- Web Application Development Enterprise applications maximize your efficiency
- Flutter App Development Building the next-gen mobile app that rocks
- Software Customization & Enhancement Make your existing software better
- Real Estate
- Construction
- Marketplace
- Data Management
- Procurement
- Recruitment
- Microservices
- Schedule a Call
Diving deep into the ocean of technology

How to build and deploy a three-layer architecture application with C#
- February 27, 2021 • Development
What’s three-layer architecture?
Layer indicates the logical separation of components. Layered architecture concentrates on grouping related functionality within an application into distinct layers that are stacked vertically on top of each other. Each layer has unique namespaces and classes.
An N-layer application may reside on the same physical computer (same tier); each layer's components communicate with another layer’s components by well-defined interfaces.
In C#, each layer is often implemented in a Dynamic Link Libraries (DLL) file.
To help you understand more about the n-layer architecture, I’ve put together all pieces of information about three-layer with a practical example in this article as follows:
- Presentation layer It is the first and topmost layer present in the application where users can interact with the application.
- Business Logic layer This is the middle layer - the heart of the application. It contains all business logic of the application, describes how business objects interact with each other, where the Presentation layer and Data Access layer can indirectly communicate with each other.
- Data Access layer The Data Access layer enforces rules regarding accessing data, providing simplified access to data stored in persistent storage, such as SQL Server. It is noteworthy that this layer only focuses on data access instead of data storage. It may create a bit of confusion with the Data-tier in a three-tier architecture.
Advantages of three-layer architecture
- Explicit code: The code is separated into each layer. Each one is dedicated to a single responsibility such as interface, business processing, and querying instead of bundling all the code in one place.
- Easy to maintain: As its roles separate each layer, it would be easier to change something. The modification can be isolated in a single layer or affected only the nearest layer without affecting the whole program.
- Easy to develop, reuse: When we want to modify a function, we can easily do that as we already have a standard architecture. In case we want to alter a complete layer such as from Winform to Web form, we only need to implement to replace the Presentation layer; other layers can be reused completely.
- Easy to transfer: We could save time on moving the application to others as they have a standard architecture to follow and apply.
- Easy to distribute the workloads: By organizing the code into different layers based on its responsibility, each team/member can write their code on each layer independently, which in turn helps developers control their workload.
How does it work?
In three-layer architecture, the Presentation layer doesn’t communicate directly with the Data Access layer. The Business Logic layer works as a bridge between the Presentation layer and the Data Access layer. The three-layer architecture works as follows:
- The Presentation layer is the only class that is directly interacted with the user. It is mainly used for presenting/collecting data from users, then passes them to the Business Logic layer for further processing.
- After receiving information from the Presentation layer, the Business Logic layer does some business logic on the data. During this process, this layer may retrieve or update some data from the application database. However, this layer doesn’t take responsibility for accessing the database; it sends requests to the next layer, the Data Access layer.
- The Data Access layer receives requests from the Business Logic layer and builds some queries to the database to handle these requests. Once the execution gets done, it sends the result back to the Business Logic layer.
- The Business Logic layer gets responses from the Data Access layer, then completes the process and sends the result to the Presentation Layer.
- The Presentation layer gets the responses and presents them to users via UI components.
The diagram below illustrates how three-layer architecture works.
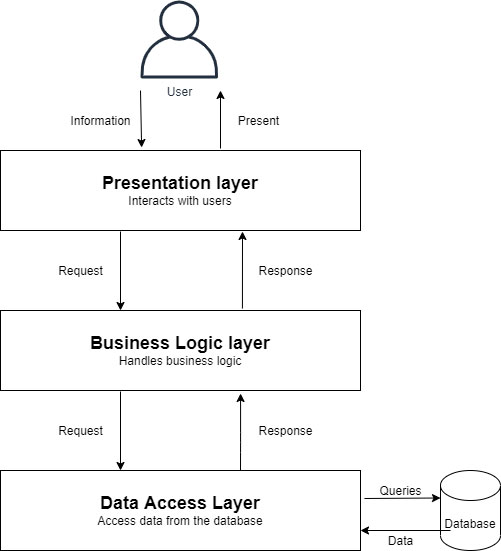
How to build and deploy a three-layer application in C#?
To guide you build and deploy a three-layer application, I have prepared a demo with the following components:
- Business Objects - Entity(Data Transfer Objects) layer: .NET Core class library
- Data Access layer: .NET Core class library
- Business Logic layer: .NET Core class library
- Presentation Layer: ASP.NET Core 5.0 Razor pages
The Business Objects layer includes objects that are used in the application and common helper functions (without logic) used for all layers. In a three-layer architecture, this layer is optional. However, as we follow the OOP with C#, we should reduce the duplicate codes as much as possible. Therefore, using a layer to keep common codes instead of holding them in each layer is essential.
To easily follow the article, you can download the demo of the three-layer architecture sample . It is built based on Repository + UnitOfWork pattern, which’s a well-designed pattern in C#. Here are the demo and practical examples of the Repository + UnitOfWork pattern .
The diagram below explains how the application was designed.
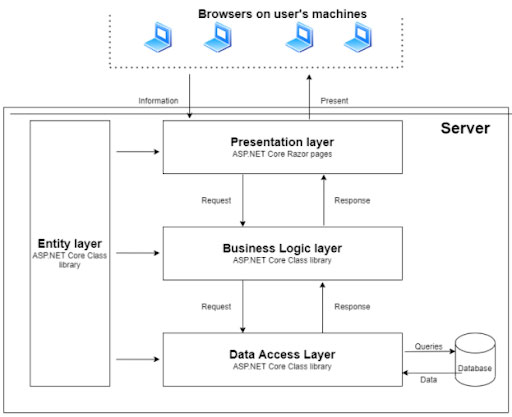
Firstly, create a database with the design below to store the application’s data. You can execute the ThreeLayerSample.Database.sql, which was placed inside the Data Access layer to create it.
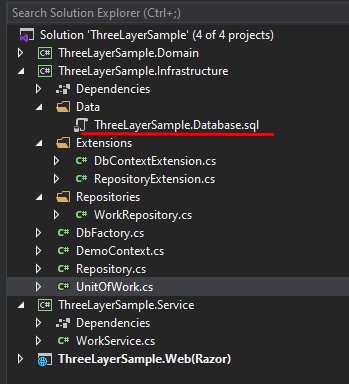
The demo will focus on getting the Work table items before representing them to users.
Business Objects/Entity layer
ThreeLayerSample.Domain class project in this example.
1. Connect to the database with Entity Framework Core
When we have the database, we need to create a mapping between the database and the application. Let’s open your Package Manager console in this layer, run a Scaffolding command, then replace SERVER, DATABASE, USER, and PASSWORD with suitable values based on your SQL Server settings.
Once the command has finished, the database tables should be created into the code and name entities. As the entities could be used in all layers without any database or business logic, we should keep them in this layer.
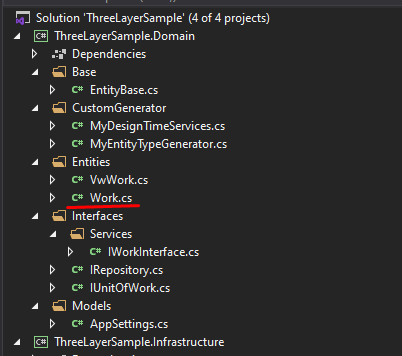
A DataContext class named “DemoContext” is also created. This class provides database access, so it should be placed in the Data Access layer.
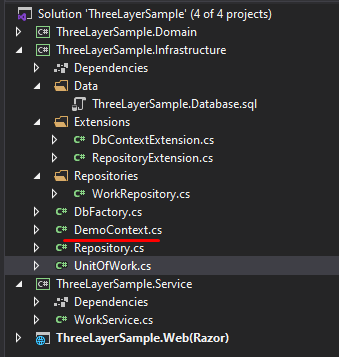
2. Create Generic interfaces for Repository and UnitOfWork
All data entities should have CRUD actions, so let’s create a generic interface named IRepository, and then the repository of each entity should implement this interface.
ThreeLayerDomain/Interfaces/IRepository.cs
We need another interface named IUnitOfWork as follows:
ThreeLayerDomain/Interfaces/IUnitOfWork.cs
They are interfaces without business/database logic here, so I put them in this layer.
Data Access layer
ThreeLayerSample.Infrastructure class project in this example.
1. Implement generic classes
By applying Generic Repository and Unit of Work design patterns, the data access layer classes are implemented as follows:
ThreeLayerSample.Infrastructure/Repository.cs
ThreeLayerSample.Infrastructure/UnitOfWork.cs
And another DbFactory class that will initialize a DbContext when we use it.
ThreeLayerSample.Infrastructure/DbFactory.cs
Business Logic layer
ThreeLayerSample.Service class project in this example.
1. Create an interface for the service
Let’s create the interface for the WorkService that the Presentation layer depends on as we don’t want to tighten the Presentation layer and Business Logic layer with a concrete implementation.
ThreeLayerSample.Domain/Interfaces/Services/IWorkService.cs
2. Implement code for the service
Next, implement business logic processing the Work service in a class named WorkService as follows. This is the business logic processing code, so we put this class in the Business Logic layer. As you can see, the service requests to get all items of the Work table via the Repository instance of the Work entity, which was implemented in the generic repository.
ThreeLayerSample.Service/WorkService.cs
Presentation layer
ThreeLayerSample.Web(Razor) ASP.NET Core Web App in this example.
Follow this tutorial to create an ASP.NET Core Razor page application . Once the application is created, create a ServiceCollectionExtensions class under the Extensions folder.
ThreeLayerSample.Web_Razor_/Extensions/ServiceCollectionExtensions .cs
Add your connection string into the appsettings.json file, and it’s the connection string that the Data Access layer will employ to establish a connection to the database (same value as the connection string in the Scaffold command).
ThreeLayerSample.Web_Razor_/appsettings.json
Add an AppSettings class to the Entity layer.
ThreeLayerSample.Domain/Models/AppSettings.cs
Open the Startup.cs file, then add the following codes.
At its constructor: read data from appsettings.json, then store it in the created AppSettings class.
In the ConfigureServices: register instances for DataContext, its Factory, UnitOfWork, and WorkService to the application (using extension methods in ServiceCollectionExtensions class).
ThreeLayerSample.Web_Razor_/Startup.cs
Open Index.cshtml.cs file, add the following code to inject WorkService, then get data from WorkService and set it to Works property.
ThreeLayerSample.Web_Razor_/Pages/Index.cshtml.cs
In the Index.cshtml file, add the following code to present data to the UI.
ThreeLayerSample.Web_Razor_/Pages/Index.cshtml.
Run the application to check for the result.
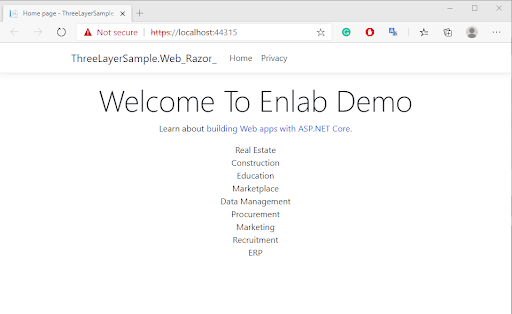
Create a folder to store your source code.
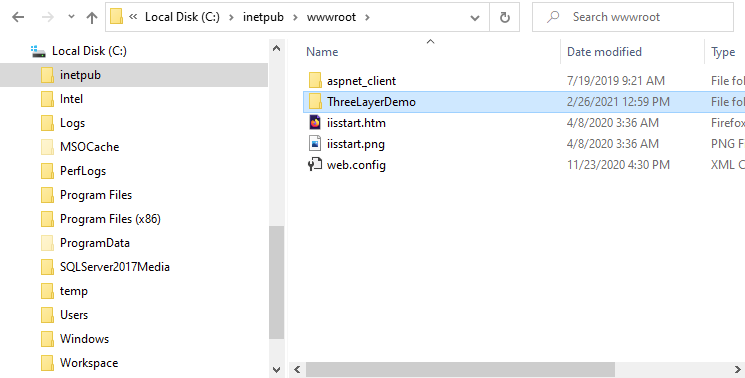
Open your IIS, then choose Create Website to host your application. Provide a name, a specific port, and a physical path to the source code folder for the Content Directory section. Make sure “Start website immediately” is ticked.
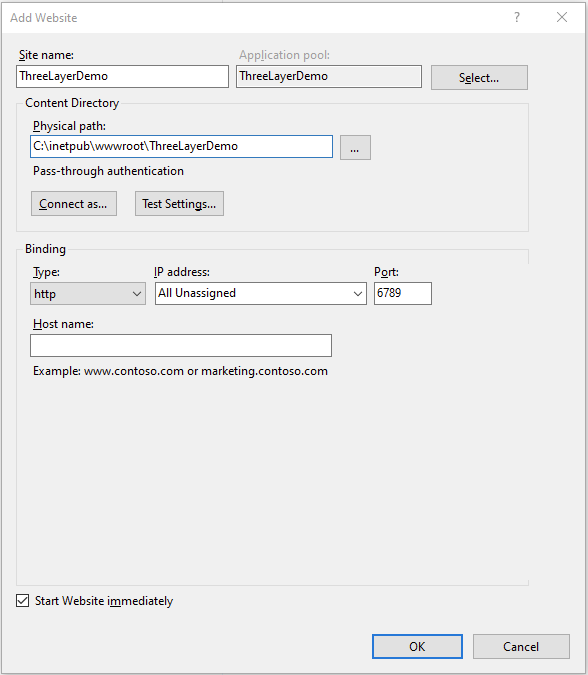
Open your solution in your Visual Studio, then follow these steps:
Right-click on ThreeLayerSample.Web(Razor), select Publish.
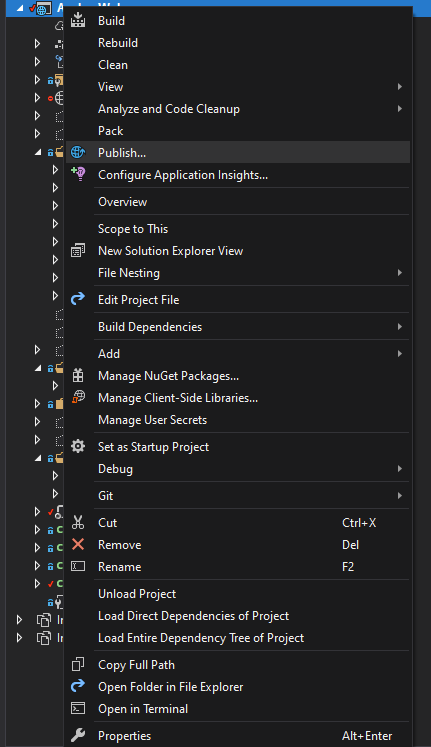
Select “Folder”.
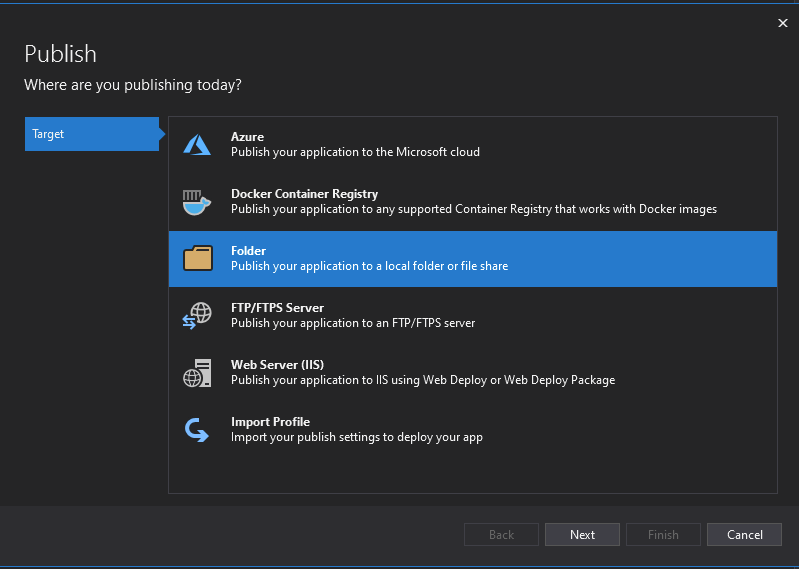
Enter “Folder location” by creating the source code folder path below.
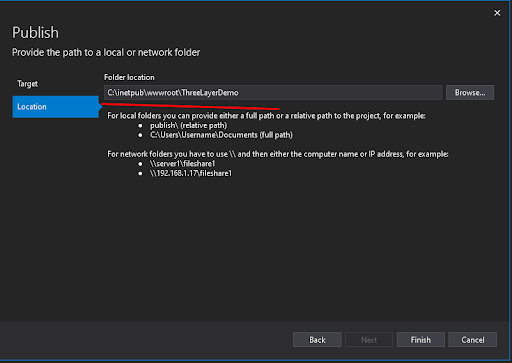
Click to publish the project.
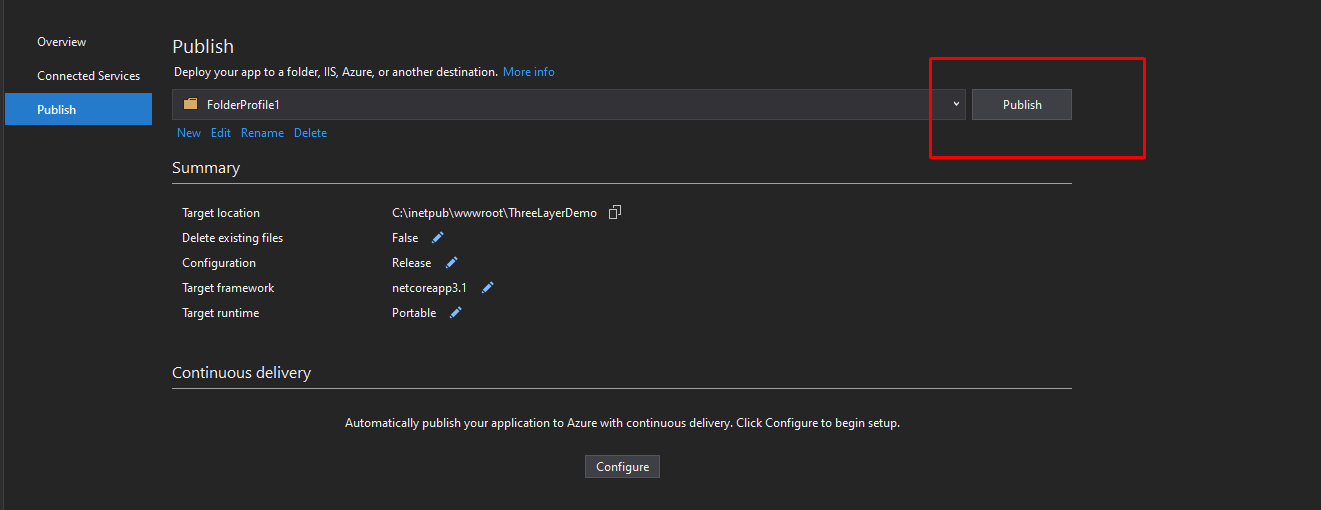
Once you’ve done publishing, open the application on your browser to check for the result.
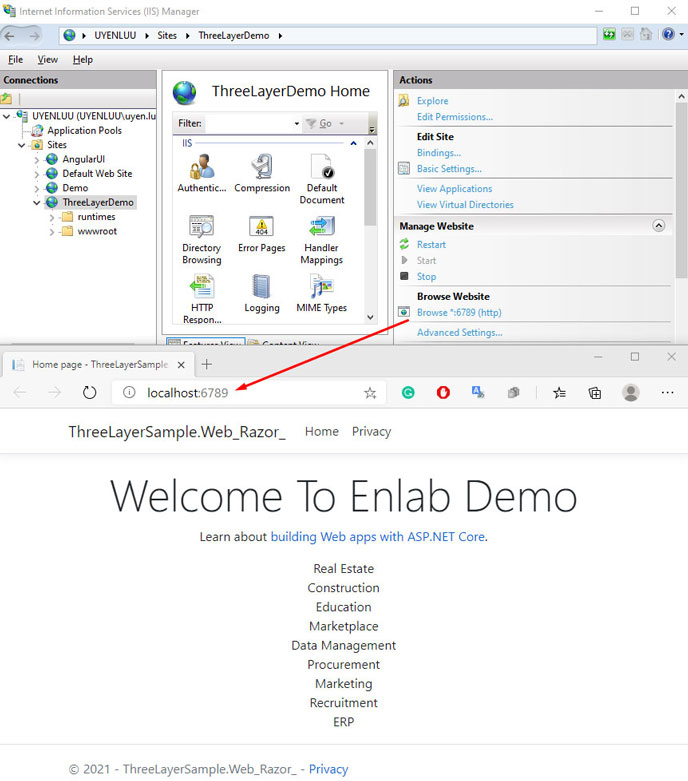
The layered architecture is well-designed for software development that helps organize your code with high maintainability, reusability, and readability. However, even though codes are well-organized in layers, they are deployed and run on the same physical machine, even on the same process. This may not be the right choice for complex applications, which require high availability and stability. We need to upgrade this architecture to another higher level named three-tier architecture, which I will share in my next article.
Thank you for reading, and happy coding!
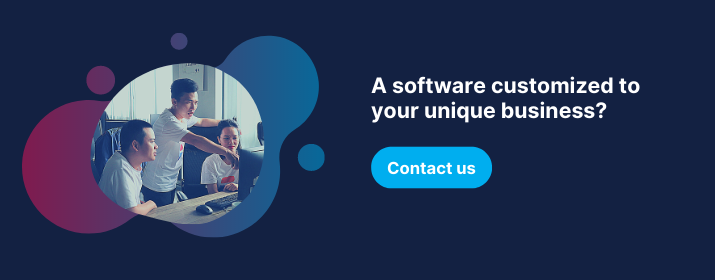
- Loc Nguyen, How to implement Repository & Unit of Work design patterns in .NET Core with practical examples [Part 1] , enlabsoftware.com/, 2021.
- Anant Patil, Three Layered Architecture , www.ecanarys.com.
- Mark Richards, Software Architecture Patterns , www.oreilly.com.
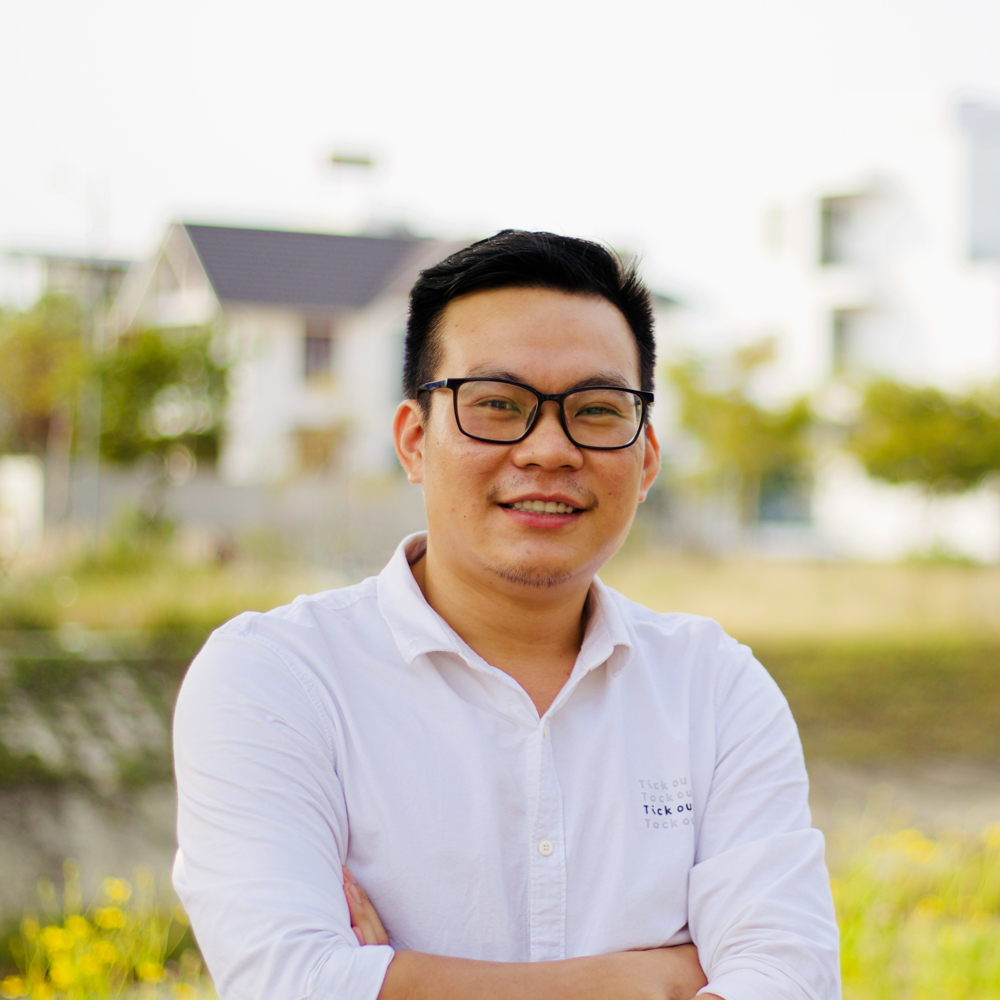
About the author
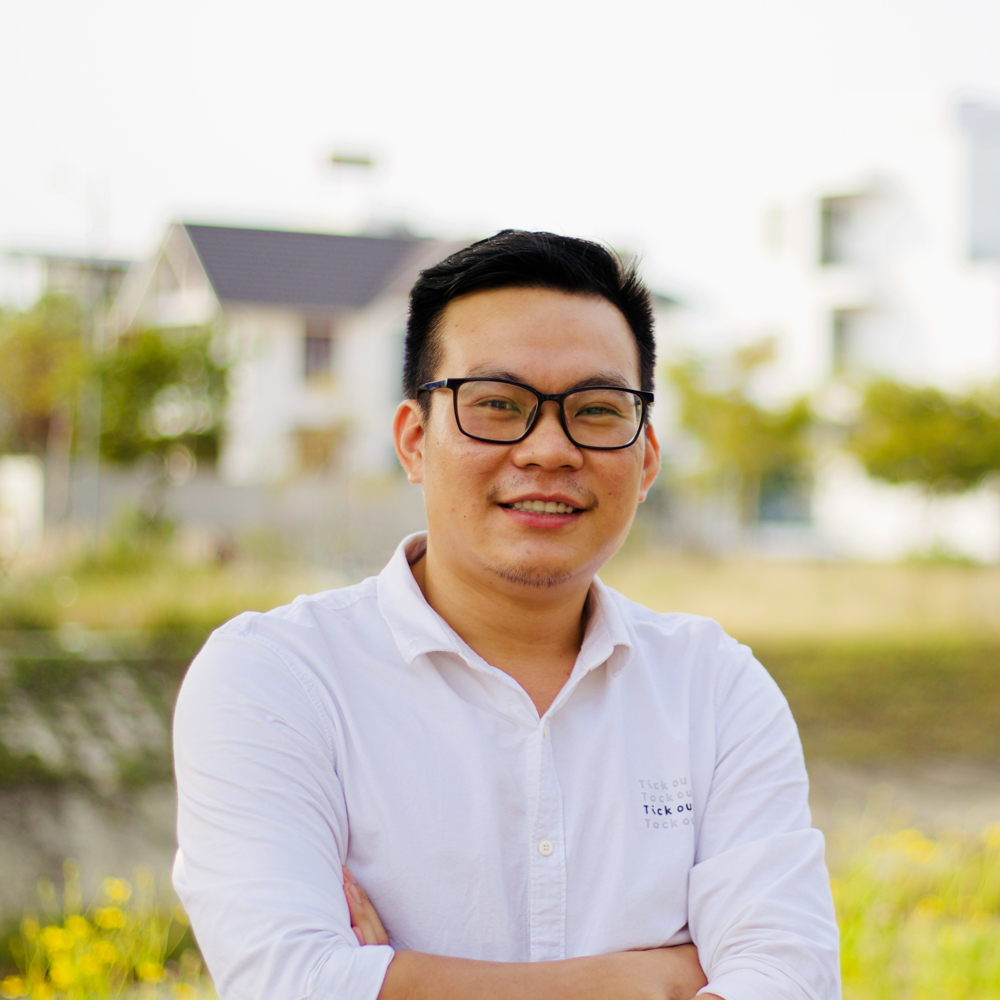
Can we send you our next blog posts? Only the best stuffs.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Common web application architectures
- 18 contributors
This content is an excerpt from the eBook, Architect Modern Web Applications with ASP.NET Core and Azure, available on .NET Docs or as a free downloadable PDF that can be read offline.
Download PDF

