5.3. Structures
- Declaration and definition
- Stack And Heap
- new And delete
- Dot And Arrow
Programmers often use the term "structure" to name two related concepts. They form structure specifications with the struct keyword and create structure objects with a variable definition or the new operator. We typically call both specifications and objects structures, relying on context to clarify the the specific usage.
Structures are an aggregate data type whose contained data items are called fields or members . Once specified, the structure name becomes a new data type or type specifier in the program. After the program creates or instantiates a structure object, it accesses the fields with one of the selection operators . Referring to the basket analogy suggested previously, a structure object is a basket, and the fields or members are the items in the basket. Although structures are more complex than the fundamental types, programmers use the same pattern when defining variables of either type.

Structure Specifications: Fields And Members
A program must specify or declare a structure before using it. The specification determines the number, the type, the name, and the order of the data items contained in the structure.
Structure Definitions And Objects
We can use a blueprint as another analogy for a structure. Blueprints show essential details like the number and size of bedrooms, the location of the kitchen, etc. Similarly, structure specifications show the structure fields' number, type, name, and order. Nevertheless, a blueprint isn't a house; you can't live in it. However, given the blueprint, we can make as many identical houses as we wish, and while each one looks like the others, it has a different, distinct set of occupants and a distinct street address. Similarly, each object created from a structure specification looks like the others, but each can store distinct values and has a distinct memory address.
- C++ code that defines three new student structure variables or objects named s1 , s2 , and s3 . The program allocates the memory for the three objects on the stack.
- The pointer variables s4 , s5 , and s6 are defined and allocated automatically on the stack. However, the structure objects they point to are allocated dynamically on the heap.
- An abstract representation of three student objects on the stack. Each object has three fields.
- An abstract representation of three student pointer variables pointing to three student objects allocated dynamically on the heap with the new operator.
Initializing Structures And Fields
Figure 3 demonstrates that we are not required to initialize structure objects when we create them - perhaps the program will read the data from the console or a data file. However, we can initialize structures when convenient. C++ inherited an aggregate field initialization syntax from the C programming language, and the 2011 ANSI standard extends that syntax, making it conform to other major programming languages. More recently, the ANSI 2020 standard added a variety of default field initializations. The following figures illustrate a few of these options.
- The field order within the structure and the data order within the initialization list are significant and must match. The program saves the first list value in the first structure field until it saves the last list value in the last structure field. If there are fewer initializers (the elements inside the braces) than there are fields, the excess fields are initialized to their zero-equivalents . Having more initializers than fields is a compile-time error.
- Similar to (a), but newer standards no longer require the = operator.
- Historically, en block structure initialization was only allowed in the structure definition, but the ANSI 2015 standard removed that restriction, allowing en bloc assignment to previously defined variables.
- Abstract representations of the structure objects or variables created and initialized in (a), (b), and (c). Each object has three fields. Although every student must have these fields, the values stored in them may be different.
- Another way to conceptualize structures and variables is as the rows and columns of a simple database table. Each column represents one field, and each row represents one structure object. The database schema fixes the number of columns, but the program can add any number of rows to the table.
- Initializing a structure object when defining it. The = operator is now optional.
- Designated initializers are more flexible than the non-designated initializers illustrated in Figure 4, which are matched to structure fields by position (first initializer to the first field, second initializer to the second field, etc.). Designated initializers may skip a field - the program does not initialize name in this example.
- Programmers may save data to previously defined structure objects, possibly skipping some fields, with designators.
The next step is accessing the individual fields within a structure object.
Field Selection: The Dot And Arrow Operators
A basket is potentially beneficial, but so far, we have only seen how to put items into it, and then only en masse . To achieve its full potential, we need some way to put items into the basket one at a time, and there must be some way to retrieve them. C++ provides two selection operators that join a specific structure object with a named field. The combination of an object and a field form a variable whose type matches the field type in the structure specification.
The Dot Operator
The C++ dot operator looks and behaves just like Java's dot operator and performs the same tasks in both languages.
- The general dot operator syntax. The left-hand operand is a structure object, and the right-hand operator is the name of one of its fields.
- Examples illustrating the dot operator based on the student examples in Figure 3(a).
- The dot operator's left operand names a specific basket or object, and the right-hand operand names the field. If we view the structure as a table, the left-hand operand selects the row, and the right-hand operand selects the column. So, we can visualize s2 . name as the intersection of a row and column.
