
- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointer Arithmetics
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary

Assignment Operators in C
In C, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable or an expression. The value to be assigned forms the right hand operand, whereas the variable to be assigned should be the operand to the left of = symbol, which is defined as a simple assignment operator in C. In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple assignment operator (=)
The = operator is the most frequently used operator in C. As per ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed. You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented assignment operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression a+=b has the same effect of performing a+b first and then assigning the result back to the variable a.
Similarly, the expression a<<=b has the same effect of performing a<<b first and then assigning the result back to the variable a.
Here is a C program that demonstrates the use of assignment operators in C:
When you compile and execute the above program, it produces the following result −
cppreference.com
Assignment operators.
Assignment operators modify the value of the object.
[ edit ] Definitions
Copy assignment replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is performed in a special member function, described in copy assignment operator .
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
Compound assignment replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
[ edit ] Assignment operator syntax
The assignment expressions have the form
- ↑ target-expr must have higher precedence than an assignment expression.
- ↑ new-value cannot be a comma expression, because its precedence is lower.
[ edit ] Built-in simple assignment operator
For the built-in simple assignment, the object referred to by target-expr is modified by replacing its value with the result of new-value . target-expr must be a modifiable lvalue.
The result of a built-in simple assignment is an lvalue of the type of target-expr , referring to target-expr . If target-expr is a bit-field , the result is also a bit-field.
[ edit ] Assignment from an expression
If new-value is an expression, it is implicitly converted to the cv-unqualified type of target-expr . When target-expr is a bit-field that cannot represent the value of the expression, the resulting value of the bit-field is implementation-defined.
If target-expr and new-value identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same).
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
[ edit ] Built-in compound assignment operator
The behavior of every built-in compound-assignment expression target-expr op = new-value is exactly the same as the behavior of the expression target-expr = target-expr op new-value , except that target-expr is evaluated only once.
The requirements on target-expr and new-value of built-in simple assignment operators also apply. Furthermore:
- For + = and - = , the type of target-expr must be an arithmetic type or a pointer to a (possibly cv-qualified) completely-defined object type .
- For all other compound assignment operators, the type of target-expr must be an arithmetic type.
In overload resolution against user-defined operators , for every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2 , where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
[ edit ] Example
Possible output:
[ edit ] Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
Operator precedence
Operator overloading
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 25 January 2024, at 22:41.
- This page has been accessed 410,142 times.
- Privacy policy
- About cppreference.com
- Disclaimers

This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Assignment operators
- 8 contributors
expression assignment-operator expression
assignment-operator : one of = *= /= %= += -= <<= >>= &= ^= |=
Assignment operators store a value in the object specified by the left operand. There are two kinds of assignment operations:
simple assignment , in which the value of the second operand is stored in the object specified by the first operand.
compound assignment , in which an arithmetic, shift, or bitwise operation is performed before storing the result.
All assignment operators in the following table except the = operator are compound assignment operators.
Assignment operators table
Operator keywords.
Three of the compound assignment operators have keyword equivalents. They are:
C++ specifies these operator keywords as alternative spellings for the compound assignment operators. In C, the alternative spellings are provided as macros in the <iso646.h> header. In C++, the alternative spellings are keywords; use of <iso646.h> or the C++ equivalent <ciso646> is deprecated. In Microsoft C++, the /permissive- or /Za compiler option is required to enable the alternative spelling.
Simple assignment
The simple assignment operator ( = ) causes the value of the second operand to be stored in the object specified by the first operand. If both objects are of arithmetic types, the right operand is converted to the type of the left, before storing the value.
Objects of const and volatile types can be assigned to l-values of types that are only volatile , or that aren't const or volatile .
Assignment to objects of class type ( struct , union , and class types) is performed by a function named operator= . The default behavior of this operator function is to perform a member-wise copy assignment of the object's non-static data members and direct base classes; however, this behavior can be modified using overloaded operators. For more information, see Operator overloading . Class types can also have copy assignment and move assignment operators. For more information, see Copy constructors and copy assignment operators and Move constructors and move assignment operators .