"If you think good architecture is expensive, try bad architecture." - Brian Foote and Joseph Yoder
Most traditional .NET applications are deployed as single units corresponding to an executable or a single web application running within a single IIS appdomain. This approach is the simplest deployment model and serves many internal and smaller public applications very well. However, even given this single unit of deployment, most non-trivial business applications benefit from some logical separation into several layers.
What is a monolithic application?
A monolithic application is one that is entirely self-contained, in terms of its behavior. It may interact with other services or data stores in the course of performing its operations, but the core of its behavior runs within its own process and the entire application is typically deployed as a single unit. If such an application needs to scale horizontally, typically the entire application is duplicated across multiple servers or virtual machines.
All-in-one applications
The smallest possible number of projects for an application architecture is one. In this architecture, the entire logic of the application is contained in a single project, compiled to a single assembly, and deployed as a single unit.
A new ASP.NET Core project, whether created in Visual Studio or from the command line, starts out as a simple "all-in-one" monolith. It contains all of the behavior of the application, including presentation, business, and data access logic. Figure 5-1 shows the file structure of a single-project app.

Figure 5-1. A single project ASP.NET Core app.
In a single project scenario, separation of concerns is achieved through the use of folders. The default template includes separate folders for MVC pattern responsibilities of Models, Views, and Controllers, as well as additional folders for Data and Services. In this arrangement, presentation details should be limited as much as possible to the Views folder, and data access implementation details should be limited to classes kept in the Data folder. Business logic should reside in services and classes within the Models folder.
Although simple, the single-project monolithic solution has some disadvantages. As the project's size and complexity grows, the number of files and folders will continue to grow as well. User interface (UI) concerns (models, views, controllers) reside in multiple folders, which aren't grouped together alphabetically. This issue only gets worse when additional UI-level constructs, such as Filters or ModelBinders, are added in their own folders. Business logic is scattered between the Models and Services folders, and there's no clear indication of which classes in which folders should depend on which others. This lack of organization at the project level frequently leads to spaghetti code .
To address these issues, applications often evolve into multi-project solutions, where each project is considered to reside in a particular layer of the application.
What are layers?
As applications grow in complexity, one way to manage that complexity is to break up the application according to its responsibilities or concerns. This approach follows the separation of concerns principle and can help keep a growing codebase organized so that developers can easily find where certain functionality is implemented. Layered architecture offers a number of advantages beyond just code organization, though.
By organizing code into layers, common low-level functionality can be reused throughout the application. This reuse is beneficial because it means less code needs to be written and because it can allow the application to standardize on a single implementation, following the don't repeat yourself (DRY) principle.
With a layered architecture, applications can enforce restrictions on which layers can communicate with other layers. This architecture helps to achieve encapsulation. When a layer is changed or replaced, only those layers that work with it should be impacted. By limiting which layers depend on which other layers, the impact of changes can be mitigated so that a single change doesn't impact the entire application.
Layers (and encapsulation) make it much easier to replace functionality within the application. For example, an application might initially use its own SQL Server database for persistence, but later could choose to use a cloud-based persistence strategy, or one behind a web API. If the application has properly encapsulated its persistence implementation within a logical layer, that SQL Server-specific layer could be replaced by a new one implementing the same public interface.
In addition to the potential of swapping out implementations in response to future changes in requirements, application layers can also make it easier to swap out implementations for testing purposes. Instead of having to write tests that operate against the real data layer or UI layer of the application, these layers can be replaced at test time with fake implementations that provide known responses to requests. This approach typically makes tests much easier to write and much faster to run when compared to running tests against the application's real infrastructure.
Logical layering is a common technique for improving the organization of code in enterprise software applications, and there are several ways in which code can be organized into layers.
Layers represent logical separation within the application. In the event that application logic is physically distributed to separate servers or processes, these separate physical deployment targets are referred to as tiers . It's possible, and quite common, to have an N-Layer application that is deployed to a single tier.
Traditional "N-Layer" architecture applications
The most common organization of application logic into layers is shown in Figure 5-2.

Figure 5-2. Typical application layers.
These layers are frequently abbreviated as UI, BLL (Business Logic Layer), and DAL (Data Access Layer). Using this architecture, users make requests through the UI layer, which interacts only with the BLL. The BLL, in turn, can call the DAL for data access requests. The UI layer shouldn't make any requests to the DAL directly, nor should it interact with persistence directly through other means. Likewise, the BLL should only interact with persistence by going through the DAL. In this way, each layer has its own well-known responsibility.
One disadvantage of this traditional layering approach is that compile-time dependencies run from the top to the bottom. That is, the UI layer depends on the BLL, which depends on the DAL. This means that the BLL, which usually holds the most important logic in the application, is dependent on data access implementation details (and often on the existence of a database). Testing business logic in such an architecture is often difficult, requiring a test database. The dependency inversion principle can be used to address this issue, as you'll see in the next section.
Figure 5-3 shows an example solution, breaking the application into three projects by responsibility (or layer).

Figure 5-3. A simple monolithic application with three projects.
Although this application uses several projects for organizational purposes, it's still deployed as a single unit and its clients will interact with it as a single web app. This allows for very simple deployment process. Figure 5-4 shows how such an app might be hosted using Azure.

Figure 5-4. Simple deployment of Azure Web App
As application needs grow, more complex and robust deployment solutions may be required. Figure 5-5 shows an example of a more complex deployment plan that supports additional capabilities.

Figure 5-5. Deploying a web app to an Azure App Service
Internally, this project's organization into multiple projects based on responsibility improves the maintainability of the application.
This unit can be scaled up or out to take advantage of cloud-based on-demand scalability. Scaling up means adding additional CPU, memory, disk space, or other resources to the server(s) hosting your app. Scaling out means adding additional instances of such servers, whether these are physical servers, virtual machines, or containers. When your app is hosted across multiple instances, a load balancer is used to assign requests to individual app instances.
The simplest approach to scaling a web application in Azure is to configure scaling manually in the application's App Service Plan. Figure 5-6 shows the appropriate Azure dashboard screen to configure how many instances are serving an app.

Figure 5-6. App Service Plan scaling in Azure.
Clean architecture
Applications that follow the Dependency Inversion Principle as well as the Domain-Driven Design (DDD) principles tend to arrive at a similar architecture. This architecture has gone by many names over the years. One of the first names was Hexagonal Architecture, followed by Ports-and-Adapters. More recently, it's been cited as the Onion Architecture or Clean Architecture . The latter name, Clean Architecture, is used as the name for this architecture in this e-book.
The eShopOnWeb reference application uses the Clean Architecture approach in organizing its code into projects. You can find a solution template you can use as a starting point for your own ASP.NET Core solutions in the ardalis/cleanarchitecture GitHub repository or by installing the template from NuGet .
Clean architecture puts the business logic and application model at the center of the application. Instead of having business logic depend on data access or other infrastructure concerns, this dependency is inverted: infrastructure and implementation details depend on the Application Core. This functionality is achieved by defining abstractions, or interfaces, in the Application Core, which are then implemented by types defined in the Infrastructure layer. A common way of visualizing this architecture is to use a series of concentric circles, similar to an onion. Figure 5-7 shows an example of this style of architectural representation.

Figure 5-7. Clean Architecture; onion view
In this diagram, dependencies flow toward the innermost circle. The Application Core takes its name from its position at the core of this diagram. And you can see on the diagram that the Application Core has no dependencies on other application layers. The application's entities and interfaces are at the very center. Just outside, but still in the Application Core, are domain services, which typically implement interfaces defined in the inner circle. Outside of the Application Core, both the UI and the Infrastructure layers depend on the Application Core, but not on one another (necessarily).
Figure 5-8 shows a more traditional horizontal layer diagram that better reflects the dependency between the UI and other layers.

Figure 5-8. Clean Architecture; horizontal layer view
Note that the solid arrows represent compile-time dependencies, while the dashed arrow represents a runtime-only dependency. With the clean architecture, the UI layer works with interfaces defined in the Application Core at compile time, and ideally shouldn't know about the implementation types defined in the Infrastructure layer. At run time, however, these implementation types are required for the app to execute, so they need to be present and wired up to the Application Core interfaces via dependency injection.
Figure 5-9 shows a more detailed view of an ASP.NET Core application's architecture when built following these recommendations.

Figure 5-9. ASP.NET Core architecture diagram following Clean Architecture.
Because the Application Core doesn't depend on Infrastructure, it's very easy to write automated unit tests for this layer. Figures 5-10 and 5-11 show how tests fit into this architecture.

Figure 5-10. Unit testing Application Core in isolation.

Figure 5-11. Integration testing Infrastructure implementations with external dependencies.
Since the UI layer doesn't have any direct dependency on types defined in the Infrastructure project, it's likewise very easy to swap out implementations, either to facilitate testing or in response to changing application requirements. ASP.NET Core's built-in use of and support for dependency injection makes this architecture the most appropriate way to structure non-trivial monolithic applications.
For monolithic applications, the Application Core, Infrastructure, and UI projects are all run as a single application. The runtime application architecture might look something like Figure 5-12.