The Arrow Operator
Java only has one way of creating objects and only needs one operator to access its fields. C++ can create objects in two ways (see Figure 3 above) and needs an additional field access operator, the arrow: -> .
- The general arrow operator syntax. The left-hand operand is a pointer to a structure object, and the right-hand operator is the name of one of the object's fields.
- Examples illustrate the arrow operator based on the student examples in Figure 3(b).
Choosing the correct selection operator

- Choose the arrow operator if the left-hand operand is a pointer.
- Otherwise, choose the dot operator.
Moving Structures Within A Program
A basket is a convenient way to carry and move many items by holding its handle. Similarly, a structure object is convenient for a program to "hold" and move many data items with a single (variable) name. Specifically, a program can assign one structure to another (as long as they are instances of the same structure specification), pass them as arguments to functions, and return them from functions as the function's return value.
Assignment is a fundamental operation regardless of the kind of data involved. The C++ code to assign one structure to another is simple and should look familiar:
- C++ code defining a new structure object and copying an existing object to it by assignment.
- An abstract representation of a new, empty structure.
- An abstract representation of how a program copies the contents of an existing structure to another structure.
Function Argument
Once the program creates a structure object, passing it to a function looks and behaves like passing a fundamental-type variable. If we view the function argument as a new, empty structure object, we see that argument passing copies one structure object to another, behaving like an assignment operation.
- The first part of the C++ code fragment defines a function (similar to a Java method). The argument is a student structure. The last statement (highlighted) passes a previously created and initialized structure as a function argument.
- In this abstract representation, a program copies the data stored in s2 to the function argument temp . The braces forming the body of the print function create a new scope distinct from the calling scope where the program defines s2 . The italicized variable names label and distinguish the illustrated objects.
Function Return Value
Just as a program can pass a structure into a function as an argument, so it can return a structure as the function's return value, as demonstrated by the following example:
- C++ code defining a function named read that returns a structure. The program defines and initializes the structure object, temp , in local or function scope with a series of console read operations and returns it as the function return value. The assignment operator saves the returned structure in s3 .
- A graphical representation of the return process. Returning an object with the return operator copies the local object, temp , to the calling scope, where the program saves it in s1 .
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Keywords & Identifier
- Variables & Constants
- C Data Types
- C Input/Output
- C Operators
- C Introduction Examples
C Flow Control
- C if...else
- C while Loop
- C break and continue
- C switch...case
- C Programming goto
- Control Flow Examples
C Functions
- C Programming Functions
- C User-defined Functions
- C Function Types
- C Recursion
- C Storage Class
- C Function Examples
- C Programming Arrays
- C Multi-dimensional Arrays
- C Arrays & Function
- C Programming Pointers
- C Pointers & Arrays
- C Pointers And Functions
- C Memory Allocation
- Array & Pointer Examples
C Programming Strings
- C Programming String
- C String Functions
- C String Examples
Structure And Union
- C Structure
- C Struct & Pointers
- C Struct & Function
- C struct Examples
C Programming Files
- C Files Input/Output
- C Files Examples
Additional Topics
- C Enumeration
- C Preprocessors
- C Standard Library
- C Programming Examples
C structs and Pointers
C Structure and Function
C Struct Examples
- Add Two Complex Numbers by Passing Structure to a Function
- Add Two Distances (in inch-feet system) using Structures
In C programming, a struct (or structure) is a collection of variables (can be of different types) under a single name.
- Define Structures
Before you can create structure variables, you need to define its data type. To define a struct, the struct keyword is used.
Syntax of struct
For example,
Here, a derived type struct Person is defined. Now, you can create variables of this type.
Create struct Variables
When a struct type is declared, no storage or memory is allocated. To allocate memory of a given structure type and work with it, we need to create variables.
Here's how we create structure variables:
Another way of creating a struct variable is:
In both cases,
- person1 and person2 are struct Person variables
- p[] is a struct Person array of size 20.
Access Members of a Structure
There are two types of operators used for accessing members of a structure.
- . - Member operator
- -> - Structure pointer operator (will be discussed in the next tutorial)
Suppose, you want to access the salary of person2 . Here's how you can do it.