An object of any unambiguously derived class from a given base class can be assigned to an object of the base class. The reverse isn't true because there's an implicit conversion from derived class to base class, but not from base class to derived class. For example:
Assignments to reference types behave as if the assignment were being made to the object to which the reference points.
For class-type objects, assignment is different from initialization. To illustrate how different assignment and initialization can be, consider the code
The preceding code shows an initializer; it calls the constructor for UserType2 that takes an argument of type UserType1 . Given the code
the assignment statement
can have one of the following effects:
Call the function operator= for UserType2 , provided operator= is provided with a UserType1 argument.
Call the explicit conversion function UserType1::operator UserType2 , if such a function exists.
Call a constructor UserType2::UserType2 , provided such a constructor exists, that takes a UserType1 argument and copies the result.
Compound assignment
The compound assignment operators are shown in the Assignment operators table . These operators have the form e1 op = e2 , where e1 is a non- const modifiable l-value and e2 is:
an arithmetic type
a pointer, if op is + or -
a type for which there exists a matching operator *op*= overload for the type of e1
The built-in e1 op = e2 form behaves as e1 = e1 op e2 , but e1 is evaluated only once.
Compound assignment to an enumerated type generates an error message. If the left operand is of a pointer type, the right operand must be of a pointer type, or it must be a constant expression that evaluates to 0. When the left operand is of an integral type, the right operand must not be of a pointer type.
Result of built-in assignment operators
The built-in assignment operators return the value of the object specified by the left operand after the assignment (and the arithmetic/logical operation in the case of compound assignment operators). The resultant type is the type of the left operand. The result of an assignment expression is always an l-value. These operators have right-to-left associativity. The left operand must be a modifiable l-value.
In ANSI C, the result of an assignment expression isn't an l-value. That means the legal C++ expression (a += b) += c isn't allowed in C.
Expressions with binary operators C++ built-in operators, precedence, and associativity C assignment operators
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources

Want to create or adapt books like this? Learn more about how Pressbooks supports open publishing practices.
Kenneth Leroy Busbee
An assignment statement sets and/or re-sets the value stored in the storage location(s) denoted by a variable name; in other words, it copies a value into the variable. [1]
The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within most programming languages the symbol used for assignment is the equal symbol. But bite your tongue, when you see the = symbol you need to start thinking: assignment. The assignment operator has two operands. The one to the left of the operator is usually an identifier name for a variable. The one to the right of the operator is a value.
Simple Assignment
The value 21 is moved to the memory location for the variable named: age. Another way to say it: age is assigned the value 21.
Assignment with an Expression
The item to the right of the assignment operator is an expression. The expression will be evaluated and the answer is 14. The value 14 would be assigned to the variable named: total_cousins.
Assignment with Identifier Names in the Expression
The expression to the right of the assignment operator contains some identifier names. The program would fetch the values stored in those variables; add them together and get a value of 44; then assign the 44 to the total_students variable.
- cnx.org: Programming Fundamentals – A Modular Structured Approach using C++
- Wikipedia: Assignment (computer science) ↵
Programming Fundamentals Copyright © 2018 by Kenneth Leroy Busbee is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License , except where otherwise noted.
Share This Book
Assignment operators
Assignment operators modify the value of the object.
Explanation
copy assignment operator replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is a special member function, described in copy assignment operator .
move assignment operator replaces the contents of the object a with the contents of b , avoiding copying if possible ( b may be modified). For class types, this is a special member function, described in move assignment operator . (since C++11)
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
compound assignment operators replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
Builtin direct assignment
The direct assignment expressions have the form
For the built-in operator, lhs may have any non-const scalar type and rhs must be implicitly convertible to the type of lhs .
The direct assignment operator expects a modifiable lvalue as its left operand and an rvalue expression or a braced-init-list (since C++11) as its right operand, and returns an lvalue identifying the left operand after modification.
For non-class types, the right operand is first implicitly converted to the cv-unqualified type of the left operand, and then its value is copied into the object identified by left operand.
When the left operand has reference type, the assignment operator modifies the referred-to object.
