table presents that semantics:
x | y | x&y | x|y |
---|---|---|---|
true | true | true | true |
true | false | false | true |
true | null | null | true |
false | true | false | true |
false | false | false | false |
false | null | false | null |
null | true | null | true |
null | false | false | null |
null | null | null | null |
The behavior of those operators differs from the typical operator behavior with nullable value types. Typically, an operator that is defined for operands of a value type can be also used with operands of the corresponding nullable value type. Such an operator produces null if any of its operands evaluates to null . However, the & and | operators can produce non-null even if one of the operands evaluates to null . For more information about the operator behavior with nullable value types, see the Lifted operators section of the Nullable value types article.
You can also use the ! and ^ operators with bool? operands, as the following example shows:
The conditional logical operators && and || don't support bool? operands.
- Compound assignment
For a binary operator op , a compound assignment expression of the form
is equivalent to
except that x is only evaluated once.
The & , | , and ^ operators support compound assignment, as the following example shows:
The conditional logical operators && and || don't support compound assignment.
Operator precedence
The following list orders logical operators starting from the highest precedence to the lowest:
Use parentheses, () , to change the order of evaluation imposed by operator precedence:
For the complete list of C# operators ordered by precedence level, see the Operator precedence section of the C# operators article.
Operator overloadability
A user-defined type can overload the ! , & , | , and ^ operators. When a binary operator is overloaded, the corresponding compound assignment operator is also implicitly overloaded. A user-defined type can't explicitly overload a compound assignment operator.
A user-defined type can't overload the conditional logical operators && and || . However, if a user-defined type overloads the true and false operators and the & or | operator in a certain way, the && or || operation, respectively, can be evaluated for the operands of that type. For more information, see the User-defined conditional logical operators section of the C# language specification .
C# language specification
For more information, see the following sections of the C# language specification :
- Logical negation operator
- Logical operators
- Conditional logical operators
- C# operators and expressions
- Bitwise and shift operators
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
- Stack Overflow Public questions & answers
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Talent Build your employer brand
- Advertising Reach developers & technologists worldwide
- Labs The future of collective knowledge sharing
- About the company
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Using the null-conditional operator on the left-hand side of an assignment
I have a few pages, each with a property named Data . On another page I'm setting this data like this:
Is there any possibility to use the null-conditional operator on MyPage ? I'm thinking of something like this:
But when I write it like this, I get the following error:
The left-hand side of an assignment must be a variable, property or indexer.
I know it's because MyPage could be null and the left-hand side wouldn't be a variable anymore.
It's not that I cannot use it like I have it already but I just want to know if there's any possibility to use the null-conditional operator on this.
- null-conditional-operator

- 16 You should be able to create a SetData method and do MyPage1?.SetData(this.data); – Joachim Isaksson Commented Mar 9, 2016 at 9:06
- 4 Possible duplicate of Why C# 6.0 doesn't let to set properties of a non-null nullable struct when using Null propagation operator? – Dovydas Šopa Commented Mar 9, 2016 at 9:08
- 1 Null propagation/conditional operator is for accessing properties, not setting them. Hence you can't use it. – Tal Avissar Commented Mar 9, 2016 at 9:14
- 8 I personally think this is a fault in the current implementation. A property on the left side is shorthand for a call to the property setter method so you should be able to use ? on a null property just as if you had explicitly called the set method itself. – Bob Provencher Commented Apr 19, 2017 at 15:48
- 1 Here is the C# issue: github.com/dotnet/csharplang/issues/2883 – user764754 Commented Apr 17, 2022 at 10:02
7 Answers 7
The null propagation operator returns a value. And since you must have a variable on the left hand side of an assignment, and not a value, you cannot use it in this way.
Sure, you could make things shorter by using the tenary operator, but that, on the other hand, doesn't really help the readability aspect.
Joachim Isaksson's comment on your question shows a different approach that should work.