Example 1: C structs
In this program, we have created a struct named Person . We have also created a variable of Person named person1 .
In main() , we have assigned values to the variables defined in Person for the person1 object.
Notice that we have used strcpy() function to assign the value to person1.name .
This is because name is a char array ( C-string ) and we cannot use the assignment operator = with it after we have declared the string.
Finally, we printed the data of person1 .
- Keyword typedef
We use the typedef keyword to create an alias name for data types. It is commonly used with structures to simplify the syntax of declaring variables.
For example, let us look at the following code:
We can use typedef to write an equivalent code with a simplified syntax:
Example 2: C typedef
Here, we have used typedef with the Person structure to create an alias person .
Now, we can simply declare a Person variable using the person alias:
- Nested Structures
You can create structures within a structure in C programming. For example,
Suppose, you want to set imag of num2 variable to 11 . Here's how you can do it:
Example 3: C Nested Structures
Why structs in c.
Suppose you want to store information about a person: his/her name, citizenship number, and salary. You can create different variables name , citNo and salary to store this information.
What if you need to store information of more than one person? Now, you need to create different variables for each information per person: name1 , citNo1 , salary1 , name2 , citNo2 , salary2 , etc.
A better approach would be to have a collection of all related information under a single name Person structure and use it for every person.
More on struct
- Structures and pointers
- Passing structures to a function
Table of Contents
- C struct (Introduction)
- Create struct variables
- Access members of a structure
- Example 1: C++ structs
Sorry about that.
Related Tutorials
cppreference.com
Assignment operators.
Assignment operators modify the value of the object.
[ edit ] Definitions
Copy assignment replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is performed in a special member function, described in copy assignment operator .
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
Compound assignment replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
[ edit ] Assignment operator syntax
The assignment expressions have the form
- ↑ target-expr must have higher precedence than an assignment expression.
- ↑ new-value cannot be a comma expression, because its precedence is lower.
[ edit ] Built-in simple assignment operator
For the built-in simple assignment, the object referred to by target-expr is modified by replacing its value with the result of new-value . target-expr must be a modifiable lvalue.
The result of a built-in simple assignment is an lvalue of the type of target-expr , referring to target-expr . If target-expr is a bit-field , the result is also a bit-field.
[ edit ] Assignment from an expression
If new-value is an expression, it is implicitly converted to the cv-unqualified type of target-expr . When target-expr is a bit-field that cannot represent the value of the expression, the resulting value of the bit-field is implementation-defined.
If target-expr and new-value identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same).
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
[ edit ] Built-in compound assignment operator
The behavior of every built-in compound-assignment expression target-expr op = new-value is exactly the same as the behavior of the expression target-expr = target-expr op new-value , except that target-expr is evaluated only once.
The requirements on target-expr and new-value of built-in simple assignment operators also apply. Furthermore:
- For + = and - = , the type of target-expr must be an arithmetic type or a pointer to a (possibly cv-qualified) completely-defined object type .
- For all other compound assignment operators, the type of target-expr must be an arithmetic type.
In overload resolution against user-defined operators , for every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2 , where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
[ edit ] Example
Possible output:
[ edit ] Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
Operator precedence
Operator overloading
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 25 January 2024, at 22:41.
- This page has been accessed 410,142 times.