If the left and the right operands identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same)
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2, where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
Builtin compound assignment
The compound assignment expressions have the form
The behavior of every builtin compound-assignment expression E1 op = E2 (where E1 is a modifiable lvalue expression and E2 is an rvalue expression or a braced-init-list (since C++11) ) is exactly the same as the behavior of the expression E1 = E1 op E2 , except that the expression E1 is evaluated only once and that it behaves as a single operation with respect to indeterminately-sequenced function calls (e.g. in f ( a + = b, g ( ) ) , the += is either not started at all or is completed as seen from inside g ( ) ).
In overload resolution against user-defined operators , for every pair A1 and A2, where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2, where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
Operator precedence
Operator overloading

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
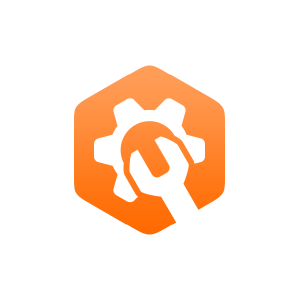
5.4: Arithmetic Assignment Operators
- Last updated
- Save as PDF
- Page ID 10264
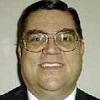
- Kenneth Leroy Busbee
- Houston Community College via OpenStax CNX
Overview of Arithmetic Assignment
The five arithmetic assignment operators are a form of short hand. Various textbooks call them "compound assignment operators" or "combined assignment operators". Their usage can be explaned in terms of the assignment operator and the arithmetic operators. In the table we will use the variable age and you can assume that it is of integer data type.
Demonstration Program in C++
Creating a folder of sub-folder for source code files.
Depending on your compiler/IDE, you should decide where to download and store source code files for processing. Prudence dictates that you create these folders as needed prior to downloading source code files. A suggested sub-folder for the Bloodshed Dev-C++ 5 compiler/IDE might be named:
- Demo_Programs
If you have not done so, please create the folder(s) and/or sub-folder(s) as appropriate.
Download the Demo Program
Download and store the following file(s) to your storage device in the appropriate folder(s). Following the methods of your compiler/IDE, compile and run the program(s). Study the soruce code file(s) in conjunction with other learning materials.
Download from Connexions: Demo_Arithmetic_Assignment.cpp
- Assignment Operators
Just like Arithmetic Operators, C++ has Assignment Operators. In order to give value to the variable in the program, an operator is needed. This function is provided by the Assignment Operators. Assignment Operators in programming languages are pre-defined operators which are used to assign values to the variables. Let us look at these Assignment operators in more detail.

- Assignment Operators are used to assigning the results of an expression or constant to a variable.
- In order to assign values to a variable, we use the Assignment Operator in the programming language.
Assignment Operator is a type of Binary Operator wherein it needs two operands to function. The left side operand is the variable and the right side of the operand is the value to be assigned.
The symbol which is used by the Assignment Operator is ‘ = ’.
Copy Code Copied Use a different Browser
The assignment operator can be used within any valid expression. An expression can be a combination of variables, constants, and operators arranged as per the syntax and precedence levels of the programming language.
During the use of the assignment operator, always the right-hand side of the operator is computed first, and then it is assigned to the variable defined on the left-hand side of the statement.
Note: The source (right-hand side) and destination (left-hand side) should always be of the same data type.
Browse all the Topics Under Operator and Expressions: Operators
- Arithmetic Operators
- C++ Shorthands
- Unary Operator
- Increment and Decrement Operators
- Relation Operator
- Logical Operators
Types of Assignment Operators
In C++, there are 2 categories of Assignment Operators
- Simple Assignment Operator
- Compound Assignment Operator
Simple Assignment Operator (=) :
This is the usual assignment operator. Simple-assignment operator assigns its right operand to its left operand. In easy terms, this operator is used to assign the value on the right to the variable on the left.
Compound Assignment Operator:
These type of Operators are also known as Short-hand assignment operators.
Its syntax is,
where OP is a binary operator and is known as short-hand assignment operator.
While first initializing the variable, we cannot use the Short-hand operators. This will give a declaration error at the compile time of the program. Let’s understand this by looking at an example –
Compile-time Error. Value of variable ‘a’ not defined.
In the above program, we can see that in the initialization statement, we did not assign the value of 10 to the variable ‘a’ but instead we used the shorthand assignment operator which means
But the value of ‘a’ has not been defined. The compiler does not know the value stored in the location of variable ‘a’. Hence a compile-time error occurs.