Figure 5-12. A sample ASP.NET Core app's runtime architecture.
Organizing code in Clean Architecture
In a Clean Architecture solution, each project has clear responsibilities. As such, certain types belong in each project and you'll frequently find folders corresponding to these types in the appropriate project.
Application Core
The Application Core holds the business model, which includes entities, services, and interfaces. These interfaces include abstractions for operations that will be performed using Infrastructure, such as data access, file system access, network calls, etc. Sometimes services or interfaces defined at this layer will need to work with non-entity types that have no dependencies on UI or Infrastructure. These can be defined as simple Data Transfer Objects (DTOs).
Application Core types
- Entities (business model classes that are persisted)
- Aggregates (groups of entities)
- Domain Services
- Specifications
- Custom Exceptions and Guard Clauses
- Domain Events and Handlers
Infrastructure
The Infrastructure project typically includes data access implementations. In a typical ASP.NET Core web application, these implementations include the Entity Framework (EF) DbContext, any EF Core Migration objects that have been defined, and data access implementation classes. The most common way to abstract data access implementation code is through the use of the Repository design pattern .
In addition to data access implementations, the Infrastructure project should contain implementations of services that must interact with infrastructure concerns. These services should implement interfaces defined in the Application Core, and so Infrastructure should have a reference to the Application Core project.
Infrastructure types
- EF Core types ( DbContext , Migration )
- Data access implementation types (Repositories)
- Infrastructure-specific services (for example, FileLogger or SmtpNotifier )
The user interface layer in an ASP.NET Core MVC application is the entry point for the application. This project should reference the Application Core project, and its types should interact with infrastructure strictly through interfaces defined in Application Core. No direct instantiation of or static calls to the Infrastructure layer types should be allowed in the UI layer.
UI Layer types
- Controllers
- Custom Filters
- Custom Middleware
The Startup class or Program.cs file is responsible for configuring the application, and for wiring up implementation types to interfaces. The place where this logic is performed is known as the app's composition root , and is what allows dependency injection to work properly at run time.
In order to wire up dependency injection during app startup, the UI layer project may need to reference the Infrastructure project. This dependency can be eliminated, most easily by using a custom DI container that has built-in support for loading types from assemblies. For the purposes of this sample, the simplest approach is to allow the UI project to reference the Infrastructure project (but developers should limit actual references to types in the Infrastructure project to the app's composition root).
Monolithic applications and containers
You can build a single and monolithic-deployment based Web Application or Service and deploy it as a container. Within the application, it might not be monolithic but organized into several libraries, components, or layers. Externally, it's a single container with a single process, single web application, or single service.
To manage this model, you deploy a single container to represent the application. To scale, just add additional copies with a load balancer in front. The simplicity comes from managing a single deployment in a single container or VM.

You can include multiple components/libraries or internal layers within each container, as illustrated in Figure 5-13. But, following the container principle of "a container does one thing, and does it in one process ", the monolithic pattern might be a conflict.
The downside of this approach comes if/when the application grows, requiring it to scale. If the entire application scales, it's not really a problem. However, in most cases, a few parts of the application are the choke points requiring scaling, while other components are used less.
Using the typical eCommerce example, what you likely need to scale is the product information component. Many more customers browse products than purchase them. More customers use their basket than use the payment pipeline. Fewer customers add comments or view their purchase history. And you likely only have a handful of employees, in a single region, that need to manage the content and marketing campaigns. By scaling the monolithic design, all the code is deployed multiple times.
In addition to the "scale everything" problem, changes to a single component require complete retesting of the entire application, and a complete redeployment of all the instances.
The monolithic approach is common, and many organizations are developing with this architectural approach. Many are having good enough results, while others are hitting limits. Many designed their applications in this model, because the tools and infrastructure were too difficult to build service-oriented architectures (SOA), and they didn't see the need until the app grew. If you find you're hitting the limits of the monolithic approach, breaking up the app to enable it to better leverage containers and microservices may be the next logical step.

Deploying monolithic applications in Microsoft Azure can be achieved using dedicated VMs for each instance. Using Azure Virtual Machine Scale Sets , you can easily scale the VMs. Azure App Services can run monolithic applications and easily scale instances without having to manage the VMs. Azure App Services can run single instances of Docker containers as well, simplifying the deployment. Using Docker, you can deploy a single VM as a Docker host, and run multiple instances. Using the Azure balancer, as shown in the Figure 5-14, you can manage scaling.
The deployment to the various hosts can be managed with traditional deployment techniques. The Docker hosts can be managed with commands like docker run performed manually, or through automation such as Continuous Delivery (CD) pipelines.
Monolithic application deployed as a container
There are benefits of using containers to manage monolithic application deployments. Scaling the instances of containers is far faster and easier than deploying additional VMs. Even when using virtual machine scale sets to scale VMs, they take time to create. When deployed as app instances, the configuration of the app is managed as part of the VM.
Deploying updates as Docker images is far faster and network efficient. Docker Images typically start in seconds, speeding rollouts. Tearing down a Docker instance is as easy as issuing a docker stop command, typically completing in less than a second.
As containers are inherently immutable by design, you never need to worry about corrupted VMs, whereas update scripts might forget to account for some specific configuration or file left on the disk.
You can use Docker containers for a monolithic deployment of simpler web applications. This approach improves continuous integration and continuous deployment pipelines and helps achieve deployment-to-production success. No more “It works on my machine, why does it not work in production?”
A microservices-based architecture has many benefits, but those benefits come at a cost of increased complexity. In some cases, the costs outweigh the benefits, so a monolithic deployment application running in a single container or in just a few containers is a better option.
A monolithic application might not be easily decomposable into well-separated microservices. Microservices should work independently of each other to provide a more resilient application. If you can't deliver independent feature slices of the application, separating it only adds complexity.
An application might not yet need to scale features independently. Many applications, when they need to scale beyond a single instance, can do so through the relatively simple process of cloning that entire instance. The additional work to separate the application into discrete services provides a minimal benefit when scaling full instances of the application is simple and cost-effective.
Early in the development of an application, you might not have a clear idea where the natural functional boundaries are. As you develop a minimum viable product, the natural separation might not yet have emerged. Some of these conditions might be temporary. You might start by creating a monolithic application, and later separate some features to be developed and deployed as microservices. Other conditions might be essential to the application's problem space, meaning that the application might never be broken into multiple microservices.
Separating an application into many discrete processes also introduces overhead. There's more complexity in separating features into different processes. The communication protocols become more complex. Instead of method calls, you must use asynchronous communications between services. As you move to a microservices architecture, you need to add many of the building blocks implemented in the microservices version of the eShopOnContainers application: event bus handling, message resiliency and retries, eventual consistency, and more.
The much simpler eShopOnWeb reference application supports single-container monolithic container usage. The application includes one web application that includes traditional MVC views, web APIs, and Razor Pages. Optionally, you can run the application's Blazor-based admin component, which requires a separate API project to run as well.
The application can be launched from the solution root using the docker-compose build and docker-compose up commands. This command configures a container for the web instance, using the Dockerfile found in the web project's root, and runs the container on a specified port. You can download the source for this application from GitHub and run it locally. Even this monolithic application benefits from being deployed in a container environment.
For one, the containerized deployment means that every instance of the application runs in the same environment. This approach includes the developer environment where early testing and development take place. The development team can run the application in a containerized environment that matches the production environment.
In addition, containerized applications scale out at a lower cost. Using a container environment enables greater resource sharing than traditional VM environments.
Finally, containerizing the application forces a separation between the business logic and the storage server. As the application scales out, the multiple containers will all rely on a single physical storage medium. This storage medium would typically be a high-availability server running a SQL Server database.
Docker support
The eShopOnWeb project runs on .NET. Therefore, it can run in either Linux-based or Windows-based containers. Note that for Docker deployment, you want to use the same host type for SQL Server. Linux-based containers allow a smaller footprint and are preferred.
You can use Visual Studio 2017 or later to add Docker support to an existing application by right-clicking on a project in Solution Explorer and choosing Add > Docker Support . This step adds the files required and modifies the project to use them. The current eShopOnWeb sample already has these files in place.
The solution-level docker-compose.yml file contains information about what images to build and what containers to launch. The file allows you to use the docker-compose command to launch multiple applications at the same time. In this case, it is only launching the Web project. You can also use it to configure dependencies, such as a separate database container.
The docker-compose.yml file references the Dockerfile in the Web project. The Dockerfile is used to specify which base container will be used and how the application will be configured on it. The Web ' Dockerfile :
Troubleshooting Docker problems
Once you run the containerized application, it continues to run until you stop it. You can view which containers are running with the docker ps command. You can stop a running container by using the docker stop command and specifying the container ID.
Note that running Docker containers may be bound to ports you might otherwise try to use in your development environment. If you try to run or debug an application using the same port as a running Docker container, you'll get an error stating that the server can't bind to that port. Once again, stopping the container should resolve the issue.
If you want to add Docker support to your application using Visual Studio, make sure Docker Desktop is running when you do so. The wizard won't run correctly if Docker Desktop isn't running when you start the wizard. In addition, the wizard examines your current container choice to add the correct Docker support. If you want to add, support for Windows Containers, you need to run the wizard while you have Docker Desktop running with Windows Containers configured. If you want to add, support for Linux containers, run the wizard while you have Docker running with Linux containers configured.
Other web application architectural styles
- Web-Queue-Worker : The core components of this architecture are a web front end that serves client requests, and a worker that performs resource-intensive tasks, long-running workflows, or batch jobs. The web front end communicates with the worker through a message queue.
- N-tier : An N-tier architecture divides an application into logical layers and physical tiers.
- Microservice : A microservices architecture consists of a collection of small, autonomous services. Each service is self-contained and should implement a single business capability within a bounded context.
References – Common web architectures
- The Clean Architecture https://blog.cleancoder.com/uncle-bob/2012/08/13/the-clean-architecture.html
- The Onion Architecture https://jeffreypalermo.com/blog/the-onion-architecture-part-1/
- The Repository Pattern https://deviq.com/repository-pattern/
- Clean Architecture Solution Template https://github.com/ardalis/cleanarchitecture
- Architecting Microservices e-book https://aka.ms/MicroservicesEbook
- DDD (Domain-Driven Design) https://learn.microsoft.com/dotnet/architecture/microservices/microservice-ddd-cqrs-patterns/
Previous Next
Additional resources