- Privacy policy
- About cppreference.com
- Disclaimers

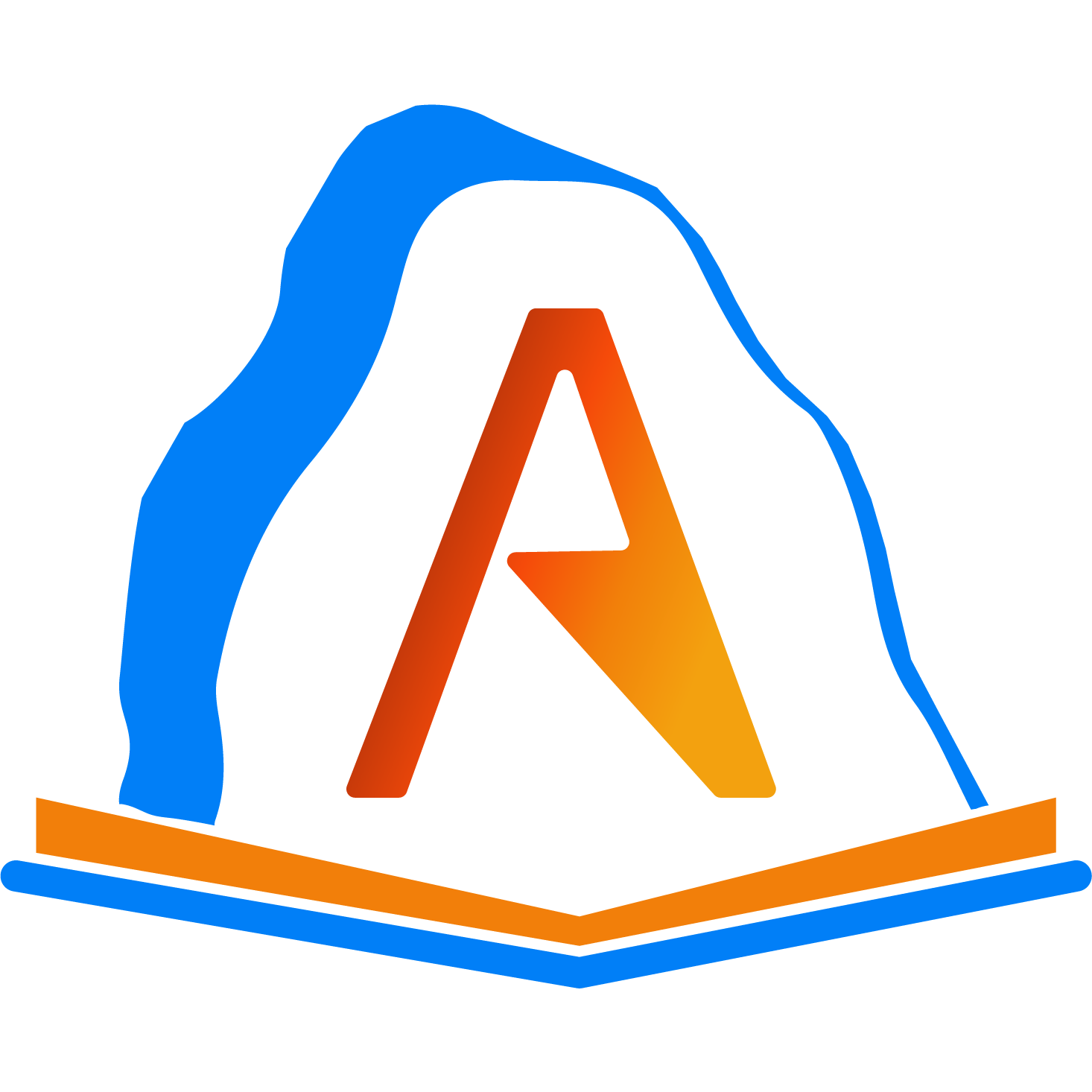
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- Mixed Up Code Practice
- Coding Practice
- Activecode Exercises
- 8.1 Compound values
- 8.2 Point objects
- 8.3 Accessing instance variables
- 8.4 Operations on structures
- 8.5 Structures as parameters
- 8.6 Call by value
- 8.7 Call by reference
- 8.8 Rectangles
- 8.9 Structures as return types
- 8.10 Passing other types by reference
- 8.11 Getting user input
- 8.12 Glossary
- 8.13 Multiple Choice Exercises
- 8.14 Mixed-Up Code Exercises
- 8.15 Coding Practice
- 8.3. Accessing instance variables" data-toggle="tooltip">
- 8.5. Structures as parameters' data-toggle="tooltip" >

8.4. Operations on structures ¶
Most of the operators we have been using on other types, like mathematical operators ( + , % , etc.) and comparison operators ( == , > , etc.), do not work on structures. Actually, it is possible to define the meaning of these operators for the new type, but we won’t do that in this book.
On the other hand, the assignment operator does work for structures. It can be used in two ways: to initialize the instance variables of a structure or to copy the instance variables from one structure to another. An initialization looks like this:
The values in squiggly braces get assigned to the instance variables of the structure one by one, in order. So in this case, x gets the first value and y gets the second.
This syntax can also be used in an assignment statement. So the following is legal.
Before C++11 (2011), a cast was required for assignment. This form of assignment was written like the following. Both forms are supported in modern compilers.