FAQs on Assignment Operators
Q1. Equality Operator is denoted by?
- None of the above
Answer. Option C
Q2. Assignment Operator is an example of ______ operator.
- Conditional
Answer. Option B
Q3. Which of the following is an invalid assignment operator?
- None of the Above
Customize your course in 30 seconds
Which class are you in.


Operator and Expressions: Operators
- Shorthand Operators
- Unary Operators
- Increment & Decrement Operators
- Relational Operators
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Specifications
Browser compatibility.
An assignment operator assigns a value to its left operand based on the value of its right operand.
The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, please clone https://github.com/mdn/interactive-examples and send us a pull request.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator is used to assign a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Using promises
- Iterators and generators
- Meta programming
- JavaScript modules
- Client-side web APIs
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.ListFormat
- Intl.Locale
- Intl.NumberFormat
- Intl.PluralRules
- Intl.RelativeTimeFormat
- ReferenceError
- SharedArrayBuffer
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical operators
- Object initializer
- Operator precedence
- (currently at stage 1) pipes the value of an expression into a function. This allows the creation of chained function calls in a readable manner. The result is syntactic sugar in which a function call with a single argument can be written like this:">Pipeline operator
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for await...of
- for each...in
- function declaration
- import.meta
- try...catch
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- The arguments object
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: 'x' is not iterable
- TypeError: More arguments needed
- TypeError: Reduce of empty array with no initial value
- TypeError: can't access dead object
- TypeError: can't access property "x" of "y"
- TypeError: can't assign to property "x" on "y": not an object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cannot use 'in' operator to search for 'x' in 'y'
- TypeError: cyclic object value
- TypeError: invalid 'instanceof' operand 'x'
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- X.prototype.y called on incompatible type
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
Learn the best of web development
Get the latest and greatest from MDN delivered straight to your inbox.
Thanks! Please check your inbox to confirm your subscription.
If you haven’t previously confirmed a subscription to a Mozilla-related newsletter you may have to do so. Please check your inbox or your spam filter for an email from us.

An operator is symbol that takes one or more values (or expressions, in programming jargon) and yields another value (so that the construction itself becomes an expression) [2] .
There are many different types of operators, but the ones we primarily concern ourselves with are boldfaced below. If you'd like to learn more about different types of operators in PHP, please click here .
- Operator Precedence
- Arithmetic Operators
- Assignment Operators
- Bitwise Operators
- Comparison Operators
- Error Control Operators
- Execution Operators
- Incrementing/Decrementing Operators
- Logical Operators
- String Operators
- Array Operators
- Type Operators
- 1 Assignment operators
- 2 Arithmetic operators
- 3 Comparison operators
- 5 The way the IB wants you to use operators
- 6 Python operators cheatsheet
- 7 Standards
- 8 References
Assignment operators [ edit ]
An assignment operator assigns a value to its left operand based on the value of its right operand. [3]
Arithmetic operators [ edit ]
Arithmetic operators take numerical values (either literals or variables) as their operands and return a single numerical value. The standard arithmetic operators are addition (+), subtraction (-), multiplication (*), and division (/). [4]
An example of some arithmetic operators in PHP can be found below [5] .
An example of some arithmetic operators in Python can be found below [6] .
Comparison operators [ edit ]
Comparison operators, as their name implies, allow you to compare two values. [7]
An example of some conditional operators in PHP can be found below [8] .
An example of some conditional operators in Python can be found below [9] .
A video [ edit ]
This video references the C programming language and scratch, but the ideas about operators are excellent. In the case of conditionals, PHP and C share similar syntax (but not exact).
The way the IB wants you to use operators [ edit ]
Please know all code submitted to the IB (with the exception of object oriented programming option) is in pseudocode . The way we write operators in pseudocode is different than the way we might write them in the real world.
Click here to view a file describing approved notation, including pseudocode This is the approved notation sheet from the IB.
Below is a graphic that is taken from the above PDF file to help you understand how the IB wants operators to look.