Clean Architecture with .NET and .NET Core — Overview

Ashish Patel
Introduction to Clean Architecture with ASP.NET Core: Getting started!
Clean Architecture style focus on a loosely coupled implementation of use cases. Use cases as central organizing structure, decoupled from frameworks and technology details.
Clean Architecture Overview
With Clean Architecture, the Domain and Application layers are at the center of the design which is known as the Core of the system. Business Logic places into these two layers, while they contain different kinds of business logic. They are seen as details and the business layers should not depend on Presentation and Infrastructure layers. Instead of having business logic depend on data access or other infrastructure concerns, this dependency is inverted: infrastructure and implementation details depend on the Application layer. This functionality is achieved by defining abstractions, or interfaces, in the Application layer, which are then implemented by types defined in the Infrastructure layer. A common way of visualizing this architecture is to use a series of concentric circles, similar to an onion architecture.
The Domain layer contains enterprise logic and types and the Application layer contains business logic and types. The difference is that enterprise logic could be shared across many systems, whereas the business logic will typically only be used within a system.
Core should not be dependent on data access and other infrastructure concerns so those dependencies are inverted. This is achieved by adding interfaces or abstractions within Core that are implemented by layers outside of Core .
All dependencies flow inwards and Core has no dependency on any other layer. Infrastructure and Presentation depend on Core , but not on one another.
Domain Layer
Domain Layer implements the core, use-case independent business logic of the domain/system. By design, this layer is highly abstracted and stable. This layer contains a considerable amount of domain entities and should not depend on external libraries and frameworks. Ideally it should be loosely coupled even to the .NET Framework.
Domain project is core and backbone project. It is the heart and center project of the Clean Architecture design. All other projects should be depended on the Domain project.
This package contains the high level modules which describe the Domain via Aggregate Roots, Entities and Value Objects.
Domain layer contains:
- Value Objects
- Domain Events
Application Layer
Application Layer implements the use cases of the application based on the domain. A use case can be thought as a user interaction on the User Interface (UI). This layer contains all application logic. It is dependent on the domain layer, but has no dependencies on any other layer or project. This layer defines interfaces that are implemented by outside layers.
Application layer contains the application Use Cases which orchestrate the high level business rules. By design the orchestration will depend on abstractions of external services (e.g. Repositories). The package exposes Boundaries Interfaces (in other terms Contracts or Ports or Interfaces) which are used by the User Interface.
For example, if the application need to access a email service, a new interface would be added to application and an implementation would be created within infrastructure.
Application layer contains:
- Abstractions/Contracts/Interfaces
- Application Services/Handlers
- Commands and Queries
- Models (DTOs)
- Specifications
Infrastructure Layer
This layer is responsible to implement the Contracts (Interfaces/Adapters) defined within the application layer to the Secondary Actors. Infrastructure Layer supports other layer by implementing the abstractions and integrations to 3rd-party library and systems.
Infrastructure layer contains most of your application’s dependencies on external resources such as file systems, web services, third party APIs, and so on. The implementation of services should be based on interfaces defined within the application layer.
If you have a very large project with many dependencies, it may make sense to have multiple Infrastructure projects, but for most projects one Infrastructure project with folders works fine.
- Infrastructure.Persistence - Infrastructure.Persistence.MySQL - Infrastructure.Persistence.MongoDB
- Infrastructure.Identity
Infrastructure layer contains:
- Identity Services
- File Storage Services
- Queue Storage Services
- Message Bus Services
- Payment Services
- Third-party Services
- Notifications - Email Service - Sms Service
Persistence Layer
This layer handles database concerns and other data access operations. By design the infrastructure depends on Application layer. This project contains implementations of the interfaces (e.g. Repositories) that defined in the Application project.
For instance an SQL Server Database is a secondary actor which is affected by the application use cases, all the implementation and dependencies required to consume the SQL Server is created on infrastructure (persistence) layer.
For example, if you wanted to implement the Repository pattern you would do so by adding an interface within Application layer and adding the implementation within Persistence (Infrastructure) layer.
Persistence layer contains:
- Data Context
- Repositories
- Data Seeding
- Data Migrations
- Caching (Distributed, In-Memory)
User Interface (Web/Api) Layer
This layer is also called a Presentation. Presentation Layer contains the UI elements (pages, components) of the application. It handles the presentation (UI, API, etc.) concerns. This layer is responsible for rendering the Graphical User Interface (GUI) to interact with the user or JSON data to other systems. It is the application entry point.
User Interface layer depends on both the Application and Infrastructure layers, however, the dependency on Infrastructure is only to support dependency injection. This layer can be an ASP.NET Core Web API with Single Page Application (SPA like Angular, React) or it can be an ASP.NET Core MVC with Razor Views.
This layer receives HTTP Requests from users, and Presenters converts the application outputs into ViewModels that are rendered as HTTP Responses.
User Interface layer contains:
- Controllers
- View Models
- Middlewares
- Filters/Attributes
- Web/API Utilities
Useful NuGet Packages
- Entity Framework Core
- FluentValidation
- Swashbuckle (Swagger)
View more from .NET Hub
- Top .NET (ASP.NET Core) Open-Source Projects
- Most popular .NET Libraries every developer should know
- Use MediatR in ASP.NET or ASP.NET Core
- Use FluentValidation in ASP.NET or ASP.NET Core
- Use AutoMapper in ASP.NET or ASP.NET Core
- Use Autofac IoC Container in ASP.NET or ASP.NET Core
Happy Coding!!!
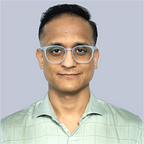
Written by Ashish Patel
Cloud Architect • 4x AWS Certified • 6x Azure Certified • 1x Kubernetes Certified • MCP • .NET • Terraform • DevOps • Blogger [ https://bit.ly/iamashishpatel ]
Text to speech
- Clean Architecture
- Modular Monolith
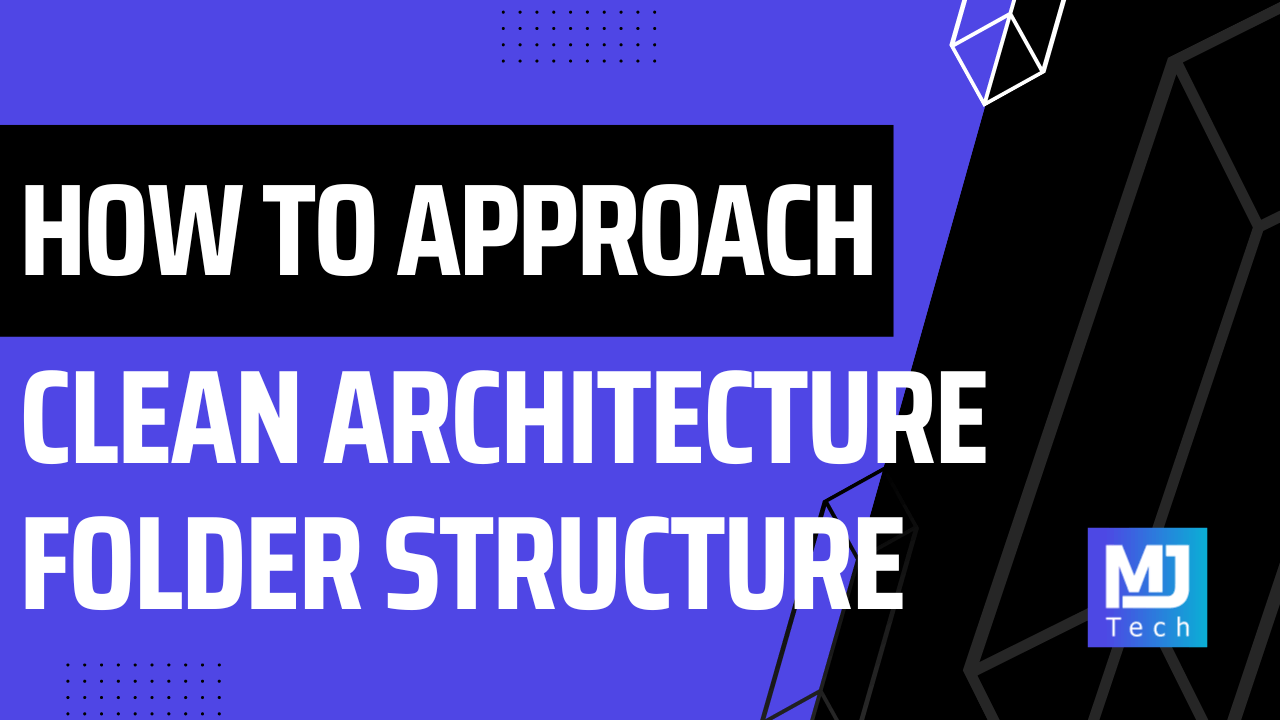
How To Approach Clean Architecture Folder Structure
3 min read · September 24, 2022
Clean Architecture is a popular approach to structuring your application.
It's a layered architecture that splits the project into four layers:
- Application
- Infrastructure
- Presentation
Each of the layers is typically one project in your solution.
Here's a visual representation of the Clean Architecture :
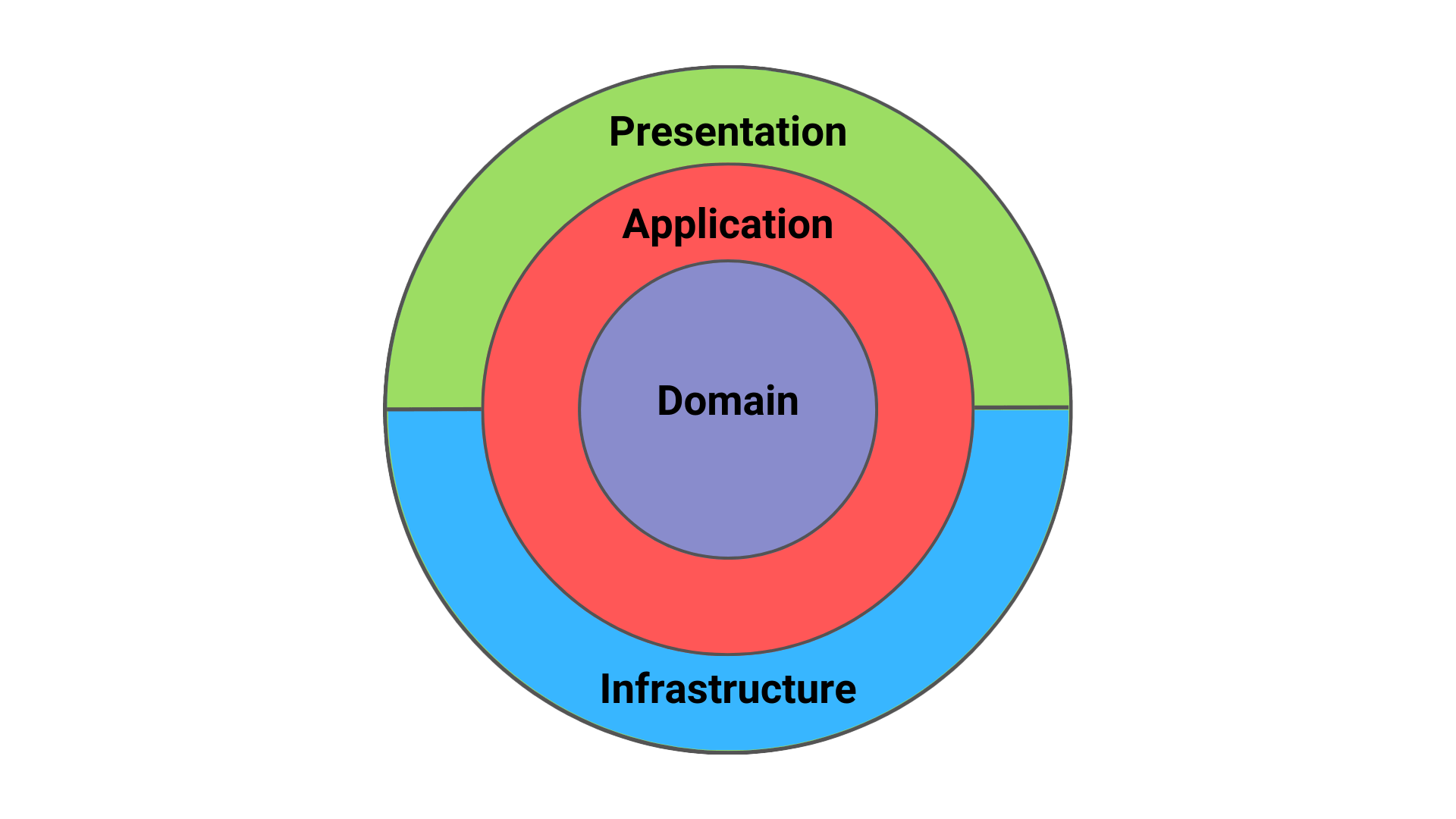
How do we create this in our .NET solutions?
Domain Layer
The Domain layer sits at the core of the Clean Architecture . Here we define things like: entities, value objects, aggregates, domain events, exceptions, repository interfaces, etc.
Here is the folder structure I like to use:
You can introduce more things here if you think it's required.
One thing to note is that the Domain layer is not allowed to reference other projects in your solution.
Application Layer
The Application layer sits right above the Domain layer . It acts as an orchestrator for the Domain layer , containing the most important use cases in your application.
You can structure your use cases using services or using commands and queries.
I'm a big fan of the CQRS pattern, so I like to use the command and query approach.
In the Abstractions folder, I define the interfaces required for the Application layer . The implementations for these interfaces are in one of the upper layers.
For every entity in the Domain layer , I create one folder with the commands, queries, and events definitions.
Infrastructure Layer
The Infrastructure layer contains implementations for external-facing services.
What would fall into this category?
- Databases - PostgreSQL, MongoDB
- Identity providers - Auth0, Keycloak
- Emails providers
- Storage services - AWS S3, Azure Blob Storage
- Message queues - Rabbit MQ
I place my DbContext implementation here if I'm using EF Core .
It's not uncommon to make the Persistence folder its project. I frequently do this to have all database facing-code inside of one project.
Presentation Layer
The Presentation layer is the entry point to our system. Typically, you would implement this as a Web API project.
The most important part of the Presentation layer is the Controllers , which define the API endpoints in our system.
Sometimes, I will move the Presentation layer away from the actual Web API project. I do this to isolate the Controllers and enforce stricter constraints. You don't have to do this if it is too complicated for you.
Is This The Only Way?
You don't have to follow the folder structure I proposed to the T. Clean Architecture is very flexible, and you can experiment with it and structure it the way you like.
Do you like more granularity? Create more specific projects.
Do you dislike a lot of projects? Separate concerns using folders.
I'm here to give you options to explore. But it's up to you to decide what's best.
Whenever you're ready, there are 4 ways I can help you:
- Pragmatic Clean Architecture: Join 3,000+ students in this comprehensive course that will teach you the system I use to ship production-ready applications using Clean Architecture. Learn how to apply the best practices of modern software architecture.
- Modular Monolith Architecture: Join 850+ engineers in this in-depth course that will transform the way you build modern systems. You will learn the best practices for applying the Modular Monolith architecture in a real-world scenario.
- Patreon Community: Join a community of 1,000+ engineers and software architects. You will also unlock access to the source code I use in my YouTube videos, early access to future videos, and exclusive discounts for my courses.
- Promote yourself to 53,000+ subscribers by sponsoring this newsletter.
Become a Better .NET Software Engineer
Join 53,000+ engineers who are improving their skills every Saturday morning.
Accelerate Your .NET Skills 🚀
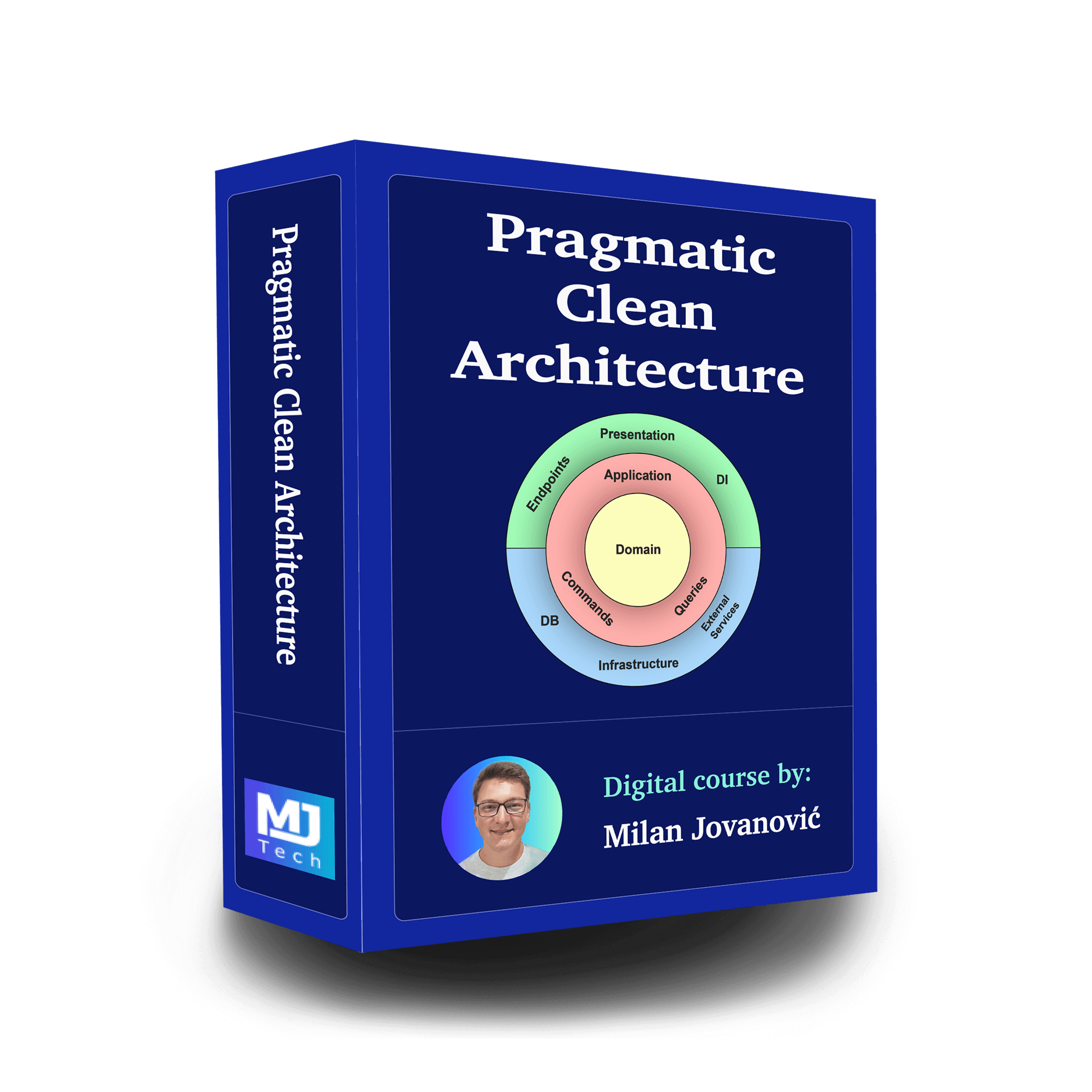
Pragmatic Clean Architecture
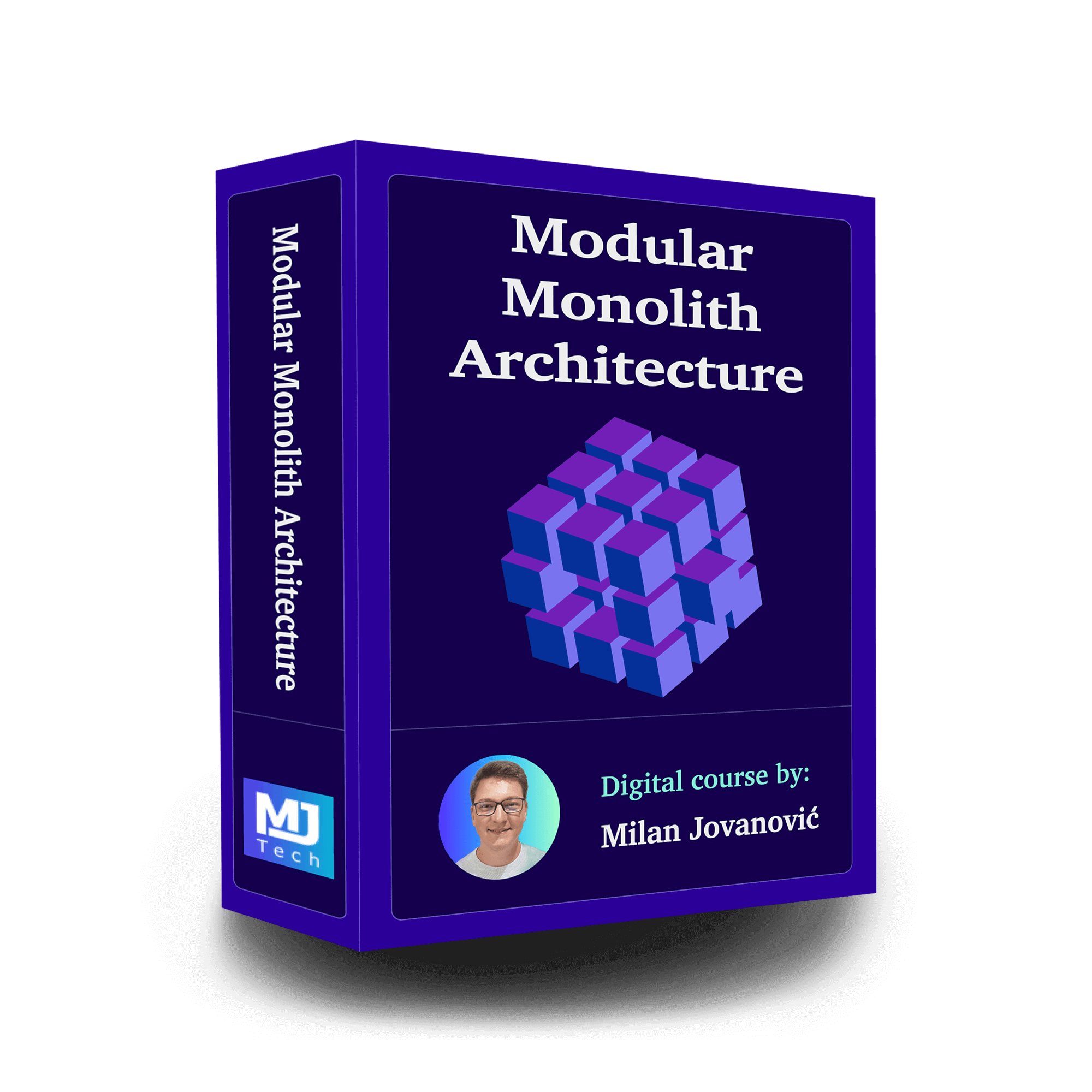
Modular Monolith Architecture
The .NET Weekly 📧
Join more than 53,000+ engineers, and get practical tips and resources every week to improve your .NET and software architecture skills.
Enterprise Application Architecture with .NET Core by Ganesan Senthilvel, Ovais Mehboob Ahmed Khan, Habib Ahmed Qureshi
Get full access to Enterprise Application Architecture with .NET Core and 60K+ other titles, with a free 10-day trial of O'Reilly.
There are also live events, courses curated by job role, and more.
Presentation layer
This layer contains all the user interfaces of an application. In terms of ASP.NET Core, it contains MVC controllers, views, static files (images, HTML pages), JavaScript files, and other client-side frameworks like Angular, Knockout, and others. This is the frontend layer with which the user interacts. Keeping this layer separate facilitates upgrading the existing interfaces or design without making any changes to the backend layers.
There are some architectural principles which should be followed when designing the presentation layer. A good presentation layer design can be achieved by following these three principles:
- First of all, our data should be separated from view and use patterns like MVC, MVVM, or MVP to address ...
Get Enterprise Application Architecture with .NET Core now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.
Don’t leave empty-handed
Get Mark Richards’s Software Architecture Patterns ebook to better understand how to design components—and how they should interact.
It’s yours, free.
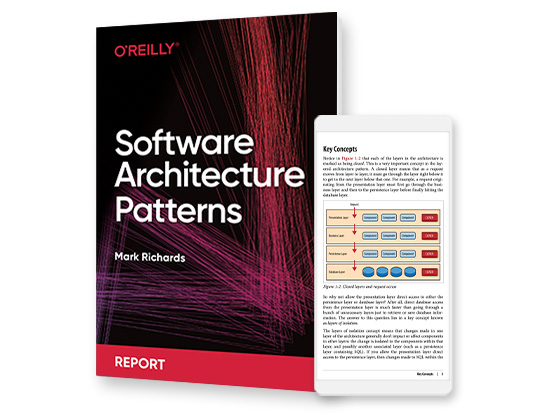
Check it out now on O’Reilly
Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
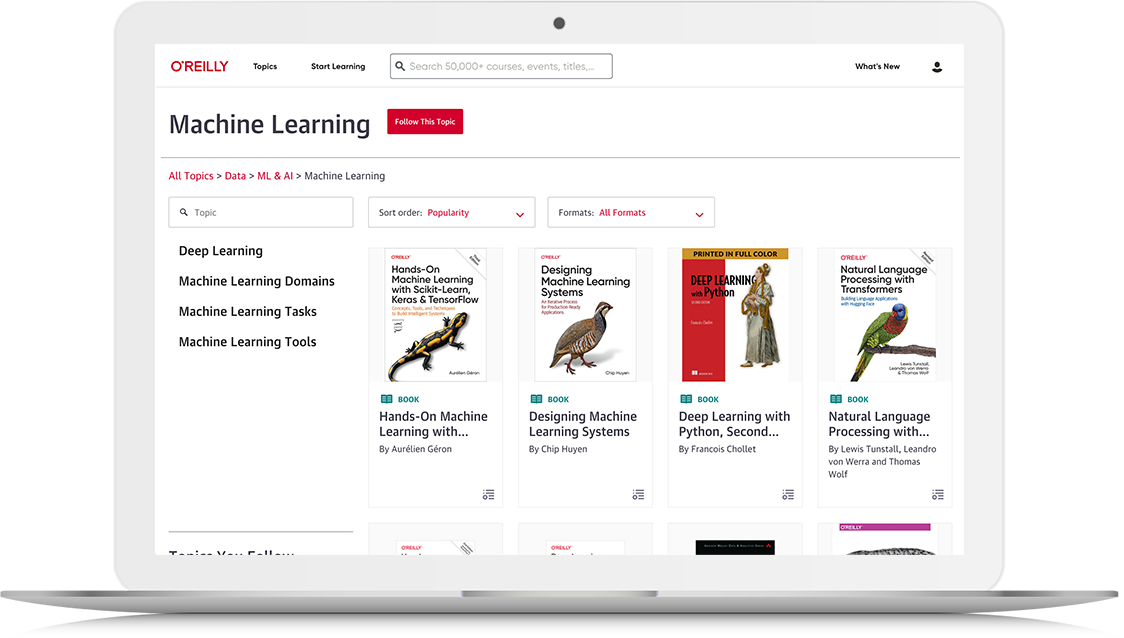
Allan Mangune
Illuminating the Presentation Layer: The Face of Your Application in Clean Architecture
In our ongoing series exploring Clean Architecture and its application within the .NET Core, C#, and ASP.NET ecosystem, we’ve progressively built up our understanding of the architectural layers that contribute to a robust, maintainable, and scalable software design. From laying the groundwork with the Domain Layer, orchestrating functionality in the Application Layer, to interfacing with external systems through the Infrastructure Layer, each piece plays a pivotal role in the architecture’s overall efficacy. Our exploration now brings us to the outermost layer—the Presentation Layer. This layer is where the application interacts with users, presenting data and gathering input, essentially serving as the face of your application.
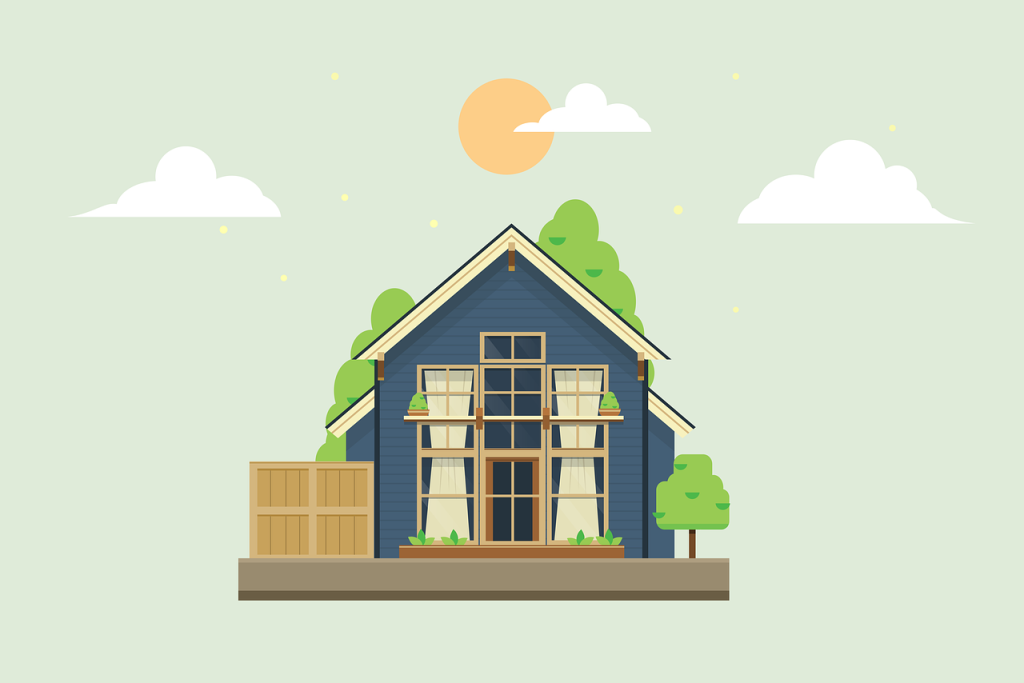
The Role of the Presentation Layer