It is legal to assign one structure to another. For example:
The output of this program is 3, 4 .
Construct a block of code that correctly initializes the instance variables of a structure.
Q-3: Which operators do NOT work on structures. Select all that apply.
- The modulo operator does not work on structures.
- The assignment operator does work on structures.
- The greater than operator does not work on structures.
- The equality operator does not work on structures.
- The addition operator does not work on structures.
Next: Unions , Previous: Overlaying Structures , Up: Structures [ Contents ][ Index ]
15.13 Structure Assignment
Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example:
Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
See Assignment Expressions .
When a structure type has a field which is an array, as here,
structure assigment such as r1 = r2 copies array fields’ contents just as it copies all the other fields.
This is the only way in C that you can operate on the whole contents of a array with one operation: when the array is contained in a struct . You can’t copy the contents of the data field as an array, because
would convert the array objects (as always) to pointers to the zeroth elements of the arrays (of type struct record * ), and the assignment would be invalid because the left operand is not an lvalue.
Home » Learn C Programming from Scratch » C Assignment Operators
C Assignment Operators
Summary : in this tutorial, you’ll learn about the C assignment operators and how to use them effectively.
Introduction to the C assignment operators
An assignment operator assigns the vale of the right-hand operand to the left-hand operand. The following example uses the assignment operator (=) to assign 1 to the counter variable:
After the assignmment, the counter variable holds the number 1.
The following example adds 1 to the counter and assign the result to the counter:
The = assignment operator is called a simple assignment operator. It assigns the value of the left operand to the right operand.
Besides the simple assignment operator, C supports compound assignment operators. A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.
The following example uses a compound-assignment operator (+=):
The expression:
is equivalent to the following expression:
The following table illustrates the compound-assignment operators in C:
- A simple assignment operator assigns the value of the left operand to the right operand.
- A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.
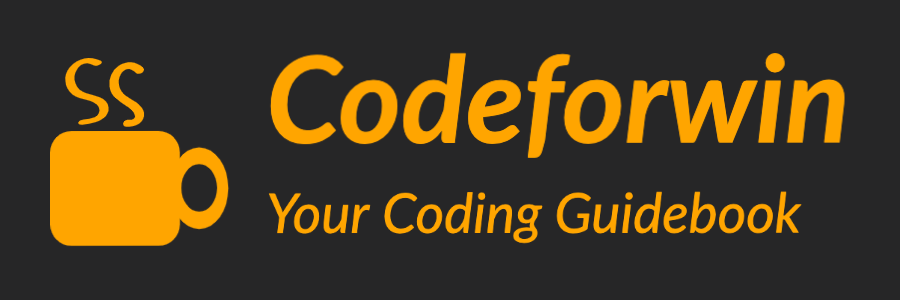
Assignment and shorthand assignment operator in C
Quick links.
- Shorthand assignment
Assignment operator is used to assign value to a variable (memory location). There is a single assignment operator = in C. It evaluates expression on right side of = symbol and assigns evaluated value to left side the variable.
For example consider the below assignment table.
The RHS of assignment operator must be a constant, expression or variable. Whereas LHS must be a variable (valid memory location).
Shorthand assignment operator
C supports a short variant of assignment operator called compound assignment or shorthand assignment. Shorthand assignment operator combines one of the arithmetic or bitwise operators with assignment operator.
For example, consider following C statements.
The above expression a = a + 2 is equivalent to a += 2 .
Similarly, there are many shorthand assignment operators. Below is a list of shorthand assignment operators in C.
C++ Tutorial
C++ functions, c++ classes, c++ examples, c++ assignment operators, assignment operators.
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

- Design Pattern
- Interview Q
C Control Statements
C functions, c dynamic memory, c structure union, c file handling, c preprocessor, c command line, c programming test, c interview.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

Archived Content
Perplexing puzzles.
Solo MP This MP, as well as all other MPs in CS 225, are to be completed without a partner.
You are welcome to get help on the MP from course staff, via open lab hours, or Piazza!
Goals and Overview
In this MP, you will:
- Work with a graph that is to large too store completely in memory
- Use a graph algorithm to solve a complex problem
- Implement an algorithm from public sources documents
- See the difference in performance between a guided and unguided search algorithm
Checking Out the Code
All assignments will be distributed via our release repo on github this semester. You will need to have set up your git directory to have our release as a remote repo as described in our git set up
You can merge the assignments as they are released into your personal repo with
if you are using multiple machines you may need to use the following to allow them to work correcly.