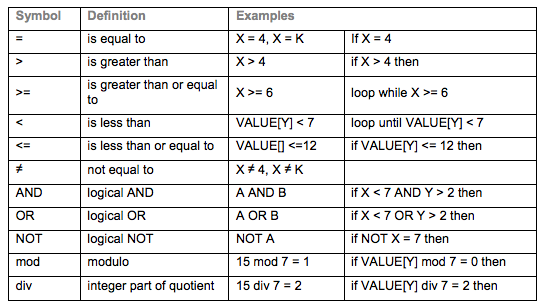
Python operators cheatsheet [ edit ]
Click here for an excellent python cheatsheet
Standards [ edit ]
- Define the terms: variable, constant, operator, object.
- Define common operators.
- Analyse the use of variables, constants and operators in algorithms.
References [ edit ]
- ↑ http://www.flaticon.com/
- ↑ http://php.net/manual/en/language.operators.php
- ↑ https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Assignment_Operators
- ↑ https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Arithmetic_Operators
- ↑ http://php.net/manual/en/language.operators.arithmetic.php
- ↑ https://www.geeksforgeeks.org/basic-operators-python/
- ↑ https://www.php.net/manual/en/language.operators.comparison.php
- ↑ http://php.net/manual/en/language.operators.comparison.php
A natural number, a negative of a natural number, or zero.
anomalous or exceptional conditions requiring special processing – often changing the normal flow of program execution
Give the precise meaning of a word, phrase, concept or physical quantity.
Break down in order to bring out the essential elements or structure. To identify parts and relationships, and to interpret information to reach conclusions.
- Computational thinking
- Programming

- A named space for holding data/information
- The name is often referred to as the identifier
- A representation of a datum
- A symbol indicating that an operation is to be performed (on one or more operands)
- A combination of variables, operators, and literals that represent a single value
- The smallest syntactically valid construct that conveys a complete command
- In Python, statements are terminated with the end-of-line character
- In Java, statements are terminated with a ; character
- In Python, white space is signficant
- In Java, extra white space is insignificant (e.g., the statement ends with the ; , wherever it is, even on another line)
- In Python, everything after a # is an in-line comment
- In Java, everything after a // is an in-line comments and everything between a /* and a */ is a block comment
- Placing a value into the memory identified by a variable/constant
- The assignment operator is a binary operator; the left-side operand is a variable/constant and the right-side operand is a literal or an expression (i.e., something that evaluates to a value)
- The assignment operation evaluates to the value of the right-side operand (so, is an expression)
- The value of the right-side operand is assigned to the left-side operand
- The address of the right-side operand is assigned to the left-side operand
- initial = 'H'
- course = "CS159"
- initial = 'H';
- ok = false;
- course = "CS159";
- A variable can only contain one value at a time
- A variable can only contain a value of the same type
- int i; i = 5; // i contains the value 5 i = 27; // i now contains the value 27 String s; s = "CS159"; // s contains the address of "CS159" s = "JMU"; // s now contains the address of "JMU"
- Confusing the assignment operator with the notion of "equality"
- Attempting to use expressions like 21 = age (which has an inappropriate left-side operand)
- int i, j; i = j = 5;
- It can cause confusion in more complicated statements, especially those involving arithmetic operators and relational operators
- In the previous example, which assignment is performed first?
- Associativity - determines whether (in the absence of other determinants) an expression is evaluated from left to right or right to left
- The assignment operator has right to left associativity, so i = j = 5 is equivalent to i = (j = 5)
- Ensuring that the type of the right-side operand is the same as the type of the left-side operand
- Java does not allow implicit losses of precision
- Java does allow widenings (though you should refrain from using them)
- double weight; int age; age = 21.0; // Not allowed (will generate a compile-time error) weight = 155; // Allowed but not recommended (use 155. instead)
- ( type ) expression
- Examples: double real; int small; long large; // ... small = (int)large; // ... small = (int)real;
- Loss of precision
- Possible loss of magnitude
- Possible change of sign
- Indicate that a value can only be assigned to a variable once
- final type variable [, variable ]... ;
- final boolean CORRECT;
- final double BUDGET;
- final int CAPACITY;
- Same as for other variables
- Must be all uppercase
- Underscores between "words" improve readability
- An error will be generated if an identifier that is declared to be final is the left-side operand of more than one assignment operator
- Include the assignment in the declaration
- New Sandbox Program
Click on one of our programs below to get started coding in the sandbox!