- Except you don’t have “a variable” on the left-hand side of a property assignment either, do you? A property assignment x.Y = z is basically just a syntax sugar for a method call like x.SetY(z) ; if C# allowed using an explicit setter call, I could use x?.SetY(z) , but this is not allowed. – Mormegil Commented Mar 1 at 18:51
As Joachim Isaksson suggested in the comments, I now have a method SetData(Data data) and use it like this:
I came up with the following extension,
Which may be called globally; this only works on public properties.
The following improved version works on anything,
And may also be called globally,
But the extension name is somewhat misleading as the Action does not exclusively require an assignment.
Rather late to the party, but I came to this article with a similar issue. I took the idea of the SetValue method and created a generic extension method, as below:
Example usage, for attaching and detaching event an handler:
Hope somebody finds it useful :)
Try this Add all your pages to myPageList.
You can Use Extension Method
A generic SetValue extension method (but only for ref properties) would be:
And will be used like
- 4 Did you test this? There's absolutely no way this will work... T property would have to be a ref parameter for the change to stick because the only thing you are doing is setting the local copy of the property inside the function, and you can't have this ref extension methods nor does C# allow ref access to properties (unlike VB, which is a real shame actually). – Mike Marynowski Commented Sep 29, 2017 at 15:35
- Oh, you are very right 👍 - it works only with ref parameters (so in my case it worked always ...by accident) – oo_dev Commented Oct 5, 2017 at 10:45
- 1 @MikeMarynowski: Actually you now can have ref this extension methods (but the restriction preventing passing a property to a ref parameter still exists) – Ben Voigt Commented Jul 12, 2021 at 21:55
- @BenVoigt Not for unconstrained T though. You can only have this ref for value types. – Mike Marynowski Commented Jul 14, 2021 at 11:00
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c# null-conditional-operator or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
- The return of Staging Ground to Stack Overflow
- Policy: Generative AI (e.g., ChatGPT) is banned
Hot Network Questions
- A TCP server which uses one thread to read while writing data with another thread
- My 5-year-old is stealing food and lying
- What is the meaning of "Wa’al"?
- What does "the dogs of prescriptivism" mean?
- A Boy Named Sue
- Why does a battery have a limit for current in amperes?
- Do wererats take falling damage?
- Tiny book about a planet full of man-eating sunflowers
- NaN is not equal to NaN
- Do I need to staple cable for new wire run through a preexisting wall?
- Paris Taxi with children seats (from and to airport)
- What was the submarine in the film "Ice Station Zebra"?
- What is the safest way to camp in a zombie apocalypse?
- Can apophatic theology offer a coherent resolution to the "problem of the creator of God"?
- Sink vs Basin distinction
- Does "my grades suffered" mean "my grades became worse" or "my grades were bad"?
- If a reference is no longer publicly available, should you include the proofs of the results you cite from it?
- Where did the pronunciation of the word "kilometer/kilometre" as "kl OM iter" rather than "KILL o meeter" originate?
- Old science fiction short story about a lawyer attempting to patent a new kind of incredibly strong cloth
- Securing HTTP File Transfer over local network
- How to fix patchy garden and turn in into lush garden
- Implement Huffman code in C17
- How can I enable read only mode in microSD card
- Visiting every digit

IMAGES
VIDEO
COMMENTS
See also. The conditional operator ?:, also known as the ternary conditional operator, evaluates a Boolean expression and returns the result of one of the two expressions, depending on whether the Boolean expression evaluates to true or false, as the following example shows: C#. Copy. Run.
The ternary operator (?:) is not designed for control flow, it's only designed for conditional assignment. If you need to control the flow of your program, use a control structure, such as if/else. Share. ... Operator (C# Reference) The conditional operator (?:) returns one of two values depending on the value of a Boolean expression.
In expressions with the null-conditional operators ?. and ?[], you can use the ?? operator to provide an alternative expression to evaluate in case the result of the expression with null-conditional operations is null:
The null-conditional operators are short-circuiting. That is, if one operation in a chain of conditional member or element access operations returns null, the rest of the chain doesn't execute. In the following example, B isn't evaluated if A evaluates to null and C isn't evaluated if A or B evaluates to null: C#. Copy.