The first git command will fetch and merge changes from the main branch on your remote repository named release into your personal. The --no-edit flag automatically generates a commit message for you, and the --no-rebase flag will merge the upstream branch into the current branch. Generally, these two flags shouldn’t be used, but are included for ease of merging assignments into your repo.
The second command will push to origin (your personal), which will allow it to track the new changes from release .
You will need to run these commands for every assignment that is released.
All the files for this mp are in the mp_puzzle directory.
Preparing Your Code
This semester for MPs we are using CMake rather than just make. This allows for us to use libraries such as Catch2 that can be installed in your system rather than providing them with each assignment. This change does mean that for each assignment you need to use CMake to build your own custom makefiles. To do this you need to run the following in the base directory of the assignment. Which in this assignment is the mp_puzzle directory.
This first makes a new directory in your assignment directory called build . This is where you will actually build the assignment and then moves to that directory. This is not included in the provided code since we are following industry standard practices and you would normally exclude the build directory from any source control system.
Now you need to actually run CMake as follows.
This runs CMake to initialize the current directory which is the build directory you just made as the location to build the assignment. The one argument to CMake here is .. which referes to the parent of the current directory which in this case is top of the assignment. This directory has the files CMake needs to setup your assignment to be build.
At this point you can in the build directory run make as described to build the various programs for the MP.
You will need to do the above once for each assignment. You will need to run make every time you change source code and want to compile it again.
Assignment Description
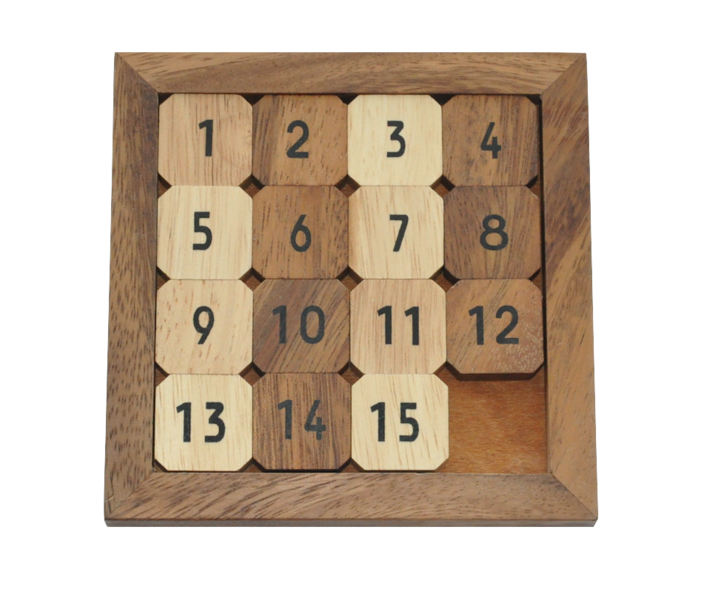
In this mp we will be developing a puzzle solver for 15 puzzle as a graph problem. If you have not heard of 15 puzzle before you may want to look at the wikipedia article on it here . We will then generate an animation of the solution.
Part 1: The PuzzleState data structure
In the first part of this assignment we will work on representing the puzzle as a graph. To do this we will have a node for every possible position of the puzzle and an edge between two nodes if there is a single move of a tile that will move from one position to the next. The tricky part in this is that there are 16 factorial possible positions for the puzzle. Since this is way too large to store in memory we will need to only use the portions of the graph that we need to get from the starting position until we can find a solution.
To do this we will build a class PuzzleState that stores a single vertex of the graph but can also create its neighbors when asked. This is not hard to do since the possible positions you can move to are easy to compute only knowing the current position. This will give you a system where you only need to create the nodes of the graph when you explore them. You will need to implement all the methods in the PuzzleState class.
Creating and Outputing
While the internal implementation of the PuzzleState class is entirely up to you we are defining two functions to make sure that we agree on what state we are referring to. These functions are the Constructor that takes an array of positions and creates a PuzzleState with those positions and asArray which returns the array version of that state. The format of this is to list the values in the puzzle starting for the upper left hand corner and moving to the right until the end of the line then moving to the next line until all 16 positions are provided.