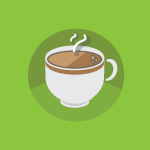
Standards Framework
For ap computer science principles 2020.
Standards in this Framework
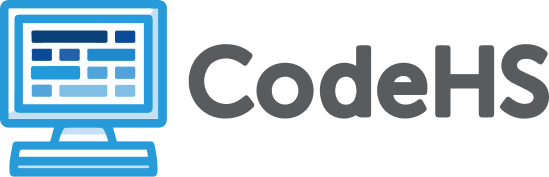
- Computer Science Curriculum
- Certifications
- Professional Development
- Assignments
- Classroom Management
- Integrations
- Course Catalog
- Project Catalog
- K-12 Pathways
- State Courses
- Spanish Courses
- Hour of Code
- Digital Textbooks
- Online PD Courses
- In-Person PD Workshops
- Virtual PD Workshops
- Free PD Workshops
- Teacher Certification Prep
- Microcredentials
- PD Membership
Programming Languages
- Case Studies
- Testimonials
- Read Write Code Blog
- Read Write Code Book
- Knowledge Base
- Student Projects
- Career Center
- Privacy Center
- Privacy Policy
- Accessibility
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Logical Operators in Python with Examples
- How To Do Math in Python 3 with Operators?
- Python 3 - Logical Operators
- Understanding Boolean Logic in Python 3
- Concatenate two strings using Operator Overloading in Python
- Relational Operators in Python
- Difference between "__eq__" VS "is" VS "==" in Python
- Modulo operator (%) in Python
- Python Bitwise Operators
- Python - Star or Asterisk operator ( * )
- New '=' Operator in Python3.8 f-string
- Format a Number Width in Python
- Difference between != and is not operator in Python
- Operator Overloading in Python
- Python Object Comparison : "is" vs "=="
- Python | a += b is not always a = a + b
- Python Arithmetic Operators
- Python Operators
- Python | Operator.countOf
Assignment Operators in Python
Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, bitwise computations. The value the operator operates on is known as Operand .
Here, we will cover Assignment Operators in Python. So, Assignment Operators are used to assigning values to variables.
Now Let’s see each Assignment Operator one by one.
1) Assign: This operator is used to assign the value of the right side of the expression to the left side operand.
2) Add and Assign: This operator is used to add the right side operand with the left side operand and then assigning the result to the left operand.
Syntax:
3) Subtract and Assign: This operator is used to subtract the right operand from the left operand and then assigning the result to the left operand.
Example –
4) Multiply and Assign: This operator is used to multiply the right operand with the left operand and then assigning the result to the left operand.
5) Divide and Assign: This operator is used to divide the left operand with the right operand and then assigning the result to the left operand.
6) Modulus and Assign: This operator is used to take the modulus using the left and the right operands and then assigning the result to the left operand.
7) Divide (floor) and Assign: This operator is used to divide the left operand with the right operand and then assigning the result(floor) to the left operand.
8) Exponent and Assign: This operator is used to calculate the exponent(raise power) value using operands and then assigning the result to the left operand.
9) Bitwise AND and Assign: This operator is used to perform Bitwise AND on both operands and then assigning the result to the left operand.
10) Bitwise OR and Assign: This operator is used to perform Bitwise OR on the operands and then assigning result to the left operand.
11) Bitwise XOR and Assign: This operator is used to perform Bitwise XOR on the operands and then assigning result to the left operand.
12) Bitwise Right Shift and Assign: This operator is used to perform Bitwise right shift on the operands and then assigning result to the left operand.
13) Bitwise Left Shift and Assign: This operator is used to perform Bitwise left shift on the operands and then assigning result to the left operand.
Please Login to comment...
Similar reads.
- Python-Operators
- How to Use ChatGPT with Bing for Free?
- 7 Best Movavi Video Editor Alternatives in 2024
- How to Edit Comment on Instagram
- 10 Best AI Grammar Checkers and Rewording Tools
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Assignment Operator: An assignment operator is the operator used to assign a new value to a variable, property, event or indexer element in C# programming language. Assignment operators can also be used for logical operations such as bitwise logical operations or operations on integral operands and Boolean operands. Unlike in C++, assignment ...