The Presentation Layer is the user interface (UI) of your application. It’s the part of the software that users see and interact with. In the context of our house analogy, if the Domain Layer is the structure, the Application Layer the internal systems, and the Infrastructure Layer the utilities, then the Presentation Layer is the interior and exterior design of the house—the paint, the fixtures, and the furniture. It’s what makes the house not just livable but inviting and functional from a user’s perspective.
Components of the Presentation Layer
The Presentation Layer can include various components, depending on the application’s nature—web, desktop, mobile, or a combination thereof. In the world of .NET Core and ASP.NET, this often manifests as:
- Web Pages and Views : Using ASP.NET MVC or Razor Pages, developers can create dynamic web pages that render data from the application and accept user input.
- API Endpoints : For applications with a separate frontend (like those built with Angular, React, or Vue), ASP.NET Core Web API provides the means to expose data and functionality through HTTP APIs.
- User Input Validation : While core validation logic resides in the Domain Layer, the Presentation Layer handles initial user input validation, ensuring that data sent to the backend is in an expected format and meets basic criteria.
- Static Resources : This includes CSS, JavaScript, images, and other assets that contribute to the UI’s look and feel, enhancing user experience.
The Importance of the Presentation Layer
The Presentation Layer is critical for several reasons:
- User Experience : It’s the direct touchpoint for users, determining how they perceive and interact with your application. A well-designed UI can significantly enhance user satisfaction and adoption.
- Separation of Concerns : By isolating the UI from the rest of the application’s logic, developers can make changes to the presentation without affecting the underlying business logic or data access code, and vice versa.
Designing the Presentation Layer in ASP.NET
Creating an effective Presentation Layer in ASP.NET involves leveraging the framework’s robust features to build responsive, user-friendly interfaces. Some best practices include:
- Model-View-Controller (MVC) and Razor Pages : These ASP.NET Core features allow for a clean separation between the UI, data handling, and user input processing, facilitating a structured approach to web development.
- Responsive Design : Utilize CSS frameworks like Bootstrap or Foundation in conjunction with ASP.NET to create interfaces that work across various devices and screen sizes.
- Client-Side Technologies : While not part of ASP.NET per se, integrating client-side technologies like JavaScript or TypeScript enhances interactivity and user engagement.
Angular: Elevating the Presentation Layer
When it comes to building dynamic, responsive, and interactive web applications, Angular stands out as a powerful framework that can significantly elevate the Presentation Layer. Angular offers a comprehensive platform with tools and libraries designed to create complex web applications that are efficient and maintainable. Key features of Angular that benefit the Presentation Layer include:
- Component-Based Architecture : Angular’s architecture is built around reusable components, enabling developers to create encapsulated UI elements that can be reused throughout the application, promoting consistency and reducing redundancy.
- Two-Way Data Binding : Angular simplifies the synchronization between the UI and the application state, making it easier to ensure that the UI always represents the latest data without requiring excessive boilerplate code.
- Modularity and Lazy Loading : Angular applications can be organized into modules, making it possible to load features on demand. This enhances performance by reducing the initial load time and resources consumed by the application.
- TypeScript Support : Leveraging TypeScript, Angular provides a more structured approach to JavaScript development, with static typing, class-based object orientation, and compile-time error checking, leading to more robust and maintainable code.
- Rich Ecosystem : Angular is supported by a wide array of tools, libraries, and resources, including Angular Material for UI components and Angular CLI for project scaffolding and development tasks, streamlining the development process and enhancing productivity.
The Presentation Layer is where all the behind-the-scenes effort in building your application comes to fruition. It’s the interface through which all the application’s capabilities are accessed and judged by its users. In the Clean Architecture approach, particularly within the .NET Core and ASP.NET framework, understanding and effectively implementing the Presentation Layer is crucial for delivering applications that not only perform well but also offer engaging user experiences. As we continue our exploration of software architecture, the insights gained from each layer contribute to a holistic view of creating sophisticated and sustainable software solutions.
Image source: https://pixabay.com/illustrations/house-home-moon-cloud-object-tree-4414916/
Share this:
Published by allan mangune.
I hold the esteemed qualification of a Certified Public Accountant and have earned a Master's degree in Science with a specialization in Computer Information Systems. Since entering the realm of software development in 2000, my focus has been on adopting secure coding practices, an endeavour I have intensified after receiving my Certified Ethical Hacker v5 certification in 2008. My professional journey includes guiding clients through their digital transformation journey, particularly emphasizing digital security issues. For more than ten years, I have provided Agile Project Management training to well-known companies. I am a Certified ScrumMaster and have completed the Prince2 Agile Foundation certification. I had the privilege of being recognized as a Microsoft MVP for ASP.NET for ten consecutive years. Previously, I also served as a Microsoft Certified Trainer. As a hobby, I enjoy assembling personal unmanned aerial vehicles during my downtime. View more posts
Leave a comment Cancel reply
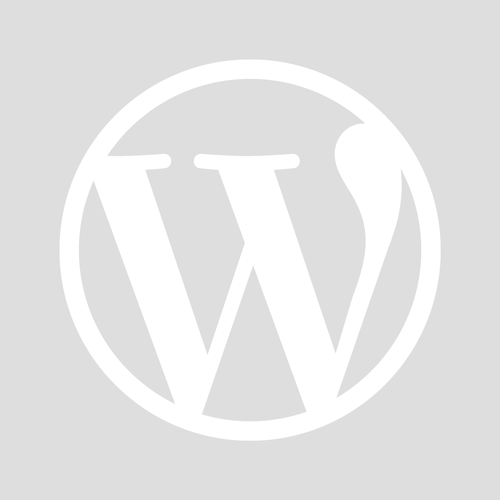
- Already have a WordPress.com account? Log in now.
- Subscribe Subscribed
- Copy shortlink
- Report this content
- View post in Reader
- Manage subscriptions
- Collapse this bar
- Engineering Mathematics
- Discrete Mathematics
- Operating System
- Computer Networks
- Digital Logic and Design
- C Programming
- Data Structures
- Theory of Computation
- Compiler Design
- Computer Org and Architecture
Presentation Layer in OSI model
Prerequisite : OSI Model
Introduction : Presentation Layer is the 6th layer in the Open System Interconnection (OSI) model. This layer is also known as Translation layer, as this layer serves as a data translator for the network. The data which this layer receives from the Application Layer is extracted and manipulated here as per the required format to transmit over the network. The main responsibility of this layer is to provide or define the data format and encryption. The presentation layer is also called as Syntax layer since it is responsible for maintaining the proper syntax of the data which it either receives or transmits to other layer(s).
Functions of Presentation Layer :
The presentation layer, being the 6th layer in the OSI model, performs several types of functions, which are described below-
- Presentation layer format and encrypts data to be sent across the network.
- This layer takes care that the data is sent in such a way that the receiver will understand the information (data) and will be able to use the data efficiently and effectively.
- This layer manages the abstract data structures and allows high-level data structures (example- banking records), which are to be defined or exchanged.
- This layer carries out the encryption at the transmitter and decryption at the receiver.
- This layer carries out data compression to reduce the bandwidth of the data to be transmitted (the primary goal of data compression is to reduce the number of bits which is to be transmitted).
- This layer is responsible for interoperability (ability of computers to exchange and make use of information) between encoding methods as different computers use different encoding methods.
- This layer basically deals with the presentation part of the data.
- Presentation layer, carries out the data compression (number of bits reduction while transmission), which in return improves the data throughput.
- This layer also deals with the issues of string representation.
- The presentation layer is also responsible for integrating all the formats into a standardized format for efficient and effective communication.
- This layer encodes the message from the user-dependent format to the common format and vice-versa for communication between dissimilar systems.
- This layer deals with the syntax and semantics of the messages.
- This layer also ensures that the messages which are to be presented to the upper as well as the lower layer should be standardized as well as in an accurate format too.
- Presentation layer is also responsible for translation, formatting, and delivery of information for processing or display.
- This layer also performs serialization (process of translating a data structure or an object into a format that can be stored or transmitted easily).
Features of Presentation Layer in the OSI model: Presentation layer, being the 6th layer in the OSI model, plays a vital role while communication is taking place between two devices in a network.
List of features which are provided by the presentation layer are:
- Presentation layer could apply certain sophisticated compression techniques, so fewer bytes of data are required to represent the information when it is sent over the network.
- If two or more devices are communicating over an encrypted connection, then this presentation layer is responsible for adding encryption on the sender’s end as well as the decoding the encryption on the receiver’s end so that it can represent the application layer with unencrypted, readable data.
- This layer formats and encrypts data to be sent over a network, providing freedom from compatibility problems.
- This presentation layer also negotiates the Transfer Syntax.
- This presentation layer is also responsible for compressing data it receives from the application layer before delivering it to the session layer (which is the 5th layer in the OSI model) and thus improves the speed as well as the efficiency of communication by minimizing the amount of the data to be transferred.
Working of Presentation Layer in the OSI model : Presentation layer in the OSI model, as a translator, converts the data sent by the application layer of the transmitting node into an acceptable and compatible data format based on the applicable network protocol and architecture. Upon arrival at the receiving computer, the presentation layer translates data into an acceptable format usable by the application layer. Basically, in other words, this layer takes care of any issues occurring when transmitted data must be viewed in a format different from the original format. Being the functional part of the OSI mode, the presentation layer performs a multitude (large number of) data conversion algorithms and character translation functions. Mainly, this layer is responsible for managing two network characteristics: protocol (set of rules) and architecture.
Presentation Layer Protocols : Presentation layer being the 6th layer, but the most important layer in the OSI model performs several types of functionalities, which makes sure that data which is being transferred or received should be accurate or clear to all the devices which are there in a closed network. Presentation Layer, for performing translations or other specified functions, needs to use certain protocols which are defined below –
- Apple Filing Protocol (AFP): Apple Filing Protocol is the proprietary network protocol (communications protocol) that offers services to macOS or the classic macOS. This is basically the network file control protocol specifically designed for Mac-based platforms.
- Lightweight Presentation Protocol (LPP): Lightweight Presentation Protocol is that protocol which is used to provide ISO presentation services on the top of TCP/IP based protocol stacks.
- NetWare Core Protocol (NCP): NetWare Core Protocol is the network protocol which is used to access file, print, directory, clock synchronization, messaging, remote command execution and other network service functions.
- Network Data Representation (NDR): Network Data Representation is basically the implementation of the presentation layer in the OSI model, which provides or defines various primitive data types, constructed data types and also several types of data representations.
- External Data Representation (XDR): External Data Representation (XDR) is the standard for the description and encoding of data. It is useful for transferring data between computer architectures and has been used to communicate data between very diverse machines. Converting from local representation to XDR is called encoding, whereas converting XDR into local representation is called decoding.
- Secure Socket Layer (SSL): The Secure Socket Layer protocol provides security to the data that is being transferred between the web browser and the server. SSL encrypts the link between a web server and a browser, which ensures that all data passed between them remains private and free from attacks.
Please Login to comment...
Similar reads.
- How to Get a Free SSL Certificate
- Best SSL Certificates Provider in India
- Elon Musk's xAI releases Grok-2 AI assistant
- What is OpenAI SearchGPT? How it works and How to Get it?
- Content Improvement League 2024: From Good To A Great Article
Improve your Coding Skills with Practice