Implementing operator<
To ensure that you can use std::map to store PuzzleStates we require you to implement an operator<() . While this operator does not represent any real relation between the different puzzle states it will satisfy the requirement for a total order so that you can use std::map .
Manhattan Distance
One function that might be unclear is the manhattanDistance function. This is asking you to compute a distance value between two states. This distance is the distance that each tile has to travel in the x dimension and the y dimension to reach the location of the tile in the other state.
Testing Your Code
Provided Catch test cases are available as well by running:
Extra Credit Submission
For extra credit, you can submit the code you have implemented and tested for part one of mp_puzzle. You must submit your work before the extra credit deadline as listed at the top of this page. See Handing in Your Code for instructions.
Part 2: The Solve Functions
In part 2 we are writing two different functions that will each solve the puzzle by finding the shortest path from the start state to the goal state. If the goal is not stated, the standard solved state will be used. The first version will solve this by implementing breadth first search. The second will use the A* algorithm. The doxygen of these functions can be seen here solveBFS and solveAstar .
A* search Algorithm
The A* algorithm is an algorithm for finding the shortest path from one point in a graph to another point in the graph. Documentation on the general algorithm can be found on wikipedia here . You should use the material provided there as the basis for your implementation. The key idea here is that A* uses a heuristic function to estimate how much further a state is from the goal state. In our case we will be using the manhattan distance function we wrote in part 1. This works since each move in the puzzle moves a single piece in a single direction so the minimum distance from a state to the goal state is enough moves to move each piece directly there.
Handing in your code
You must submit your work on PL for grading. We will use the following files for grading:
All other files will not be used for grading.
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- Increment (++) and Decrement (--) Operator Overloading in C++
- C++ Ternary or Conditional Operator
- C++ Logical Operators
- C++ Relational Operators
- Types of Operator Overloading in C++
- How to Overload the Function Call Operator () in C++?
- Increment Operator Behavior When Passed as Function Parameters in C++
- How to Use the Not-Equal (!=) Operator in C++?
- Operators in C++
- Constants in C++
- C++ Comparison Operators
- Casting Operators in C++
- C++ sizeof Operator
- Typecast Operator Overloading in C++
- C++ Program For Iterative Quick Sort
- How to Parse an Array of Objects in C++ Using RapidJson?
- C++ Increment and Decrement Operators
- C++ Arithmetic Operators
- How to Lock Window Resize C++ sfml?
C++ Assignment Operator Overloading
Prerequisite: Operator Overloading
The assignment operator,”=”, is the operator used for Assignment. It copies the right value into the left value. Assignment Operators are predefined to operate only on built-in Data types.
- Assignment operator overloading is binary operator overloading.
- Overloading assignment operator in C++ copies all values of one object to another object.
- Only a non-static member function should be used to overload the assignment operator.
We can’t directly use the Assignment Operator on objects. The simple explanation for this is that the Assignment Operator is predefined to operate only on built-in Data types. As the class and objects are user-defined data types, so the compiler generates an error.
here, a and b are of type integer, which is a built-in data type. Assignment Operator can be used directly on built-in data types.
c1 and c2 are variables of type “class C”. Here compiler will generate an error as we are trying to use an Assignment Operator on user-defined data types.
The above example can be done by implementing methods or functions inside the class, but we choose operator overloading instead. The reason for this is, operator overloading gives the functionality to use the operator directly which makes code easy to understand, and even code size decreases because of it. Also, operator overloading does not affect the normal working of the operator but provides extra functionality to it.
Now, if the user wants to use the assignment operator “=” to assign the value of the class variable to another class variable then the user has to redefine the meaning of the assignment operator “=”. Redefining the meaning of operators really does not change their original meaning, instead, they have been given additional meaning along with their existing ones.
Please Login to comment...
Similar reads.