Different types of assignment operators are shown below: 1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: 2. "+=": This operator is combination of '+' and '=' operators.
Assignment (computer science) In computer programming, an assignment statement sets and/or re-sets the value stored in the storage location (s) denoted by a variable name; in other words, it copies a value into the variable. In most imperative programming languages, the assignment statement (or expression) is a fundamental construct.
The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol.
Assignment Operators in C - In C, the assignment operator stores a certain value in an already declared variable. ... Computer Science Subjects Python Technologies Software Testing ... f = 5; // definition and initializing d and f. char x = 'x'; // the variable x has the value 'x'. Once a variable of a certain type is declared, it cannot be ...
Assignment operators modify the value of the object. replaces the contents of the object is not modified). For class types, this is performed in a special member function, described in. ↑ target-expr must have higher precedence than an assignment expression.
The Operation: Placing a value into the memory identified by a variable/constant; The Operator (in Java): = Operands: The assignment operator is a binary operator; the left-side operand is a variable/constant and the right-side operand is a literal or an expression (i.e., something that evaluates to a value)
Definition of Assignment Operator. An assignment operator is a fundamental programming concept used to assign a value to a variable. In most programming languages, it is represented by the equal sign (=). ... The assignment operator (=) is an essential component in computer programming languages and is frequently used in various real-world ...
The built-in assignment operators return the value of the object specified by the left operand after the assignment (and the arithmetic/logical operation in the case of compound assignment operators). The resultant type is the type of the left operand. The result of an assignment expression is always an l-value.
The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within most programming languages the symbol used for assignment is the equal symbol.
Assignment operators. Assignment operators modify the value of the object. All built-in assignment operators return *this, and most user-defined overloads also return *this so that the user-defined operators can be used in the same manner as the built-ins. However, in a user-defined operator overload, any type can be used as return type ...
This page titled 5.4: Arithmetic Assignment Operators is shared under a CC BY license and was authored, remixed, and/or curated by Kenneth Leroy Busbee ( OpenStax CNX) . The five arithmetic assignment operators are a form of short hand. Various textbooks call them "compound assignment operators" or "combined assignment operators". Their usage ...
In the context of programming and computer science, an assignment operator is a symbol or operator used to assign a value to a variable. It is a fundamental concept in most programming languages. It is used to store a value in a variable so that it can be manipulated and used in computations later in the code.
Learn what assignment operators are and how to use them in C++ programming language. Find out the difference between simple and compound assignment operators, and see examples of syntax, usage and FAQs on assignment operators.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x. The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples. Name. Shorthand operator.
Assignment: An assignment is a statement in computer programming that is used to set a value to a variable name. The operator used to do assignment is denoted with an equal sign (=). This operand works by assigning the value on the right-hand side of the operand to the operand on the left-hand side. It is possible for the same variable to hold ...
The language definition simply states: An assignment operator stores a value in the object designated by the left operand. (6.5.16, para 3). The only general constraint is that the left operand be a modifiable lvalue. An lvalue can correspond to a register (which has no address) or an addressable memory location.
An assignment operator is a symbol that allows you to modify the value of a variable or an expression. Learn about different types of assignment operators, such as incrementing, decrementing, copy, and list, and how they are used in various programming languages, such as C, C++, Perl, and NASL.
An assignment operator assigns a value to its left operand based on the value of its right operand. Arithmetic operators . Arithmetic operators take numerical values (either literals or variables) as their operands and return a single numerical value. The standard arithmetic operators are addition (+), subtraction (-), multiplication (*), and ...
The Assignment Operator. An Introduction with Examples in Java. Prof. David Bernstein. James Madison University. Computer Science Department. [email protected].
The assignment operator allows a program to change the value represented by a variable. AAP-1.B.2: The exam reference sheet provides the "←" operator to use for assignment. For example, a ← expression which evaluates expression and then assigns the result to the variable a. AAP-1.B.3
Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, bitwise computations. The value the operator operates on is known as Operand. Here, we will cover Assignment Operators in Python. So, Assignment Operators are used to assigning values to variables.