What kind of Experience do you want to share?
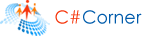
- TECHNOLOGIES
- An Interview Question
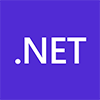
Web API 3 Layers Architecture With .NET 6

- Thiago Vivas
- Nov 09, 2022
- Other Artcile
This article will explain one of the most used software architectural patterns, the 3 layers architecture. Besides the theoretical explanation of the 3 layers architecture, it will also be given a practical example using .NET 6 in a Web API Project.
What is a 3-Layered Architecture?
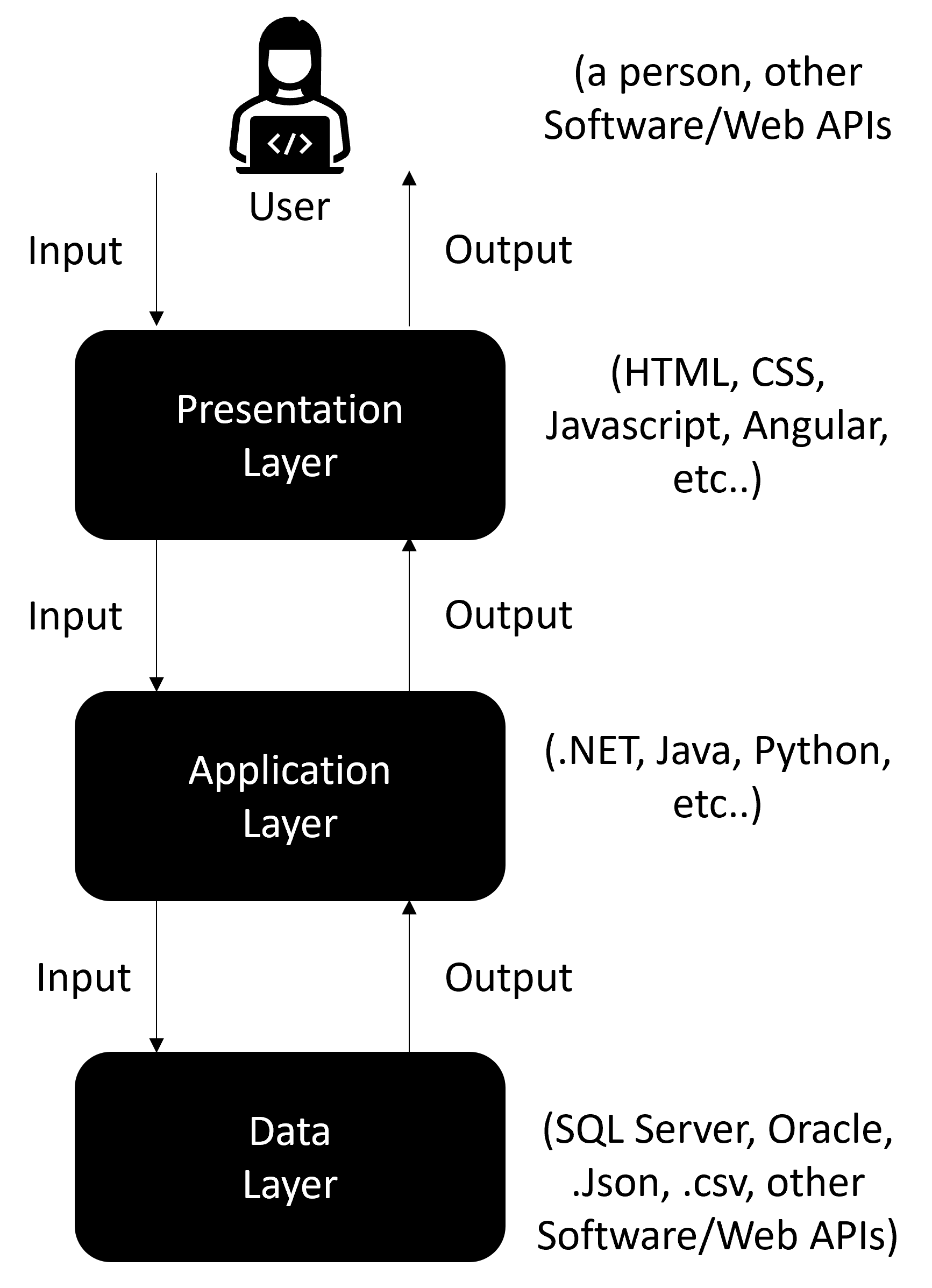
The 3 layers architecture was created based on the n-tiered patterns, it groups its main software development components in three different horizontal layers. Based on your software application type you may find yourself with the need to have more than 3 organizational layers, but that is not a problem as far as you may have those specific layers as sub-layers of one of those main layers.
The 3-layered architecture can be applied in any project type or size, and how you are organizing each layer inside your project does not matter. What matters is to have those layers visible and grouped, each layer has to be easily identifiable. In some projects, we have each layer on a separate and independent project, which facilitates parallel development. And in other projects, we have all the 3 layers sharing the same project.
Each layer is responsible to validate its input data and provide a meaningful response.
The first layer is the presentation layer or the interface layer. This layer is responsible for making the bridge between the user and our application, its responsibility is to receive and validate user input and to provide a meaningful response.
The second layer is the application layer, business logic layer, or middle layer. This layer is responsible to apply business logic and transform the input data to our database, also this layer should transport the response from the database to the first layer.
The third layer is the data-access layer, or data layer, back-end layer, or database layer. This layer is responsible for manipulating and managing data, no matter where this data comes from. The data source could be a database, a file, or another software application.
The most important part of any design pattern is to have its pattern explicit, and easy to understand, resulting in increased maintainability. With the 3-layered architecture pattern, it is very important to have each layer's role understood and its role limits well-stabilized.
Some of the most common ways to organize each layer:
- Naming, using its layer's suffix.
- Separated projects.
- Folders with their layer's name on them.
Benefits of using 3-Layered Architecture
As this is an architectural design pattern, the biggest benefit will be well-organized architecture. Being easier for a Software Development team to work together on it and give maintenance, but the main benefits are listed below:
- Loosely coupled, being easier to unit test or replacing an entire layer.
- Unit testing, make use of mocks to test the smallest units of your project.
- Maintainability, easier to understand where the changes in the code have to be made.
- Scalability, with any tier being called independent from the others.
- Reliability, if one layer fails will not impact the others.
- Security, as the presentation layer, does not communicate directly with the data layer.
3 Layers Architecture Implementation Step by Step in .NET 6
The first layer, the presentation layer.
The first layer is responsible to interact with our end user, and should not have any business logic validated here. Must be as simple as possible.
Nugets packages to install:
- System.Linq
Project configuration & Dependency Injection
The controllers
The second layer, the Application Layer
Here you place your business validation and logic.
- AutoMapper.Extensions.Microsoft.DependencyInjection
AutoMapper Profiles
DTO classes
Managers Interfaces
Managers classes
The third layer, the Data Layer
The third layer is responsible to connect to our database, we will be using Entity Framework as the object-database mapper. If you do not know how to set up your database access you can check my previous article explaining about Entity Framework Database first approach, as follows:
- Entity Framework Core 6 With Database First
- EntityFramework
- Microsoft.EntityFrameworkCore.Design
- Microsoft.EntityFrameworkCore.SqlServer
- Microsoft.EntityFrameworkCore.Tools
The Repository Interfaces
The Repository Classes
Congratulations, you have successfully created a Web API project using the famous 3-layered architecture.
Project on GitHub .
External References
- Microsoft documentation .
- architecture
- entity framework
- entity framework core
- layered architecture
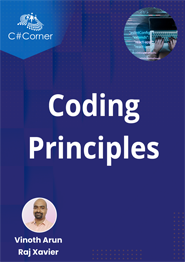
Coding Principles
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Presentation VS Application layer in DDD
I have trouble drawing a clear line between Presentation and Application layer in Domain Driven Design.
Where should Controllers, Views, Layouts, Javascript and CSS files go?
Is it in the Application or the Presentation layer?
And if they go all together in the same layer, what contains the other one? Is it empty?
- architecture
- domain-driven-design
3 Answers 3
Just because someone created and named "Application Layer" and "Presentation Layer" doesn't mean your application should have them. You should create layers AFTER you created substantial amount of code which you grouped together and want to name this group for sake of communication between developers and clarity of code.
From point of DDD. Application Layer is everything that is not Domain layer. Which includes application logic, presentation and application services.
- 3 Thank you, indeed you made me realize that for my case separating Application and Presentation is useless. Simplicity first! – Matthieu Napoli Commented Dec 24, 2012 at 9:43
- If DDD has REST API instead of UI in presentation layer, would REST API be an application or presentation layer. I am now confused, since I was sure that REST API is a presentation layer.. – Dario Granich Commented May 6, 2016 at 13:33
- 15 Actually, DDD prescribes four layers in the following order, from higher to lower: Presentation, Application, Domain, Infrastructure. So, the Application layer does not include "presentation". Also, it's always a good idea to decide on the layers before a significant amount of code is written, as it isn't only about grouping code together but also about constraining the direction of compile-time dependencies. – Rogério Commented Feb 21, 2017 at 17:36
There is a big difference between the application layer and the presentation layer from a DDD view point.
Although DDD centers around how to model the domain using the DDD building blocks and concepts such as bounded contexts, Ubiquitous language and so, it is still vital to clearly identify and separate the various layers in your app.
The architecture plays a big role in implementing a successful DDD app. A famous architecture that gained a lot of hype lately is the onion architecture:

In this design the UI/Presentation layer and the application layer are clearly separated. Merging the 2 together introduces tight coupling between 2 layers that have clear separate concerns and responsibilities.
The Presentation layer should only house presentation logic. Avoid Smart UIs that know too much. This mainly houses the MVC's Controllers and views in addition to CSS, JS, templates, forms and everything that relates to response and request objects.
The actions issued through presentation are delegated to the application layer through commands. The application layer contains the application logic. It normally maps to a use case. It contains WHAT the system should do to satisfy a use case. A typical application service will ask a repository to return an aggregate then invoke an action on that aggregate.
Have a look at the sample project from Vaughn Vernon's IDDD
- 2 +1. This is how i've implemented my project. Immediately, i was able to make gains by doing so. Since I abstracted to an application layer, I was able to have multiple presentation layers. For example, our web api and our web site both consume the application layer which saved a lot of time and duplicated code since my web app doesn't have to frame messaging to and from the web api and it keeps all of the logic in sync between the two. – Sinaesthetic Commented Jan 21, 2016 at 1:47
- Where are entry point and composition root placed? I always thought it was a responsibility of Application layer. But now it looks like this is Presentation layer. – Denis535 Commented Feb 24, 2020 at 22:00
Domain Driven Design has nothing to do with either Presentation layer or Application layer. DDD is a methodology whose main focus is on the Domain layer. That is, DDD does not impose any constraints regarding any other layer except for the Domain layer and Your question as well could be asked in the context of any other methodology.
That being said, it's very common to use a four-layer architecture for DDD applications. Here's an example of one such application showing the layers and their intended use: DDDSample Architecture . So, if you choose to use this architecture your views and layouts would go to the Interfaces layer and the controllers, if interface-independent, would go to the Application layer.
You might as well choose any other kind of architecture, as I've said DDD does not impose constraints. There are many MVC frameworks out there that have different structures and yet could also be used for DDD applications. Then, of course, you would place Your views and layouts accordingly.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Software Engineering Stack Exchange. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged architecture domain-driven-design layers or ask your own question .
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
Hot Network Questions
- Worth replacing greenboard for shower wall
- What is the meaning of "against" in "against such things there is no law" Galatians 5:23?
- How much payload could the Falcon 9 send to geostationary orbit?
- What is an intuitive way to rename a column in a Dataset?
- Reusing own code at work without losing licence
- about flag changes in 16-bit calculations on the MC6800
- Is it advisable to contact faculty members at U.S. universities prior to submitting a PhD application?
- Why are complex coordinates outlawed in physics?
- Stuck on Sokoban
- Using Thin Lens Equation to find how far 1972 Blue Marble photo was taken
- If inflation/cost of living is such a complex difficult problem, then why has the price of drugs been absoultly perfectly stable my whole life?
- How to remove obligation to run as administrator in Windows?
- I'm trying to remember a novel about an asteroid threatening to destroy the earth. I remember seeing the phrase "SHIVA IS COMING" on the cover
- Integral concerning the floor function
- Can probabilistic predictions be obtained from gradient boosting models CatBoost and XGBoost?
- Why is PUT Request not allowed by default in OWASP CoreRuleSet
- Command-line script that strips out all comments in given source files
- What is the name of this simulator
- How can I prove the existence of multiplicative inverses for the complex number system
- Do metal objects attract lightning?
- Why does a halfing's racial trait lucky specify you must use the next roll?
- Dress code for examiner in UK PhD viva
- Are carbon fiber parts riveted, screwed or bolted?
- Too many \setmathfont leads to "Too many symbol fonts declared" error
Difference between Layers and Tiers
In this article, I am going to discuss one of the most frequently asked interview questions i.e. what is the difference between layers and tiers? This question can also be asked in many different ways like
The above three layers reside in their own projects, maybe in 3 different projects or even more. When we compile the projects we get the respective layer DLL. So we have 3 DLL’s now.
So, in short, we can define the Layers are the logical separation and the Tiers are the physical separation of an application. We can also say that tiers are the physical deployment of layers .
The architecture of a typical n layer and n-tier Application:
Responsibilities of each layer or tier in an application:, advantages of a layered architecture:.
Layered architecture increases flexibility, maintainability, and scalability. In Layered architecture, we separate the user interface (i.e. presentation layer) from the business logic and the business logic from the data access logic layer. Separation of concerns among these logical layers and components is easily achieved with the help of layered architecture.
The layered architecture enables teams to work on different parts of the application parallel with minimal dependencies on other teams. For example, some developers may work on the presentation layer while some other developers may work on the business logic layer at the same time some developers might work on the data access layer as well.
Different components of the application can be independently deployed, maintained, and updated, on different time schedules.
Disadvantages of a Layered Architecture:
There might be a negative impact on the performance as we have the extra overhead of passing through layers instead of calling a component directly.
In this article, I try to explain the difference between layers and tiers in an application . I hope you enjoy this article and understand the difference between Layers and Tiers in an application.
- Tìm việc IT
- Tạo CV Online
- CÔNG TY TUYỂN DỤNG IT
- AI – Machine Learning
- Xu hướng công nghệ
- Báo cáo thị trường IT
- Con đường sự nghiệp IT
- Chuyên gia nói
- Sách lập trình
- Tài liệu lập trình
- Source Code
- Kinh nghiệm phỏng vấn
- Chuẩn hóa CV IT
- Đàm phán lương thưởng
- Tìm kiếm nhân tài
- Nghệ thuật quản trị
- 028.6273.3496
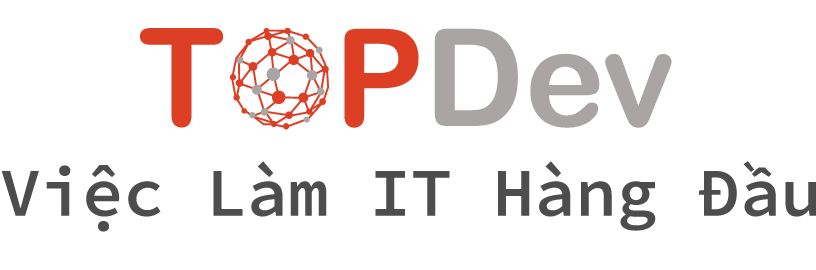
Mô hình 3 lớp (three-layer) có gì hay?
“Biết địch biết ta, trăm trận trăm thắng “. Vậy muốn biết có gì hay thì phải hiểu rõ về nó. Được rồi, nhân dịp đang học nhập môn công nghệ phần mềm ở trường, kiến thức còn nóng hổi nên mình sẽ phân tích về mô hình 3 lớp (3-layer).
Trước tiên, cần phân biệt 2 khái niệm tầng (tier) và lớp (layer)
3 lớp (three-layer) là gì?
3-tiers là một kiến trúc kiểu client/server mà trong đó giao diện người dùng (UI-user interface), các quy tắc xử lý (BR-business rule hay BL-business logic), và việc lưu trữ dữ liệu được phát triển như những module độc lập, và hầu hết là được duy trì trên các nền tảng độc lập, và mô hình 3 tầng (3-tiers) được coi là một kiến trúc phần mềm và là một mẫu thiết kế.” (dịch lại từ wikipedia tiếng Anh).
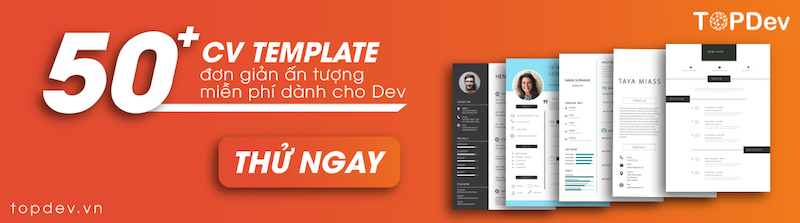
Đây là kiến trúc triển khai ứng dụng ở mức vật lý. Kiến trúc gồm 3 module chính và riêng biệt:
- Tầng Presentation: hiển thị các thành phần giao diện để tương tác với người dùng như tiếp nhận thông tin, thông báo lỗi, …
- Tầng Business Logic: thực hiện các hành động nghiệp vụ của phần mềm như tính toán, đánh giá tính hợp lệ của thông tin, … Tầng này còn di chuyển, xử lí thông tin giữa 2 tầng trên dưới.
- Tầng Data: nơi lưu trữ và trích xuất dữ liệu từ các hệ quản trị CSDL hay các file trong hệ thống. Cho phép tầng Business logic thực hiện các truy vấn dữ liệu .
Mọi người vẫn hay nhầm lẫn giữa tier và layer vì cấu trúc phân chia giống nhau (presentation, bussiness , data). Tuy nhiên, thực tế chúng hoàn toàn khác nhau. Nếu 3 tiers có tính vật lí thì 3 layer có tính logic. Nghĩa là ta phân chia ứng dụng thành các phần (các lớp) theo chức năng hoặc vai trò một cách logic. Các layer khác nhau được thực thi trong 1 phân vùng bộ nhớ của process. Vì thế nên một tier có thể có nhiều layer.
TÌM VIỆC LÀM NGÀNH IT LƯƠNG CAO
Giới thiệu mô hình 3-layer ( 3 lớp)
Mô hình 3-layer gồm có 3 phần chính:
– Presentation Layer (GUI) : Lớp này có nhiệm vụ chính giao tiếp với người dùng. Nó gồm các thành phần giao diện ( win form, web form,…) và thực hiện các công việc như nhập liệu, hiển thị dữ liêu, kiểm tra tính đúng đắn dữ liệu trước khi gọi lớp Business Logic Layer (BLL).
– Business Logic Layer (BLL) : Layer này phân ra 2 thành nhiệm vụ :
- Đây là nơi đáp ứng các yêu cầu thao tác dữ liệu của GUI layer, xử lý chính nguồn dữ liệu từ Presentation Layer trước khi truyền xuống Data Access Layer và lưu xuống hệ quản trị CSDL.
- Đây còn là nơi kiểm tra các ràng buộc, tính toàn vẹn và hợp lệ dữ liệu, thực hiện tính toán và xử lý các yêu cầu nghiệp vụ, trước khi trả kết quả về Presentation Layer.
– Data Access Layer (DAL) : Lớp này có chức năng giao tiếp với hệ quản trị CSDL như thực hiện các công việc liên quan đến lưu trữ và truy vấn dữ liệu ( tìm kiếm, thêm, xóa, sửa,…).
- Việc phân chia thành từng lớp giúp cho code được tường minh hơn. Nhờ vào việc chia ra từng lớp đảm nhận các chức năng khác nhau và riêng biệt như giao diện, xử lý, truy vấn thay vì để tất cả lại một chỗ. Nhằm giảm sự kết dính.
- Dễ bảo trì khi được phân chia, thì một thành phần của hệ thống sẽ dễ thay đổi. Việc thay đổi này có thể được cô lập trong 1 lớp, hoặc ảnh hưởng đến lớp gần nhất mà không ảnh hưởng đến cả chương trình.
- Dễ phát triển, tái sử dụng: khi chúng ta muốn thêm một chức năng nào đó thì việc lập trình theo một mô hình sẽ dễ dàng hơn vì chúng ta đã có chuẩn để tuân theo. Và việc sử dụng lại khi có sự thay đổi giữa hai môi trường ( Winform sang Webfrom ) thì chỉ việc thay đổi lại lớp GUI.
- Dễ bàn giao. Nếu mọi người đều theo một quy chuẩn đã được định sẵn, thì công việc bàn giao, tương tác với nhau sẽ dễ dàng hơn và tiết kiệm được nhiều thời gian.
- Dễ phân phối khối lượng công việc. Mỗi một nhóm, một bộ phận sẽ nhận một nhiệm vụ trong mô hình 3 lớp. Việc phân chia rõ ràng như thế sẽ giúp các lập trình viên kiểm soát được khối lượng công việc của mình.
Phân tích chi tiết từng layer trong mô hình 3 lớp.
1. Presentation Layer (GUI):
Có hai thành phần chính sau đây với những tác vụ cụ thể :
- UI Components : gồm các thành phần tạo nên giao diện của ứng dụng (GUI). Chúng chịu trách nhiệm thu nhận và hiển thị dữ liệu cho người dùng… Ví dụ : textbox, button, combobox, …
- UI Process Components : là thành phần chịu trách nhiệm quản lý các quá trình chuyển đổi giữa các UI… Ví dụ : Sắp xếp quá trình kiểm tra thông tin khách hàng:
1.Hiển thị màn hình tra cứu ID
2.Hiển thị màn hình thông tin chi tiết khách hàng tương ứng
3.Hiển thị màn hình liên lạc với khách hàng.
2. Bussiness Layer (BLL) :
Lớp này gồm 4 thành phần:
- Service Interface : là thành phần giao diện lập trình mà lớp này cung cấp cho lớp Presentation sử dụng.
- Bussiness Workflows : chịu trách nhiệm xác định và điều phối các quy trình nghiệp vụ gồm nhiều bước và kéo dài. Những quy trình này phải được sắp xếp và thực hiện theo một thứ tự chính xác.
- Ví dụ : Thực hiện mua một đơn hàng trên tiki qua nhiều bước : kiểm tra gói hàng còn không?, tính tổng chi phí, cho phép giao dịch và sắp xếp việc giao hàng.
- Bussiness Components : chịu trách nhiệm kiểm tra các quy tắc nghiệp vụ, ràng buộc logic và thực hiện các công việc . Các thành phần này cũng thực hiện các dịch vụ mà Service Interface cung cấp và Business Workflows sẽ sử dụng nó.
- Ví dụ : Tiếp tục ví dụ ở trên. Bạn sẽ cần một Bussiness Component để kiểm tra gói hàng có khả dụng không ? hay một component để tính tổng chi phí,…
- Bussiness Entities : thường được sử dụng như Data Transfer Objects ( DTO ) . Bạn có thể sử dụng để truyền dữ liệu giữa các lớp (Presentation và Data Layer). Chúng thường là cấu trúc dữ liệu ( DataSets, XML,… ) hay các lớp đối tượng đã được tùy chỉnh.
- Ví dụ : tạo 1 class Student lưu trữ các dữ liệu về tên, ngày sinh, ID, lớp.
3. Data Layer (DAL) :
- Data Access Logic Components : chịu trách nhiệm chính lưu trữ và truy xuất dữ liệu từ các nguồn dữ liệu ( Data Sources ) như XML, file system,… Hơn nữa còn tạo thuận lợi cho việc dễ cấu hình và bảo trì.
- Service Agents: giúp bạn gọi và tương tác với các dịch vụ từ bên ngoài một cách dễ dàng và đơn giản.
Cấu trúc mô hình 3 lớp
Để hiểu rõ hơn về cấu trúc và cách xây dựng của mô hình 3 lớp, chúng ta cùng tham khảo một ví dụ về mô hình quản lí công nhân g ồm các lớp BUS, DAO, GUI. (Các đoạn code sẽ bị lược bỏ bớt )
Đầu tiên là GUI gồm các button insert, update, reset ,delete ,exit .Người dùng sẽ giao tiếp với màn hình giao diện này
Lớp DTO, đây không phải là layer, đây chỉ là 1 gói dữ liệu đươc trao đổi giữa các lớp. Gói dữ liệu này được xây dựng dưới dạng lớp đối tượng. Mỗi một công nhân sẽ mang những thuộc tính sau:
Các nghiệp vụ xử lý chính sẽ được đặt ở lớp BUS (hay là BLL) gồm các nghiệp vụ insert, update, delete, retrieve
Và cuối cùng là lớp DAO ( hay là DAL ). Truy vấn đến cơ sở dữ liệu
Vì đây là ví dụ mô phỏng nên tôi chỉ chú trọng đến cách cấu trúc một chương trình sử dụng mô hình 3 lớp. Cảm ơn đã đọc hết.
Đừng bỏ lỡ những bài viết hay về lập trình hướng đối tượng:
- Mô hình quan hệ – thực thể (Entity – Relationship Model) là gì?
- Lập trình hướng chức năng đang thống trị mảng UI với Pure Views
- Cấu trúc 3 lớp trong C# .Net
Xem thêm việc làm Web Developer mới nhất tại TopDev
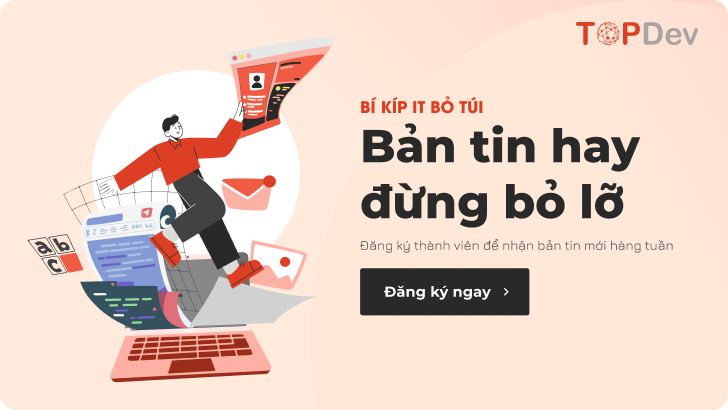
RELATED ARTICLES MORE FROM AUTHOR
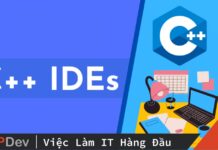
IDE C++ là gì? Tiêu chí lựa chọn IDE cho lập trình C++
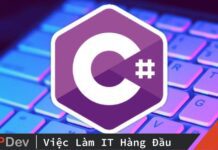
5 lỗi sai cơ bản thường gặp trong C#
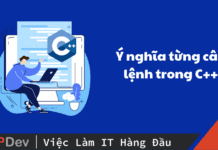
Ý nghĩa từng câu lệnh trong C++, giải thích đơn giản, dễ hiểu
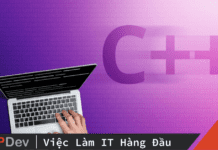
C++ Developer là gì? Cách học lập trình C++ hiệu quả
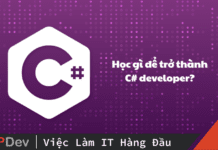
Học gì để trở thành C# developer? Những kiến thức quan trọng
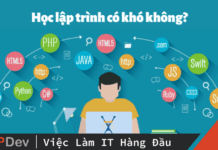
Học lập trình có khó không? Ngôn ngữ lập trình nào “dễ học”
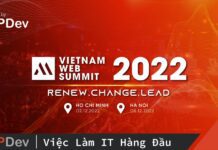
Vietnam Web Summit 2022: Serverless Architecture, Web3 và Data-driven – Bệ phóng đưa ngành Công nghệ bứt phá trong kỷ nguyên mới
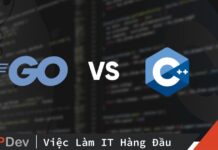
So sánh giữa C++ và Golang
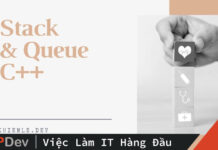
Ngăn xếp (stack) và hàng đợi (queue) trong C++
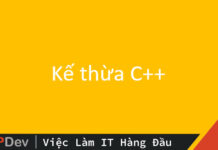
Kế thừa trong C++
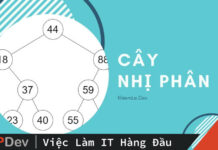
Cây nhị phân trong C++
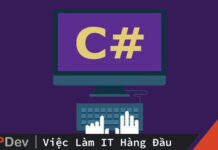
Dự án anh hóa – ngôn ngữ C#
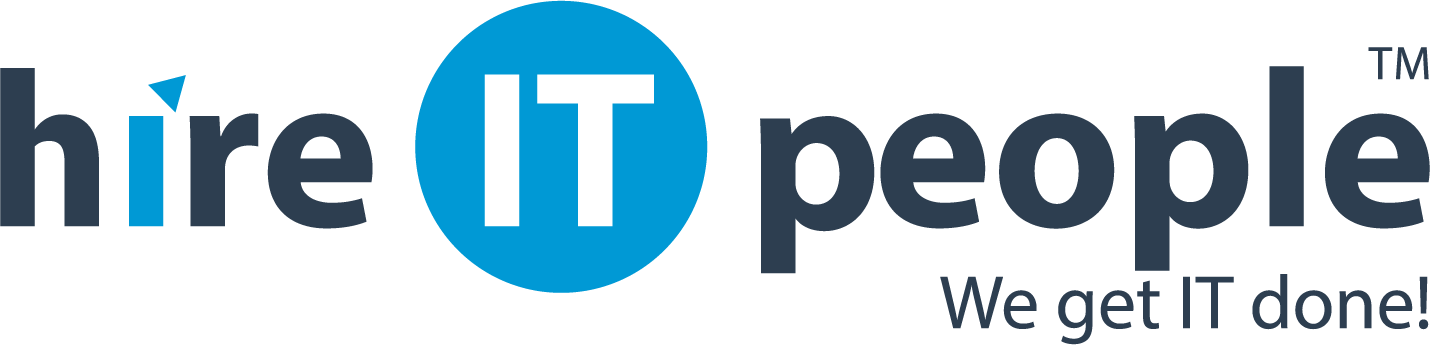
We provide IT Staff Augmentation Services!
Senior developer resume, cupertino, ca.
- 9+ years of experience in the System Analysis, Design and implementation in Client/Server Environment and Web based Internet/Intranet Application environments using Java and Web related technologies.
- 6+ years of experience in Java applications coding, developing, testing and documenting the requirements.
- Expertise in severalJ2EE TechnologieslikeJSP, Servlets, Web Services with Soap and Rest APIs in different projects.
- Expertise in writing Queries, Procedures, Functionsand performeddata analysisusingSQL server and Oracle.
- Expertise in creating Conceptual Data Models, Process/Data Flow Diagrams,Use Case Diagrams, Class Diagrams and State Diagrams.
- Experience working withcore JavaandJava Multi - Threadingapplication
- Excellent experience withDatabasessuch asMySQL, Oracle 10g/11g and SQL Server 2005
- Proficiency inSystem Life Cycleincluding developing and testing the applications.
- Experience inMVC (Model View Controller)architecture, usingStruts, AJAX and Spring Frameworkwith variousJava/J2EE design patterns.
- Proficient inJava/J2EEtechnologies likeJSP, Hibernate, Spring, Java Servlets, AJAX, XML, web service usingIDEs likeEclipse 3.1/3.5.
- Experience usingAgileandExtreme Programmingmethodologies.
- Conversant with Web/application Servers-Jboss, Tomcat 5.5/6.0/7.0.
- Extensively worked ondebuggingusing Eclipse debugger.
- Efficient team member with excellent work/time management skills, excellent leadership, communications and Interpersonal Skills, ability to work under strict deadlines.
- Project co-ordinationand management skills.
TECHNICAL SKILLS
Design Patterns: DAO, Factory Pattern, MVC, Singleton.
Technologies: J2EE, JDBC, JSP, Servlets, JSTL, .Net, AJAX
SOA: Web Services, RestFull web services.
Databases: Sql-Server, MS-Access, Oracle, Postgre-Sql.
Operating Systems: MAC OSX 10.8.3, Windows Server 2003 Windows 9x/2000/2003/XP/Vista
Servers: Apache Tomcat, Web logic, Jboss, IIS.
Languages: Java, J2EE, PL/SQL, SQL, C#, VB.net
Framework: Spring, Struts, Hibernate, MVC Architecture.
IDEs: Eclipse Indigo, Eclipse Juno, intellij
Source Control: Maven, ANT, JUnit, Log4j, VSS
PROFESSIONAL EXPERIENCE
Confidential, Cupertino, CA
Senior Developer
Responsibilities:
- Designed and developed screens usingEclipse, HTML, JSP, Servlets and Java Script.
- Worked with project teams in developing various sales applications.
- Extensively worked on springs and set their dependencies in a spring context file.
- Experience in using IDEs Eclipse Juno, Indigo etc. for Application development.
- Very Strong knowledge in using J2EE based App Servers as JBOSS.
- Wrote JavaScript to validate the reports.
- Worked on most of the core Java concepts.
- Designed/developed UI with HTML, DHTMLand JavaScript for interactive web pages.
- Worked on backend databases and integrated withHibernateto retrieve Data Access Objects.
- Designed and prepared Unit test case usingJUnit and removed the maximum bugs before passing on the application to the QA team.
- Wrote build and deployed scripts using Maven.
- Developed and deployed the restful web services.
- Worked in multiple projects and responsible for core technical support of componentsin multiple environments.
- Responsible for technical design, development, coding, testing, debugging and documentation of programming applications to meet project requirements.
- Developed and implemented the User Interfaces using Java Server Pages (JSP)
- Front-end validations are done using JavaScript.
- Extensively worked on core java.
- Session Management was implemented in Hibernate and JVM is handled.
- Association and Lazy loading was implemented in Hibernate
- Follow established enterprise development and design standards, guidelines and processes.
- Identify / troubleshoot and resolve application code issues identified during test cycles.
- Interact with IT management and other teams at a highly professional and technical level.
- Demonstrate accurate and complete understanding of system functionality and technical components.
Environment: Java, J2EE, J-Query, Spring-Framework, Spring-MVC, HTML, JavaScript, JSP, DHTML, AJAX, Jboss, XML, XSL, XSLT, XSD, JAX-RS/REST, Eclipse IDE, Corda, Teradata, Pages, Keynote, Omni graph, SQL workbench.
Confidential, Sunnyvale, CA
- Used hibernate to query from database. Developed web pages and validated these pages using JSP, HTML, CSS, Jscript, and JavaScript.
- Worked in Struts with Hibernate using MVC design Pattern.
- Created Different validators and registered with config file.
- Developed and implemented the User Interfaces using Java Server Pages (JSP).
- Created stored procedure in Oracle 9g and called from application using callable statements.
- Created stored procedures, triggers and functions in SQl server.
- Worked on Web Service development for dynamic interaction between web applications.
- Wrote and implemented test plans using JUnit.
- Involved in Code Review, Unit testing and Integration testing for Quality Assurance.
- Performed Unit Testing, Functional Testing and Integration Testing.
- Developed the Cascading Style Sheets (CSS), which were used for the entire site giving the site a dynamic look.
- Conceptualized, designed, developed, and implemented the look and feel of web pages that are visually pleasing, load quickly, and meet established design standards.
- Provided competitive market analysis on the e - commerce site and proposed suggestions to the design and available functionality.
- Gathered requirements from users and translated in to system requirements and specification.
- Developed java classes as per the requirement.
- Created UML Diagrams like Class Diagrams, Sequence Diagrams, and Activity Diagrams Using Rational Rose and Microsoft Visio.
Environment: Java, J2EE, JSP, Servlet, Sql Server, UML, Hibernate, Javascript.HTML, DHTML, TOMCAT and Eclipse.
Confidential, Warren, NJ
Portal Developer
- Requirements gathering, design, development and testing of features and enhancements.
- Providing guidance for developers on how to use the framework and associated services to build the application.
- Continual enhancement and maintenance and bug fixes for existing features.
- Occasional demonstration of the framework and features to interested teams across Team.
- Worked independently on self-registration, quick access, bulletin alerts & feeds modules etc.
- Extensive programming in core Java.
- Designed the CSS and UI interface for the web pages.
- Created/ Managed various tickets in Team Track for code deployment in PRODUCTION and UAT environment
- Involved in designing and coding of Presentation Layer, Business Logic Layer and Data Access Layer.
- Connected with search engine to search in specific directories or subfolders.
- Developed database access service layer for the soap Web Services and integrated modules.
- Extensively used OOPS java concepts.
- Wrote Hibernate POJO classes and corresponding mapping files, which maps bean properties to the table columns in database.
- Created template-based and original designs for intranet sites.
Environment: Java, JDBC, J2EE (Servlets/JSP), Strut, JSF Java, Eclipse, TOMCAT, HTML, CSS, XML, CVS, Hibernate, Ajax, WebServices (SOAP/WSDL), Oracle 10g, JavaScript, CSS, TOAD.
Confidential
UI/Web Application Developer
- Involved in Design and Documentation of Individual Applications Screen Specifications for ZDI
- Coded various JavaScript and created Asynchronous JavaScript & XML using AJAX.
- Coding stored procedures, functions & cursors in the SQL-Server 2000.
- Designed Publication Workflow & updated in DB of various modules.
- Created classes, interfaces, properties, methods, user controls in C# using ASP.NET.
- Responsible in implementing validation using ASP.NET validation controls.
- Improving Software Quality through Effective Source Code Control using Microsoft Visual Source Safe and SourceGear Vault 1.0.
- Code reusability done using Code Smith- 31 by creating corresponding templates.
- Used Re-sharper 1.5.1 tool for code review.
- Extensively used Dataset, Data Grid and Repeater controls to display and manipulate data.
- XSLT style sheet files were created for transforming results from XML output.
- Quick Base is used as requirement tracking tool.
Environment: Visual Studio.NET 2003, C# 1.1, Asp.net 1.1, ADO. Net 1.1, MS .NET Framework 1.1, Vignette Version 7, HTML/DHTML, CSS, SQL Server-2000, AJAX, JavaScript, IS, Web Forms, User Controls, XML, XSL, SourceGear Vault 1.0, Re-sharper 1.5.1, Code Smith-31, VSS, MS-Visio 2003, Quick Base, Web Service.
Software Developer
- Requirement analysis and design based on n-tier architecture.
- Analyzed the existing PowerBuilder application functionality and redesigned the application framework into the .net.
- Involved in the development of ARM framework using Infragistics controls and VB.net.
- Involved in the analysis, design, coding, bug fixing, bug tracking and unit testing of framework and modules.
- Extensively used assemblies implemented using VB.net.
- Used Delegates and Events to create the ARM application’s events using VB.net.
- Created Crystal Reports using windows forms.
- Used ADO.NET for data access layer to communicate with the Oracle 9i database using ODBC provider
- Used Infragistics controls for UI, Charts and Events using Winforms.
- Coded Sql Queries using Joins, Stored Procedures, Functions, and Triggers & Cursors in the Oracle 9i.
- Toad tool used for database operations.
- Involved in Unit testing of various modules.
- Involved in writing test cases for various modules.
- Involved in domain study & workflow analysis between various modules of the software.
Environment: Visual Studio .NET 2003, VB.NET, ADO.NET, XML, Oracle 9i, PowerBuilder 7.0, Infragistics Net Advantage 2005 Volume 3, Winforms PVCS-Repository Tool, Re-sharper, Rational Rose, Toad, MS Front Page
We'd love your feedback!
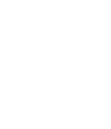
Resume Categories
- .NET Developers/Architects Resumes
- Java Developers/Architects Resumes
- Informatica Developers/Architects Resumes
- Business Analyst (BA) Resumes
- Quality Assurance (QA) Resumes
- Network and Systems Administrators Resumes
- Help Desk and Support specialists Resumes
- Oracle Developers Resumes
- SAP Resumes
- Web Developer Resumes
- Datawarehousing, ETL, Informatica Resumes
- Business Intelligence, Business Object Resumes
- MainFrame Resumes
- Network Admin Resumes
- Oracle Resumes
- ORACLE DBA Resumes
- Other Resumes
- Peoplesoft Resumes
- Project Manager Resumes
- Quality Assurance Resumes
- Recruiter Resumes
- SAS Resumes
- Sharepoint Resumes
- SQL Developers Resumes
- Technical Writers Resumes
- WebSphere Resumes
- Hot Resumes