- cpp-operator
- cpp-operator-overloading
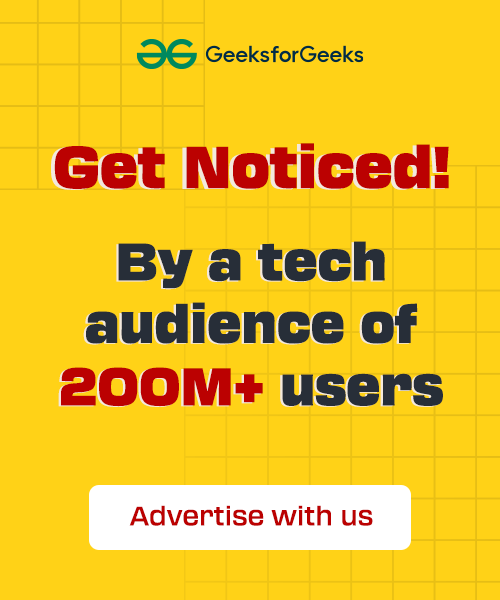
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
Move Assignment Operator. Calls the base class move assignment operator passing the src object. Then calls the move assignment operator on each member using the src object as the value to be copied. If you define a class like this: struct some_struct: public some_base { std::string str1; int a; float b; char* c; std::string str2; };
As you can see that a assignment is simply replaced by a "mov" instruction in assembly, the assignment operator simply means moving data from one memory location to another memory location. The assignment will only do it for immediate members of a structures and will fail to copy when you have Complex datatypes in a structure.
Different types of assignment operators are shown below: 1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current ...
The assignment operator works with structures. Used with structure operands, the assignment operator copies a block of bits from one memory address (the address of the right-hand object) to another (the address of the left-hand object). C++ code defining a new structure object and copying an existing object to it by assignment.
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
Access Members of a Structure. There are two types of operators used for accessing members of a structure.. - Member operator-> - Structure pointer operator (will be discussed in the next tutorial) ... and we cannot use the assignment operator = with it after we have declared the string. Finally, we printed the data of person1.
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
On the other hand, the assignment operator does work for structures. It can be used in two ways: to initialize the instance variables of a structure or to copy the instance variables from one structure to another. An initialization looks like this: Point blank = { 3.0, 4.0 }; The values in squiggly braces get assigned to the instance variables ...
15.13 Structure Assignment. Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example: Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
The = assignment operator is called a simple assignment operator. It assigns the value of the left operand to the right operand. Besides the simple assignment operator, C supports compound assignment operators. A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.
Structures and the assignment operator. With regular primitive types we have a wide variety of operations available, including assignment, comparisons, arithmetic, etc. ... Clearly, direct assignment between entire structures is easier, if a full copy of the whole thing is the desired result!
C Programming & Data Structures: Assignment Operators in CTopics discussed:1. Introduction to Assignment Operators in C language.2. Types of Shorthand Assign...
C Structures. The structure in C is a user-defined data type that can be used to group items of possibly different types into a single type. The struct keyword is used to define the structure in the C programming language. The items in the structure are called its member and they can be of any valid data type.
C supports a short variant of assignment operator called compound assignment or shorthand assignment. Shorthand assignment operator combines one of the arithmetic or bitwise operators with assignment operator. For example, consider following C statements. The above expression a = a + 2 is equivalent to a += 2.
C++ Structures C++ References. Create References Memory Address. C++ Pointers. Create Pointers Dereferencing Modify Pointers. C++ Functions ... Assignment operators are used to assign values to variables. In the example below, we use the assignment operator (=) to assign the value 10 to a variable called x:
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
The assignment operator is used to assign the value, variable and function to another variable. Let's discuss the various types of the assignment operators such as =, +=, -=, /=, *= and %=. Example of the Assignment Operators: A = 5; // use Assignment symbol to assign 5 to the operand A. B = A; // Assign operand A to the B.
Assignment Operators; Relational Operators; Logical Operators; Ternary Operators; Flow Control in Java. Flow Control; ... the only operation that can be applied to struct variables is assignment. Any other operation (e.g. equality check) is not allowed on struct ... // whole structures { printf("p1 and p2 are same "); } getchar(); return 0 ; } ...
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.
Part 1: The PuzzleState data structure. In the first part of this assignment we will work on representing the puzzle as a graph. To do this we will have a node for every possible position of the puzzle and an edge between two nodes if there is a single move of a tile that will move from one position to the next. ... Implementing operator< To ...
The assignment operator,"=", is the operator used for Assignment. It copies the right value into the left value. Assignment Operators are predefined to operate only on built-in Data types. Assignment operator overloading is binary operator overloading. Overloading assignment operator in C++ copies all values of one object to another object.