The problem (with the syntax) is not with the assignment, as the assignment operator in C# is a valid expression. Rather, it is with the desired declaration as declarations are statements. ... Casting to different types with the conditional operator. 11. Inline assignment on IF statement. 0. Avoiding twice coding, One in if Condition and One in ...
ApplCount():0); Here we use the Console.WriteLine()method to print the number of appliances we need for an order. We base that count on the isKitchenBoolean variable. When that one is true, the conditional operator executes ApplCount()and returns that method's value. Should that variable be false, the operator returns 0.
Example: Ternary operator. int x = 10, y = 100; var result = x > y ? "x is greater than y" : "x is less than y"; Console.WriteLine(result); output: x is less than y. Thus, a ternary operator is short form of if else statement. The above example can be re-write using if else condition, as shown below. Example: Ternary operator replaces if statement.
Nested conditional operator (?:) in C#. In some scenarios, where there are cascading if-else conditions of variable assignment. We can use chaining conditional operators to replace cascading if-else conditions to a single line, by including a conditional expression as a second statement. Let's take below example of cascading/nested if-else ...
Although at first glance this code appears to check whether input equals 9, Chapter 1 showed that = represents the assignment operator, not a check for equality. The return from the assignment operator is the value assigned to the variable—in this case, 9.However, 9 is an int, so it does not qualify as a Boolean expression and is not allowed by the C# compiler.
1) Set variable with if statement. 2) Update variable with if/else statement. 3) Set variable with conditional operator. Two quick ways to set variable conditionally. Replace if/else with default value. Replace if/else with conditional operator. Summary. Most variables have a different value at various stages in our program's execution. A ...
Just like C# and Java, the expression will only be evaluated if, and only if, the expression is the matching one for the condition given; the other expression will not be evaluated. ... Appropriate use of the conditional operator in a variable assignment context reduces the probability of a bug from a faulty assignment as the assigned variable ...
The most basic type of conditional statement in C# is the if statement. Here's an example: // code to execute if condition is true. In this example, condition is a boolean expression that evaluates to either true or false. If condition is true, the code inside the if statement will be executed.
This has the conditional operator always either execute its second or third expression. The logic of the conditional operator is: "if this is true, do the first; otherwise, do the second." This has the operator behave like an if/else statement. That is, the conditional operator first evaluates a true/false condition.
In this article. The assignment operator = assigns the value of its right-hand operand to a variable, a property, or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or ...
Learn C# Tutorial ... Arithmetic Assignment Comparison Logical. C# Math C# Strings. Strings Concatenation Interpolation Access Strings Special Characters. ... There is also a short-hand if else, which is known as the ternary operator because it consists of three operands. It can be used to replace multiple lines of code with a single line.
In this article. C# provides a number of operators. Many of them are supported by the built-in types and allow you to perform basic operations with values of those types. Those operators include the following groups: Arithmetic operators that perform arithmetic operations with numeric operands; Comparison operators that compare numeric operands; Boolean logical operators that perform logical ...
The alternatives include using traditional conditional statements or ternary operators, but these may result in less readable and more verbose code. The addition of conditional assignment operators would offer a concise and expressive way to handle conditional assignments, especially when dealing with minimum and maximum value assignments.
There is no specific operator in C# that does exactly this. Share. Follow answered Mar 26, 2014 at 18:33. Servy ... Using the null-conditional operator on the left-hand side of an assignment. 0. c# Null-conditional Operator. 2. Null coalescing operator with the variable itself. 4.
For more information, see the User-defined conditional logical operators section of the C# language specification. C# language specification. For more information, see the following sections of the C# language specification: Logical negation operator; Logical operators; Conditional logical operators; Compound assignment; See also. C# operators ...
21. The null propagation operator returns a value. And since you must have a variable on the left hand side of an assignment, and not a value, you cannot use it in this way. Sure, you could make things shorter by using the tenary operator, but that, on the other hand, doesn't really help the readability aspect.