Are you Hiring?
Talk to a Recruitment Specialist Call: (800) 693-8939

- Schedule A Meeting
- Franchise Opportunity
- LCA Posting Notices
- Hire IT Global, Inc - LCA Posting Notices
- Electronic PERM posting Notice
Client Services
- IT Staff Augmentation
- Hire Programmers
- Government Services
- IT Project Management
- Industry Expertise
- Resume Database
Job Seekers
- Browse Jobs
- Upload Resume
- Employee Benefits
- Resume Marketing
- Us Citizens/Green Cards
Visa Sponsorship
- Employer on Record Services (EOR)
- Forms Checklists
- H1B Visa Transfer
- E3 Visa Sponsorship
- TN Visa Sponsorship
- EB3 to EB2 Porting
- Green Card Sponsorship
- Tips & How-To
- Newsletters
- White Papers
- .NET Tips and Tricks
- The Data Science Lab
- Practical .NET
- The Practical Client
- Data Driver
- PDF Back Issues
- HTML Issue Archive
- Code Samples
- Agile/Scrum
- Open Source
- Cross-Platform C#
- Mobile Corner
- Live! Video
- Visual Studio
- Visual Studio Code
- Blazor/ASP.NET
- C#/VB/TypeScript
- Xamarin/Mobile
- AI/Machine Learning
Newly Open-Sourced '.NET Smart Components' Demoed at AI Event
- By David Ramel
Yesterday's .NET Conf Focus on AI online event highlighted Microsoft's latest/greatest AI dev tooling, including the newly open-sourced .NET Smart Components.
Debuting as an " experiment " earlier this year from Blazor creator Steve Sanderson, they are AI-infused ready-made components that devs can just drop into their projects to instantly supercharge UI with AI.
Sanderson announced they were open source a day before the event in a social media post viewed by some 16,000 people. He had long been pushing for the open sourcing , which was requested by many developers.
And yesterday they were highlighted by Scott Hanselman and Maria Naggaga during the opening keynote titled " State of .NET + AI ."
The duo explained the project in the context of an example shopping app, specifically showing how AI enhances text boxes used in a customer support scenario.
"They're not very sophisticated, right?" remarked Hanselman, VP of Developer Community. "Oftentimes, the support ticket page is just a bunch of text boxes that are not clever text boxes, and I just end up typing into them, and I presume that it generates some email on the back end. It's not a smart box."
When a Smart Component text box was used, they showed how its AI enhanced the experience with autocomplete functionality and the ability to take vague customer input and figure out what the user actually intended.
"And we did this with something called Smart Components," said Naggaga, senior program manager on the Visual Studio .NET team. "So Smart Components is like a UI AI element that we experimented with on the .NET team. So Steve Sanderson ... said, 'How would developers, how would consumers actually benefit from AI inside of their UI?' So this is the experience of it."
As the project's new GitHub repo shows, that experience comes in Smart Paste and Smart ComboBox in addition to the Smart TextArea that was demoed. Here they are in action:
"The .NET Smart Components are an experiment and are initially available for Blazor, MVC, and Razor Pages with .NET 6 and later," said Microsoft's Daniel Roth, principal product manager, ASP.NET, in announcing the AI-powered UI controls in March. "We expect to provide components for other .NET UI frameworks as well, like .NET MAUI, WPF, and Windows Forms, but first we're interested in your feedback on how useful these components are and what additional capabilities you'd like to see added."
Roth demoed more Smart Component functionality in his own session, " Build interactive AI-powered web apps with Blazor and .NET ," which invites devs to "Learn how you can quickly and easily build interactive AI-powered web apps with Blazor and .NET using a variety of ready-made AI components from the .NET ecosystem."
He continued: "Now we've made the code for the .NET Smart Components freely available as reference implementations. They're basically a bunch of samples so that they can help bootstrap an ecosystem of AI-enabled .NET components."
Roth also gave a shout-out to that ecosystem -- specifically third-party vendors who have come out with their own AI-infused UI constructs -- showing offerings from Progress Telerik, DevExpress and Syncfusion.
No doubt open sourcing will help bootstrap that ecosystem.
Both Roth's presentation and the keynote are available for replay , along with many more sessions and the entire livestream recording of the event, nearly 8-1/2 hours of video. Those two presentations, however, are by far and away the most-viewed videos, notching about 1,400 views each, with some 12,000 views for the entire event.
As a guide to what you might want to watch, Microsoft earlier provided this list of session highlights:
- Speaker: Stephen Toub
- Discover how easy it is to get started with AI in your .NET applications. This session will take you from a blank project to a fully integrated AI solution in no time.
- Speakers: Steve Sanderson, Matthew Bolanos
- Understand the synergy between .NET Aspire and Semantic Kernel for developing intelligent applications.
- Speakers: Dan Roth
- Learn how to create interactive AI-driven web applications using Blazor and .NET, including smart components and chatbot UI. We’ll also highlight a few AI-based demos from vendors in the .NET ecosystem.
- Speaker: Bruno Capuano
- Explore various AI models and learn how to integrate them into your .NET applications. This session will cover everything from local development to cloud deployment, using real-world scenarios.
- Speakers: Davide Mauri
- Learn how to build Retrieval Augmented Generation solutions on data stored in SQL Server or Azure SQL. This session will cover existing and future options, leveraging Azure SQL as a vector store.
- Speaker: Tim Spann
- Discover how Milvus leverages vector embeddings to enable powerful semantic search and similarity matching capabilities in .NET environments. Learn best practices and see practical examples of using Milvus in .NET applications.
- Speaker: Vin Kamat
- Hear real-world experiences from H&R Block on researching, developing, deploying, and improving AI assistants and applications using .NET and Azure.
About the Author
David Ramel is an editor and writer for Converge360.
Printable Format
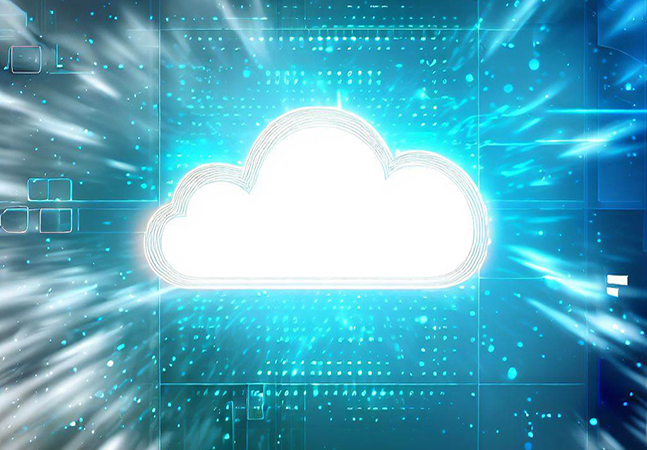
From Core to Containers to Orchestration: Modernizing Your Azure Compute
The cloud changed IT forever. And then containers changed the cloud. And then Kubernetes changed containers. And then microservices usurped monoliths, and so it goes in the cloudscape. Here's help to sort it all out.
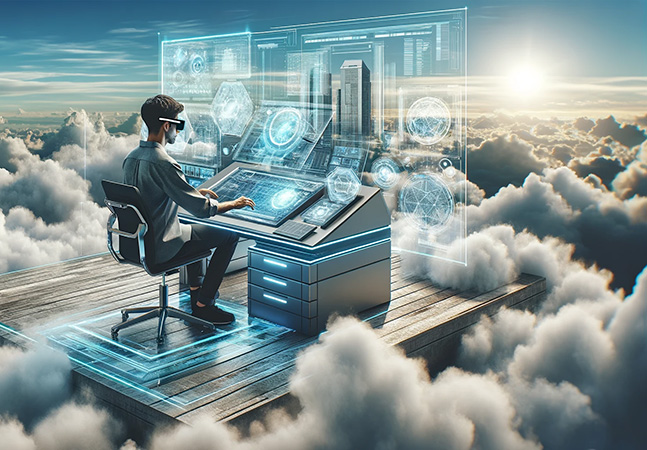
The Well-Architected Architect on Azure
In the dynamic field of cloud computing, the architect's role is increasingly pivotal as they must navigate a complex landscape, considering everything from the overarching architecture and individual service configurations to the various trade-offs involved. Here's help.
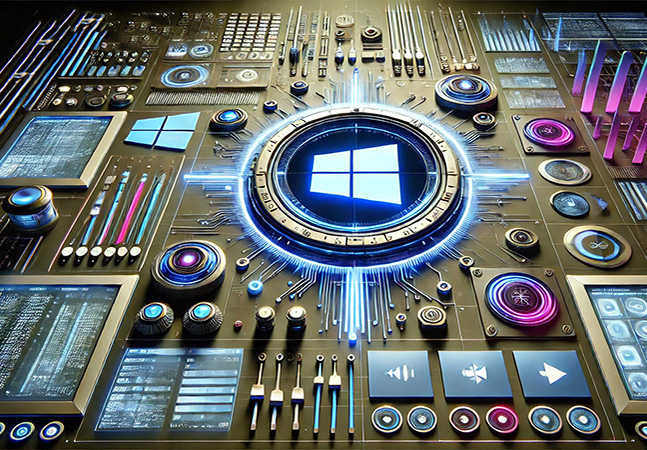
Windows Community Toolkit Update Improves Controls
The Windows Community Toolkit advanced to version 8.1, adding new features, improving existing controls and making dependency changes.
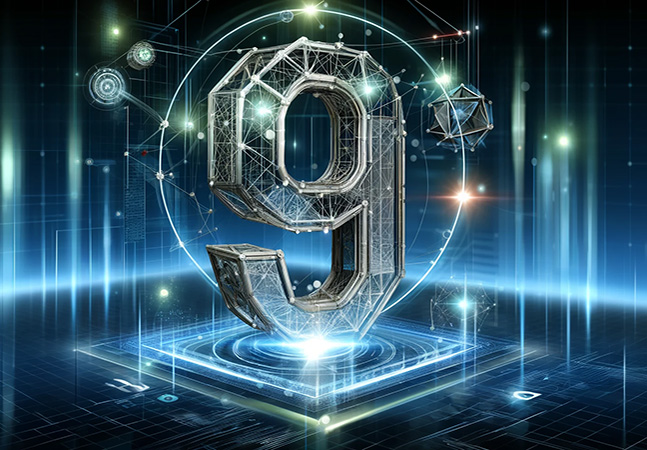
ASP.NET Core, .NET MAUI Updated as .NET 9 Nears
The web-dev ASP.NET Core framework and Xamarin.Forms' successor .NET MAUI received the lion's share of dev attention in the seventh preview of .NET 9 as Microsoft preps for a November launch at .NET Conf 2024.
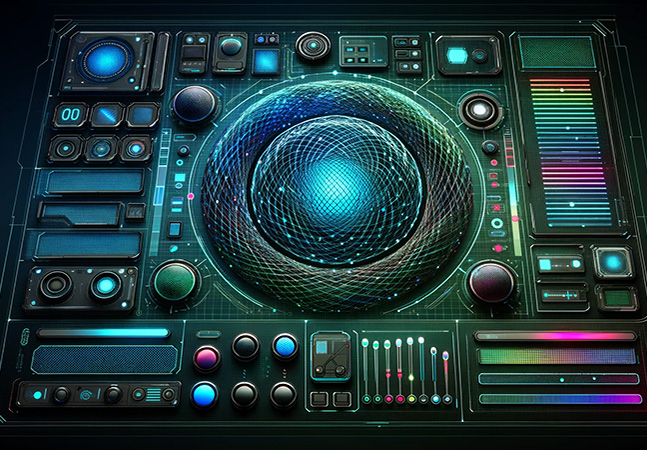
.NET Community Toolkit Gets Native AOT and .NET 8 Support
The .NET Community Toolkit is Microsoft's latest dev tooling to get native ahead-of-time compilation, continuing a years-long push for that capability across the board.
- Most Popular Articles
- Most Emailed Articles
Newly Open-Sourced '.NET Smart Components' Demoed at AI Event
Integrating blazor with existing .net web apps.
Subscribe on YouTube

Upcoming Training Events
Free Webcasts
- Myths and Realities in Telemetry Data Handling
- How .NET MAUI Changes the Cross-Platform Game Summit
- MoneyTree Achieves Compliance and Speeds Innovation with AWS and Sumo Logic
- Best Practices for AWS Monitoring
> More Webcasts
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
DTO definition in presentation layer and application layer
I am learning about DDD and I would like to say what is the correct way to implement DTO using DDD principles ?
In my onion architecture I implement a first DTO definition in presentation layer to map datas between RabbitMQViewModel(presentation layer) and RabbitMQModelsResultDTO (application layer).
And I implement a second DTO definition between application layer (RabbitMQModelsResultDTO) and domain layer (entity LogRabbitMQ).
However, I am not sure if it's a good way to implement two DTO definition ?
Light description of my DTO definition in presentation layer :
and application layer :
It's a screen of my project architecture :

- asp.net-core
- domain-driven-design
- onion-architecture
Translating presentation object ( RabbitMQViewModel ) into application object ( RabbitMQModelsResultDTO ) then application object into domain object ( LogRabbitMQ ) is considered to be a good practice in the DDD world.
However, it is pointless to make anemic, flat translations: it would be much easier to use the same object through all three layers (in which case DDD becomes irrelevant).
Here is how it can be done in the DDD way:
- Application object reflects domain context properties, namely value objects, into which presentation object's primitives are translated.
- Domain object is a "smart" object, implemented with all OOP goodies in order to keep it consistent all the time, and this object is being constructed using application object's properties.
As for the term "DTO", it should be used primarily for presentation object. It is less appropriate to use it in the context of application/domain objects, which are considered to be business objects.

- Ok thanks for help, I will take a look – Julien Martin Commented Nov 15, 2021 at 12:28
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged asp.net-core domain-driven-design layer dto onion-architecture or ask your own question .
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions
- Safety pictograms
- Using conditionals within \tl_put_right from latex3 explsyntax
- What is the meaning of "against" in "against such things there is no law" Galatians 5:23?
- What to do when 2 light switches are too far apart for the light switch cover plate?
- Distinctive form of "לאהוב ל-" instead of "לאהוב את"
- Book or novel about an intelligent monolith from space that crashes into a mountain
- Parody of Fables About Authenticity
- Searching for an illustrated children's book with anthropomorphic animals, a sled, and a train
- Is there a way to resist spells or abilities with an AOE coming from my teammates, or exclude certain beings from the effect?
- Why does a rolling ball slow down?
- How do eradicated diseases make a comeback?
- What is the name of this simulator
- Passport Carry in Taiwan
- How do we reconcile the story of the woman caught in adultery in John 8 and the man stoned for picking up sticks on Sabbath in Numbers 15?
- Why does a halfing's racial trait lucky specify you must use the next roll?
- Is this a new result about hexagon?
- What is an intuitive way to rename a column in a Dataset?
- Worth replacing greenboard for shower wall
- Manifest Mind vs Shatter
- Plotting orbitals on a lattice
- Could someone tell me what this part of an A320 is called in English?
- Correct Expression for Centripetal Force
- How to reply to reviewers who ask for more work by responding that the paper is complete as it stands?
- How much missing data is too much (part 2)? statistical power, effective sample size

IMAGES
VIDEO
COMMENTS
Presentation Layer (PL) The Presentation layer is the top-most layer of the 3-tier architecture, and its major role is to display the results to the user, or to put it another way, to present the data that we acquire from the business access layer and offer the results to the front-end user. Business Access Layer (BAL) The logic layer interacts ...
Business Logic layer:.NET Core class library; Presentation Layer: ASP.NET Core 5.0 Razor pages; The Business Objects layer includes objects that are used in the application and common helper functions (without logic) used for all layers. In a three-layer architecture, this layer is optional. However, as we follow the OOP with C#, we should ...
A new ASP.NET Core project, whether created in Visual Studio or from the command line, starts out as a simple "all-in-one" monolith. It contains all of the behavior of the application, including presentation, business, and data access logic. Figure 5-1 shows the file structure of a single-project app. Figure 5-1.
This layer is also called a Presentation. Presentation Layer contains the UI elements (pages, components) of the application. It handles the presentation (UI, API, etc.) concerns.
Clean Architecture is a popular approach to structuring your .NET application. It's a layered architecture and splits into four layers: Domain, Application, Infrastructure, and Presentation. ... The most important part of the Presentation layer is the Controllers, which define the API endpoints in our system. Here is the folder structure I like ...
This is the top-most layer of the application where the user performs their activity. Let's take the example of any application where the user needs to fill up a form. This form is nothing but the Presentation Layer. In Windows applications Windows Forms are the Presentation Layer and in web applications the web form belongs to the Presentation ...
Your solution will look as in the following: Step 2: Add a Business Logic Layer, Data Access Layer and Common Layer : Right-click the Business Logic Layer folder and add a C# class library project to it, call it BLL.csproj. Doing this will add a C# class library project to our solution in the Business Logic Layer Folder.
Presentation layer. This layer contains all the user interfaces of an application. In terms of ASP.NET Core, it contains MVC controllers, views, static files (images, HTML pages), JavaScript files, and other client-side frameworks like Angular, Knockout, and others. This is the frontend layer with which the user interacts.
The Presentation Layer can include various components, depending on the application's nature—web, desktop, mobile, or a combination thereof. In the world of .NET Core and ASP.NET, this often manifests as: ... In the Clean Architecture approach, particularly within the .NET Core and ASP.NET framework, understanding and effectively ...
The dependency rule will flow like: Infrastructure -> Presentation -> Application. Note that the dependency rule does not mean that you have to go through just an immediate underlying layer, rather it tells which other layers you can use and see from another. For example: Infrastructure -> Presentation -> Application does not mean that from ...
An API consumer / user can add a Customer via a SOAP / HTTP-POST to a web service which will call the Customer.Create () method. Imagine these layers: The presentation layer SOAP calls the Create () web method, which calls the facade's Create () method which calls the business object's Create () method which wires via the data access layer.
The presentation layer ensures the information that the application layer of one system sends out is readable by the application layer of another system. On the sending system it is responsible for conversion to standard, transmittable formats. [ 7] On the receiving system it is responsible for the translation, formatting, and delivery of ...
Prerequisite : OSI Model. Introduction : Presentation Layer is the 6th layer in the Open System Interconnection (OSI) model. This layer is also known as Translation layer, as this layer serves as a data translator for the network. The data which this layer receives from the Application Layer is extracted and manipulated here as per the required ...
Security, as the presentation layer, does not communicate directly with the data layer. 3 Layers Architecture Implementation Step by Step in .NET 6 The first layer, The Presentation Layer. The first layer is responsible to interact with our end user, and should not have any business logic validated here. Must be as simple as possible.
15. There is a big difference between the application layer and the presentation layer from a DDD view point. Although DDD centers around how to model the domain using the DDD building blocks and concepts such as bounded contexts, Ubiquitous language and so, it is still vital to clearly identify and separate the various layers in your app. The ...
Responsibilities of each layer or tier in an application: The Presentation Layer or Tier is usually responsible for interacting with the user. Business Layer or Tier is responsible for implementing the business logic of the application. The Data Access Layer or Tier is responsible for encapsulating the code that accesses the persistent data ...
Mô hình 3-layer gồm có 3 phần chính: - Presentation Layer (GUI) : Lớp này có nhiệm vụ chính giao tiếp với người dùng. Nó gồm các thành phần giao diện ( win form, web form,…) và thực hiện các công việc như nhập liệu, hiển thị dữ liêu, kiểm tra tính đúng đắn dữ liệu trước ...
Involved in designing and coding of Presentation Layer, Business Logic Layer and Data Access Layer. Connected with search engine to search in specific directories or subfolders. Developed database access service layer for the soap Web Services and integrated modules. Extensively used OOPS java concepts.
I have a 3-tier .NET 2.0 app. The presentation layer references the middle tier. The middle tier references the database layer. For some reason, when I compile, the dll's for the data layer appear ...
"The .NET Smart Components are an experiment and are initially available for Blazor, MVC, and Razor Pages with .NET 6 and later," said Microsoft's Daniel Roth, principal product manager, ASP.NET, in announcing the AI-powered UI controls in March. "We expect to provide components for other .NET UI frameworks as well, like .NET MAUI, WPF, and Windows Forms, but first we're interested in your ...
Domain object is a "smart" object, implemented with all OOP goodies in order to keep it consistent all the time, and this object is being constructed using application object's properties. As for the term "DTO", it should be used primarily for presentation object. It is less appropriate to use it in the context of application/domain objects ...